URL
https://opencores.org/ocsvn/z3/z3/trunk
Subversion Repositories z3
Compare Revisions
- This comparison shows the changes necessary to convert path
/z3
- from Rev 1 to Rev 2
- ↔ Reverse comparison
Rev 1 → Rev 2
/trunk/utils/screen.c
0,0 → 1,128
#include "SDL.h" |
#include <stdio.h> |
|
unsigned short myPixels[320*240]; |
|
int hex2num(char c) |
{ |
if (c>='0' && c<='9') |
return c-'0'; |
if (c>='a' && c<='f') |
return c-'a'+10; |
if (c>='A' && c<='F') |
return c-'A'+10; |
printf("Bad hex:%c\n", c); |
return 0; |
} |
|
int main(int argc, char* argv[]) |
{ |
FILE *f; |
SDL_Window *window; |
SDL_Renderer *ren; |
SDL_Texture *tex; |
unsigned short curReg=0; |
unsigned short curData=0; |
int curRecv=0; |
int curX=0; |
int curY=0; |
int curIdx=0; |
if (argc!=2) |
{ |
printf("Usage: screen.out simulation.log\n"); |
return 1; |
} |
f=fopen(argv[1],"r"); |
if (!f) |
{ |
printf("Couldn't open %s\n", argv[1]); |
return 1; |
} |
while (1) |
{ |
char line[1024]; |
char *start; |
if (!fgets(line, sizeof(line)-1, f)) |
{ |
break; |
} |
start=strchr(line, 'P'); |
if (start && start[1]=='E' && start[2]==':') |
{ |
int lcdWR=start[3]-'0'; |
int lcdCS=start[4]-'0'; |
int lcdReset=start[6]-'0'; |
if (lcdCS==0 && lcdReset==1 && lcdWR==0) |
{ |
int lcdRS=start[5]-'0'; |
char *data=strchr(start, 'D'); |
unsigned char d; |
if (data && data[1]==':') |
{ |
d=(hex2num(data[2])<<4)|hex2num(data[3]); |
} |
if (lcdRS==0) |
{ |
if (curRecv>=2) |
curRecv=0; |
curRecv++; |
curReg<<=8; |
curReg|=d; |
} |
else |
{ |
curRecv++; |
curData<<=8; |
curData|=d; |
if (curRecv>=4 && !(curRecv&1)) |
{ |
if (curReg==0x22) |
{ |
myPixels[curIdx%(320*240)]=curData; |
curIdx++; |
} |
else if (curReg==0x20) |
{ |
curX=curData; |
curIdx=curY*240+curX; |
} |
else if (curReg==0x21) |
{ |
curY=curData; |
curIdx=curY*240+curX; |
} |
} |
} |
} |
} |
} |
SDL_Init(SDL_INIT_VIDEO); |
window = SDL_CreateWindow("TFTLCD",SDL_WINDOWPOS_UNDEFINED,SDL_WINDOWPOS_UNDEFINED,240,320,SDL_WINDOW_SHOWN); |
if (window == NULL) |
{ |
printf("Could not create window: %s\n", SDL_GetError()); |
return 1; |
} |
ren = SDL_CreateRenderer(window, -1, SDL_RENDERER_ACCELERATED | SDL_RENDERER_PRESENTVSYNC); |
if (ren == NULL) |
{ |
printf("Could not create renderer: %s\n", SDL_GetError()); |
return 1; |
} |
tex = SDL_CreateTexture(ren, SDL_PIXELFORMAT_RGB565, SDL_TEXTUREACCESS_STREAMING, 240, 320); |
if (tex == NULL) |
{ |
printf("Could not create texture: %s\n", SDL_GetError()); |
return 1; |
} |
SDL_UpdateTexture(tex, NULL, myPixels, 240*sizeof(myPixels[0])); |
SDL_RenderClear(ren); |
SDL_RenderCopy(ren, tex, NULL, NULL); |
SDL_RenderPresent(ren); |
SDL_Delay(10000); |
SDL_DestroyTexture(tex); |
SDL_DestroyRenderer(ren); |
SDL_DestroyWindow(window); |
SDL_Quit(); |
return 0; |
} |
trunk/utils/screen.c
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/utils/gradient.h
===================================================================
--- trunk/utils/gradient.h (nonexistent)
+++ trunk/utils/gradient.h (revision 2)
@@ -0,0 +1,275 @@
+/* GIMP header image file format (INDEXED): /Users/charlie/code/verilog/Z2/gradient.h */
+
+static unsigned int width = 32;
+static unsigned int height = 1;
+
+/* Call this macro repeatedly. After each use, the pixel data can be extracted */
+
+#define HEADER_PIXEL(data,pixel) {\
+pixel[0] = header_data_cmap[(unsigned char)data[0]][0]; \
+pixel[1] = header_data_cmap[(unsigned char)data[0]][1]; \
+pixel[2] = header_data_cmap[(unsigned char)data[0]][2]; \
+data ++; }
+
+static char header_data_cmap[256][3] = {
+ { 0, 7,255},
+ { 0, 39,255},
+ {255, 0, 0},
+ { 0, 72,255},
+ {255, 30, 0},
+ { 0,105,255},
+ {255, 64, 0},
+ { 0,137,255},
+ {255, 96, 0},
+ { 0,170,255},
+ {255,129, 0},
+ { 0,203,255},
+ {255,162, 0},
+ { 0,255, 12},
+ { 0,255, 45},
+ { 20,255, 0},
+ { 0,236,255},
+ { 0,255, 78},
+ { 0,255,111},
+ { 0,255,143},
+ {255,195, 0},
+ { 54,255, 0},
+ { 0,255,176},
+ { 0,255,209},
+ { 0,255,242},
+ { 86,255, 0},
+ {119,255, 0},
+ {152,255, 0},
+ {255,227, 0},
+ {185,255, 0},
+ {217,255, 0},
+ {250,255, 0},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255}
+ };
+static char header_data[] = {
+ 2,4,6,8,10,12,20,28,31,30,29,27,26,25,21,15,
+ 13,14,17,18,19,22,23,24,16,11,9,7,5,3,1,0
+ };
trunk/utils/gradient.h
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/utils/16bitcolor.c
===================================================================
--- trunk/utils/16bitcolor.c (nonexistent)
+++ trunk/utils/16bitcolor.c (revision 2)
@@ -0,0 +1,22 @@
+#include
+
+unsigned short ToU16(unsigned long a)
+{
+ unsigned short out=(a>>8)&0xF800;
+ out|=(a>>5)&0x07E0;
+ out|=(a>>3)&0x001F;
+ return out;
+}
+
+int main(int argc, char **argv)
+{
+ int c;
+ if (argc!=2)
+ {
+ printf("Usage: 16bitcolor hexcolor\n");
+ return 1;
+ }
+ sscanf(argv[1], "%x", &c);
+ printf("0x%06x->0x%04x\n", c, ToU16(c));
+ return 0;
+}
trunk/utils/16bitcolor.c
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/utils/touchgenerator.c
===================================================================
--- trunk/utils/touchgenerator.c (nonexistent)
+++ trunk/utils/touchgenerator.c (revision 2)
@@ -0,0 +1,108 @@
+#include
+#include
+
+int touch[][3]={
+ { 'z', 200, 850 },
+ { 'x', 300, 850 },
+ { 'c', 400, 850 },
+ { 'v', 450, 850 },
+ { 'b', 550, 850 },
+ { 'n', 640, 850 },
+ { 'm', 720, 850 },
+ { ',', 800, 850 },
+ { 'a', 150, 800 },
+ { 's', 250, 800 },
+ { 'd', 320, 800 },
+ { 'f', 410, 800 },
+ { 'g', 500, 800 },
+ { 'h', 600, 800 },
+ { 'j', 700, 800 },
+ { 'k', 780, 800 },
+ { 'l', 850, 800 },
+ { 'q', 150, 750 },
+ { 'w', 200, 750 },
+ { 'e', 300, 750 },
+ { 'r', 400, 750 },
+ { 't', 450, 750 },
+ { 'y', 550, 750 },
+ { 'u', 650, 750 },
+ { 'i', 700, 750 },
+ { 'o', 800, 750 },
+ { 'p', 900, 750 },
+ { ' ', 500, 900 },
+ { 'Z', 200, 850 },
+ { 'X', 300, 850 },
+ { 'C', 400, 850 },
+ { 'V', 450, 850 },
+ { 'B', 550, 850 },
+ { 'N', 640, 850 },
+ { 'M', 720, 850 },
+ { 'A', 150, 800 },
+ { 'S', 250, 800 },
+ { 'D', 320, 800 },
+ { 'F', 410, 800 },
+ { 'G', 500, 800 },
+ { 'H', 600, 800 },
+ { 'J', 700, 800 },
+ { 'K', 780, 800 },
+ { 'L', 850, 800 },
+ { 'Q', 150, 750 },
+ { 'W', 200, 750 },
+ { 'E', 300, 750 },
+ { 'R', 400, 750 },
+ { 'T', 450, 750 },
+ { 'Y', 550, 750 },
+ { 'U', 650, 750 },
+ { 'I', 700, 750 },
+ { 'O', 800, 750 },
+ { 'P', 900, 750 },
+ { '\n',800, 900 }
+};
+
+int main(int argc, char **argv)
+{
+ FILE *f=fopen("script2.txt", "r");
+ while (!feof(f))
+ {
+ char line[1024];
+ fgets(line, sizeof(line), f);
+ int c=0;
+ while (line[c])
+ {
+ int x,y;
+ int z=1023;
+ int i;
+ for (i=0; i
trunk/utils/touchgenerator.c
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/utils/makegradient.c
===================================================================
--- trunk/utils/makegradient.c (nonexistent)
+++ trunk/utils/makegradient.c (revision 2)
@@ -0,0 +1,21 @@
+#include
+#include "gradient.h"
+
+int main(int argc, char **argv)
+{
+ int i=0;
+ while (i<32)
+ {
+ unsigned char r=header_data_cmap[header_data[i]][0];
+ unsigned char g=header_data_cmap[header_data[i]][1];
+ unsigned char b=header_data_cmap[header_data[i]][2];
+ printf("@db $%04x;", ((r&0xF8)<<8)|((g&0xFC)<<5)|(b>>3));
+ if ((i&3)==3)
+ printf("\n");
+ else
+ printf(" ");
+ i++;
+ }
+ return 0;
+}
+
trunk/utils/makegradient.c
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/utils/zops.c
===================================================================
--- trunk/utils/zops.c (nonexistent)
+++ trunk/utils/zops.c (revision 2)
@@ -0,0 +1,1646 @@
+#include "common.h"
+#include "file.h"
+#include "mem.h"
+#include "random.h"
+#include "stack.h"
+#include
+#include "SDL.h"
+
+#define MAX_TOKEN_LEN 256
+#define USE_BIOS 1
+
+u16 randomseed=1;
+
+enum
+{
+ SrcImmediate,
+ SrcVariable
+};
+
+enum
+{
+ Form0OP,
+ Form1OP,
+ Form2OP,
+ FormVAR
+};
+
+static int zeroOpStoreInstructions[]={};
+static int oneOpStoreInstructions[]={0x01,0x02,0x03,0x04,0x08,0x0E,0x0F};
+static int twoOpStoreInstructions[]={0x08,0x09,0x0F,0x10,0x11,0x12,0x13,0x14,0x15,0x16,0x17,0x18,0x19};
+static int varOpStoreInstructions[]={0x00,0x07,0x0C,0x16,0x17,0x18,0x1E};
+
+static int zeroOpBranchInstructions[]={0x05,0x06,0x0D};
+static int oneOpBranchInstructions[]={0x00,0x01,0x02};
+static int twoOpBranchInstructions[]={0x01,0x02,0x03,0x04,0x05,0x06,0x07,0x0A};
+static int varOpBranchInstructions[]={0x17};
+
+static char alphabetLookup[3][27]={
+ { 'a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z' },
+ { 'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z' },
+ { ' ', '\n','0', '1', '2', '3', '4', '5', '6', '7', '8', '9', '.', ',', '!', '?', '_', '#', '\'','"', '/', '\\','-', ':', '(', ')' },
+};
+
+typedef struct ZOperand_s
+{
+ int value;
+ int src;
+} ZOperand;
+
+typedef struct ZBranch_s
+{
+ int offset;
+ int negate;
+} ZBranch;
+
+typedef struct ZInstruction_s
+{
+ int op;
+ int form;
+ int store;
+ int numOps;
+ ZBranch branch;
+ ZOperand operands[4];
+} ZInstruction;
+
+typedef struct ZCallStack_s
+{
+ int returnAddr;
+ int returnStore;
+ int locals[16];
+ int depth;
+} ZCallStack;
+
+typedef struct ZObject_s
+{
+ int addr;
+ int propTable;
+} ZObject;
+
+typedef struct ZProperty_s
+{
+ int addr;
+ int size;
+ int bDefault;
+} ZProperty;
+
+typedef struct ZToken_s
+{
+ char token[MAX_TOKEN_LEN];
+ int offset;
+} ZToken;
+
+typedef struct ZDictEntry_s
+{
+ int coded[4];
+ int current;
+} ZDictEntry;
+
+typedef char byte;
+
+static ZInstruction m_ins;
+static int m_pc;
+static int m_globalVariables;
+static int m_abbrevTable;
+static int m_objectTable;
+static int m_dictionaryTable;
+static int m_memSize;
+static int m_memOffset;
+static byte *rom;
+static byte *memory;
+static byte *biosRAM;
+static u16 *screen;
+static int m_numberstack[1024];
+static stack m_stack;
+static ZCallStack m_callstackcontents[1024];
+static stack m_callStack;
+
+int forceDynamic=0;
+int curIdx=0;
+int curX=0;
+int curY=0;
+int winXMin=0;
+int winXMax=240-1;
+int winYMin=0;
+int winYMax=320-1;
+SDL_Window *window;
+SDL_Renderer *ren;
+SDL_Texture *tex;
+int mouseDown=0;
+int mouseX=0;
+int mouseY=0;
+
+byte ReadMem(int addr)
+{
+ return memory[addr+m_memOffset];
+}
+
+void WriteMem(int addr, byte val)
+{
+ memory[addr+m_memOffset]=val;
+}
+
+byte ReadMemDyn(int addr)
+{
+ if (addr>=0x10000 && forceDynamic)
+ return biosRAM[addr-0x10000];
+ return memory[addr+m_memOffset];
+}
+
+void displayState();
+
+void WriteMemDyn(int addr, byte val)
+{
+ if (addr>=0x10000)
+ {
+ if (1)//forceDynamic)
+ biosRAM[addr-0x10000]=val;
+ else
+ {
+ printf("Bad write:%x (PC:%x)\n", addr, m_pc);
+ displayState();
+ }
+ }
+ else
+ {
+ memory[addr+m_memOffset]=val;
+ }
+}
+
+int makeS16(int msb, int lsb)
+{
+ int ret=(msb<<8)+lsb;
+ if ((ret&0x8000)!=0)
+ {
+ ret+=-0x10000;
+ }
+ return ret;
+}
+
+int makeU16(int msb, int lsb)
+{
+ return (msb<<8)+lsb;
+}
+
+int readBytePC()
+{
+ return ReadMem(m_pc++)&0xFF;
+}
+
+int readS16PC()
+{
+ int msb=readBytePC();
+ int lsb=readBytePC();
+ return makeS16(msb, lsb);
+}
+
+int readVariable(int var)
+{
+ if (var==0)
+ {
+ return *(int*)stackPop(&m_stack);
+ }
+ if (var<16)
+ {
+ return ((ZCallStack*)stackPeek(&m_callStack))->locals[var-1];
+ }
+ int off=2*(var-16);
+ off+=m_globalVariables;
+ return makeS16(ReadMem(off)&0xFF, ReadMem(off+1)&0xFF);
+}
+
+void setVariable(int var, int value)
+{
+ value&=0xFFFF;
+ if ((value&0x8000)!=0)
+ {
+ value+=-0x10000;
+ }
+ if (var==0)
+ {
+ *(int*)stackPush(&m_stack)=value;
+ return;
+ }
+ if (var<16)
+ {
+ ((ZCallStack*)stackPeek(&m_callStack))->locals[var-1]=value;
+ return;
+ }
+ int off=2*(var-16);
+ off+=m_globalVariables;
+ WriteMem(off+0,(byte)((value&0xFF00)>>8));
+ WriteMem(off+1,(byte)((value&0x00FF)>>0));
+}
+
+void setVariableIndirect(int var, int value)
+{
+ if (var==0)
+ {
+ stackPop(&m_stack);
+ }
+ setVariable(var, value);
+}
+
+int readVariableIndirect(int var)
+{
+ int ret=readVariable(var);
+ if (var==0)
+ {
+ setVariable(var, ret);
+ }
+ return ret;
+}
+
+ZObject getObject(int id)
+{
+ ZObject ret;
+ ret.addr=m_objectTable+2*31+9*(id-1);
+ ret.propTable=makeU16(ReadMem(ret.addr+7)&0xFF, ReadMem(ret.addr+8)&0xFF);
+ return ret;
+}
+
+ZProperty getProperty(ZObject obj, int id)
+{
+ ZProperty ret;
+ int address=obj.propTable;
+ int textLen=ReadMem(address++)&0xFF;
+ address+=textLen*2;
+ while (ReadMem(address)!=0)
+ {
+ int sizeId=ReadMem(address++)&0xFF;
+ int size=1+(sizeId>>5);
+ int propId=sizeId&31;
+ if (propId==id)
+ {
+ ret.addr=address;
+ ret.size=size;
+ ret.bDefault=FALSE;
+ return ret;
+ }
+ address+=size;
+ }
+ ret.addr=(m_objectTable+(id-1)*2)&0xFFFF;
+ ret.size=2;
+ ret.bDefault=TRUE;
+ return ret;
+}
+
+void returnRoutine(int value)
+{
+ ZCallStack *cs=stackPop(&m_callStack);
+ while (cs->depthreturnStore>=0)
+ {
+ setVariable(cs->returnStore, value);
+ }
+ m_pc=cs->returnAddr;
+ if (cs->returnStore==-2)
+ {
+ returnRoutine(1);
+ }
+}
+
+void doBranch(int cond, ZBranch branch)
+{
+ if (branch.negate)
+ {
+ cond=!cond;
+ }
+ if (cond)
+ {
+ if (branch.offset==0)
+ returnRoutine(0);
+ else if (branch.offset==1)
+ returnRoutine(1);
+ else
+ m_pc+=branch.offset-2;
+ }
+}
+
+void readOperand(int operandType)
+{
+ if (operandType==3) //omitted
+ {
+ return;
+ }
+ ZOperand *operand = &m_ins.operands[m_ins.numOps++];
+ switch (operandType)
+ {
+ case 0: // long constant
+ operand->value = readS16PC();
+ operand->src = SrcImmediate;
+ break;
+ case 1: // small constant
+ operand->value = readBytePC();
+ operand->src = SrcImmediate;
+ break;
+ case 2: // variable
+ operand->value = readVariable(readBytePC());
+ operand->src = SrcVariable;
+ break;
+ }
+}
+
+void readShortForm(int opcode)
+{
+ int operand=(opcode>>4)&3;
+ int op=opcode&15;
+ m_ins.op=op;
+ if (operand==3)
+ m_ins.form=Form0OP;
+ else
+ m_ins.form=Form1OP;
+ readOperand(operand);
+}
+
+void readLongForm(int opcode)
+{
+ int op=opcode&31;
+ m_ins.op=op;
+ m_ins.form=Form2OP;
+ readOperand(((opcode&(1<<6))!=0)?2:1);
+ readOperand(((opcode&(1<<5))!=0)?2:1);
+}
+
+void readVariableForm(int opcode)
+{
+ int op=opcode&31;
+ int operandTypes=readBytePC();
+ int i;
+ m_ins.op=op;
+ if ((opcode&0xF0)>=0xE0)
+ m_ins.form=FormVAR;
+ else
+ m_ins.form=Form2OP;
+ for (i=3; i>=0; i--)
+ {
+ readOperand((operandTypes>>(2*i))&3);
+ }
+}
+
+int readStoreInstruction(int *match, int length, int op)
+{
+ int i;
+ for (i=0; i>2;
+ characters[1]=((pair1&3)<<3) + ((pair2&0xE0)>>5);
+ characters[2]=pair2&0x1F;
+ for (i=0; i<3; i++)
+ {
+ if (longNext>0)
+ {
+ longChar<<=5;
+ longChar&=0x3FF;
+ longChar|=characters[i];
+ longNext--;
+ if (longNext==0)
+ {
+ printf("%c", (char)longChar);
+ }
+ }
+ else if (!abbrNext)
+ {
+ if (characters[i]==6 && alphabet==2)
+ {
+ longNext=2;
+ }
+ else if (characters[i]>=6)
+ {
+ characters[i]-=6;
+ printf("%c", alphabetLookup[alphabet][characters[i]]);
+ alphabet=0;
+ }
+ else if (characters[i]==4)
+ {
+ alphabet=1;
+ }
+ else if (characters[i]==5)
+ {
+ alphabet=2;
+ }
+ else if (characters[i]==0)
+ {
+ printf(" ");
+ }
+ else
+ {
+ abbrChar=characters[i];
+ abbrNext=TRUE;
+ }
+ }
+ else
+ {
+ int idx=32*(abbrChar-1)+characters[i];
+ int abbrevTable=m_abbrevTable+2*idx;
+ int abbrevAddress=makeU16(ReadMem(abbrevTable)&0xFF, ReadMem(abbrevTable+1)&0xFF);
+ printText(2*abbrevAddress);
+ abbrNext=FALSE;
+ }
+ }
+ }
+ return address;
+}
+
+void removeObject(int childId)
+{
+ ZObject child=getObject(childId);
+ int parentId=ReadMem(child.addr+4)&0xFF;
+ if (parentId!=0)
+ {
+ ZObject parent=getObject(parentId);
+ if ((ReadMem(parent.addr+6)&0xFF)==childId)
+ {
+ WriteMem(parent.addr+6,ReadMem(child.addr+5)); // parent.child=child.sibling
+ }
+ else
+ {
+ int siblingId=ReadMem(parent.addr+6)&0xFF;
+ while (siblingId!=0)
+ {
+ ZObject sibling=getObject(siblingId);
+ int nextSiblingId=ReadMem(sibling.addr+5)&0xFF;
+ if (nextSiblingId==childId)
+ {
+ WriteMem(sibling.addr+5,ReadMem(child.addr+5)); // sibling.sibling=child.sibling
+ break;
+ }
+ siblingId=nextSiblingId;
+ }
+ if (siblingId==0)
+ {
+ illegalInstruction();
+ }
+ }
+ WriteMem(child.addr+4,0);
+ WriteMem(child.addr+5,0);
+ }
+}
+
+void addChild(int parentId, int childId)
+{
+ ZObject child=getObject(childId);
+ ZObject parent=getObject(parentId);
+ WriteMem(child.addr+5,ReadMem(parent.addr+6)); // child.sibling=parent.child
+ WriteMem(child.addr+4,(byte)parentId); // child.parent=parent
+ WriteMem(parent.addr+6,(byte)childId); // parent.child=child
+}
+
+void zDictInit(ZDictEntry *entry)
+{
+ entry->current=0;
+ entry->coded[0]=0;
+ entry->coded[1]=0;
+ entry->coded[2]=0x80;
+ entry->coded[3]=0;
+}
+
+void zDictAddCharacter(ZDictEntry *entry, int code)
+{
+ code&=31;
+ switch (entry->current)
+ {
+ case 0: entry->coded[0]|=code<<2; break;
+ case 1: entry->coded[0]|=code>>3; entry->coded[1]|=(code<<5)&0xFF; break;
+ case 2: entry->coded[1]|=code; break;
+ case 3: entry->coded[2]|=code<<2; break;
+ case 4: entry->coded[2]|=code>>3; entry->coded[3]|=(code<<5)&0xFF; break;
+ case 5: entry->coded[3]|=code; break;
+ }
+ entry->current++;
+}
+
+ZDictEntry encodeToken(char* token)
+{
+ ZDictEntry ret;
+ int tokenLen=strlen(token);
+ int t;
+ zDictInit(&ret);
+ for (t=0; t>5);
+ zDictAddCharacter(&ret, curChar&31);
+ }
+ else
+ {
+ if (alphabet>0)
+ {
+ int shift=alphabet+3;
+ zDictAddCharacter(&ret, shift);
+ }
+ zDictAddCharacter(&ret, code+6);
+ }
+ }
+ for (t=0; t<6; t++) // pad
+ {
+ zDictAddCharacter(&ret, 5);
+ }
+ return ret;
+}
+
+int getDictionaryAddress(char* token, int dictionary)
+{
+ int entryLength = ReadMem(dictionary++)&0xFF;
+ int numEntries = makeU16(ReadMem(dictionary+0)&0xFF, ReadMem(dictionary+1)&0xFF);
+ ZDictEntry zde = encodeToken(token);
+ int i;
+ dictionary+=2;
+ for (i=0; i>8)&0xFF));
+ WriteMem(parseBuffer++,(byte)((outAddress>>0)&0xFF));
+ WriteMem(parseBuffer++,(byte)strlen(tokens[i].token));
+ WriteMem(parseBuffer++,(byte)(tokens[i].offset+1));
+ }
+
+ return MIN(maxEntries, numTokens);
+}
+
+void restart()
+{
+ memcpy(memory, rom, m_memSize);
+ memset(biosRAM, 0, 0x10000);
+ ASSERT(ReadMem(0)==3);
+ m_globalVariables=makeU16(ReadMem(0xC)&0xFF, ReadMem(0xD)&0xFF);
+ m_abbrevTable=makeU16(ReadMem(0x18)&0xFF, ReadMem(0x19)&0xFF);
+ m_objectTable=makeU16(ReadMem(0xA)&0xFF, ReadMem(0xB)&0xFF);
+ m_dictionaryTable=makeU16(ReadMem(0x8)&0xFF, ReadMem(0x9)&0xFF);
+ m_pc=makeU16(ReadMem(6)&0xFF, ReadMem(7)&0xFF);
+ //WriteMem(1,ReadMem(1)|(1<<4)); // status line not available
+ //WriteMem(1,ReadMem(1)&~(1<<5)); // screen splitting available
+ //WriteMem(1,ReadMem(1)&~(1<<6)); // variable pitch font
+ //WriteMem(0x10,ReadMem(0x10)|(1<<0)); // transcripting
+ //WriteMem(0x10,ReadMem(0x10)|(1<<1)); // fixed font
+ stackInit(&m_stack, m_numberstack, sizeof(m_numberstack[0]), ARRAY_SIZEOF(m_numberstack));
+ stackInit(&m_callStack, m_callstackcontents, sizeof(m_callstackcontents[0]), ARRAY_SIZEOF(m_callstackcontents));
+#if USE_BIOS
+ callBIOS(0,FALSE);
+#endif
+}
+
+void process0OPInstruction()
+{
+ switch (m_ins.op)
+ {
+ case 0: //rtrue
+ returnRoutine(1);
+ break;
+ case 1: //rfalse
+ returnRoutine(0);
+ break;
+ case 2: //print
+ {
+#if !USE_BIOS
+ m_pc=printText(m_pc);
+#else
+ int origAddr=m_pc;
+ while (!(ReadMem(m_pc)&0x80))
+ {
+ m_pc+=2;
+ }
+ m_pc+=2;
+ m_ins.operands[1].value=origAddr&1;
+ m_ins.operands[2].value=origAddr>>1;
+ m_ins.numOps=3;
+ callBIOS(1,FALSE);
+#endif
+ break;
+ }
+ case 3: //print_ret
+ {
+#if !USE_BIOS
+ m_pc=printText(m_pc);
+ printf("\n");
+ returnRoutine(1);
+#else
+ int origAddr=m_pc;
+ while (!(ReadMem(m_pc)&0x80))
+ {
+ m_pc+=2;
+ }
+ m_pc+=2;
+ m_ins.operands[1].value=(origAddr&1)|2;
+ m_ins.operands[2].value=origAddr>>1;
+ m_ins.numOps=3;
+ callBIOS(1,TRUE);
+#endif
+ break;
+ }
+ case 4: //nop
+ break;
+ case 5: //save
+ doBranch(FALSE, m_ins.branch);
+ break;
+ case 6: //restore
+ doBranch(FALSE, m_ins.branch);
+ break;
+ case 7: //restart
+ restart();
+ break;
+ case 8: //ret_popped
+ returnRoutine(*(int*)stackPop(&m_stack));
+ break;
+ case 9: //pop
+ stackPop(&m_stack);
+ break;
+ case 0xA: //quit
+#if !USE_BIOS
+ haltInstruction();
+#else
+ callBIOS(6,FALSE);
+#endif
+ break;
+ case 0xB: //new_line
+#if !USE_BIOS
+ printf("\n");
+#else
+ m_ins.operands[1].value='\n';
+ m_ins.numOps=2;
+ callBIOS(2,FALSE);
+#endif
+ break;
+ case 0xC: //show_status
+#if !USE_BIOS
+ haltInstruction();
+#else
+ callBIOS(5,FALSE);
+#endif
+ break;
+ case 0xD: //verify
+ doBranch(TRUE, m_ins.branch);
+ break;
+ case 0xE: //extended
+ illegalInstruction();
+ break;
+ case 0xF: //piracy
+// doBranch(TRUE, m_ins.branch);
+ forceDynamic=1;
+ break;
+ }
+}
+
+void process1OPInstruction()
+{
+ switch (m_ins.op)
+ {
+ case 0: //jz
+ doBranch(m_ins.operands[0].value==0, m_ins.branch);
+ break;
+ case 1: //get_sibling
+ {
+ ZObject child=getObject(m_ins.operands[0].value);
+ int siblingId=ReadMem(child.addr+5)&0xFF;
+ setVariable(m_ins.store, siblingId);
+ doBranch(siblingId!=0, m_ins.branch);
+ break;
+ }
+ case 2: //get_child
+ {
+ ZObject child=getObject(m_ins.operands[0].value);
+ int childId=ReadMem(child.addr+6)&0xFF;
+ setVariable(m_ins.store, childId);
+ doBranch(childId!=0, m_ins.branch);
+ break;
+ }
+ case 3: //get_parent_object
+ {
+ ZObject child=getObject(m_ins.operands[0].value);
+ setVariable(m_ins.store, ReadMem(child.addr+4)&0xFF);
+ break;
+ }
+ case 4: //get_prop_len
+ {
+ int propAddress=(m_ins.operands[0].value&0xFFFF)-1;
+ int sizeId=ReadMem(propAddress)&0xFF;
+ int size=(sizeId>>5)+1;
+ setVariable(m_ins.store, size);
+ break;
+ }
+ case 5: //inc
+ {
+ int value=readVariable(m_ins.operands[0].value);
+ setVariable(m_ins.operands[0].value, value+1);
+ break;
+ }
+ case 6: //dec
+ {
+ int value=readVariable(m_ins.operands[0].value);
+ setVariable(m_ins.operands[0].value, value-1);
+ break;
+ }
+ case 7: //print_addr
+#if !USE_BIOS
+ printText(m_ins.operands[0].value);
+#else
+ m_ins.operands[1].value=m_ins.operands[0].value&1;
+ m_ins.operands[2].value=m_ins.operands[0].value>>1;
+ m_ins.numOps=3;
+ callBIOS(1,FALSE);
+#endif
+ break;
+ case 8: //call_1s
+ m_memOffset=(m_ins.operands[0].value&3)*0x20000;
+ restart();
+ break;
+ case 9: //remove_obj
+ {
+ removeObject(m_ins.operands[0].value);
+ break;
+ }
+ case 0xA: //print_obj
+ {
+#if !USE_BIOS
+ ZObject obj=getObject(m_ins.operands[0].value);
+ printText(obj.propTable+1);
+#else
+ ZObject obj=getObject(m_ins.operands[0].value);
+ m_ins.operands[1].value=(obj.propTable+1)&1;
+ m_ins.operands[2].value=(obj.propTable+1)>>1;
+ m_ins.numOps=3;
+ callBIOS(1,FALSE);
+#endif
+ break;
+ }
+ case 0xB: //ret
+ returnRoutine(m_ins.operands[0].value);
+ break;
+ case 0xC: //jump
+ m_pc+=m_ins.operands[0].value-2;
+ break;
+ case 0xD: //print_paddr
+#if !USE_BIOS
+ printText(2*(m_ins.operands[0].value&0xFFFF));
+#else
+ m_ins.operands[1].value=0;
+ m_ins.operands[2].value=m_ins.operands[0].value;
+ m_ins.numOps=3;
+ callBIOS(1,FALSE);
+#endif
+ break;
+ case 0xE: //load
+ setVariable(m_ins.store, readVariableIndirect(m_ins.operands[0].value));
+ break;
+ case 0xF: //not
+ setVariable(m_ins.store, ~m_ins.operands[0].value);
+ break;
+ }
+}
+
+void process2OPInstruction()
+{
+ switch (m_ins.op)
+ {
+ case 0:
+ illegalInstruction();
+ break;
+ case 1: //je
+ {
+ int takeBranch=FALSE;
+ int test=m_ins.operands[0].value;
+ int i;
+ for (i=1; im_ins.operands[1].value, m_ins.branch);
+ break;
+ case 4: //dec_chk
+ {
+ int value=readVariable(m_ins.operands[0].value);
+ value--;
+ setVariable(m_ins.operands[0].value, value);
+ doBranch(valuem_ins.operands[1].value, m_ins.branch);
+ break;
+ }
+ case 6: //jin
+ {
+ ZObject child=getObject(m_ins.operands[0].value);
+ doBranch((ReadMem(child.addr+4)&0xFF)==m_ins.operands[1].value, m_ins.branch);
+ break;
+ }
+ case 7: //test
+ {
+ int flags=m_ins.operands[1].value;
+ doBranch((m_ins.operands[0].value&flags)==flags, m_ins.branch);
+ break;
+ }
+ case 8: //or
+ setVariable(m_ins.store, m_ins.operands[0].value|m_ins.operands[1].value);
+ break;
+ case 9: //and
+ setVariable(m_ins.store, m_ins.operands[0].value&m_ins.operands[1].value);
+ break;
+ case 0xA: //test_attr
+ {
+ ZObject obj=getObject(m_ins.operands[0].value);
+ int attr=m_ins.operands[1].value;
+ int offset=attr/8;
+ int bit=0x80>>(attr%8);
+ doBranch((ReadMem(obj.addr+offset)&bit)==bit, m_ins.branch);
+ break;
+ }
+ case 0xB: //set_attr
+ {
+ ZObject obj=getObject(m_ins.operands[0].value);
+ int attr=m_ins.operands[1].value;
+ int offset=attr/8;
+ int bit=0x80>>(attr%8);
+ WriteMem(obj.addr+offset,ReadMem(obj.addr+offset)|bit);
+ break;
+ }
+ case 0xC: //clear_attr
+ {
+ ZObject obj=getObject(m_ins.operands[0].value);
+ int attr=m_ins.operands[1].value;
+ int offset=attr/8;
+ int bit=0x80>>(attr%8);
+ WriteMem(obj.addr+offset,ReadMem(obj.addr+offset)&~bit);
+ break;
+ }
+ case 0xD: //store
+ setVariableIndirect(m_ins.operands[0].value, m_ins.operands[1].value);
+ break;
+ case 0xE: //insert_obj
+ {
+ removeObject(m_ins.operands[0].value);
+ addChild(m_ins.operands[1].value, m_ins.operands[0].value);
+ break;
+ }
+ case 0xF: //loadw
+ {
+ int address=((m_ins.operands[0].value&0xFFFF)+2*(m_ins.operands[1].value&0xFFFF));
+ setVariable(m_ins.store, makeS16(ReadMemDyn(address)&0xFF, ReadMemDyn(address+1)&0xFF));
+ forceDynamic=0;
+ break;
+ }
+ case 0x10: //loadb
+ {
+ int address=((m_ins.operands[0].value&0xFFFF)+(m_ins.operands[1].value&0xFFFF));
+ setVariable(m_ins.store, ReadMemDyn(address)&0xFF);
+ forceDynamic=0;
+ break;
+ }
+ case 0x11: //get_prop
+ {
+ ZObject obj=getObject(m_ins.operands[0].value);
+ ZProperty prop=getProperty(obj, m_ins.operands[1].value);
+ if (prop.size==1)
+ {
+ setVariable(m_ins.store, ReadMem(prop.addr)&0xFF);
+ }
+ else if (prop.size==2)
+ {
+ setVariable(m_ins.store, makeS16(ReadMem(prop.addr)&0xFF, ReadMem(prop.addr+1)&0xFF));
+ }
+ else
+ {
+ illegalInstruction();
+ }
+ break;
+ }
+ case 0x12: //get_prop_addr
+ {
+ ZObject obj=getObject(m_ins.operands[0].value);
+ ZProperty prop=getProperty(obj, m_ins.operands[1].value);
+ if (prop.bDefault)
+ setVariable(m_ins.store, 0);
+ else
+ setVariable(m_ins.store, prop.addr);
+ break;
+ }
+ case 0x13: //get_next_prop
+ {
+ ZObject obj=getObject(m_ins.operands[0].value);
+ if (m_ins.operands[1].value==0)
+ {
+ int address=obj.propTable;
+ int textLen=ReadMem(address++)&0xFF;
+ address+=textLen*2;
+ int nextSizeId=ReadMem(address)&0xFF;
+ setVariable(m_ins.store, nextSizeId&31);
+ }
+ else
+ {
+ ZProperty prop=getProperty(obj, m_ins.operands[1].value);
+ if (prop.bDefault)
+ {
+ illegalInstruction();
+ }
+ else
+ {
+ int nextSizeId=ReadMem(prop.addr+prop.size)&0xFF;
+ setVariable(m_ins.store, nextSizeId&31);
+ }
+ }
+ break;
+ }
+ case 0x14: //add
+ setVariable(m_ins.store, m_ins.operands[0].value+m_ins.operands[1].value);
+ break;
+ case 0x15: //sub
+ setVariable(m_ins.store, m_ins.operands[0].value-m_ins.operands[1].value);
+ break;
+ case 0x16: //mul
+ setVariable(m_ins.store, m_ins.operands[0].value*m_ins.operands[1].value);
+ break;
+ case 0x17: //div
+ setVariable(m_ins.store, m_ins.operands[0].value/m_ins.operands[1].value);
+ break;
+ case 0x18: //mod
+ setVariable(m_ins.store, m_ins.operands[0].value%m_ins.operands[1].value);
+ break;
+ case 0x19: //call_2s
+ illegalInstruction();
+ break;
+ case 0x1A: //call_2n
+ illegalInstruction();
+ break;
+ case 0x1B: //set_colour
+ illegalInstruction();
+ break;
+ case 0x1C: //throw
+ illegalInstruction();
+ break;
+ case 0x1D:
+ illegalInstruction();
+ break;
+ case 0x1E:
+ //printf("WriteReg: %04x %04x\n", m_ins.operands[0].value&0xFFFF, m_ins.operands[1].value&0xFFFF);
+ if (m_ins.operands[0].value==0x22)
+ {
+ screen[curIdx%(320*240)]=m_ins.operands[1].value;
+ curIdx++;
+ curX++;
+ if (curX>winXMax)
+ {
+ curX=winXMin;
+ curY++;
+ if (curY>winYMax)
+ {
+ curY=winYMin;
+ }
+ curIdx=curY*240+curX;
+ }
+ }
+ else if (m_ins.operands[0].value==0x20)
+ {
+ curX=m_ins.operands[1].value;
+ curIdx=curY*240+curX;
+ }
+ else if (m_ins.operands[0].value==0x21)
+ {
+ curY=m_ins.operands[1].value;
+ curIdx=curY*240+curX;
+ }
+ else if (m_ins.operands[0].value==0x50)
+ {
+ winXMin=m_ins.operands[1].value;
+ }
+ else if (m_ins.operands[0].value==0x51)
+ {
+ winXMax=m_ins.operands[1].value;
+ }
+ else if (m_ins.operands[0].value==0x52)
+ {
+ winYMin=m_ins.operands[1].value;
+ }
+ else if (m_ins.operands[0].value==0x53)
+ {
+ winYMax=m_ins.operands[1].value;
+ }
+ else if (m_ins.operands[0].value==7)
+ {
+ SDL_UpdateTexture(tex, NULL, screen, 240*sizeof(screen[0]));
+ SDL_RenderClear(ren);
+ SDL_RenderCopy(ren, tex, NULL, NULL);
+ SDL_RenderPresent(ren);
+ SDL_Delay(1);
+ }
+ break;
+ case 0x1F:
+ illegalInstruction();
+ break;
+ }
+}
+
+void processVARInstruction()
+{
+ switch (m_ins.op)
+ {
+ case 0: // call
+ callRoutine(2*(m_ins.operands[0].value&0xFFFF), m_ins.store, TRUE);
+ break;
+ case 1: //storew
+ {
+ int address=((m_ins.operands[0].value&0xFFFF)+2*(m_ins.operands[1].value&0xFFFF));
+ int value=m_ins.operands[2].value;
+ WriteMemDyn(address,(byte)((value>>8)&0xFF));
+ WriteMemDyn(address+1,(byte)(value&0xFF));
+ forceDynamic=0;
+ break;
+ }
+ case 2: //storeb
+ {
+ int address=((m_ins.operands[0].value&0xFFFF)+(m_ins.operands[1].value&0xFFFF));
+ int value=m_ins.operands[2].value;
+ WriteMemDyn(address,(byte)(value&0xFF));
+ forceDynamic=0;
+ break;
+ }
+ case 3: //put_prop
+ {
+ ZObject obj=getObject(m_ins.operands[0].value);
+ ZProperty prop=getProperty(obj, m_ins.operands[1].value);
+ if (!prop.bDefault)
+ {
+ if (prop.size==1)
+ {
+ WriteMem(prop.addr,(byte)(m_ins.operands[2].value&0xFF));
+ }
+ else if (prop.size==2)
+ {
+ WriteMem(prop.addr+0,(byte)((m_ins.operands[2].value>>8)&0xFF));
+ WriteMem(prop.addr+1,(byte)(m_ins.operands[2].value&0xFF));
+ }
+ }
+ else
+ {
+ illegalInstruction();
+ }
+ break;
+ }
+ case 4: //sread
+ {
+#if !USE_BIOS
+ static char input[4096];
+ int bufferAddr=m_ins.operands[0].value;
+ int parseAddr=m_ins.operands[1].value;
+ int maxLength=ReadMem(bufferAddr++)&0xFF;
+ int maxParse=ReadMem(parseAddr++)&0xFF;
+ int realInLen=0;
+ int inLen;
+ int i;
+ fgets(input, sizeof(input), stdin);
+ inLen=strlen(input);
+ for (i=0; i0)
+ {
+ randomseed=randomseed^(randomseed<<13);
+ randomseed=randomseed^(randomseed>>9);
+ randomseed=randomseed^(randomseed<<7);
+ ret=((randomseed&0x7FFF)%(maxValue))+1;
+ }
+ else if (maxValue<0)
+ {
+ randomseed=maxValue;
+ }
+ setVariable(m_ins.store, ret);
+ break;
+ }
+ case 8: //push
+ setVariable(0, m_ins.operands[0].value);
+ break;
+ case 9: //pull
+ setVariableIndirect(m_ins.operands[0].value, readVariable(0));
+ break;
+ case 0xA: //split_window
+ haltInstruction();
+ break;
+ case 0xB: //set_window
+ haltInstruction();
+ break;
+ case 0xC: //call_vs2
+ illegalInstruction();
+ break;
+ case 0xD: //erase_window
+ illegalInstruction();
+ break;
+ case 0xE: //erase_line
+ illegalInstruction();
+ break;
+ case 0xF: //set_cursor
+ illegalInstruction();
+ break;
+ case 0x10: //get_cursor
+ illegalInstruction();
+ break;
+ case 0x11: //set_text_style
+ illegalInstruction();
+ break;
+ case 0x12: //buffer_mode
+ illegalInstruction();
+ break;
+ case 0x13: //output_stream
+ haltInstruction();
+ break;
+ case 0x14: //input_stream
+ haltInstruction();
+ break;
+ case 0x15: //sound_effect
+ haltInstruction();
+ break;
+ case 0x16: //read_char
+ illegalInstruction();
+ break;
+ case 0x17: //scan_table
+ illegalInstruction();
+ break;
+ case 0x18: //not
+ illegalInstruction();
+ break;
+ case 0x19: //call_vn
+ illegalInstruction();
+ break;
+ case 0x1A: //call_vn2
+ illegalInstruction();
+ break;
+ case 0x1B: //tokenise
+ illegalInstruction();
+ break;
+ case 0x1C: //encode_text
+ illegalInstruction();
+ break;
+ case 0x1D: //copy_table
+ illegalInstruction();
+ break;
+ case 0x1E: //print_table
+ {
+ // printf("GetTouch: %04x\n", m_ins.operands[0].value);
+ SDL_Event e;
+ while (SDL_PollEvent(&e))
+ {
+ if (e.type == SDL_QUIT)
+ exit(1);
+ else if (e.type == SDL_MOUSEBUTTONDOWN)
+ mouseDown=1;
+ else if (e.type == SDL_MOUSEBUTTONUP)
+ mouseDown=0;
+ else if (e.type == SDL_MOUSEMOTION)
+ {
+ SDL_MouseMotionEvent *mm=(SDL_MouseMotionEvent*)&e;
+ mouseX=mm->x;
+ mouseY=mm->y;
+ }
+ }
+ if (m_ins.operands[0].value==0x93) // touching?
+ setVariable(m_ins.store, mouseDown?0:1024);
+ else if (m_ins.operands[0].value==0x95) // X
+ {
+ float m=(840.0f-170.0f)/(216.0f-23.0f);
+ float c=840.0f-216.0f*m;
+ float v=mouseX*m+c;
+ setVariable(m_ins.store, (int)MIN(MAX(v,0),1023));
+ }
+ else if (m_ins.operands[0].value==0x1A) // Y
+ {
+ float m=(870.0f-720.0f)/(302.0f-243.0f);
+ float c=870.0f-302.0f*m;
+ float v=mouseY*m+c;
+ setVariable(m_ins.store, (int)MIN(MAX(v,0),1023));
+ }
+ else
+ setVariable(m_ins.store, 0);
+ break;
+ }
+ case 0x1F: //check_arg_count
+ {
+ int i;
+ //printf("Blit: %04x %04x %04x %04x\n", m_ins.operands[0].value&0xFFFF, m_ins.operands[1].value&0xFFFF, m_ins.operands[2].value&0xFFFF, m_ins.operands[3].value&0xFFFF);
+ forceDynamic=1;
+ for (i=0; i<(m_ins.operands[1].value&0xFFFF); i++)
+ {
+ byte data=ReadMemDyn(2*(m_ins.operands[0].value&0xFFFF)+i);
+ screen[curIdx%(320*240)]=(data&0x80)?m_ins.operands[3].value:m_ins.operands[2].value;
+ curIdx++;
+ screen[curIdx%(320*240)]=(data&0x40)?m_ins.operands[3].value:m_ins.operands[2].value;
+ curIdx++;
+ screen[curIdx%(320*240)]=(data&0x20)?m_ins.operands[3].value:m_ins.operands[2].value;
+ curIdx++;
+ screen[curIdx%(320*240)]=(data&0x10)?m_ins.operands[3].value:m_ins.operands[2].value;
+ curIdx++;
+ screen[curIdx%(320*240)]=(data&0x08)?m_ins.operands[3].value:m_ins.operands[2].value;
+ curIdx++;
+ screen[curIdx%(320*240)]=(data&0x04)?m_ins.operands[3].value:m_ins.operands[2].value;
+ curIdx++;
+ screen[curIdx%(320*240)]=(data&0x02)?m_ins.operands[3].value:m_ins.operands[2].value;
+ curIdx++;
+ screen[curIdx%(320*240)]=(data&0x01)?m_ins.operands[3].value:m_ins.operands[2].value;
+ curIdx++;
+ curX+=8;
+ if (curX>winXMax)
+ {
+ curX=winXMin;
+ curY++;
+ if (curY>winYMax)
+ {
+ curY=winYMin;
+ }
+ curIdx=curY*240+curX;
+ }
+ }
+ SDL_UpdateTexture(tex, NULL, screen, 240*sizeof(screen[0]));
+ SDL_RenderClear(ren);
+ SDL_RenderCopy(ren, tex, NULL, NULL);
+ SDL_RenderPresent(ren);
+ SDL_Delay(1);
+ forceDynamic=0;
+ break;
+ }
+ }
+}
+
+void executeInstruction()
+{
+ m_ins.numOps=0;
+ //printf("\nPC:%05x ", m_pc);
+ //System.out.println(String.format("%04x", m_pc));
+ int opcode=readBytePC();
+ if ((opcode&0xC0)==0xC0)
+ {
+ readVariableForm(opcode);
+ }
+ else if ((opcode&0xC0)==0x80)
+ {
+ readShortForm(opcode);
+ }
+ else
+ {
+ readLongForm(opcode);
+ }
+ switch (m_ins.form)
+ {
+ case Form0OP:
+ //printf("Doing op0:%2d\n", m_ins.op);
+ m_ins.store=readStoreInstruction(zeroOpStoreInstructions,ARRAY_SIZEOF(zeroOpStoreInstructions),m_ins.op);
+ m_ins.branch=readBranchInstruction(zeroOpBranchInstructions,ARRAY_SIZEOF(zeroOpBranchInstructions),m_ins.op);
+ //dumpCurrentInstruction();
+ process0OPInstruction();
+ break;
+ case Form1OP:
+ //printf("Doing op1:%2d Operands:%04x\n", m_ins.op, m_ins.operands[0].value&0xFFFF);
+ m_ins.store=readStoreInstruction(oneOpStoreInstructions,ARRAY_SIZEOF(oneOpStoreInstructions),m_ins.op);
+ m_ins.branch=readBranchInstruction(oneOpBranchInstructions,ARRAY_SIZEOF(oneOpBranchInstructions),m_ins.op);
+ //dumpCurrentInstruction();
+ process1OPInstruction();
+ break;
+ case Form2OP:
+ //printf("Doing op2:%2d Operands:%04x %04x\n", m_ins.op, m_ins.operands[0].value&0xFFFF, m_ins.operands[1].value&0xFFFF);
+ m_ins.store=readStoreInstruction(twoOpStoreInstructions,ARRAY_SIZEOF(twoOpStoreInstructions),m_ins.op);
+ m_ins.branch=readBranchInstruction(twoOpBranchInstructions,ARRAY_SIZEOF(twoOpBranchInstructions),m_ins.op);
+ //dumpCurrentInstruction();
+ process2OPInstruction();
+ break;
+ case FormVAR:
+ //if (m_ins.numOps==4)
+ // printf("Doing opvar:%2d Operands:%04x %04x %04x %04x\n", m_ins.op, m_ins.operands[0].value&0xFFFF, m_ins.operands[1].value&0xFFFF, m_ins.operands[2].value&0xFFFF, m_ins.operands[3].value&0xFFFF);
+ //else if (m_ins.numOps==3)
+ // printf("Doing opvar:%2d Operands:%04x %04x %04x\n", m_ins.op, m_ins.operands[0].value&0xFFFF, m_ins.operands[1].value&0xFFFF, m_ins.operands[2].value&0xFFFF);
+ //else if (m_ins.numOps==2)
+ // printf("Doing opvar:%2d Operands:%04x %04x\n", m_ins.op, m_ins.operands[0].value&0xFFFF, m_ins.operands[1].value&0xFFFF);
+ //else if (m_ins.numOps==1)
+ // printf("Doing opvar:%2d Operands:%04x\n", m_ins.op, m_ins.operands[0].value&0xFFFF);
+ //else
+ // printf("Doing opvar:%2d Operands:\n", m_ins.op);
+ m_ins.store=readStoreInstruction(varOpStoreInstructions,ARRAY_SIZEOF(varOpStoreInstructions),m_ins.op);
+ m_ins.branch=readBranchInstruction(varOpBranchInstructions,ARRAY_SIZEOF(varOpBranchInstructions),m_ins.op);
+ //dumpCurrentInstruction();
+ processVARInstruction();
+ break;
+ }
+}
+
+int main(int argc, char **argv)
+{
+ TFile fh;
+ if (argc!=2)
+ {
+ printf("Usage: zops game.z3\n");
+ }
+ else if (fileOpen(&fh, argv[1], TRUE))
+ {
+ m_memSize=fileSize(&fh);
+
+ SDL_Init(SDL_INIT_VIDEO);
+ window = SDL_CreateWindow("TFTLCD",SDL_WINDOWPOS_UNDEFINED,SDL_WINDOWPOS_UNDEFINED,240,320,SDL_WINDOW_SHOWN);
+ if (window == NULL)
+ {
+ printf("Could not create window: %s\n", SDL_GetError());
+ return 1;
+ }
+ ren = SDL_CreateRenderer(window, -1, SDL_RENDERER_ACCELERATED | SDL_RENDERER_PRESENTVSYNC);
+ if (ren == NULL)
+ {
+ printf("Could not create renderer: %s\n", SDL_GetError());
+ return 1;
+ }
+ tex = SDL_CreateTexture(ren, SDL_PIXELFORMAT_RGB565, SDL_TEXTUREACCESS_STREAMING, 240, 320);
+ if (tex == NULL)
+ {
+ printf("Could not create texture: %s\n", SDL_GetError());
+ return 1;
+ }
+
+ rom=memAlloc(m_memSize);
+ memory=memAlloc(m_memSize);
+ biosRAM=memAlloc(0x10000);
+ screen=memAlloc(320*240*sizeof(u16));
+ fileReadData(&fh, rom, m_memSize);
+ fileClose(&fh);
+ restart();
+ while (1)
+ {
+ executeInstruction();
+ }
+
+ SDL_DestroyTexture(tex);
+ SDL_DestroyRenderer(ren);
+ SDL_DestroyWindow(window);
+ SDL_Quit();
+ return 0;
+ }
+ return 1;
+}
trunk/utils/zops.c
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/gates.txt
===================================================================
--- trunk/gates.txt (nonexistent)
+++ trunk/gates.txt (revision 2)
@@ -0,0 +1,87 @@
+2785 (61%) - Procedual
+2146 (47%) - DoOp moved to state
+2082 (45%) - + Delayed assignment
+1508 (33%) - + Oper read assignment
+1531 (33%) - + Fix memStack stuff (ish)
+
+2145 (47%) - Delayed assignment fixes + added print + better testbed + data change oper
+24882 (540%) - Routines (32 deep) and more ops
+11460 (222%) - Routines (4 deep) => Stack needs moving into memory. On chip?
+4257 (92%) - Removed Routines
+2451 (53%) - Removed Routines + new ops
+2451 (53%) - Removed Routines + new ops
+2529 (45%) - + 0Ops
+4266 (93%) - + 2Ops
+3653 (79%) - + 2Ops (-LoadB/LoadW)
+2785 (60%) - + 2Ops (-IncChk)
+
+6476 (141%) - Enough ops to get to first input read
+266 - No DoOp logic (probably everything compiled out)
+1644 (36%) - + 0Ops
+3023 (66%) - + 1Ops
+4183 (91%) - + VarOps
+6669 (145%) - Back to full with fixed MOD instruction
+6597 (143%) - + Simple DoBranch instructions moved to state
+5460 (118%) - + Reduce number stack from 32->4 (FIXME!)
+4991 (108%) - + Remove MOD (not used)
+4626 (100%) - + Remove DIV (could do less efficiently?)
+
+3271 (71%) - Removed localRegs + stack from internal state (and -MOD) + StoreRegister now a state
+3657 - Removed memStack (+MOD)
+3332 (72%) - Removed memStack (-MOD) : Erm... why bigger?
+3222 (70%) - Reduce phase to 4 bits
+3202 (69%) - Remove temp2
+
+4034 (88%) - Printing system + All ops but (MOD/remove_obj/insert_obj)
+4503 (98%) - All ops except MOD
+
+5373 (117%) - Reset + full printing system (except print_num)
+5125 (111%) - Print state collapse
+5053 (110%) - Removed some duplicate driving
+4906 (106%) - Multicycle divide (and implements MOD)
+4910 (107%) - + Working random
+5081 (110%) - + Implemented print_num
+4538 (98%) - Without print/sread
+
+5012 (109%) - Full (with tweak)
+1022 (22%) - - All ops
+1711 (37%) - + 0Ops (~700)
+2945 (64%) - + 1Ops (~1200)
+4048 (88%) - + VarOps (~1100)
+5012 (109%) - + 2Ops (~1000)
+4651 (101%) - Full - 0Ops (~400)
+4202 (91%) - Full - 1Ops (~800)
+4048 (88%) - Full - 2Ops (~1000)
+4177 (91%) - Full - VarOps (~800)
+
+4741 (103%) - Full - Call (~250)
+4867 (106%) - Full - Call - AvoidReadFunction
+4974 (108%) - Full - StoreW/StoreB (~40)
+4931 (107%) - Full - PutProp (~80)
+4831 (105%) - Full - printchar/num (~180)
+4853 (105%) - Full - printnum (~140)
+4938 (107%) - Full - Random (~75)
+4937 (107%) - Full - Push/Pull (~75)
+
+4755 (103%) - Full (Modified call/read function states. Very suspicious!)
+4591 (100%) - Full - Load (~160)
+4569 (99%) - Full - RemoveObj (~190)
+4648 (101%) - Full (RemoveObj calls insert obj)
+4586 (100%) - Full (fussed Pull) <- Yay!
+
+4353 (94%) - Print/PrintChar/PrintNum BIOS
+4522 (98%) - Blit and writeReg ops
+4533 (98%) - + Added extra output pins (Tested and works!)
+4408 (96%) - Added default cases
+4475 (97%) - + Blit colour setting
+
+4463 (97%) - Passes Czech and Planetfall test
+4401 (96%) - Blit as arguments
+4406 (96%) - New relocatable BIOS
+
+4545 (99%) - Touch screen reading (maybe buggy)
+4548 (99%) - Reset switch (and working touch screen)
+4495 (98%) - Removed wasted cycle when fetching ops
+4550 (99%) - Removed wasted cycle when storing results on some ops
+4580 (99%) - Added last stored variable cache
+4555 (99%) - 1 cycle @dynamic, faster function calls + stream draw
trunk/gates.txt
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/LICENSE.md
===================================================================
--- trunk/LICENSE.md (nonexistent)
+++ trunk/LICENSE.md (revision 2)
@@ -0,0 +1,27 @@
+Copyright (c) 2014 Charles Cole
+All rights reserved.
+
+Redistribution and use in source and binary forms, with or without
+modification, are permitted provided that the following conditions are met:
+
+* Redistributions of source code must retain the above copyright notice, this
+ list of conditions and the following disclaimer.
+
+* Redistributions in binary form must reproduce the above copyright notice,
+ this list of conditions and the following disclaimer in the documentation
+ and/or other materials provided with the distribution.
+
+* Neither the name of Z3 nor the names of its
+ contributors may be used to endorse or promote products derived from
+ this software without specific prior written permission.
+
+THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
+AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
+IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
+DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
+FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
+DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
+SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
+CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
+OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
+OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
trunk/LICENSE.md
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/instructions.txt
===================================================================
--- trunk/instructions.txt (nonexistent)
+++ trunk/instructions.txt (revision 2)
@@ -0,0 +1,129 @@
+BUILDING THE BIOS
+-----------------
+
+TO BUILD PATCHED INFORM 6
+
+Clone the git repository of...
+https://github.com/charcole/Inform6.git
+
+git checkout inform6b
+clang -O3 *.c -o inform6b.out
+
+
+ASSEMBLE THE ROM
+
+Obtain 4 Z machine V3 games that are less than 120Kb. Update the roms array in
+combinedata.c to reference the games you are using. The first game in the
+array must be around 90Kb (like Zork I) as the icons are stored in this space.
+
+cd bios
+clang makefont.c -o makefont.out && ./makefont.out
+clang -O3 compressicon.c -o compressicon.out && ./compressicon.out
+clang -O3 combinedata.c -o combinedata.out && ./combinedata.out
+inform6b.out -v3 -t -l -y bios.inf
+
+To alter font, load zfont2.xcf in the GIMP. Alter the image as needed. Bottom
+row is for specifying how wide each character should be. Save image as
+zfont2.h. Recompile and run makefont.out.
+
+To alter icons, create an image 100x480 in the GIMP. Make indexed using 128
+colour palette. Save as icons.h. Recompile and run compressicon then
+combinedata. The compression ratio achieved must be better than around 60% to
+fit after Zork I.
+
+
+
+SIMULATING
+----------
+
+I used: Icarus-0.8.1-2005Sep12
+
+Make sure you'll built the BIOS and it's in the same directory you are going
+to run the Verilog simulation in.
+
+iverilog z3.vl && ./a.out
+
+If you want to run passed first request for input then touch.txt can be
+generated using TouchGenerator in the utilities. Using this a script can be
+provided which can be typed automatically during the simulation.
+
+To check screen output Screen (in utilties) can be run on the output from
+a.out. You may have to remove this section from the z3.vl...
+
+ if (b.operNum==`OP_VAR && b.op==`OPVAR_BLIT1 && b.phase==0 && b.state==`STATE_DO_OP) begin
+ b.operand[1]=1;
+ end
+
+Another way of checking output is to look at ram.dat. At the end it contains
+the scroll back buffer from the BIOS decoded and can be viewed in a text
+editor.
+
+
+
+RUNNING ON HARDWARE
+-------------------
+
+THE HARDWARE
+
+A "EP2C5 Cyclone II Mini Board" AKA "CycloneII EP2C5T144 Learning Board".
+These contain an EP2C5T144 which is an Cyclone II FPGA with around 4600 LEs.
+They are very reasonably priced on eBay (~$10).
+
+'2.4" TFT LCD Shield' by http://mcufriend.com was used as a screen. Again very
+cheap off eBay (~$3). It's a colour screen with resistive touch screen.
+
+RS Part No. Part No. Description Price
+7118029 A29L040-70F Parallel FLASH 4MB, 512Kx8, 3V, DIP32 £1.24
+7444542 AS6C1008-55PCN SRAM,1M,128Kx8,55ns,3V,Low Power,DIP32 £1.65
+6696064 MCP3008-I/P A/D Converter 10Bit 8-ch 2.7V SPI PDIP16 £1.53
+
+
+TO PROGRAM THE FPGA
+
+Obtain Altera Quantus II 13.0sp1. I believe this was the last version to
+support Cyclone II. Open project altera/ZMachine.qpf and hit compile.
+Nb. ZMachine.v is identical to z3.vl.
+
+
+TO PROGRAM THE ROM
+
+Using a Minipro TL866CS
+
+minipro -w rom.z3 -p "A29L040 @DIP32"
+
+
+
+OPTIONAL EXTRAS
+---------------
+
+TO BUILD THE BENCHMARK
+
+inform6.out -v3 -t -l -y benchmark.inf \$MAX_STATIC_DATA=20000
+
+Nb. This is the unpatched version of Inform 6.
+
+You can include this in as one of the games in combinedata.c.
+
+
+TO BUILD UTILITIES
+
+Z-Ops: High level emulator. Useful for working on the BIOS.
+clang -framework SDL2 -F/Users/charlie/Library/Frameworks
+-I/Users/charlie/Library/Frameworks/SDL2.framework/Headers -I../../lib -O3 -g zops.c ../../lib/cclib64.a -o zops.out
+
+16BitColor: Takes hex colours and turns them into 16 bit needed by screen
+eg. ./16bitcolor.out 0xffe1a2
+clang 16bitcolor.c -o 16bitcolor.out
+
+TouchGenerator: Takes script2.txt and generates a list of simulated touch data
+to type out the script. Used to automatically test the simulation.
+clang touchgenerator.c -o touchgenerator.out
+
+MakeGradient: Makes gradient for Mandelbrot generator easter egg. Image is
+from gradient.h saved from the GIMP as a header in indexed mode. Output needs
+pasting into bios.inf.
+clang -O3 makegradient.c -o makegradient.out && ./makegradient.out
+
+Screen: Takes the output from the simulator and draws the resultant screen
+output. Outdated now, use zops instead.
+clang -framework SDL2 -F/Users/charlie/Library/Frameworks -I/Users/charlie/Library/Frameworks/SDL2.framework/Headers screen.c -o screen.out
trunk/instructions.txt
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/altera/altpll0_bb.v
===================================================================
--- trunk/altera/altpll0_bb.v (nonexistent)
+++ trunk/altera/altpll0_bb.v (revision 2)
@@ -0,0 +1,202 @@
+// megafunction wizard: %ALTPLL%VBB%
+// GENERATION: STANDARD
+// VERSION: WM1.0
+// MODULE: altpll
+
+// ============================================================
+// File Name: altpll0.v
+// Megafunction Name(s):
+// altpll
+//
+// Simulation Library Files(s):
+// altera_mf
+// ============================================================
+// ************************************************************
+// THIS IS A WIZARD-GENERATED FILE. DO NOT EDIT THIS FILE!
+//
+// 13.0.1 Build 232 06/12/2013 SP 1 SJ Web Edition
+// ************************************************************
+
+//Copyright (C) 1991-2013 Altera Corporation
+//Your use of Altera Corporation's design tools, logic functions
+//and other software and tools, and its AMPP partner logic
+//functions, and any output files from any of the foregoing
+//(including device programming or simulation files), and any
+//associated documentation or information are expressly subject
+//to the terms and conditions of the Altera Program License
+//Subscription Agreement, Altera MegaCore Function License
+//Agreement, or other applicable license agreement, including,
+//without limitation, that your use is for the sole purpose of
+//programming logic devices manufactured by Altera and sold by
+//Altera or its authorized distributors. Please refer to the
+//applicable agreement for further details.
+
+module altpll0 (
+ inclk0,
+ c0,
+ locked);
+
+ input inclk0;
+ output c0;
+ output locked;
+
+endmodule
+
+// ============================================================
+// CNX file retrieval info
+// ============================================================
+// Retrieval info: PRIVATE: ACTIVECLK_CHECK STRING "0"
+// Retrieval info: PRIVATE: BANDWIDTH STRING "1.000"
+// Retrieval info: PRIVATE: BANDWIDTH_FEATURE_ENABLED STRING "0"
+// Retrieval info: PRIVATE: BANDWIDTH_FREQ_UNIT STRING "MHz"
+// Retrieval info: PRIVATE: BANDWIDTH_PRESET STRING "Low"
+// Retrieval info: PRIVATE: BANDWIDTH_USE_AUTO STRING "1"
+// Retrieval info: PRIVATE: BANDWIDTH_USE_CUSTOM STRING "0"
+// Retrieval info: PRIVATE: BANDWIDTH_USE_PRESET STRING "0"
+// Retrieval info: PRIVATE: CLKBAD_SWITCHOVER_CHECK STRING "0"
+// Retrieval info: PRIVATE: CLKLOSS_CHECK STRING "0"
+// Retrieval info: PRIVATE: CLKSWITCH_CHECK STRING "1"
+// Retrieval info: PRIVATE: CNX_NO_COMPENSATE_RADIO STRING "0"
+// Retrieval info: PRIVATE: CREATE_CLKBAD_CHECK STRING "0"
+// Retrieval info: PRIVATE: CREATE_INCLK1_CHECK STRING "0"
+// Retrieval info: PRIVATE: CUR_DEDICATED_CLK STRING "c0"
+// Retrieval info: PRIVATE: CUR_FBIN_CLK STRING "c0"
+// Retrieval info: PRIVATE: DEVICE_SPEED_GRADE STRING "8"
+// Retrieval info: PRIVATE: DIV_FACTOR0 NUMERIC "1"
+// Retrieval info: PRIVATE: DUTY_CYCLE0 STRING "50.00000000"
+// Retrieval info: PRIVATE: EFF_OUTPUT_FREQ_VALUE0 STRING "10.000000"
+// Retrieval info: PRIVATE: EXPLICIT_SWITCHOVER_COUNTER STRING "0"
+// Retrieval info: PRIVATE: EXT_FEEDBACK_RADIO STRING "0"
+// Retrieval info: PRIVATE: GLOCKED_COUNTER_EDIT_CHANGED STRING "1"
+// Retrieval info: PRIVATE: GLOCKED_FEATURE_ENABLED STRING "1"
+// Retrieval info: PRIVATE: GLOCKED_MODE_CHECK STRING "1"
+// Retrieval info: PRIVATE: GLOCK_COUNTER_EDIT NUMERIC "1048575"
+// Retrieval info: PRIVATE: HAS_MANUAL_SWITCHOVER STRING "1"
+// Retrieval info: PRIVATE: INCLK0_FREQ_EDIT STRING "50.000"
+// Retrieval info: PRIVATE: INCLK0_FREQ_UNIT_COMBO STRING "MHz"
+// Retrieval info: PRIVATE: INCLK1_FREQ_EDIT STRING "100.000"
+// Retrieval info: PRIVATE: INCLK1_FREQ_EDIT_CHANGED STRING "1"
+// Retrieval info: PRIVATE: INCLK1_FREQ_UNIT_CHANGED STRING "1"
+// Retrieval info: PRIVATE: INCLK1_FREQ_UNIT_COMBO STRING "MHz"
+// Retrieval info: PRIVATE: INTENDED_DEVICE_FAMILY STRING "Cyclone II"
+// Retrieval info: PRIVATE: INT_FEEDBACK__MODE_RADIO STRING "1"
+// Retrieval info: PRIVATE: LOCKED_OUTPUT_CHECK STRING "1"
+// Retrieval info: PRIVATE: LONG_SCAN_RADIO STRING "1"
+// Retrieval info: PRIVATE: LVDS_MODE_DATA_RATE STRING "Not Available"
+// Retrieval info: PRIVATE: LVDS_MODE_DATA_RATE_DIRTY NUMERIC "0"
+// Retrieval info: PRIVATE: LVDS_PHASE_SHIFT_UNIT0 STRING "deg"
+// Retrieval info: PRIVATE: MIG_DEVICE_SPEED_GRADE STRING "Any"
+// Retrieval info: PRIVATE: MIRROR_CLK0 STRING "0"
+// Retrieval info: PRIVATE: MULT_FACTOR0 NUMERIC "1"
+// Retrieval info: PRIVATE: NORMAL_MODE_RADIO STRING "1"
+// Retrieval info: PRIVATE: OUTPUT_FREQ0 STRING "10.00000000"
+// Retrieval info: PRIVATE: OUTPUT_FREQ_MODE0 STRING "1"
+// Retrieval info: PRIVATE: OUTPUT_FREQ_UNIT0 STRING "MHz"
+// Retrieval info: PRIVATE: PHASE_RECONFIG_FEATURE_ENABLED STRING "0"
+// Retrieval info: PRIVATE: PHASE_RECONFIG_INPUTS_CHECK STRING "0"
+// Retrieval info: PRIVATE: PHASE_SHIFT0 STRING "0.00000000"
+// Retrieval info: PRIVATE: PHASE_SHIFT_STEP_ENABLED_CHECK STRING "0"
+// Retrieval info: PRIVATE: PHASE_SHIFT_UNIT0 STRING "deg"
+// Retrieval info: PRIVATE: PLL_ADVANCED_PARAM_CHECK STRING "0"
+// Retrieval info: PRIVATE: PLL_ARESET_CHECK STRING "0"
+// Retrieval info: PRIVATE: PLL_AUTOPLL_CHECK NUMERIC "1"
+// Retrieval info: PRIVATE: PLL_ENA_CHECK STRING "0"
+// Retrieval info: PRIVATE: PLL_ENHPLL_CHECK NUMERIC "0"
+// Retrieval info: PRIVATE: PLL_FASTPLL_CHECK NUMERIC "0"
+// Retrieval info: PRIVATE: PLL_FBMIMIC_CHECK STRING "0"
+// Retrieval info: PRIVATE: PLL_LVDS_PLL_CHECK NUMERIC "0"
+// Retrieval info: PRIVATE: PLL_PFDENA_CHECK STRING "0"
+// Retrieval info: PRIVATE: PLL_TARGET_HARCOPY_CHECK NUMERIC "0"
+// Retrieval info: PRIVATE: PRIMARY_CLK_COMBO STRING "inclk0"
+// Retrieval info: PRIVATE: RECONFIG_FILE STRING "altpll0.mif"
+// Retrieval info: PRIVATE: SACN_INPUTS_CHECK STRING "0"
+// Retrieval info: PRIVATE: SCAN_FEATURE_ENABLED STRING "0"
+// Retrieval info: PRIVATE: SELF_RESET_LOCK_LOSS STRING "0"
+// Retrieval info: PRIVATE: SHORT_SCAN_RADIO STRING "0"
+// Retrieval info: PRIVATE: SPREAD_FEATURE_ENABLED STRING "0"
+// Retrieval info: PRIVATE: SPREAD_FREQ STRING "50.000"
+// Retrieval info: PRIVATE: SPREAD_FREQ_UNIT STRING "KHz"
+// Retrieval info: PRIVATE: SPREAD_PERCENT STRING "0.500"
+// Retrieval info: PRIVATE: SPREAD_USE STRING "0"
+// Retrieval info: PRIVATE: SRC_SYNCH_COMP_RADIO STRING "0"
+// Retrieval info: PRIVATE: STICKY_CLK0 STRING "1"
+// Retrieval info: PRIVATE: SWITCHOVER_COUNT_EDIT NUMERIC "1"
+// Retrieval info: PRIVATE: SWITCHOVER_FEATURE_ENABLED STRING "1"
+// Retrieval info: PRIVATE: SYNTH_WRAPPER_GEN_POSTFIX STRING "0"
+// Retrieval info: PRIVATE: USE_CLK0 STRING "1"
+// Retrieval info: PRIVATE: USE_CLKENA0 STRING "0"
+// Retrieval info: PRIVATE: USE_MIL_SPEED_GRADE NUMERIC "0"
+// Retrieval info: PRIVATE: ZERO_DELAY_RADIO STRING "0"
+// Retrieval info: LIBRARY: altera_mf altera_mf.altera_mf_components.all
+// Retrieval info: CONSTANT: CLK0_DIVIDE_BY NUMERIC "5"
+// Retrieval info: CONSTANT: CLK0_DUTY_CYCLE NUMERIC "50"
+// Retrieval info: CONSTANT: CLK0_MULTIPLY_BY NUMERIC "1"
+// Retrieval info: CONSTANT: CLK0_PHASE_SHIFT STRING "0"
+// Retrieval info: CONSTANT: COMPENSATE_CLOCK STRING "CLK0"
+// Retrieval info: CONSTANT: GATE_LOCK_COUNTER NUMERIC "1048575"
+// Retrieval info: CONSTANT: GATE_LOCK_SIGNAL STRING "YES"
+// Retrieval info: CONSTANT: INCLK0_INPUT_FREQUENCY NUMERIC "20000"
+// Retrieval info: CONSTANT: INTENDED_DEVICE_FAMILY STRING "Cyclone II"
+// Retrieval info: CONSTANT: INVALID_LOCK_MULTIPLIER NUMERIC "5"
+// Retrieval info: CONSTANT: LPM_TYPE STRING "altpll"
+// Retrieval info: CONSTANT: OPERATION_MODE STRING "NORMAL"
+// Retrieval info: CONSTANT: PORT_ACTIVECLOCK STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_ARESET STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_CLKBAD0 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_CLKBAD1 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_CLKLOSS STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_CLKSWITCH STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_CONFIGUPDATE STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_FBIN STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_INCLK0 STRING "PORT_USED"
+// Retrieval info: CONSTANT: PORT_INCLK1 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_LOCKED STRING "PORT_USED"
+// Retrieval info: CONSTANT: PORT_PFDENA STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_PHASECOUNTERSELECT STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_PHASEDONE STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_PHASESTEP STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_PHASEUPDOWN STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_PLLENA STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_SCANACLR STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_SCANCLK STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_SCANCLKENA STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_SCANDATA STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_SCANDATAOUT STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_SCANDONE STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_SCANREAD STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_SCANWRITE STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clk0 STRING "PORT_USED"
+// Retrieval info: CONSTANT: PORT_clk1 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clk2 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clk3 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clk4 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clk5 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clkena0 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clkena1 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clkena2 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clkena3 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clkena4 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clkena5 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_extclk0 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_extclk1 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_extclk2 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_extclk3 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: VALID_LOCK_MULTIPLIER NUMERIC "1"
+// Retrieval info: USED_PORT: @clk 0 0 6 0 OUTPUT_CLK_EXT VCC "@clk[5..0]"
+// Retrieval info: USED_PORT: @extclk 0 0 4 0 OUTPUT_CLK_EXT VCC "@extclk[3..0]"
+// Retrieval info: USED_PORT: c0 0 0 0 0 OUTPUT_CLK_EXT VCC "c0"
+// Retrieval info: USED_PORT: inclk0 0 0 0 0 INPUT_CLK_EXT GND "inclk0"
+// Retrieval info: USED_PORT: locked 0 0 0 0 OUTPUT GND "locked"
+// Retrieval info: CONNECT: @inclk 0 0 1 1 GND 0 0 0 0
+// Retrieval info: CONNECT: @inclk 0 0 1 0 inclk0 0 0 0 0
+// Retrieval info: CONNECT: c0 0 0 0 0 @clk 0 0 1 0
+// Retrieval info: CONNECT: locked 0 0 0 0 @locked 0 0 0 0
+// Retrieval info: GEN_FILE: TYPE_NORMAL altpll0.v TRUE
+// Retrieval info: GEN_FILE: TYPE_NORMAL altpll0.ppf TRUE
+// Retrieval info: GEN_FILE: TYPE_NORMAL altpll0.inc FALSE
+// Retrieval info: GEN_FILE: TYPE_NORMAL altpll0.cmp FALSE
+// Retrieval info: GEN_FILE: TYPE_NORMAL altpll0.bsf TRUE
+// Retrieval info: GEN_FILE: TYPE_NORMAL altpll0_inst.v FALSE
+// Retrieval info: GEN_FILE: TYPE_NORMAL altpll0_bb.v TRUE
+// Retrieval info: LIB_FILE: altera_mf
+// Retrieval info: CBX_MODULE_PREFIX: ON
trunk/altera/altpll0_bb.v
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/altera/boss.bsf
===================================================================
--- trunk/altera/boss.bsf (nonexistent)
+++ trunk/altera/boss.bsf (revision 2)
@@ -0,0 +1,155 @@
+/*
+WARNING: Do NOT edit the input and output ports in this file in a text
+editor if you plan to continue editing the block that represents it in
+the Block Editor! File corruption is VERY likely to occur.
+*/
+/*
+Copyright (C) 1991-2013 Altera Corporation
+Your use of Altera Corporation's design tools, logic functions
+and other software and tools, and its AMPP partner logic
+functions, and any output files from any of the foregoing
+(including device programming or simulation files), and any
+associated documentation or information are expressly subject
+to the terms and conditions of the Altera Program License
+Subscription Agreement, Altera MegaCore Function License
+Agreement, or other applicable license agreement, including,
+without limitation, that your use is for the sole purpose of
+programming logic devices manufactured by Altera and sold by
+Altera or its authorized distributors. Please refer to the
+applicable agreement for further details.
+*/
+(header "symbol" (version "1.1"))
+(symbol
+ (rect 16 16 216 320)
+ (text "boss" (rect 5 0 23 12)(font "Arial" ))
+ (text "inst" (rect 8 288 20 300)(font "Arial" ))
+ (port
+ (pt 0 32)
+ (input)
+ (text "clk" (rect 0 0 10 12)(font "Arial" ))
+ (text "clk" (rect 21 27 31 39)(font "Arial" ))
+ (line (pt 0 32)(pt 16 32)(line_width 1))
+ )
+ (port
+ (pt 0 48)
+ (input)
+ (text "adcDout" (rect 0 0 33 12)(font "Arial" ))
+ (text "adcDout" (rect 21 43 54 55)(font "Arial" ))
+ (line (pt 0 48)(pt 16 48)(line_width 1))
+ )
+ (port
+ (pt 0 64)
+ (input)
+ (text "reset" (rect 0 0 20 12)(font "Arial" ))
+ (text "reset" (rect 21 59 41 71)(font "Arial" ))
+ (line (pt 0 64)(pt 16 64)(line_width 1))
+ )
+ (port
+ (pt 200 48)
+ (output)
+ (text "waddress[16..0]" (rect 0 0 61 12)(font "Arial" ))
+ (text "waddress[16..0]" (rect 118 43 179 55)(font "Arial" ))
+ (line (pt 200 48)(pt 184 48)(line_width 3))
+ )
+ (port
+ (pt 200 64)
+ (output)
+ (text "we" (rect 0 0 10 12)(font "Arial" ))
+ (text "we" (rect 169 59 179 71)(font "Arial" ))
+ (line (pt 200 64)(pt 184 64)(line_width 1))
+ )
+ (port
+ (pt 200 80)
+ (output)
+ (text "pe" (rect 0 0 9 12)(font "Arial" ))
+ (text "pe" (rect 170 75 179 87)(font "Arial" ))
+ (line (pt 200 80)(pt 184 80)(line_width 1))
+ )
+ (port
+ (pt 200 96)
+ (output)
+ (text "romCS" (rect 0 0 29 12)(font "Arial" ))
+ (text "romCS" (rect 150 91 179 103)(font "Arial" ))
+ (line (pt 200 96)(pt 184 96)(line_width 1))
+ )
+ (port
+ (pt 200 112)
+ (output)
+ (text "ramCS" (rect 0 0 29 12)(font "Arial" ))
+ (text "ramCS" (rect 150 107 179 119)(font "Arial" ))
+ (line (pt 200 112)(pt 184 112)(line_width 1))
+ )
+ (port
+ (pt 200 128)
+ (output)
+ (text "led0" (rect 0 0 15 12)(font "Arial" ))
+ (text "led0" (rect 164 123 179 135)(font "Arial" ))
+ (line (pt 200 128)(pt 184 128)(line_width 1))
+ )
+ (port
+ (pt 200 144)
+ (output)
+ (text "led1" (rect 0 0 14 12)(font "Arial" ))
+ (text "led1" (rect 165 139 179 151)(font "Arial" ))
+ (line (pt 200 144)(pt 184 144)(line_width 1))
+ )
+ (port
+ (pt 200 160)
+ (output)
+ (text "led2" (rect 0 0 15 12)(font "Arial" ))
+ (text "led2" (rect 164 155 179 167)(font "Arial" ))
+ (line (pt 200 160)(pt 184 160)(line_width 1))
+ )
+ (port
+ (pt 200 176)
+ (output)
+ (text "lcdCS" (rect 0 0 23 12)(font "Arial" ))
+ (text "lcdCS" (rect 156 171 179 183)(font "Arial" ))
+ (line (pt 200 176)(pt 184 176)(line_width 1))
+ )
+ (port
+ (pt 200 192)
+ (output)
+ (text "lcdRS" (rect 0 0 24 12)(font "Arial" ))
+ (text "lcdRS" (rect 155 187 179 199)(font "Arial" ))
+ (line (pt 200 192)(pt 184 192)(line_width 1))
+ )
+ (port
+ (pt 200 208)
+ (output)
+ (text "lcdReset" (rect 0 0 35 12)(font "Arial" ))
+ (text "lcdReset" (rect 144 203 179 215)(font "Arial" ))
+ (line (pt 200 208)(pt 184 208)(line_width 1))
+ )
+ (port
+ (pt 200 224)
+ (output)
+ (text "nadcCS" (rect 0 0 31 12)(font "Arial" ))
+ (text "nadcCS" (rect 148 219 179 231)(font "Arial" ))
+ (line (pt 200 224)(pt 184 224)(line_width 1))
+ )
+ (port
+ (pt 200 240)
+ (output)
+ (text "a17" (rect 0 0 12 12)(font "Arial" ))
+ (text "a17" (rect 167 235 179 247)(font "Arial" ))
+ (line (pt 200 240)(pt 184 240)(line_width 1))
+ )
+ (port
+ (pt 200 256)
+ (output)
+ (text "a18" (rect 0 0 12 12)(font "Arial" ))
+ (text "a18" (rect 167 251 179 263)(font "Arial" ))
+ (line (pt 200 256)(pt 184 256)(line_width 1))
+ )
+ (port
+ (pt 200 32)
+ (bidir)
+ (text "data[7..0]" (rect 0 0 36 12)(font "Arial" ))
+ (text "data[7..0]" (rect 143 27 179 39)(font "Arial" ))
+ (line (pt 200 32)(pt 184 32)(line_width 3))
+ )
+ (drawing
+ (rectangle (rect 16 16 184 288)(line_width 1))
+ )
+)
trunk/altera/boss.bsf
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/altera/altpll0.qip
===================================================================
--- trunk/altera/altpll0.qip (nonexistent)
+++ trunk/altera/altpll0.qip (revision 2)
@@ -0,0 +1,6 @@
+set_global_assignment -name IP_TOOL_NAME "ALTPLL"
+set_global_assignment -name IP_TOOL_VERSION "13.0"
+set_global_assignment -name VERILOG_FILE [file join $::quartus(qip_path) "altpll0.v"]
+set_global_assignment -name MISC_FILE [file join $::quartus(qip_path) "altpll0.bsf"]
+set_global_assignment -name MISC_FILE [file join $::quartus(qip_path) "altpll0_bb.v"]
+set_global_assignment -name MISC_FILE [file join $::quartus(qip_path) "altpll0.ppf"]
trunk/altera/altpll0.qip
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/altera/altpll0.bsf
===================================================================
--- trunk/altera/altpll0.bsf (nonexistent)
+++ trunk/altera/altpll0.bsf (revision 2)
@@ -0,0 +1,76 @@
+/*
+WARNING: Do NOT edit the input and output ports in this file in a text
+editor if you plan to continue editing the block that represents it in
+the Block Editor! File corruption is VERY likely to occur.
+*/
+/*
+Copyright (C) 1991-2013 Altera Corporation
+Your use of Altera Corporation's design tools, logic functions
+and other software and tools, and its AMPP partner logic
+functions, and any output files from any of the foregoing
+(including device programming or simulation files), and any
+associated documentation or information are expressly subject
+to the terms and conditions of the Altera Program License
+Subscription Agreement, Altera MegaCore Function License
+Agreement, or other applicable license agreement, including,
+without limitation, that your use is for the sole purpose of
+programming logic devices manufactured by Altera and sold by
+Altera or its authorized distributors. Please refer to the
+applicable agreement for further details.
+*/
+(header "symbol" (version "1.2"))
+(symbol
+ (rect 0 0 256 152)
+ (text "altpll0" (rect 111 0 153 16)(font "Arial" (font_size 10)))
+ (text "inst" (rect 8 137 26 148)(font "Arial" ))
+ (port
+ (pt 0 64)
+ (input)
+ (text "inclk0" (rect 0 0 34 13)(font "Arial" (font_size 8)))
+ (text "inclk0" (rect 4 51 31 63)(font "Arial" (font_size 8)))
+ (line (pt 0 64)(pt 40 64))
+ )
+ (port
+ (pt 256 64)
+ (output)
+ (text "c0" (rect 0 0 15 13)(font "Arial" (font_size 8)))
+ (text "c0" (rect 241 51 253 63)(font "Arial" (font_size 8)))
+ )
+ (port
+ (pt 256 80)
+ (output)
+ (text "locked" (rect 0 0 37 13)(font "Arial" (font_size 8)))
+ (text "locked" (rect 221 67 252 79)(font "Arial" (font_size 8)))
+ )
+ (drawing
+ (text "Cyclone II" (rect 203 138 449 286)(font "Arial" ))
+ (text "inclk0 frequency: 50.000 MHz" (rect 50 60 226 130)(font "Arial" ))
+ (text "Operation Mode: Normal" (rect 50 72 203 154)(font "Arial" ))
+ (text "Clk " (rect 51 91 117 192)(font "Arial" ))
+ (text "Ratio" (rect 71 91 165 192)(font "Arial" ))
+ (text "Ph (dg)" (rect 97 91 225 192)(font "Arial" ))
+ (text "DC (%)" (rect 132 91 296 192)(font "Arial" ))
+ (text "c0" (rect 54 104 119 218)(font "Arial" ))
+ (text "1/5" (rect 76 104 166 218)(font "Arial" ))
+ (text "0.00" (rect 103 104 225 218)(font "Arial" ))
+ (text "50.00" (rect 136 104 296 218)(font "Arial" ))
+ (line (pt 0 0)(pt 257 0))
+ (line (pt 257 0)(pt 257 153))
+ (line (pt 0 153)(pt 257 153))
+ (line (pt 0 0)(pt 0 153))
+ (line (pt 48 89)(pt 164 89))
+ (line (pt 48 101)(pt 164 101))
+ (line (pt 48 114)(pt 164 114))
+ (line (pt 48 89)(pt 48 114))
+ (line (pt 68 89)(pt 68 114)(line_width 3))
+ (line (pt 94 89)(pt 94 114)(line_width 3))
+ (line (pt 129 89)(pt 129 114)(line_width 3))
+ (line (pt 163 89)(pt 163 114))
+ (line (pt 40 48)(pt 207 48))
+ (line (pt 207 48)(pt 207 135))
+ (line (pt 40 135)(pt 207 135))
+ (line (pt 40 48)(pt 40 135))
+ (line (pt 255 64)(pt 207 64))
+ (line (pt 255 80)(pt 207 80))
+ )
+)
trunk/altera/altpll0.bsf
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/altera/ZMachine.v
===================================================================
--- trunk/altera/ZMachine.v (nonexistent)
+++ trunk/altera/ZMachine.v (revision 2)
@@ -0,0 +1,1624 @@
+module boss(clk, adcDout, reset, data, waddress, we, pe, romCS, ramCS, led0, led1, led2, lcdCS, lcdRS, lcdReset, nadcCS, a17, a18);
+
+input clk;
+input adcDout;
+input reset;
+
+inout [7:0] data;
+
+output [16:0] waddress;
+output we;
+output pe;
+output romCS;
+output ramCS;
+output led0;
+output led1;
+output led2;
+output lcdCS;
+output lcdRS;
+output lcdReset;
+output nadcCS;
+output a17;
+output a18;
+
+reg [16:0] address;
+reg [7:0] dataOut;
+reg printEnable;
+reg writeEnable;
+reg rlcdRS;
+reg rlcdReset;
+reg adcCS;
+reg a17;
+reg a18;
+
+wire [16:0] waddress;
+wire we;
+wire pe;
+wire romCS;
+wire ramCS;
+wire led0;
+wire led1;
+wire led2;
+wire lcdCS;
+wire lcdRS;
+wire lcdReset;
+wire nadcCS;
+
+
+//`define HARDWARE_PRINT
+//`define REAL_HARDWARE
+
+`define CALLBACK_BASE 'h1E58B
+`define INIT_CALLBACK 'h0
+`define PRINT_CALLBACK 'h1
+`define PRINTCHAR_CALLBACK 'h2
+`define PRINTNUM_CALLBACK 'h3
+`define READ_CALLBACK 'h4
+`define STATUS_CALLBACK 'h5
+`define QUIT_CALLBACK 'h6
+`define EXCEPTION_CALLBACK 'h7
+
+`define OPER_LARGE 2'b00
+`define OPER_SMALL 2'b01
+`define OPER_VARI 2'b10
+`define OPER_OMIT 2'b11
+
+`define STATE_RESET 0
+`define STATE_FETCH_OP 1
+`define STATE_READ_TYPES 2
+`define STATE_READ_OPERS 3
+`define STATE_READ_INDIRECT 4
+`define STATE_READ_STORE 5
+`define STATE_READ_BRANCH 6
+`define STATE_DO_OP 7
+`define STATE_READ_FUNCTION 8
+`define STATE_CALL_FUNCTION 9
+`define STATE_RET_FUNCTION 10
+`define STATE_STORE_REGISTER 11
+`define STATE_PRINT 12
+`define STATE_DIVIDE 13
+`define STATE_HALT 14
+`define STATE_PRINT_CHAR 15
+
+`define OP_0 2'b00
+`define OP_1 2'b01
+`define OP_2 2'b10
+`define OP_VAR 2'b11
+
+`define OP0_RTRUE 'h0
+`define OP0_RFALSE 'h1
+`define OP0_PRINT 'h2
+`define OP0_PRINTRET 'h3
+`define OP0_NOP 'h4
+`define OP0_SAVE 'h5
+`define OP0_RESTORE 'h6
+`define OP0_RESTART 'h7
+`define OP0_RETPOP 'h8
+`define OP0_POP 'h9
+`define OP0_QUIT 'hA
+`define OP0_NEWLINE 'hB
+`define OP0_SHOWSTATUS 'hC
+`define OP0_VERIFY 'hD
+`define OP0_DYNAMIC 'hF
+
+`define OP1_JZ 'h0
+`define OP1_GETSIBLING 'h1
+`define OP1_GETCHILD 'h2
+`define OP1_GETPARENT 'h3
+`define OP1_GETPROPLEN 'h4
+`define OP1_INC 'h5
+`define OP1_DEC 'h6
+`define OP1_PRINTADDR 'h7
+`define OP1_SWITCHBANK 'h8
+`define OP1_REMOVEOBJ 'h9
+`define OP1_PRINTOBJ 'hA
+`define OP1_RET 'hB
+`define OP1_JUMP 'hC
+`define OP1_PRINTPADDR 'hD
+`define OP1_LOAD 'hE
+`define OP1_NOT 'hF
+
+`define OP2_JE 'h1
+`define OP2_JL 'h2
+`define OP2_JG 'h3
+`define OP2_DEC_CHK 'h4
+`define OP2_INC_CHK 'h5
+`define OP2_JIN 'h6
+`define OP2_TEST 'h7
+`define OP2_OR 'h8
+`define OP2_AND 'h9
+`define OP2_TESTATTR 'hA
+`define OP2_SETATTR 'hB
+`define OP2_CLEARATTR 'hC
+`define OP2_STORE 'hD
+`define OP2_INSERTOBJ 'hE
+`define OP2_LOADW 'hF
+`define OP2_LOADB 'h10
+`define OP2_GETPROP 'h11
+`define OP2_GETPROPADDR 'h12
+`define OP2_GETNEXTPROP 'h13
+`define OP2_ADD 'h14
+`define OP2_SUB 'h15
+`define OP2_MUL 'h16
+`define OP2_DIV 'h17
+`define OP2_MOD 'h18
+`define OP2_WRITEREG 'h1E
+
+`define OPVAR_CALL 'h0
+`define OPVAR_STOREW 'h1
+`define OPVAR_STOREB 'h2
+`define OPVAR_PUTPROP 'h3
+`define OPVAR_SREAD 'h4
+`define OPVAR_PRINTCHAR 'h5
+`define OPVAR_PRINTNUM 'h6
+`define OPVAR_RANDOM 'h7
+`define OPVAR_PUSH 'h8
+`define OPVAR_PULL 'h9
+`define OPVAR_GETTOUCH 'h1E
+`define OPVAR_BLIT1 'h1F
+
+`define PRINTEFFECT_FETCH 0
+`define PRINTEFFECT_FETCHAFTER 1
+`define PRINTEFFECT_RET1 2
+`define PRINTEFFECT_ABBREVRET 3
+
+reg forceStaticRead;
+reg forceDynamicRead;
+
+reg [3:0] state;
+reg [3:0] phase;
+
+reg [16:0] pc;
+reg [16:0] curPC;
+reg [15:0] globalsAddress;
+reg [15:0] objectTable;
+
+reg readHigh;
+
+reg [4:0] op;
+reg [1:0] operNum;
+reg [1:0] operTypes [4:0];
+reg [15:0] operand [3:0];
+reg [2:0] operandIdx;
+reg [13:0] branch;
+reg [7:0] store;
+reg negate;
+
+reg delayedBranch;
+reg divideAddOne;
+
+reg [15:0] returnValue;
+
+reg [6:0] opsToRead;
+reg [6:0] currentLocal;
+reg [16:0] csStack;
+reg [15:0] stackAddress;
+
+reg [16:0] temp;
+reg [15:0] random;
+
+reg [1:0] alphabet;
+reg [1:0] long;
+reg nextLoadIsDynamic;
+reg lastWriteWasDraw;
+
+reg adcClk;
+reg adcDin;
+
+reg [7:0] cachedReg;
+reg [15:0] cachedValue;
+
+// xp<=adcConfig[0]?0:'bZ;
+// yp<=adcConfig[1]?1:'bZ;
+// xm<=adcConfig[2]?1:'bZ;
+// ym<=adcConfig[3]?0:'bZ;
+// XP dataOut[6]
+// XM lcsRS
+// YP dataOut[7]
+// YM lcdWR
+
+initial
+begin
+ state=`STATE_RESET;
+ forceDynamicRead=0;
+ forceStaticRead=0;
+ writeEnable=0;
+ printEnable=0;
+ phase=0;
+ readHigh=0;
+ nextLoadIsDynamic=0;
+ adcCS=0;
+ a17=0;
+ a18=0;
+ cachedReg=0;
+ cachedValue=0;
+ lastWriteWasDraw=0;
+end
+
+assign waddress[16:2] = address[16:2];
+assign waddress[0] = adcCS?adcClk:address[0];
+assign waddress[1] = adcCS?adcDin:address[1];
+assign data[5:0] = (writeEnable || printEnable)?dataOut[5:0]:6'bZ;
+assign data[6] = (writeEnable || printEnable)?dataOut[6]:((adcCS && operand[0][0])?0:'bZ);
+assign data[7] = (writeEnable || printEnable)?dataOut[7]:((adcCS && operand[0][1])?1:'bZ);
+assign romCS = !(!adcCS && !printEnable && !writeEnable && !forceDynamicRead && (forceStaticRead || address[16]==1));
+assign ramCS = !(!adcCS && !printEnable && (writeEnable || forceDynamicRead || (!forceStaticRead && address[16]==0)));
+assign pe = adcCS?(operand[0][3]?0:'bZ):(!printEnable || !clk);
+assign we = !writeEnable;
+assign led0 = !address[12];
+assign led1 = !address[13];
+assign led2 = !address[14];
+assign lcdCS = !printEnable || !clk;
+assign lcdRS = adcCS?(operand[0][2]?1:'bZ):rlcdRS;
+assign lcdReset = rlcdReset;
+assign nadcCS = !adcCS;
+
+task StoreB;
+ begin
+ if (phase==0) begin
+ cachedReg<=15; nextLoadIsDynamic<=0; address<=operand[0]+operand[1]; writeEnable<=1; dataOut<=operand[2][7:0]; phase<=phase+1;
+ end else begin
+ address<=pc; writeEnable<=0; state<=`STATE_FETCH_OP;
+ end
+ end
+endtask
+
+task StoreW;
+ begin
+ case (phase)
+ 0: begin cachedReg<=15; nextLoadIsDynamic<=0; address<=operand[0]+operand[1]*2; writeEnable<=1; dataOut<=operand[2][15:8]; phase<=phase+1; end
+ 1: begin address<=address+1; dataOut<=operand[2][7:0]; phase<=phase+1; end
+ default: begin address<=pc; writeEnable<=0; state<=`STATE_FETCH_OP; end
+ endcase
+ end
+endtask
+
+task StoreRegisterAndBranch;
+ input [7:0] regNum;
+ input [15:0] value;
+ input doBranch;
+ begin
+ state<=`STATE_STORE_REGISTER;
+ store<=regNum;
+ returnValue<=value;
+ delayedBranch<=doBranch;
+ phase<=0;
+ end
+endtask
+
+task StoreResultAndBranch;
+ input [15:0] result;
+ input doBranch;
+ begin
+ if (store>=16) begin
+ address<=globalsAddress+2*(store-16);
+ end else if (store==0) begin
+ address<=2*stackAddress;
+ stackAddress<=stackAddress+1;
+ end else begin
+ address<=csStack+8+2*(store-1);
+ end
+ dataOut<=result[15:8];
+ writeEnable<=1;
+ state<=`STATE_STORE_REGISTER;
+ returnValue[7:0]<=result[7:0];
+ delayedBranch<=doBranch;
+ phase<=1;
+ end
+endtask
+
+task StoreResult;
+ input [15:0] result;
+ begin
+ StoreResultAndBranch(result, negate); // negate means won't branch
+ end
+endtask
+
+task StoreResultSlow;
+ input [15:0] result;
+ begin
+ StoreRegisterAndBranch(store, result, negate); // negate means won't branch
+ end
+endtask
+
+task ReturnFunction;
+ input [15:0] value;
+ begin
+ returnValue<=value;
+ phase<=0;
+ state<=`STATE_RET_FUNCTION;
+ end
+endtask
+
+task CallFunction;
+ input doubleReturn;
+ input noStoreOnReturn;
+ begin
+ negate<=doubleReturn;
+ delayedBranch<=noStoreOnReturn;
+ phase<=0;
+ state<=`STATE_CALL_FUNCTION;
+ end
+endtask
+
+task DoBranch;
+ input result;
+ begin
+ if ((!result)==negate) begin
+ if (branch==0) begin
+ ReturnFunction(0);
+ end else if (branch==1) begin
+ ReturnFunction(1);
+ end else begin
+ pc<=$signed(pc)+$signed(branch)-2;
+ address<=$signed(pc)+$signed(branch)-2;
+ state<=`STATE_FETCH_OP;
+ end
+ end else begin
+ address<=pc;
+ state<=`STATE_FETCH_OP;
+ end
+ end
+endtask
+
+task LoadAndStore;
+ input [16:0] loadAddress;
+ input word;
+ begin
+ if (phase==0) begin
+ store<=data;
+ forceDynamicRead<=nextLoadIsDynamic;
+ nextLoadIsDynamic<=0;
+ address<=loadAddress;
+ temp[7:0]<=0;
+ phase<=word?phase+1:2;
+ end else if (phase==1) begin
+ temp[7:0]<=data;
+ address<=address+1;
+ phase<=phase+1;
+ end else begin
+ forceDynamicRead<=0;
+ StoreResultSlow((temp[7:0]<<8)|data);
+ end
+ end
+endtask
+
+function [16:0] GetObjectAddr;
+ input [7:0] object;
+ begin
+ GetObjectAddr=objectTable+2*31+9*(object-1);
+ end
+endfunction
+
+task TestAttr;
+ begin
+ if (phase==0) begin
+ address<=GetObjectAddr(operand[0])+(operand[1]/8); phase<=phase+1;
+ end else begin
+ DoBranch(data[7-(operand[1]&7)]);
+ end
+ end
+endtask
+
+task SetAttr;
+ begin
+ case (phase)
+ 0: begin address<=GetObjectAddr(operand[0])+(operand[1]/8); phase<=phase+1; end
+ 1: begin dataOut<=data|(1<<(7-(operand[1]&7))); writeEnable<=1; phase<=phase+1; end
+ default: begin writeEnable<=0; address<=pc; state=`STATE_FETCH_OP; end
+ endcase
+ end
+endtask
+
+task ClearAttr;
+ begin
+ case (phase)
+ 0: begin address<=GetObjectAddr(operand[0])+(operand[1]/8); phase<=phase+1; end
+ 1: begin dataOut<=data&(~(1<<(7-(operand[1]&7)))); writeEnable<=1; phase<=phase+1; end
+ default: begin writeEnable<=0; address<=pc; state=`STATE_FETCH_OP; end
+ endcase
+ end
+endtask
+
+task JumpIfParent;
+ begin
+ if (phase==0) begin
+ address<=GetObjectAddr(operand[0])+4; phase<=phase+1;
+ end else begin
+ DoBranch(operand[1]==data);
+ end
+ end
+endtask
+
+task GetRelative;
+ input [1:0] offset;
+ input branch;
+ begin
+ if (phase==0) begin
+ address<=GetObjectAddr(operand[0])+4+offset; phase<=phase+1;
+ end else begin
+ StoreResultAndBranch(data, branch?data!=0:negate);
+ end
+ end
+endtask
+
+task FindProp;
+ begin
+ case (phase[2:0])
+ 0: begin store<=data; address<=GetObjectAddr(operand[0])+7; phase<=phase+1; end
+ 1: begin temp[16]<=0; temp[15:8]<=data; temp[7:0]<=0; address<=address+1; phase<=phase+1; end
+ 2: begin address<=temp|data; phase<=phase+1; end
+ 3: begin address<=address+2*data+1; phase<=phase+1; end
+ 4: begin
+ if (data==0) begin // end of search (get default)
+ address<=objectTable+2*(operand[1]-1);
+ phase<=phase+1;
+ end else if (data[4:0]==operand[1]) begin // found property
+ address<=address+1;
+ if (data[7:5]==0) // only 1 byte
+ phase<=6;
+ else
+ phase<=5;
+ end else begin // skip over data
+ address<=address+data[7:5]+2;
+ end
+ end
+ 5: begin temp[7:0]<=data; address<=address+1; phase<=phase+1; end
+ default: begin StoreResultSlow((temp[7:0]<<8)|data); end
+ endcase
+ end
+endtask
+
+task SetProp;
+ begin
+ case (phase[2:0])
+ 0: begin address<=GetObjectAddr(operand[0])+7; phase<=phase+1; end
+ 1: begin temp[16]<=0; temp[15:8]<=data; temp[7:0]<=0; address<=address+1; phase<=phase+1; end
+ 2: begin address<=temp|data; phase<=phase+1; end
+ 3: begin address<=address+2*data+1; phase<=phase+1; end
+ 4: begin
+`ifndef REAL_HARDWARE
+ if (data==0) begin // end of search
+ state<=`STATE_HALT;
+ end else
+`endif
+ if (data[4:0]==operand[1]) begin // found property
+ if (data[7:5]==0) begin // only 1 byte
+ dataOut<=operand[2][7:0];
+ phase<=6;
+ address<=address+1;
+ writeEnable<=1;
+ end else begin
+ dataOut<=operand[2][15:8];
+ phase<=5;
+ address<=address+1;
+ writeEnable<=1;
+ end
+ end else begin // skip over data
+ address<=address+data[7:5]+2;
+ end
+ end
+ 5: begin dataOut<=operand[2][7:0]; address<=address+1; phase<=phase+1; end
+ default: begin state<=`STATE_FETCH_OP; address<=pc; writeEnable<=0; end
+ endcase
+ end
+endtask
+
+task FindPropAddrLen;
+ begin
+ case (phase[2:0])
+ 0: begin store<=data; address<=GetObjectAddr(operand[0])+7; phase<=phase+1; end
+ 1: begin temp[16]<=0; temp[15:8]<=data; temp[7:0]<=0; address<=address+1; phase<=phase+1; end
+ 2: begin address<=temp|data; phase<=phase+1; end
+ 3: begin address<=address+2*data+1; phase<=phase+1; end
+ default: begin
+ if (data==0) begin // end of search
+ StoreResultSlow(0);
+ end else if (data[4:0]==operand[1]) begin // found property
+ StoreResultSlow(address+1);
+ end else begin // skip over data
+ address<=address+data[7:5]+2;
+ end
+ end
+ endcase
+ end
+endtask
+
+task FindNextProp;
+ begin
+ case (phase[2:0])
+ 0: begin store<=data; address<=GetObjectAddr(operand[0])+7; phase<=phase+1; end
+ 1: begin temp[16]<=0; temp[15:8]<=data; temp[7:0]<=0; address<=address+1; phase<=phase+1; end
+ 2: begin address<=temp|data; phase<=phase+1; end
+ 3: begin address<=address+2*data+1; phase<=phase+1; end
+ default: begin
+ if (operand[1]==0)
+ StoreResultSlow(data[4:0]);
+`ifndef REAL_HARDWARE
+ else if (data==0) // end of search
+ state<=`STATE_HALT;
+`endif
+ else begin // skip over data
+ if (data[4:0]==operand[1])
+ operand[1]<=0;
+ address<=address+data[7:5]+2;
+ end
+ end
+ endcase
+ end
+endtask
+
+task GetPropLen;
+ begin
+ if (phase==0) begin
+ address<=operand[0]-1; phase<=phase+1;
+ end else begin
+ StoreResultSlow((operand[0]==0)?0:(data[7:5]+1));
+ end
+ end
+endtask
+
+task Pull;
+ input return;
+ begin
+ case (phase)
+ 0: begin
+ address<=2*(stackAddress-1);
+ forceDynamicRead<=1;
+ phase<=phase+1;
+ end
+ 1: begin
+ temp[7:0]<=data;
+ stackAddress<=address>>1;
+ address<=address+1;
+ phase<=phase+1;
+ end
+ default: begin
+ forceDynamicRead<=0;
+ if (return) begin
+ ReturnFunction((temp[7:0]<<8)|data);
+ end else begin
+ if (operand[0]==0)
+ stackAddress<=stackAddress-1;
+ StoreRegisterAndBranch(operand[0], (temp[7:0]<<8)|data, negate);
+ end
+ end
+ endcase
+
+ end
+endtask
+
+task Print;
+ input [16:0] addr;
+ input [1:0] effect;
+ begin
+ temp<=addr;
+ state<=`STATE_PRINT;
+ returnValue[1:0]<=effect;
+ delayedBranch<=0;
+ phase<=0;
+ end
+endtask
+
+task PrintObj;
+ begin
+ case (phase[1:0])
+ 0: begin address<=GetObjectAddr(operand[0])+7; phase<=phase+1; end
+ 1: begin store<=data; address<=address+1; phase<=phase+1; end
+ default: Print((store<<8)+data+1, `PRINTEFFECT_FETCH);
+ endcase
+ end
+endtask
+
+task RemoveObject;
+ begin
+ case (phase[3:0])
+ 0: begin address<=GetObjectAddr(operand[0])+4; /*obj.parent*/ phase<=phase+1; end
+ 1: begin
+ if (data==0) begin
+ phase<=(operand[1]!=0)?6:9;
+ end else begin
+ phase<=phase+1;
+ end
+ temp[15:8]<=data;
+ writeEnable<=1;
+ dataOut<=operand[1];
+ end
+ 2: begin writeEnable<=0; address<=address+1; /*obj.sibling*/ phase<=phase+1; end
+ 3: begin temp[7:0]<=data; writeEnable<=1; dataOut<=0; phase<=phase+1; end
+ 4: begin writeEnable<=0; address<=GetObjectAddr(temp[15:8])+6; /*parent.child*/ phase<=phase+1; end
+ 5: begin
+ if (data==operand[0]) begin // found object
+ dataOut<=temp[7:0];
+ writeEnable<=1;
+ phase<=(operand[1]!=0)?phase+1:9;
+ end else begin
+ address<=GetObjectAddr(data)+5; /*follow sibling*/
+ end
+ end
+
+ 6: begin address<=GetObjectAddr(operand[1])+6; writeEnable<=0; phase<=phase+1; end
+ 7: begin temp[7:0]<=data; writeEnable<=1; dataOut<=operand[0]; phase<=phase+1; end
+ 8: begin address<=GetObjectAddr(operand[0])+5; dataOut<=temp[7:0]; phase<=phase+1; end
+ default: begin
+ writeEnable<=0;
+ state<=`STATE_FETCH_OP;
+ address<=pc;
+ end
+ endcase
+ end
+endtask
+
+task Random;
+ begin
+ case (phase)
+ 0: begin if ($signed(operand[0])<0) begin random<=operand[0]; state<=`STATE_FETCH_OP; end else begin random<=random^(random<<13); end phase<=phase+1; end
+ 1: begin random<=random^(random>>9); phase<=phase+1; end
+ 2: begin random<=random^(random<<7); phase<=phase+1; end
+ default: begin state<=`STATE_DIVIDE; delayedBranch<=1; divideAddOne<=1; operand[1]<=operand[0]; operand[0]<=random&'h7FFF; phase<=0; end
+ endcase
+ end
+endtask
+
+task PrintNum;
+ begin
+`ifdef HARDWARE_PRINT
+ case (phase)
+ 0: begin negate<=0; operand[1]<=1; operand[2][2:0]<=2; operand[3][3:0]<=0; phase<=phase+1; end
+ 1,2,3,4: begin operand[1]<=(operand[1]<<3)+(operand[1]<<1); printEnable<=0; phase<=phase+1; end
+ 5: begin
+ if (operand[0]>=operand[1]) begin
+ operand[0]<=operand[0]-operand[1];
+ operand[3][3:0]<=operand[3][3:0]+1;
+ negate<=1;
+ printEnable<=0;
+ end else begin
+ printEnable<=negate;
+ dataOut<=operand[3][3:0]+48;
+ operand[3][3:0]<=0;
+ operand[1]<=1;
+ operand[2][2:0]<=operand[2][2:0]+1;
+ phase<=operand[2][2:0];
+ end
+ end
+ default: begin printEnable<=0; state<=`STATE_FETCH_OP; end
+ endcase
+`else
+ operand[0]<=`PRINTNUM_CALLBACK;
+ operand[1]<=operand[0];
+ operTypes[1]<=`OPER_LARGE;
+ CallFunction(0, 1);
+`endif
+ end
+endtask
+
+task PrintChar;
+ input [7:0] char;
+ begin
+ $display("Print char: %d\n", char);
+`ifdef HARDWARE_PRINT
+ printEnable<=1;
+ dataOut<=char;
+ state<=`STATE_PRINT_CHAR;
+`else
+ operand[0]<=`PRINTCHAR_CALLBACK;
+ operand[1]<=char;
+ operTypes[1]<=`OPER_LARGE;
+ CallFunction(0, 1);
+`endif
+ end
+endtask
+
+task SRead;
+ begin
+ operand[0]<=`READ_CALLBACK;
+ operand[2]<=operand[0]; // Am swapping arguments just to save space on FPGA
+ operTypes[2]<=`OPER_LARGE;
+ CallFunction(0, 1);
+ end
+endtask
+
+task WriteReg;
+ begin
+ case (phase)
+ 0: begin lastWriteWasDraw<=(operand[0]=='h22); if (operand[0]=='h22 && lastWriteWasDraw) begin rlcdRS<=1; phase<=4; end else begin rlcdRS<=0; phase<=phase+1; end end
+ 1: begin dataOut<=operand[0][15:8]; printEnable<=1; phase<=phase+1; end
+ 2: begin dataOut<=operand[0][7:0]; if (operand[0]==0) phase<=4; else phase<=phase+1; end
+ 3: begin rlcdRS<=1; printEnable<=0; phase<=phase+1; end
+ 4: begin dataOut<=operand[1][15:8]; printEnable<=1; phase<=phase+1; end
+ 5: begin dataOut<=operand[1][7:0]; phase<=phase+1; end
+ default: begin printEnable<=0; address<=pc; state<=`STATE_FETCH_OP; end
+ endcase
+ end
+endtask
+
+task Blit1;
+ begin
+ case (phase)
+ 0: begin forceDynamicRead<=1; rlcdRS<=0; phase<=phase+1; end
+ 1: begin dataOut<=0; printEnable<=1; phase<=phase+1; end
+ 2: begin address<=operand[0]*2; dataOut<=34; returnValue<=0; phase<=phase+1; end
+ 3: begin
+ rlcdRS<=1;
+ printEnable<=0;
+ if (operand[1]==0) begin
+ forceDynamicRead<=0;
+ address<=pc;
+ state<=`STATE_FETCH_OP;
+ end
+ phase<=phase+1;
+ end
+ 4: begin
+ store<=data;
+ dataOut<=data[7]?operand[3][15:8]:operand[2][15:8];
+ printEnable<=1;
+ phase<=6;
+ end
+ 5: begin
+ dataOut<=store[7]?operand[3][15:8]:operand[2][15:8];
+ printEnable<=1;
+ phase<=phase+1;
+ end
+ default: begin
+ dataOut<=store[7]?operand[3][7:0]:operand[2][7:0];
+ store[7:1]=store[6:0];
+ returnValue<=returnValue+1;
+ if (returnValue[2:0]==7) begin
+ operand[1]=operand[1]-1;
+ phase<=3;
+ address<=address+1;
+ end else begin
+ phase<=5;
+ end
+ end
+ endcase
+ end
+endtask
+
+task GetTouch;
+ begin
+ case (phase)
+ 0: begin
+ adcCS<=1;
+ adcClk<=0;
+ adcDin<=1;
+ operand[1]<='hffff;
+ operand[2]<=1;
+ phase<=1;
+ end
+ 1: begin
+ operand[2][3:0]<=operand[2][3:0]+1;
+ if (operand[2][3:0]==0) begin // Max clock rate for ADC is ~ 1MHz so divide our clock by 16
+ adcClk<=1;
+ operand[1]<=(operand[1]<<1)+adcDout;
+ phase<=(!operand[1][9])?3:2;
+ end
+ end
+ 2: begin
+ operand[2][3:0]<=operand[2][3:0]+1;
+ if (operand[2][3:0]==0) begin // Max clock rate for ADC is ~ 1MHz so divide our clock by 16
+ operand[0][8:4]<=(operand[0][8:4]>>1);
+ adcDin<=operand[0][4];
+ adcClk<=0;
+ phase<=1;
+ end
+ end
+ default: begin adcCS<=0; StoreResultSlow(operand[1]&'h3FF); end
+ endcase
+ end
+endtask
+
+task DoOp;
+ begin
+ case (operNum)
+ `OP_0: begin
+ if (phase==0)
+ $display("PC:%h Doing op0:%d Store:%h Branch:%h/%d", curPC, op, operand[0], store, branch, negate);
+ case (op[3:0])
+ `OP0_RTRUE: ReturnFunction(1);
+ `OP0_RFALSE: ReturnFunction(0);
+ `OP0_PRINT: Print(pc, `PRINTEFFECT_FETCHAFTER);
+ `OP0_PRINTRET: Print(pc, `PRINTEFFECT_RET1);
+ `OP0_SAVE,`OP0_RESTORE: DoBranch(0);
+ `OP0_RESTART: state<=`STATE_RESET;
+ `OP0_RETPOP: Pull(1);
+ `OP0_QUIT: begin operand[0]<=`QUIT_CALLBACK; CallFunction(0,1); end
+ `OP0_NEWLINE: PrintChar(10);
+ `OP0_SHOWSTATUS: begin operand[0]<=`STATUS_CALLBACK; CallFunction(0,1); end
+ `OP0_VERIFY: DoBranch(1);
+ default: state<=`STATE_HALT;
+ endcase
+ end
+ `OP_1: begin
+ if (phase==0)
+ $display("PC:%h Doing op1:%d Operands:%h Store:%h Branch:%h/%d", curPC, op, operand[0], store, branch, negate);
+ case (op[3:0])
+ `OP1_JZ: DoBranch(operand[0]==0);
+ `OP1_GETSIBLING: GetRelative(1,1);
+ `OP1_GETCHILD: GetRelative(2,1);
+ `OP1_GETPARENT: GetRelative(0,0);
+ `OP1_GETPROPLEN: GetPropLen();
+ `OP1_INC: StoreResult(operand[0]+1);
+ `OP1_DEC: StoreResult(operand[0]-1);
+ `OP1_PRINTADDR: Print(operand[0], `PRINTEFFECT_FETCH);
+ `OP1_SWITCHBANK: begin a17<=operand[0][0]; a18<=operand[0][1]; state<=`STATE_RESET; end
+ `OP1_REMOVEOBJ: begin operNum<=`OP_2; op<=`OP2_INSERTOBJ; operand[1]<=0; end
+ `OP1_PRINTOBJ: PrintObj();
+ `OP1_RET: ReturnFunction(operand[0]);
+ `OP1_JUMP: begin pc<=$signed(pc)+$signed(operand[0])-2; address<=$signed(pc)+$signed(operand[0])-2; state<=`STATE_FETCH_OP; end
+ `OP1_PRINTPADDR: Print(2*operand[0], `PRINTEFFECT_FETCH);
+ `OP1_LOAD: StoreResult(operand[0]);
+ `OP1_NOT: StoreResult(~operand[0]);
+ default: state<=`STATE_HALT;
+ endcase
+ end
+ `OP_2: begin
+ if (phase==0)
+ $display("PC:%h Doing op2:%d Operands:%h %h Store:%h Branch:%h/%d/%h", curPC, op, operand[0], operand[1], store, branch, negate, $signed(pc)+$signed(branch-2));
+ case (op)
+ `OP2_JE: DoBranch(operand[0]==operand[1] || (operTypes[2]!=`OPER_OMIT && operand[0]==operand[2]) || (operTypes[3]!=`OPER_OMIT && operand[0]==operand[3]));
+ `OP2_JL: DoBranch($signed(operand[0])<$signed(operand[1]));
+ `OP2_JG: DoBranch($signed(operand[0])>$signed(operand[1]));
+ `OP2_INC_CHK: StoreResultAndBranch(operand[0]+1, $signed(operand[0])+1>$signed(operand[1]));
+ `OP2_DEC_CHK: StoreResultAndBranch(operand[0]-1, $signed(operand[0])-1<$signed(operand[1]));
+ `OP2_JIN: JumpIfParent();
+ `OP2_TEST: DoBranch((operand[0]&operand[1])==operand[1]);
+ `OP2_OR: StoreResult(operand[0]|operand[1]);
+ `OP2_AND: StoreResult(operand[0]&operand[1]);
+ `OP2_TESTATTR: TestAttr();
+ `OP2_SETATTR: SetAttr();
+ `OP2_CLEARATTR: ClearAttr();
+ `OP2_STORE: begin if (operand[0]==0) stackAddress<=stackAddress-1; StoreRegisterAndBranch(operand[0], operand[1], negate); end
+ `OP2_INSERTOBJ: RemoveObject();
+ `OP2_LOADW: LoadAndStore(operand[0]+2*operand[1], 1);
+ `OP2_LOADB: LoadAndStore(operand[0]+operand[1], 0);
+ `OP2_GETPROP: FindProp();
+ `OP2_GETPROPADDR: FindPropAddrLen();
+ `OP2_GETNEXTPROP: FindNextProp();
+ `OP2_ADD: StoreResult($signed(operand[0])+$signed(operand[1]));
+ `OP2_SUB: StoreResult($signed(operand[0])-$signed(operand[1]));
+ `OP2_MUL: StoreResult($signed(operand[0])*$signed(operand[1]));
+ `OP2_DIV: begin store<=data; state=`STATE_DIVIDE; delayedBranch<=0; divideAddOne<=0; end
+ `OP2_MOD: begin store<=data; state=`STATE_DIVIDE; delayedBranch<=1; divideAddOne<=0; end
+ `OP2_WRITEREG: WriteReg();
+ default: state<=`STATE_HALT;
+ endcase
+ end
+ default: begin // 'OP_VAR
+ if (phase==0) begin
+ if (operTypes[0]==`OPER_OMIT)
+ $display("PC:%h Doing opvar:%d Operands: Store:%h Branch:%h/%d/%h", curPC, op, store, branch, negate, $signed(pc)+$signed(branch-2));
+ else if (operTypes[1]==`OPER_OMIT)
+ $display("PC:%h Doing opvar:%d Operands:%h Store:%h Branch:%h/%d/%h", curPC, op, operand[0], store, branch, negate, $signed(pc)+$signed(branch-2));
+ else if (operTypes[2]==`OPER_OMIT)
+ $display("PC:%h Doing opvar:%d Operands:%h %h Store:%h Branch:%h/%d/%h", curPC, op, operand[0], operand[1], store, branch, negate, $signed(pc)+$signed(branch-2));
+ else if (operTypes[3]==`OPER_OMIT)
+ $display("PC:%h Doing opvar:%d Operands:%h %h %h Store:%h Branch:%h/%d/%h", curPC, op, operand[0], operand[1], operand[2], store, branch, negate, $signed(pc)+$signed(branch-2));
+ else
+ $display("PC:%h Doing opvar:%d Operands:%h %h %h %h Store:%h Branch:%h/%d/%h", curPC, op, operand[0], operand[1], operand[2], operand[3], store, branch, negate, $signed(pc)+$signed(branch-2));
+ end
+ case (op)
+ `OPVAR_CALL: if (operand[0]==0) StoreResultSlow(0); else CallFunction(0, 0);
+ `OPVAR_STOREW: StoreW();
+ `OPVAR_STOREB: StoreB();
+ `OPVAR_PUTPROP: SetProp();
+ `OPVAR_SREAD: SRead();
+ `OPVAR_PRINTCHAR: PrintChar(operand[0]);
+ `OPVAR_PRINTNUM: PrintNum();
+ `OPVAR_RANDOM: Random();
+ `OPVAR_PUSH: StoreRegisterAndBranch(0, operand[0], negate);
+ `OPVAR_PULL: Pull(0);
+ `OPVAR_GETTOUCH: GetTouch();
+ `OPVAR_BLIT1: Blit1();
+ default: state<=`STATE_HALT;
+ endcase
+ end
+ endcase
+ end
+endtask
+
+`ifdef HARDWARE_PRINT
+task DoPrint;
+ input [4:0] char;
+ begin
+ if (delayedBranch) begin
+ store[4:0]<=char;
+ operand[1]<=phase+1;
+ delayedBranch<=0;
+ phase<=6;
+ end else if (long==1) begin
+ operand[3][4:0]=char;
+ long<=long+1;
+ end else if (long==2) begin
+ printEnable<=1;
+ dataOut<=(operand[3][4:0]<<5)|char;
+ long<=0;
+ end else begin
+ phase<=phase+1;
+ if (char==0) begin
+ printEnable<=1;
+ dataOut<=32;
+ end else if (char==4 || char==5) begin
+ printEnable<=0;
+ alphabet=char-3;
+ end else if (char>=6) begin
+ printEnable<=!(alphabet==2 && char==6);
+ if (alphabet==2) begin
+ case (char)
+ 6: long<=1;
+ 7: dataOut<=10;
+ 8,9,10,11,12,13,14,15,16,17: dataOut<=char-8+48;
+ 18: dataOut<=46;
+ 19: dataOut<=44;
+ 20: dataOut<=33;
+ 21: dataOut<=63;
+ 22: dataOut<=95;
+ 23: dataOut<=35;
+ 24: dataOut<=39;
+ 25: dataOut<=34;
+ 26: dataOut<=47;
+ 27: dataOut<=92;
+ 28: dataOut<=45;
+ 29: dataOut<=58;
+ 30: dataOut<=40;
+ 31: dataOut<=41;
+ endcase
+ end else begin
+ dataOut<=char-6+((alphabet==0)?97:65);
+ end
+ alphabet<=0;
+ end else begin
+ printEnable<=0;
+ store[7:5]<=(char-1);
+ delayedBranch<=1;
+ end
+ end
+ end
+endtask
+`endif
+
+task FinishReadingOps;
+begin
+ case (operNum)
+ `OP_1: begin
+ case (op[3:0])
+ `OP1_INC, `OP1_DEC, `OP1_LOAD: state<=`STATE_READ_INDIRECT;
+ `OP1_GETSIBLING,`OP1_GETCHILD,`OP1_GETPARENT,`OP1_GETPROPLEN,`OP1_NOT: state<=`STATE_READ_STORE;
+ `OP1_JZ: state<=`STATE_READ_BRANCH;
+ default: state<=`STATE_DO_OP;
+ endcase
+ pc<=pc+1;
+ end
+ `OP_2: begin
+ case (op[4:0])
+ `OP2_INC_CHK,`OP2_DEC_CHK: begin pc<=pc+1; state<=`STATE_READ_INDIRECT; end
+ `OP2_OR,`OP2_AND,`OP2_ADD,`OP2_SUB,`OP2_MUL: begin pc<=pc+1; state<=`STATE_READ_STORE; end
+ `OP2_LOADW,`OP2_LOADB,`OP2_GETPROP ,`OP2_GETPROPADDR,`OP2_GETNEXTPROP,`OP2_DIV,`OP2_MOD: begin pc<=pc+2; state<=`STATE_DO_OP; end
+ `OP2_JE,`OP2_JL,`OP2_JG,`OP2_JIN,`OP2_TEST,`OP2_TESTATTR: begin pc<=pc+1; state<=`STATE_READ_BRANCH; end
+ default: begin pc<=pc+1; state<=`STATE_DO_OP; end
+ endcase
+ end
+ default: begin // `OP_VAR
+ pc<=pc+1;
+ case (op[4:0])
+ `OPVAR_CALL,`OPVAR_RANDOM,`OPVAR_GETTOUCH: state<=`STATE_READ_STORE;
+ default: state<=`STATE_DO_OP;
+ endcase
+ end
+ endcase
+end
+endtask
+
+always @ (posedge clk)
+begin
+ case(state)
+ `STATE_RESET: begin
+ case (phase)
+ 0: begin printEnable<=0; random<=1; forceStaticRead<=1; address<='h6; phase<=phase+1; end
+ 1: begin address<='h7; phase<=phase+1; pc[15:8]<=data; pc[16]<=0; end
+ 2: begin address<='hA; phase<=phase+1; pc[7:0]<=data; end
+ 3: begin address<='hB; phase<=phase+1; objectTable[15:8]<=data; end
+ 4: begin address<='hC; phase<=phase+1; objectTable[7:0]<=data; end
+ 5: begin address<='hD; phase<=phase+1; globalsAddress[15:8]<=data; end
+ 6: begin address<=0; phase<=phase+1; globalsAddress[7:0]<=data; end
+ 7: begin dataOut<=data; writeEnable<=1; phase<=8; end
+ 8: begin writeEnable<=0; address<=address+1; if (address[15:0]=='hffff) phase<=9; else phase<=7; end
+ 9: begin writeEnable<=1; dataOut<=0; address<=address+1; if (address[15:0]=='hffff) begin writeEnable<=0; phase<=10; end end
+ 10: begin rlcdReset<=address[10]; rlcdRS<=1; printEnable<=0; address<=address+1; if (address[10:0]=='h7ff) phase<=11; end
+ default: begin
+ cachedReg<=15;
+ address<=pc;
+ forceStaticRead<=0;
+ stackAddress<=64*1024/2;
+ csStack<=65*1024;
+ operand[0]<=`INIT_CALLBACK;
+ operTypes[1]<=`OPER_OMIT;
+ CallFunction(0, 1);
+ end
+ endcase
+ end
+ `STATE_CALL_FUNCTION: begin
+ case (phase)
+ 0: begin $display("Call function %h", operand[0]*2); address<=csStack; writeEnable<=1; dataOut<=(delayedBranch<<2)|(negate<<1)|pc[16]; phase<=phase+1; end
+ 1: begin address<=address+1; dataOut<=pc[15:8]; phase<=phase+1; end
+ 2: begin address<=address+1; dataOut<=pc[7:0]; phase<=4; end
+ 3: begin end // pointless phase but for some reason saves gates
+ 4: begin address<=address+1; dataOut<=stackAddress[15:8]; phase<=phase+1; end
+ 5: begin address<=address+1; dataOut<=stackAddress[7:0]; phase<=phase+1; end
+ 6: begin address<=address+1; dataOut<=store; phase<=(operand[0][15:3]==0)?phase+1:10; end
+ 7: begin address<=`CALLBACK_BASE+5*(operand[0]&7); writeEnable<=0; phase<=phase+1; end
+ 8: begin address<=address+1; operand[0][15:8]<=data; phase<=phase+1; end
+ 9: begin operand[0][7:0]<=data; phase<=phase+1; end
+ default: begin
+ cachedReg<=15;
+ csStack<=csStack+38;
+ address<=2*operand[0];
+ state<=`STATE_READ_FUNCTION;
+ writeEnable<=0;
+ phase<=0;
+ end
+ endcase
+ end
+ `STATE_RET_FUNCTION: begin
+ case (phase)
+ 0: begin csStack<=csStack-38; address<=csStack-38; forceDynamicRead<=1; phase<=phase+1; end
+ 1: begin pc[16]<=data[0]; negate<=data[1]; delayedBranch<=data[2]; address<=address+1; phase<=phase+1; end
+ 2: begin pc[15:8]<=data; address<=address+1; phase<=phase+1; end
+ 3: begin pc[7:0]<=data; address<=address+1; phase<=phase+1; end
+ 4: begin stackAddress[15:8]<=data; address<=address+1; phase<=phase+1; end
+ 5: begin stackAddress[7:0]<=data; address<=address+1; phase<=phase+1; end
+ default: begin
+ forceDynamicRead<=0;
+ cachedReg<=15;
+ if (negate) begin
+ phase<=0;
+ end else if (delayedBranch) begin
+ state<=`STATE_FETCH_OP;
+ address<=pc;
+ end else begin
+ StoreRegisterAndBranch(data, returnValue, negate);
+ end
+ end
+ endcase
+ end
+ `STATE_READ_FUNCTION: begin
+ case (phase)
+ 0: begin
+ if (data==0) begin
+ state<=`STATE_FETCH_OP;
+ end else begin
+ opsToRead<=4*data-1;
+ phase<=phase+1;
+ end
+ currentLocal<=0;
+ pc<=address+1;
+ address<=address+1;
+ end
+ default: begin
+ if (opsToRead&1) begin
+ if (currentLocal<6 && operTypes[(currentLocal>>1)+1]!=`OPER_OMIT) begin
+ dataOut<=currentLocal[0]?operand[(currentLocal>>1)+1][7:0]:operand[(currentLocal>>1)+1][15:8];
+ end else begin
+ dataOut<=data;
+ end
+ address<=csStack+8+currentLocal;
+ writeEnable<=1;
+ currentLocal<=currentLocal+1;
+ end else begin
+ pc<=pc+1;
+ address<=pc+1;
+ writeEnable<=0;
+ end
+ if (opsToRead==0) begin
+ state<=`STATE_FETCH_OP;
+ end
+ opsToRead<=opsToRead-1;
+ end
+ endcase
+ end
+ `STATE_FETCH_OP: begin
+ phase<=0;
+ operTypes[2]<=`OPER_OMIT;
+ operTypes[3]<=`OPER_OMIT;
+ operTypes[4]<=`OPER_OMIT;
+ curPC<=pc;
+ $display("Fetching op at %h", pc);
+ if (!reset) begin
+ state<=`STATE_RESET;
+ a17<=0;
+ a18<=0;
+ end else begin
+ case (data[7:6])
+ 2'b10: begin
+ // short form
+ op[4:0]<=data[3:0];
+ operTypes[0]<=data[5:4];
+ operTypes[1]<=`OPER_OMIT;
+ if (data[5:4]==2'b11) begin
+ operNum[1:0]<=`OP_0;
+ case (data[3:0])
+ `OP0_POP: begin cachedReg<=15; stackAddress<=stackAddress-1; end
+ `OP0_NOP: begin /*nop*/ end
+ `OP0_DYNAMIC: nextLoadIsDynamic<=1;
+ `OP0_SAVE,`OP0_RESTORE,`OP0_VERIFY: state<=`STATE_READ_BRANCH;
+ default: state<=`STATE_DO_OP;
+ endcase
+ end else begin
+ operNum[1:0]<=`OP_1;
+ state<=`STATE_READ_OPERS;
+ end
+ end
+ 2'b11: begin
+ // variable form
+ op[4:0]<=data[4:0];
+ operNum[1:0]<=(data[5]?`OP_VAR:`OP_2);
+ state<=`STATE_READ_TYPES;
+ end
+ default: begin
+ // long form
+ op[4:0]<=data[4:0];
+ operNum[1:0]<=`OP_2;
+ operTypes[0]<=(data[6] ? `OPER_VARI : `OPER_SMALL);
+ operTypes[1]<=(data[5] ? `OPER_VARI : `OPER_SMALL);
+ state<=`STATE_READ_OPERS;
+ end
+ endcase
+ end
+ operandIdx<=0;
+ pc<=pc+1;
+ address<=pc+1;
+ end
+ `STATE_READ_TYPES: begin
+ operTypes[0]<=data[7:6];
+ operTypes[1]<=data[5:4];
+ operTypes[2]<=data[3:2];
+ operTypes[3]<=data[1:0];
+ address<=pc+1;
+ if (data[7:6]==`OPER_OMIT)
+ FinishReadingOps();
+ else begin
+ state<=`STATE_READ_OPERS;
+ pc<=pc+1;
+ end
+ end
+ `STATE_READ_OPERS: begin
+ case (operTypes[operandIdx])
+ `OPER_SMALL: begin
+ operand[operandIdx]<=data[7:0];
+ address<=pc+1;
+ operandIdx<=operandIdx+1;
+ if (operTypes[operandIdx+1]==`OPER_OMIT)
+ FinishReadingOps();
+ else
+ pc<=pc+1;
+ end
+ `OPER_LARGE: begin
+ address<=pc+1;
+ if (!readHigh) begin
+ operand[operandIdx][15:8]<=data[7:0];
+ readHigh<=1;
+ pc<=pc+1;
+ end else begin
+ operand[operandIdx][7:0]<=data[7:0];
+ operandIdx<=operandIdx+1;
+ readHigh<=0;
+ if (operTypes[operandIdx+1]==`OPER_OMIT)
+ FinishReadingOps();
+ else
+ pc<=pc+1;
+ end
+ end
+ default: begin // OPER_VARI
+ case (phase)
+ 0: begin
+ if (data==0) begin
+ stackAddress<=stackAddress-1;
+ end
+ if (cachedReg!=15 && data==cachedReg) begin
+ operand[operandIdx]<=cachedValue;
+ address<=pc+1;
+ operandIdx<=operandIdx+1;
+ if (operTypes[operandIdx+1]==`OPER_OMIT)
+ FinishReadingOps();
+ else
+ pc<=pc+1;
+ if (cachedReg==0)
+ cachedReg<=15;
+ end else begin
+ if (data>=16) begin
+ address<=globalsAddress+2*(data-16);
+ end else if (data==0) begin
+ address<=2*(stackAddress-1);
+ end else begin
+ address<=csStack+8+2*(data-1);
+ end
+ forceDynamicRead<=1;
+ phase<=phase+1;
+ end
+ end
+ 1: begin
+ operand[operandIdx]<=(data<<8);
+ address<=address+1;
+ phase<=phase+1;
+ end
+ default: begin
+ forceDynamicRead<=0;
+ operand[operandIdx]<=operand[operandIdx]|data;
+ address<=pc+1;
+ operandIdx<=operandIdx+1;
+ if (operTypes[operandIdx+1]==`OPER_OMIT)
+ FinishReadingOps();
+ else
+ pc<=pc+1;
+ phase<=0;
+ end
+ endcase
+ end
+ endcase
+ end
+ `STATE_READ_INDIRECT: begin
+ case (phase)
+ 0: begin
+ cachedReg<=15;
+ store<=operand[0];
+ if (operand[0]>=16) begin
+ address<=globalsAddress+2*(operand[0]-16);
+ end else if (operand[0]==0) begin
+ if (op[4:0]!=`OP1_LOAD)
+ stackAddress<=stackAddress-1;
+ address<=2*(stackAddress-1);
+ end else begin
+ address<=csStack+8+2*(operand[0]-1);
+ end
+ forceDynamicRead<=1;
+ phase<=phase+1;
+ end
+ 1: begin
+ operand[0][15:8]<=data;
+ address<=address+1;
+ phase<=phase+1;
+ end
+ default: begin
+ forceDynamicRead<=0;
+ operand[0][7:0]<=data;
+ if (op[4:0]==`OP1_LOAD)
+ state<=`STATE_READ_STORE;
+ else if (operNum==`OP_2)
+ state<=`STATE_READ_BRANCH;
+ else
+ state<=`STATE_DO_OP;
+ address<=pc;
+ phase<=0;
+ end
+ endcase
+ end
+ `STATE_READ_STORE: begin
+ case (op[4:0])
+ `OP1_GETSIBLING, `OP1_GETCHILD: state<=`STATE_READ_BRANCH;
+ default: state<=`STATE_DO_OP;
+ endcase
+ store<=data;
+ pc<=pc+1;
+ address<=pc+1;
+ end
+ `STATE_READ_BRANCH: begin
+ if (!readHigh) begin
+ if (data[6]) begin
+ branch<=data[5:0];
+ negate<=!data[7];
+ state<=`STATE_DO_OP;
+ end else begin
+ branch[13:8]<=data[5:0];
+ negate<=!data[7];
+ readHigh<=1;
+ end
+ end else begin
+ branch[7:0]<=data;
+ readHigh<=0;
+ state<=`STATE_DO_OP;
+ end
+ pc<=pc+1;
+ address<=pc+1;
+ end
+ `STATE_DO_OP: begin
+ DoOp();
+ end
+ `STATE_STORE_REGISTER: begin
+ case (phase)
+ 0: begin
+ if (store>=16) begin
+ address<=globalsAddress+2*(store-16);
+ end else if (store==0) begin
+ address<=2*stackAddress;
+ stackAddress<=stackAddress+1;
+ end else begin
+ address<=csStack+8+2*(store-1);
+ end
+ dataOut<=returnValue[15:8];
+ writeEnable<=1;
+ phase<=phase+1;
+ end
+ 1: begin
+ cachedReg<=store;
+ cachedValue[15:8]<=dataOut;
+ cachedValue[7:0]<=returnValue[7:0];
+ dataOut<=returnValue[7:0];
+ address<=address+1;
+ phase<=phase+1;
+ end
+ default: begin
+ DoBranch(delayedBranch);
+ writeEnable<=0;
+ phase<=0;
+ end
+ endcase
+ end
+ `STATE_DIVIDE: begin
+ case (phase)
+ 0: begin
+ negate<=operand[0][15]^operand[1][15];
+ readHigh<=operand[0][15];
+ if ($signed(operand[0])<0)
+ operand[0]<=-operand[0];
+ else
+ operand[0]<=operand[0];
+`ifndef REAL_HARDWARE
+ if (operand[1]==0)
+ state=`STATE_HALT;
+ else
+`endif
+ if ($signed(operand[1])<0)
+ operand[1]<=-operand[1];
+ operand[2]<=1;
+ operand[3]<=0;
+ phase<=phase+1;
+ end
+ 1: begin
+ if (operand[1][15] || operand[0]<=operand[1]) begin
+ phase<=phase+1;
+ end else begin
+ operand[1]<=operand[1]<<1;
+ operand[2]<=operand[2]<<1;
+ end
+ end
+ 2: begin
+ if (operand[1]>operand[0]) begin
+ if (operand[2]==1) begin
+ if (negate) begin
+ operand[0]<=-operand[3];
+ end else begin
+ operand[0]<=operand[3];
+ end
+ if (readHigh) begin
+ operand[1]<=-operand[0];
+ end else begin
+ operand[1]<=operand[0];
+ end
+ readHigh<=0;
+ phase<=phase+1;
+ end else begin
+ operand[1]<=operand[1]>>1;
+ operand[2]<=operand[2]>>1;
+ end
+ end else begin
+ operand[0]<=operand[0]-operand[1];
+ operand[3]<=operand[3]+operand[2];
+ end
+ end
+ default: begin
+ StoreResultSlow(delayedBranch?(operand[1]+divideAddOne):operand[0]);
+ end
+ endcase
+ end
+ `STATE_PRINT: begin
+`ifdef HARDWARE_PRINT
+ case (phase)
+ 0: begin delayedValue[15:8]<=data; address<=address+1; phase<=phase+1; end
+ 1: begin delayedValue[7:0] <=data; address<=address+1; phase<=phase+1; end
+ 2,3,4: begin DoPrint(delayedValue[14:10]); delayedValue[14:5]<=delayedValue[9:0]; end
+ 5: begin
+ printEnable<=0;
+ if (delayedValue[15]) begin
+ alphabet<=0;
+ long<=0;
+ case (returnValue[1:0])
+ `PRINTEFFECT_FETCH: begin
+ address<=pc;
+ state<=`STATE_FETCH_OP;
+ end
+ `PRINTEFFECT_FETCHAFTER: begin
+ pc<=address;
+ state<=`STATE_FETCH_OP;
+ end
+ `PRINTEFFECT_RET1: begin
+ ReturnFunction(1);
+ end
+ `PRINTEFFECT_ABBREVRET: begin
+ address<=temp;
+ delayedValue<=operand[0];
+ phase<=operand[1];
+ returnValue[1:0]<=returnValue[3:2];
+ end
+ endcase
+ end else begin
+ phase<=0;
+ end
+ end
+ // branch to abbrev
+ 6: begin
+ address<='h18;
+ phase<=phase+1;
+ temp<=address;
+ operand[0]<=delayedValue;
+ returnValue[3:2]=returnValue[1:0];
+ returnValue[1:0]=`PRINTEFFECT_ABBREVRET;
+ end
+ 7: begin address<=address+1; operand[2][7:0]=data; phase<=phase+1; end
+ 8: begin address<=(operand[2][7:0]<<8)|data+2*store; phase<=phase+1; end
+ 9: begin address<=address+1; operand[2][7:0]<=data; phase<=phase+1; end
+ default: begin address<=2*((operand[2][7:0]<<8)|data); phase<=0; end
+ endcase
+`else
+ if (data[7] || returnValue[1:0]!=`PRINTEFFECT_FETCHAFTER) begin
+ if (returnValue[1:0]==`PRINTEFFECT_FETCHAFTER)
+ pc<=address+2;
+ operand[0]<=`PRINT_CALLBACK;
+ $display("Print from %h\n", temp);
+ operand[1]<=temp[0]|((returnValue[1:0]==`PRINTEFFECT_RET1)?2:0); operand[2]<=temp[16:1];
+ operTypes[1]=`OPER_LARGE; operTypes[2]=`OPER_LARGE;
+ CallFunction(returnValue[1:0]==`PRINTEFFECT_RET1, 1);
+ end else begin
+ address<=address+2;
+ end
+`endif
+ end
+`ifdef HARDWARE_PRINT
+ `STATE_PRINT_CHAR: begin
+ address<=pc;
+ printEnable<=0;
+ state<=`STATE_FETCH_OP;
+ end
+`endif
+ default: begin
+ $display("HALT");
+ operand[1]<=(pc>>1);
+ operand[2]<=operand[0];
+ operand[3]<=operand[1];
+ operand[0]<=`EXCEPTION_CALLBACK;
+ //operTypes[0]<=`OPER_LARGE;
+ operTypes[1]<=`OPER_LARGE;
+ operTypes[2]<=`OPER_LARGE;
+ operTypes[3]<=`OPER_LARGE;
+ CallFunction(0,1);
+ end
+ endcase
+end
+
+endmodule
+
+module main();
+
+reg clk;
+reg adcDout;
+reg reset;
+wire [7:0] data;
+wire [16:0] address;
+wire writeEnable;
+wire printEnable;
+wire romCS;
+wire ramCS;
+wire led0;
+wire led1;
+wire led2;
+wire lcdCS;
+wire lcdRS;
+wire lcdReset;
+wire adcCS;
+wire a17;
+wire a18;
+
+integer file,readData,i,j,numOps,cycles,stateCycles[15:0],opCycles[255:0],ops[255:0];
+integer touch,touchX, touchY, touchZ;
+reg [7:0] mem [128*1024:0];
+reg [7:0] dynamicMem [128*1024:0];
+
+assign data=(!romCS ? mem[address] : (!ramCS ? (!writeEnable ? 8'bZ : dynamicMem[address] ) : 8'bZ ) );
+
+initial
+begin
+ $display("Welcome");
+ file=$fopen("rom.z3","rb");
+ readData=$fread(mem, file);
+ $display("File read:%h %d", file, readData);
+ $fclose(file);
+ touch=$fopen("touch.txt","r");
+ adcDout=0;
+ reset=1;
+ numOps=0;
+ cycles=0;
+ mem['h1E57F]=1; // Suppress game select screen as it's slow
+ for (i=0; i<16; i=i+1)
+ stateCycles[i]=0;
+ for (i=0; i<256; i=i+1) begin
+ opCycles[i]=0;
+ ops[i]=0;
+ end
+ //$monitor("%h %h %h State:%d(%d) Op:%h Num:%h OperType:%h%h%h%h OperIdx:%d Operand:%h %h Store:%h Branch:%h", clk, data, address, b.state, b.phase, b.op, b.operNum, b.operTypes[0], b.operTypes[1], b.operTypes[2], b.operTypes[3], b.operandIdx, b.operand[0], b.operand[1], b.store, b.branch);
+ for (i=0; i*505*/10000000; i=i+1) begin
+ clk=1;
+ #5 clk=0;
+ if (!ramCS && !writeEnable) begin
+ dynamicMem[address]=data;
+ end
+`ifdef HARDWARE_PRINT
+ if (!printEnable) begin
+ $display("PRINT: %c", data);
+ end
+`endif
+ if (b.state==`STATE_HALT) begin
+ $display("Halt");
+ i=10000000;
+ end
+ if (b.operNum==`OP_VAR && b.op==`OPVAR_GETTOUCH && b.phase==0 && b.state==`STATE_DO_OP) begin
+ b.phase=3;
+ if ($fscanf(touch, "%d %d %d", touchX, touchY, touchZ)==-1) begin
+ i=10000000;
+ end
+ $display("Read: %d %d %d", touchX, touchY, touchZ);
+ case (b.operand[0])
+ 'h93: b.operand[1]=touchZ;
+ 'h95: b.operand[1]=touchX;
+ 'h1A: b.operand[1]=touchY;
+ default: begin $display("Bad touch"); $finish; end
+ endcase
+ end
+ if (b.operNum==`OP_VAR && b.op==`OPVAR_BLIT1 && b.phase==0 && b.state==`STATE_DO_OP) begin
+ b.operand[1]=1;
+ end
+ //if (b.curPC<'h1e000)
+ begin
+ if (b.state==`STATE_FETCH_OP) begin
+ ops[b.op+(32*b.operNum)]=ops[b.op+(32*b.operNum)]+1;
+ numOps=numOps+1;
+ end
+ if (b.state!=`STATE_RESET) begin
+ cycles=cycles+1;
+ opCycles[b.op+(32*b.operNum)]=opCycles[b.op+(32*b.operNum)]+1;
+ end
+ stateCycles[b.state]=stateCycles[b.state]+1;
+ end
+ $display("Mem req: %h WE:%d PE:%d%d%d%d RoS:%d RaS:%d D:%h LEDs:%d%d%d ADC:%d%d%d%d%d%d%d Ops:%d/%d", address, !writeEnable, printEnable, lcdCS, lcdRS, lcdReset, !romCS, !ramCS, data, !led0, !led1, !led2, !adcCS, address[0], address[1], lcdRS, printEnable, data[7], data[6], numOps, cycles);
+ end
+ for (i=0; i<16; i=i+1)
+ if (stateCycles[i]>0)
+ $display("State:%d %d", i, stateCycles[i]);
+ for (i=0; i<256; i=i+1)
+ if (opCycles[i]>0)
+ $display("OpCyc:%d/%d %d/%d=%d", i/32, i%32, opCycles[i], ops[i], opCycles[i]/ops[i]);
+ file=$fopen("ram.dat", "wb");
+ for (i=0; i<'h20000; i=i+16) begin
+ $fwrite(file, "%05h: ", i);
+ for (j=0; j<16; j=j+2)
+ $fwrite(file, "%02x%02x ", dynamicMem[i+j][7:0], dynamicMem[i+j+1][7:0]);
+ for (j=0; j<16; j=j+1)
+ $fwrite(file, "%c", (dynamicMem[i+j][7:0]>=32)?dynamicMem[i+j][7:0]:46);
+ $fwrite(file, "\n");
+ end
+ $fwrite(file, "\nBitmap:\n");
+ for (i='h1b500; i<'h20000; i=i+30) begin
+ for (j=0; j<30; j=j+1)
+ $fwrite(file, "%d%d%d%d%d%d%d%d",
+ dynamicMem[i+j][7], dynamicMem[i+j][6],
+ dynamicMem[i+j][5], dynamicMem[i+j][4],
+ dynamicMem[i+j][3], dynamicMem[i+j][2],
+ dynamicMem[i+j][1], dynamicMem[i+j][0]);
+ $fwrite(file, "\n");
+ end
+ $fclose(file);
+ $fclose(touch);
+ $finish;
+end
+
+boss b(clk, adcDout, reset, data, address, writeEnable, printEnable, romCS, ramCS, led0, led1, led2, lcdCS, lcdRS, lcdReset, adcCS, a17, a18);
+
+endmodule
trunk/altera/ZMachine.v
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/altera/ZMachine.qpf
===================================================================
--- trunk/altera/ZMachine.qpf (nonexistent)
+++ trunk/altera/ZMachine.qpf (revision 2)
@@ -0,0 +1,30 @@
+# -------------------------------------------------------------------------- #
+#
+# Copyright (C) 1991-2013 Altera Corporation
+# Your use of Altera Corporation's design tools, logic functions
+# and other software and tools, and its AMPP partner logic
+# functions, and any output files from any of the foregoing
+# (including device programming or simulation files), and any
+# associated documentation or information are expressly subject
+# to the terms and conditions of the Altera Program License
+# Subscription Agreement, Altera MegaCore Function License
+# Agreement, or other applicable license agreement, including,
+# without limitation, that your use is for the sole purpose of
+# programming logic devices manufactured by Altera and sold by
+# Altera or its authorized distributors. Please refer to the
+# applicable agreement for further details.
+#
+# -------------------------------------------------------------------------- #
+#
+# Quartus II 64-Bit
+# Version 13.0.1 Build 232 06/12/2013 Service Pack 1 SJ Web Edition
+# Date created = 21:16:13 September 09, 2014
+#
+# -------------------------------------------------------------------------- #
+
+QUARTUS_VERSION = "13.0"
+DATE = "21:16:13 September 09, 2014"
+
+# Revisions
+
+PROJECT_REVISION = "ZMachine"
trunk/altera/ZMachine.qpf
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/altera/ZMachine.qsf
===================================================================
--- trunk/altera/ZMachine.qsf (nonexistent)
+++ trunk/altera/ZMachine.qsf (revision 2)
@@ -0,0 +1,115 @@
+# -------------------------------------------------------------------------- #
+#
+# Copyright (C) 1991-2013 Altera Corporation
+# Your use of Altera Corporation's design tools, logic functions
+# and other software and tools, and its AMPP partner logic
+# functions, and any output files from any of the foregoing
+# (including device programming or simulation files), and any
+# associated documentation or information are expressly subject
+# to the terms and conditions of the Altera Program License
+# Subscription Agreement, Altera MegaCore Function License
+# Agreement, or other applicable license agreement, including,
+# without limitation, that your use is for the sole purpose of
+# programming logic devices manufactured by Altera and sold by
+# Altera or its authorized distributors. Please refer to the
+# applicable agreement for further details.
+#
+# -------------------------------------------------------------------------- #
+#
+# Quartus II 64-Bit
+# Version 13.0.1 Build 232 06/12/2013 Service Pack 1 SJ Web Edition
+# Date created = 21:16:13 September 09, 2014
+#
+# -------------------------------------------------------------------------- #
+#
+# Notes:
+#
+# 1) The default values for assignments are stored in the file:
+# ZMachine_assignment_defaults.qdf
+# If this file doesn't exist, see file:
+# assignment_defaults.qdf
+#
+# 2) Altera recommends that you do not modify this file. This
+# file is updated automatically by the Quartus II software
+# and any changes you make may be lost or overwritten.
+#
+# -------------------------------------------------------------------------- #
+
+
+set_global_assignment -name FAMILY "Cyclone II"
+set_global_assignment -name DEVICE EP2C5T144C8
+set_global_assignment -name TOP_LEVEL_ENTITY ZMachine
+set_global_assignment -name ORIGINAL_QUARTUS_VERSION "13.0 SP1"
+set_global_assignment -name PROJECT_CREATION_TIME_DATE "21:16:13 SEPTEMBER 09, 2014"
+set_global_assignment -name LAST_QUARTUS_VERSION "13.0 SP1"
+set_global_assignment -name PROJECT_OUTPUT_DIRECTORY output_files
+set_global_assignment -name MIN_CORE_JUNCTION_TEMP 0
+set_global_assignment -name MAX_CORE_JUNCTION_TEMP 85
+set_global_assignment -name ERROR_CHECK_FREQUENCY_DIVISOR 1
+set_global_assignment -name EDA_SIMULATION_TOOL "ModelSim-Altera (Verilog)"
+set_global_assignment -name EDA_OUTPUT_DATA_FORMAT "VERILOG HDL" -section_id eda_simulation
+set_global_assignment -name VERILOG_FILE ZMachine.v
+set_global_assignment -name PARTITION_NETLIST_TYPE SOURCE -section_id Top
+set_global_assignment -name PARTITION_FITTER_PRESERVATION_LEVEL PLACEMENT_AND_ROUTING -section_id Top
+set_global_assignment -name PARTITION_COLOR 16764057 -section_id Top
+set_global_assignment -name BDF_FILE ZMachine.bdf
+set_global_assignment -name STRATIX_DEVICE_IO_STANDARD "3.3-V LVTTL"
+set_location_assignment PIN_142 -to address[16]
+set_location_assignment PIN_141 -to address[15]
+set_location_assignment PIN_48 -to address[14]
+set_location_assignment PIN_53 -to address[13]
+set_location_assignment PIN_139 -to address[12]
+set_location_assignment PIN_58 -to address[11]
+set_location_assignment PIN_60 -to address[10]
+set_location_assignment PIN_57 -to address[9]
+set_location_assignment PIN_55 -to address[8]
+set_location_assignment PIN_137 -to address[7]
+set_location_assignment PIN_136 -to address[6]
+set_location_assignment PIN_135 -to address[5]
+set_location_assignment PIN_134 -to address[4]
+set_location_assignment PIN_133 -to address[3]
+set_location_assignment PIN_132 -to address[2]
+set_location_assignment PIN_129 -to address[1]
+set_location_assignment PIN_126 -to address[0]
+set_location_assignment PIN_64 -to data[7]
+set_location_assignment PIN_65 -to data[6]
+set_location_assignment PIN_67 -to data[5]
+set_location_assignment PIN_69 -to data[4]
+set_location_assignment PIN_70 -to data[3]
+set_location_assignment PIN_121 -to data[2]
+set_location_assignment PIN_122 -to data[1]
+set_location_assignment PIN_125 -to data[0]
+set_location_assignment PIN_17 -to osc_clk
+set_location_assignment PIN_52 -to WE
+set_global_assignment -name PHYSICAL_SYNTHESIS_COMBO_LOGIC_FOR_AREA OFF
+set_global_assignment -name PHYSICAL_SYNTHESIS_MAP_LOGIC_TO_MEMORY_FOR_AREA OFF
+set_global_assignment -name PHYSICAL_SYNTHESIS_EFFORT NORMAL
+set_global_assignment -name POWER_PRESET_COOLING_SOLUTION "23 MM HEAT SINK WITH 200 LFPM AIRFLOW"
+set_global_assignment -name POWER_BOARD_THERMAL_MODEL "NONE (CONSERVATIVE)"
+set_location_assignment PIN_43 -to PE
+set_location_assignment PIN_63 -to ramCS
+set_location_assignment PIN_120 -to romCS
+set_global_assignment -name SDC_FILE zmachine.sdc
+set_global_assignment -name CYCLONEII_OPTIMIZATION_TECHNIQUE AREA
+set_global_assignment -name SMART_RECOMPILE ON
+set_global_assignment -name ADV_NETLIST_OPT_SYNTH_WYSIWYG_REMAP ON
+set_global_assignment -name AUTO_SHIFT_REGISTER_RECOGNITION ALWAYS
+set_location_assignment PIN_3 -to led0
+set_location_assignment PIN_7 -to led1
+set_location_assignment PIN_9 -to led2
+set_location_assignment PIN_143 -to A18
+set_location_assignment PIN_118 -to A17
+set_location_assignment PIN_119 -to romOE
+set_location_assignment PIN_51 -to ramCE2
+set_location_assignment PIN_59 -to ramOE
+set_location_assignment PIN_41 -to lcdCS
+set_location_assignment PIN_40 -to lcdReset
+set_location_assignment PIN_42 -to lcdRS
+set_location_assignment PIN_44 -to lcdRD
+set_location_assignment PIN_45 -to nadcCS
+set_location_assignment PIN_47 -to adcDout
+set_instance_assignment -name WEAK_PULL_UP_RESISTOR ON -to adcDout
+set_global_assignment -name QIP_FILE altpll0.qip
+set_location_assignment PIN_144 -to reset
+set_instance_assignment -name WEAK_PULL_UP_RESISTOR ON -to reset
+set_instance_assignment -name PARTITION_HIERARCHY root_partition -to | -section_id Top
\ No newline at end of file
trunk/altera/ZMachine.qsf
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/altera/zmachine.sdc
===================================================================
--- trunk/altera/zmachine.sdc (nonexistent)
+++ trunk/altera/zmachine.sdc (revision 2)
@@ -0,0 +1,3 @@
+create_clock -period 20.000 -name osc_clk osc_clk
+derive_pll_clocks
+derive_clock_uncertainty
\ No newline at end of file
trunk/altera/zmachine.sdc
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/altera/ZMachine.qws
===================================================================
Cannot display: file marked as a binary type.
svn:mime-type = application/octet-stream
Index: trunk/altera/ZMachine.qws
===================================================================
--- trunk/altera/ZMachine.qws (nonexistent)
+++ trunk/altera/ZMachine.qws (revision 2)
trunk/altera/ZMachine.qws
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Added: svn:mime-type
## -0,0 +1 ##
+application/octet-stream
\ No newline at end of property
Index: trunk/altera/ZMachine.bdf
===================================================================
--- trunk/altera/ZMachine.bdf (nonexistent)
+++ trunk/altera/ZMachine.bdf (revision 2)
@@ -0,0 +1,776 @@
+/*
+WARNING: Do NOT edit the input and output ports in this file in a text
+editor if you plan to continue editing the block that represents it in
+the Block Editor! File corruption is VERY likely to occur.
+*/
+/*
+Copyright (C) 1991-2013 Altera Corporation
+Your use of Altera Corporation's design tools, logic functions
+and other software and tools, and its AMPP partner logic
+functions, and any output files from any of the foregoing
+(including device programming or simulation files), and any
+associated documentation or information are expressly subject
+to the terms and conditions of the Altera Program License
+Subscription Agreement, Altera MegaCore Function License
+Agreement, or other applicable license agreement, including,
+without limitation, that your use is for the sole purpose of
+programming logic devices manufactured by Altera and sold by
+Altera or its authorized distributors. Please refer to the
+applicable agreement for further details.
+*/
+(header "graphic" (version "1.4"))
+(pin
+ (input)
+ (rect -368 256 -200 272)
+ (text "INPUT" (rect 125 0 154 10)(font "Arial" (font_size 6)))
+ (text "osc_clk" (rect 5 0 43 11)(font "Arial" ))
+ (pt 168 8)
+ (drawing
+ (line (pt 84 12)(pt 109 12))
+ (line (pt 84 4)(pt 109 4))
+ (line (pt 113 8)(pt 168 8))
+ (line (pt 84 12)(pt 84 4))
+ (line (pt 109 4)(pt 113 8))
+ (line (pt 109 12)(pt 113 8))
+ )
+ (text "VCC" (rect 128 7 149 17)(font "Arial" (font_size 6)))
+ (annotation_block (location)(rect -424 272 -368 288))
+)
+(pin
+ (input)
+ (rect 152 304 320 320)
+ (text "INPUT" (rect 125 0 154 10)(font "Arial" (font_size 6)))
+ (text "adcDout" (rect 5 0 47 11)(font "Arial" ))
+ (pt 168 8)
+ (drawing
+ (line (pt 84 12)(pt 109 12))
+ (line (pt 84 4)(pt 109 4))
+ (line (pt 113 8)(pt 168 8))
+ (line (pt 84 12)(pt 84 4))
+ (line (pt 109 4)(pt 113 8))
+ (line (pt 109 12)(pt 113 8))
+ )
+ (text "VCC" (rect 128 7 149 17)(font "Arial" (font_size 6)))
+ (annotation_block (location)(rect 96 320 152 336))
+)
+(pin
+ (input)
+ (rect 152 320 320 336)
+ (text "INPUT" (rect 125 0 154 10)(font "Arial" (font_size 6)))
+ (text "reset" (rect 5 0 31 11)(font "Arial" ))
+ (pt 168 8)
+ (drawing
+ (line (pt 84 12)(pt 109 12))
+ (line (pt 84 4)(pt 109 4))
+ (line (pt 113 8)(pt 168 8))
+ (line (pt 84 12)(pt 84 4))
+ (line (pt 109 4)(pt 113 8))
+ (line (pt 109 12)(pt 113 8))
+ )
+ (text "VCC" (rect 128 7 149 17)(font "Arial" (font_size 6)))
+)
+(pin
+ (output)
+ (rect 560 304 736 320)
+ (text "OUTPUT" (rect 1 0 41 10)(font "Arial" (font_size 6)))
+ (text "address[16..0]" (rect 90 0 160 11)(font "Arial" ))
+ (pt 0 8)
+ (drawing
+ (line (pt 0 8)(pt 52 8))
+ (line (pt 52 4)(pt 78 4))
+ (line (pt 52 12)(pt 78 12))
+ (line (pt 52 12)(pt 52 4))
+ (line (pt 78 4)(pt 82 8))
+ (line (pt 82 8)(pt 78 12))
+ (line (pt 78 12)(pt 82 8))
+ )
+ (annotation_block (location)(rect 736 320 784 336))
+)
+(pin
+ (output)
+ (rect 560 320 736 336)
+ (text "OUTPUT" (rect 1 0 41 10)(font "Arial" (font_size 6)))
+ (text "WE" (rect 90 0 110 11)(font "Arial" ))
+ (pt 0 8)
+ (drawing
+ (line (pt 0 8)(pt 52 8))
+ (line (pt 52 4)(pt 78 4))
+ (line (pt 52 12)(pt 78 12))
+ (line (pt 52 12)(pt 52 4))
+ (line (pt 78 4)(pt 82 8))
+ (line (pt 82 8)(pt 78 12))
+ (line (pt 78 12)(pt 82 8))
+ )
+ (annotation_block (location)(rect 736 336 792 352))
+)
+(pin
+ (output)
+ (rect 560 368 736 384)
+ (text "OUTPUT" (rect 1 0 41 10)(font "Arial" (font_size 6)))
+ (text "ramCS" (rect 90 0 125 11)(font "Arial" ))
+ (pt 0 8)
+ (drawing
+ (line (pt 0 8)(pt 52 8))
+ (line (pt 52 4)(pt 78 4))
+ (line (pt 52 12)(pt 78 12))
+ (line (pt 52 12)(pt 52 4))
+ (line (pt 78 4)(pt 82 8))
+ (line (pt 82 8)(pt 78 12))
+ (line (pt 78 12)(pt 82 8))
+ )
+ (annotation_block (location)(rect 736 384 792 400))
+)
+(pin
+ (output)
+ (rect 560 352 736 368)
+ (text "OUTPUT" (rect 1 0 41 10)(font "Arial" (font_size 6)))
+ (text "romCS" (rect 90 0 125 11)(font "Arial" ))
+ (pt 0 8)
+ (drawing
+ (line (pt 0 8)(pt 52 8))
+ (line (pt 52 4)(pt 78 4))
+ (line (pt 52 12)(pt 78 12))
+ (line (pt 52 12)(pt 52 4))
+ (line (pt 78 4)(pt 82 8))
+ (line (pt 82 8)(pt 78 12))
+ (line (pt 78 12)(pt 82 8))
+ )
+ (annotation_block (location)(rect 736 368 792 384))
+)
+(pin
+ (output)
+ (rect 560 336 736 352)
+ (text "OUTPUT" (rect 1 0 41 10)(font "Arial" (font_size 6)))
+ (text "PE" (rect 90 0 106 11)(font "Arial" ))
+ (pt 0 8)
+ (drawing
+ (line (pt 0 8)(pt 52 8))
+ (line (pt 52 4)(pt 78 4))
+ (line (pt 52 12)(pt 78 12))
+ (line (pt 52 12)(pt 52 4))
+ (line (pt 78 4)(pt 82 8))
+ (line (pt 82 8)(pt 78 12))
+ (line (pt 78 12)(pt 82 8))
+ )
+ (annotation_block (location)(rect 736 352 792 368))
+)
+(pin
+ (output)
+ (rect 560 384 736 400)
+ (text "OUTPUT" (rect 1 0 41 10)(font "Arial" (font_size 6)))
+ (text "led0" (rect 90 0 112 11)(font "Arial" ))
+ (pt 0 8)
+ (drawing
+ (line (pt 0 8)(pt 52 8))
+ (line (pt 52 4)(pt 78 4))
+ (line (pt 52 12)(pt 78 12))
+ (line (pt 52 12)(pt 52 4))
+ (line (pt 78 4)(pt 82 8))
+ (line (pt 82 8)(pt 78 12))
+ (line (pt 78 12)(pt 82 8))
+ )
+ (annotation_block (location)(rect 736 400 784 416))
+)
+(pin
+ (output)
+ (rect 560 400 736 416)
+ (text "OUTPUT" (rect 1 0 41 10)(font "Arial" (font_size 6)))
+ (text "led1" (rect 90 0 112 11)(font "Arial" ))
+ (pt 0 8)
+ (drawing
+ (line (pt 0 8)(pt 52 8))
+ (line (pt 52 4)(pt 78 4))
+ (line (pt 52 12)(pt 78 12))
+ (line (pt 52 12)(pt 52 4))
+ (line (pt 78 4)(pt 82 8))
+ (line (pt 82 8)(pt 78 12))
+ (line (pt 78 12)(pt 82 8))
+ )
+ (annotation_block (location)(rect 736 416 784 432))
+)
+(pin
+ (output)
+ (rect 560 416 736 432)
+ (text "OUTPUT" (rect 1 0 41 10)(font "Arial" (font_size 6)))
+ (text "led2" (rect 90 0 112 11)(font "Arial" ))
+ (pt 0 8)
+ (drawing
+ (line (pt 0 8)(pt 52 8))
+ (line (pt 52 4)(pt 78 4))
+ (line (pt 52 12)(pt 78 12))
+ (line (pt 52 12)(pt 52 4))
+ (line (pt 78 4)(pt 82 8))
+ (line (pt 82 8)(pt 78 12))
+ (line (pt 78 12)(pt 82 8))
+ )
+ (annotation_block (location)(rect 736 432 784 448))
+)
+(pin
+ (output)
+ (rect 560 512 736 528)
+ (text "OUTPUT" (rect 1 0 41 10)(font "Arial" (font_size 6)))
+ (text "A18" (rect 90 0 111 11)(font "Arial" ))
+ (pt 0 8)
+ (drawing
+ (line (pt 0 8)(pt 52 8))
+ (line (pt 52 4)(pt 78 4))
+ (line (pt 52 12)(pt 78 12))
+ (line (pt 52 12)(pt 52 4))
+ (line (pt 78 4)(pt 82 8))
+ (line (pt 82 8)(pt 78 12))
+ (line (pt 78 12)(pt 82 8))
+ )
+ (annotation_block (location)(rect 736 528 792 544))
+)
+(pin
+ (output)
+ (rect 560 496 736 512)
+ (text "OUTPUT" (rect 1 0 41 10)(font "Arial" (font_size 6)))
+ (text "A17" (rect 90 0 111 11)(font "Arial" ))
+ (pt 0 8)
+ (drawing
+ (line (pt 0 8)(pt 52 8))
+ (line (pt 52 4)(pt 78 4))
+ (line (pt 52 12)(pt 78 12))
+ (line (pt 52 12)(pt 52 4))
+ (line (pt 78 4)(pt 82 8))
+ (line (pt 82 8)(pt 78 12))
+ (line (pt 78 12)(pt 82 8))
+ )
+ (annotation_block (location)(rect 736 512 792 528))
+)
+(pin
+ (output)
+ (rect 296 120 472 136)
+ (text "OUTPUT" (rect 1 0 41 10)(font "Arial" (font_size 6)))
+ (text "romOE" (rect 90 0 126 11)(font "Arial" ))
+ (pt 0 8)
+ (drawing
+ (line (pt 0 8)(pt 52 8))
+ (line (pt 52 4)(pt 78 4))
+ (line (pt 52 12)(pt 78 12))
+ (line (pt 52 12)(pt 52 4))
+ (line (pt 78 4)(pt 82 8))
+ (line (pt 82 8)(pt 78 12))
+ (line (pt 78 12)(pt 82 8))
+ )
+ (annotation_block (location)(rect 472 136 528 152))
+)
+(pin
+ (output)
+ (rect 296 56 472 72)
+ (text "OUTPUT" (rect 1 0 41 10)(font "Arial" (font_size 6)))
+ (text "ramCE2" (rect 90 0 132 11)(font "Arial" ))
+ (pt 0 8)
+ (drawing
+ (line (pt 0 8)(pt 52 8))
+ (line (pt 52 4)(pt 78 4))
+ (line (pt 52 12)(pt 78 12))
+ (line (pt 52 12)(pt 52 4))
+ (line (pt 78 4)(pt 82 8))
+ (line (pt 82 8)(pt 78 12))
+ (line (pt 78 12)(pt 82 8))
+ )
+ (annotation_block (location)(rect 472 72 528 88))
+)
+(pin
+ (output)
+ (rect 296 88 472 104)
+ (text "OUTPUT" (rect 1 0 41 10)(font "Arial" (font_size 6)))
+ (text "ramOE" (rect 90 0 126 11)(font "Arial" ))
+ (pt 0 8)
+ (drawing
+ (line (pt 0 8)(pt 52 8))
+ (line (pt 52 4)(pt 78 4))
+ (line (pt 52 12)(pt 78 12))
+ (line (pt 52 12)(pt 52 4))
+ (line (pt 78 4)(pt 82 8))
+ (line (pt 82 8)(pt 78 12))
+ (line (pt 78 12)(pt 82 8))
+ )
+ (annotation_block (location)(rect 472 104 528 120))
+)
+(pin
+ (output)
+ (rect 560 432 736 448)
+ (text "OUTPUT" (rect 1 0 41 10)(font "Arial" (font_size 6)))
+ (text "lcdCS" (rect 90 0 120 11)(font "Arial" ))
+ (pt 0 8)
+ (drawing
+ (line (pt 0 8)(pt 52 8))
+ (line (pt 52 4)(pt 78 4))
+ (line (pt 52 12)(pt 78 12))
+ (line (pt 52 12)(pt 52 4))
+ (line (pt 78 4)(pt 82 8))
+ (line (pt 82 8)(pt 78 12))
+ (line (pt 78 12)(pt 82 8))
+ )
+ (annotation_block (location)(rect 736 448 792 464))
+)
+(pin
+ (output)
+ (rect 560 448 736 464)
+ (text "OUTPUT" (rect 1 0 41 10)(font "Arial" (font_size 6)))
+ (text "lcdRS" (rect 90 0 120 11)(font "Arial" ))
+ (pt 0 8)
+ (drawing
+ (line (pt 0 8)(pt 52 8))
+ (line (pt 52 4)(pt 78 4))
+ (line (pt 52 12)(pt 78 12))
+ (line (pt 52 12)(pt 52 4))
+ (line (pt 78 4)(pt 82 8))
+ (line (pt 82 8)(pt 78 12))
+ (line (pt 78 12)(pt 82 8))
+ )
+ (annotation_block (location)(rect 736 464 792 480))
+)
+(pin
+ (output)
+ (rect 560 464 736 480)
+ (text "OUTPUT" (rect 1 0 41 10)(font "Arial" (font_size 6)))
+ (text "lcdReset" (rect 90 0 134 11)(font "Arial" ))
+ (pt 0 8)
+ (drawing
+ (line (pt 0 8)(pt 52 8))
+ (line (pt 52 4)(pt 78 4))
+ (line (pt 52 12)(pt 78 12))
+ (line (pt 52 12)(pt 52 4))
+ (line (pt 78 4)(pt 82 8))
+ (line (pt 82 8)(pt 78 12))
+ (line (pt 78 12)(pt 82 8))
+ )
+ (annotation_block (location)(rect 736 480 792 496))
+)
+(pin
+ (output)
+ (rect 296 32 472 48)
+ (text "OUTPUT" (rect 1 0 41 10)(font "Arial" (font_size 6)))
+ (text "lcdRD" (rect 90 0 121 11)(font "Arial" ))
+ (pt 0 8)
+ (drawing
+ (line (pt 0 8)(pt 52 8))
+ (line (pt 52 4)(pt 78 4))
+ (line (pt 52 12)(pt 78 12))
+ (line (pt 52 12)(pt 52 4))
+ (line (pt 78 4)(pt 82 8))
+ (line (pt 82 8)(pt 78 12))
+ (line (pt 78 12)(pt 82 8))
+ )
+ (annotation_block (location)(rect 472 48 528 64))
+)
+(pin
+ (output)
+ (rect 560 480 736 496)
+ (text "OUTPUT" (rect 1 0 41 10)(font "Arial" (font_size 6)))
+ (text "nadcCS" (rect 90 0 131 11)(font "Arial" ))
+ (pt 0 8)
+ (drawing
+ (line (pt 0 8)(pt 52 8))
+ (line (pt 52 4)(pt 78 4))
+ (line (pt 52 12)(pt 78 12))
+ (line (pt 52 12)(pt 52 4))
+ (line (pt 78 4)(pt 82 8))
+ (line (pt 82 8)(pt 78 12))
+ (line (pt 78 12)(pt 82 8))
+ )
+ (annotation_block (location)(rect 736 496 792 512))
+)
+(pin
+ (bidir)
+ (rect 560 288 736 304)
+ (text "BIDIR" (rect 1 0 28 10)(font "Arial" (font_size 6)))
+ (text "data[7..0]" (rect 90 0 136 11)(font "Arial" ))
+ (pt 0 8)
+ (drawing
+ (line (pt 56 4)(pt 78 4))
+ (line (pt 0 8)(pt 52 8))
+ (line (pt 56 12)(pt 78 12))
+ (line (pt 78 4)(pt 82 8))
+ (line (pt 78 12)(pt 82 8))
+ (line (pt 56 4)(pt 52 8))
+ (line (pt 52 8)(pt 56 12))
+ )
+ (text "VCC" (rect 4 7 25 17)(font "Arial" (font_size 6)))
+ (annotation_block (location)(rect 736 304 792 320))
+)
+(symbol
+ (rect 264 200 296 232)
+ (text "GND" (rect 8 16 30 26)(font "Arial" (font_size 6)))
+ (text "inst1" (rect 3 21 27 32)(font "Arial" )(invisible))
+ (port
+ (pt 16 0)
+ (output)
+ (text "1" (rect 18 0 26 11)(font "Courier New" (bold))(invisible))
+ (text "1" (rect 18 0 26 11)(font "Courier New" (bold))(invisible))
+ (line (pt 16 8)(pt 16 0))
+ )
+ (drawing
+ (line (pt 8 8)(pt 16 16))
+ (line (pt 16 16)(pt 24 8))
+ (line (pt 8 8)(pt 24 8))
+ )
+)
+(symbol
+ (rect 264 16 296 32)
+ (text "VCC" (rect 7 0 28 10)(font "Arial" (font_size 6)))
+ (text "ramCE2_1" (rect 3 5 57 16)(font "Arial" )(invisible))
+ (port
+ (pt 16 16)
+ (output)
+ (text "1" (rect 19 7 27 18)(font "Courier New" (bold))(invisible))
+ (text "1" (rect 19 7 27 18)(font "Courier New" (bold))(invisible))
+ (line (pt 16 16)(pt 16 8))
+ )
+ (drawing
+ (line (pt 8 8)(pt 24 8))
+ )
+)
+(symbol
+ (rect -184 200 72 352)
+ (text "altpll0" (rect 111 0 153 16)(font "Arial" (font_size 10)))
+ (text "inst2" (rect 8 137 32 148)(font "Arial" ))
+ (port
+ (pt 0 64)
+ (input)
+ (text "inclk0" (rect 0 0 34 13)(font "Arial" (font_size 8)))
+ (text "inclk0" (rect 4 51 38 64)(font "Arial" (font_size 8)))
+ (line (pt 0 64)(pt 40 64))
+ )
+ (port
+ (pt 256 64)
+ (output)
+ (text "c0" (rect 0 0 15 13)(font "Arial" (font_size 8)))
+ (text "c0" (rect 241 51 256 64)(font "Arial" (font_size 8)))
+ )
+ (port
+ (pt 256 80)
+ (output)
+ (text "locked" (rect 0 0 37 13)(font "Arial" (font_size 8)))
+ (text "locked" (rect 221 67 258 80)(font "Arial" (font_size 8)))
+ )
+ (drawing
+ (text "Cyclone II" (rect 203 138 252 149)(font "Arial" ))
+ (text "inclk0 frequency: 50.000 MHz" (rect 50 60 196 71)(font "Arial" ))
+ (text "Operation Mode: Normal" (rect 50 72 172 83)(font "Arial" ))
+ (text "Clk " (rect 51 91 71 102)(font "Arial" ))
+ (text "Ratio" (rect 71 91 98 102)(font "Arial" ))
+ (text "Ph (dg)" (rect 97 91 134 102)(font "Arial" ))
+ (text "DC (%)" (rect 132 91 169 102)(font "Arial" ))
+ (text "c0" (rect 54 104 66 115)(font "Arial" ))
+ (text "1/5" (rect 76 104 92 115)(font "Arial" ))
+ (text "0.00" (rect 103 104 126 115)(font "Arial" ))
+ (text "50.00" (rect 136 104 165 115)(font "Arial" ))
+ (line (pt 0 0)(pt 257 0))
+ (line (pt 257 0)(pt 257 153))
+ (line (pt 0 153)(pt 257 153))
+ (line (pt 0 0)(pt 0 153))
+ (line (pt 48 89)(pt 164 89))
+ (line (pt 48 101)(pt 164 101))
+ (line (pt 48 114)(pt 164 114))
+ (line (pt 48 89)(pt 48 114))
+ (line (pt 68 89)(pt 68 114)(line_width 3))
+ (line (pt 94 89)(pt 94 114)(line_width 3))
+ (line (pt 129 89)(pt 129 114)(line_width 3))
+ (line (pt 163 89)(pt 163 114))
+ (line (pt 40 48)(pt 207 48))
+ (line (pt 207 48)(pt 207 135))
+ (line (pt 40 135)(pt 207 135))
+ (line (pt 40 48)(pt 40 135))
+ (line (pt 255 64)(pt 207 64))
+ (line (pt 255 80)(pt 207 80))
+ )
+)
+(symbol
+ (rect 96 248 160 296)
+ (text "AND2" (rect 1 0 29 10)(font "Arial" (font_size 6)))
+ (text "inst3" (rect 3 37 27 48)(font "Arial" ))
+ (port
+ (pt 0 16)
+ (input)
+ (text "IN1" (rect 2 7 23 18)(font "Courier New" (bold))(invisible))
+ (text "IN1" (rect 2 7 23 18)(font "Courier New" (bold))(invisible))
+ (line (pt 0 16)(pt 14 16))
+ )
+ (port
+ (pt 0 32)
+ (input)
+ (text "IN2" (rect 2 23 23 34)(font "Courier New" (bold))(invisible))
+ (text "IN2" (rect 2 23 23 34)(font "Courier New" (bold))(invisible))
+ (line (pt 0 32)(pt 14 32))
+ )
+ (port
+ (pt 64 24)
+ (output)
+ (text "OUT" (rect 48 15 69 26)(font "Courier New" (bold))(invisible))
+ (text "OUT" (rect 48 15 69 26)(font "Courier New" (bold))(invisible))
+ (line (pt 42 24)(pt 64 24))
+ )
+ (drawing
+ (line (pt 14 12)(pt 30 12))
+ (line (pt 14 37)(pt 31 37))
+ (line (pt 14 12)(pt 14 37))
+ (arc (pt 31 37)(pt 30 12)(rect 18 12 43 37))
+ )
+)
+(symbol
+ (rect 336 264 536 568)
+ (text "boss" (rect 5 0 29 11)(font "Arial" ))
+ (text "inst" (rect 8 288 26 299)(font "Arial" ))
+ (port
+ (pt 0 32)
+ (input)
+ (text "clk" (rect 0 0 15 11)(font "Arial" ))
+ (text "clk" (rect 21 27 36 38)(font "Arial" ))
+ (line (pt 0 32)(pt 16 32))
+ )
+ (port
+ (pt 0 48)
+ (input)
+ (text "adcDout" (rect 0 0 42 11)(font "Arial" ))
+ (text "adcDout" (rect 21 43 63 54)(font "Arial" ))
+ (line (pt 0 48)(pt 16 48))
+ )
+ (port
+ (pt 0 64)
+ (input)
+ (text "reset" (rect 0 0 25 11)(font "Arial" ))
+ (text "reset" (rect 21 59 46 70)(font "Arial" ))
+ (line (pt 0 64)(pt 16 64))
+ )
+ (port
+ (pt 200 48)
+ (output)
+ (text "waddress[16..0]" (rect 0 0 77 11)(font "Arial" ))
+ (text "waddress[16..0]" (rect 114 43 179 54)(font "Arial" ))
+ (line (pt 200 48)(pt 184 48)(line_width 3))
+ )
+ (port
+ (pt 200 64)
+ (output)
+ (text "we" (rect 0 0 15 11)(font "Arial" ))
+ (text "we" (rect 167 59 179 70)(font "Arial" ))
+ (line (pt 200 64)(pt 184 64))
+ )
+ (port
+ (pt 200 80)
+ (output)
+ (text "pe" (rect 0 0 14 11)(font "Arial" ))
+ (text "pe" (rect 168 75 179 86)(font "Arial" ))
+ (line (pt 200 80)(pt 184 80))
+ )
+ (port
+ (pt 200 96)
+ (output)
+ (text "romCS" (rect 0 0 35 11)(font "Arial" ))
+ (text "romCS" (rect 150 91 179 102)(font "Arial" ))
+ (line (pt 200 96)(pt 184 96))
+ )
+ (port
+ (pt 200 112)
+ (output)
+ (text "ramCS" (rect 0 0 35 11)(font "Arial" ))
+ (text "ramCS" (rect 150 107 179 118)(font "Arial" ))
+ (line (pt 200 112)(pt 184 112))
+ )
+ (port
+ (pt 200 128)
+ (output)
+ (text "led0" (rect 0 0 22 11)(font "Arial" ))
+ (text "led0" (rect 161 123 179 134)(font "Arial" ))
+ (line (pt 200 128)(pt 184 128))
+ )
+ (port
+ (pt 200 144)
+ (output)
+ (text "led1" (rect 0 0 22 11)(font "Arial" ))
+ (text "led1" (rect 161 139 179 150)(font "Arial" ))
+ (line (pt 200 144)(pt 184 144))
+ )
+ (port
+ (pt 200 160)
+ (output)
+ (text "led2" (rect 0 0 22 11)(font "Arial" ))
+ (text "led2" (rect 161 155 179 166)(font "Arial" ))
+ (line (pt 200 160)(pt 184 160))
+ )
+ (port
+ (pt 200 176)
+ (output)
+ (text "lcdCS" (rect 0 0 30 11)(font "Arial" ))
+ (text "lcdCS" (rect 154 171 179 182)(font "Arial" ))
+ (line (pt 200 176)(pt 184 176))
+ )
+ (port
+ (pt 200 192)
+ (output)
+ (text "lcdRS" (rect 0 0 30 11)(font "Arial" ))
+ (text "lcdRS" (rect 154 187 179 198)(font "Arial" ))
+ (line (pt 200 192)(pt 184 192))
+ )
+ (port
+ (pt 200 208)
+ (output)
+ (text "lcdReset" (rect 0 0 44 11)(font "Arial" ))
+ (text "lcdReset" (rect 142 203 179 214)(font "Arial" ))
+ (line (pt 200 208)(pt 184 208))
+ )
+ (port
+ (pt 200 224)
+ (output)
+ (text "nadcCS" (rect 0 0 41 11)(font "Arial" ))
+ (text "nadcCS" (rect 145 219 179 230)(font "Arial" ))
+ (line (pt 200 224)(pt 184 224))
+ )
+ (port
+ (pt 200 240)
+ (output)
+ (text "a17" (rect 0 0 20 11)(font "Arial" ))
+ (text "a17" (rect 163 235 179 246)(font "Arial" ))
+ (line (pt 200 240)(pt 184 240))
+ )
+ (port
+ (pt 200 256)
+ (output)
+ (text "a18" (rect 0 0 20 11)(font "Arial" ))
+ (text "a18" (rect 163 251 179 262)(font "Arial" ))
+ (line (pt 200 256)(pt 184 256))
+ )
+ (port
+ (pt 200 32)
+ (bidir)
+ (text "data[7..0]" (rect 0 0 46 11)(font "Arial" ))
+ (text "data[7..0]" (rect 143 27 189 38)(font "Arial" ))
+ (line (pt 200 32)(pt 184 32)(line_width 3))
+ )
+ (drawing
+ (rectangle (rect 16 16 184 288))
+ )
+)
+(connector
+ (pt 296 128)
+ (pt 280 128)
+)
+(connector
+ (pt 280 96)
+ (pt 280 128)
+)
+(connector
+ (pt 280 96)
+ (pt 296 96)
+)
+(connector
+ (pt 296 64)
+ (pt 280 64)
+)
+(connector
+ (pt 296 40)
+ (pt 280 40)
+)
+(connector
+ (pt 280 32)
+ (pt 280 40)
+)
+(connector
+ (pt 280 40)
+ (pt 280 64)
+)
+(connector
+ (pt 560 296)
+ (pt 536 296)
+ (bus)
+)
+(connector
+ (pt 320 312)
+ (pt 336 312)
+)
+(connector
+ (pt 560 312)
+ (pt 536 312)
+ (bus)
+)
+(connector
+ (pt 560 328)
+ (pt 536 328)
+)
+(connector
+ (pt 560 344)
+ (pt 536 344)
+)
+(connector
+ (pt 560 360)
+ (pt 536 360)
+)
+(connector
+ (pt 560 376)
+ (pt 536 376)
+)
+(connector
+ (pt 560 392)
+ (pt 536 392)
+)
+(connector
+ (pt 560 408)
+ (pt 536 408)
+)
+(connector
+ (pt 560 424)
+ (pt 536 424)
+)
+(connector
+ (pt 560 440)
+ (pt 536 440)
+)
+(connector
+ (pt 560 456)
+ (pt 536 456)
+)
+(connector
+ (pt 560 472)
+ (pt 536 472)
+)
+(connector
+ (pt 560 488)
+ (pt 536 488)
+)
+(connector
+ (pt 536 504)
+ (pt 560 504)
+)
+(connector
+ (pt 280 128)
+ (pt 280 200)
+)
+(connector
+ (pt 536 520)
+ (pt 560 520)
+)
+(connector
+ (pt -200 264)
+ (pt -184 264)
+)
+(connector
+ (pt 72 264)
+ (pt 96 264)
+)
+(connector
+ (pt 72 280)
+ (pt 96 280)
+)
+(connector
+ (pt 160 272)
+ (pt 248 272)
+)
+(connector
+ (pt 248 272)
+ (pt 248 296)
+)
+(connector
+ (pt 336 296)
+ (pt 248 296)
+)
+(connector
+ (pt 336 328)
+ (pt 320 328)
+)
+(junction (pt 280 128))
+(junction (pt 280 40))
trunk/altera/ZMachine.bdf
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/altera/altpll0.v
===================================================================
--- trunk/altera/altpll0.v (nonexistent)
+++ trunk/altera/altpll0.v (revision 2)
@@ -0,0 +1,312 @@
+// megafunction wizard: %ALTPLL%
+// GENERATION: STANDARD
+// VERSION: WM1.0
+// MODULE: altpll
+
+// ============================================================
+// File Name: altpll0.v
+// Megafunction Name(s):
+// altpll
+//
+// Simulation Library Files(s):
+// altera_mf
+// ============================================================
+// ************************************************************
+// THIS IS A WIZARD-GENERATED FILE. DO NOT EDIT THIS FILE!
+//
+// 13.0.1 Build 232 06/12/2013 SP 1 SJ Web Edition
+// ************************************************************
+
+
+//Copyright (C) 1991-2013 Altera Corporation
+//Your use of Altera Corporation's design tools, logic functions
+//and other software and tools, and its AMPP partner logic
+//functions, and any output files from any of the foregoing
+//(including device programming or simulation files), and any
+//associated documentation or information are expressly subject
+//to the terms and conditions of the Altera Program License
+//Subscription Agreement, Altera MegaCore Function License
+//Agreement, or other applicable license agreement, including,
+//without limitation, that your use is for the sole purpose of
+//programming logic devices manufactured by Altera and sold by
+//Altera or its authorized distributors. Please refer to the
+//applicable agreement for further details.
+
+
+// synopsys translate_off
+`timescale 1 ps / 1 ps
+// synopsys translate_on
+module altpll0 (
+ inclk0,
+ c0,
+ locked);
+
+ input inclk0;
+ output c0;
+ output locked;
+
+ wire [5:0] sub_wire0;
+ wire sub_wire2;
+ wire [0:0] sub_wire5 = 1'h0;
+ wire [0:0] sub_wire1 = sub_wire0[0:0];
+ wire c0 = sub_wire1;
+ wire locked = sub_wire2;
+ wire sub_wire3 = inclk0;
+ wire [1:0] sub_wire4 = {sub_wire5, sub_wire3};
+
+ altpll altpll_component (
+ .inclk (sub_wire4),
+ .clk (sub_wire0),
+ .locked (sub_wire2),
+ .activeclock (),
+ .areset (1'b0),
+ .clkbad (),
+ .clkena ({6{1'b1}}),
+ .clkloss (),
+ .clkswitch (1'b0),
+ .configupdate (1'b0),
+ .enable0 (),
+ .enable1 (),
+ .extclk (),
+ .extclkena ({4{1'b1}}),
+ .fbin (1'b1),
+ .fbmimicbidir (),
+ .fbout (),
+ .fref (),
+ .icdrclk (),
+ .pfdena (1'b1),
+ .phasecounterselect ({4{1'b1}}),
+ .phasedone (),
+ .phasestep (1'b1),
+ .phaseupdown (1'b1),
+ .pllena (1'b1),
+ .scanaclr (1'b0),
+ .scanclk (1'b0),
+ .scanclkena (1'b1),
+ .scandata (1'b0),
+ .scandataout (),
+ .scandone (),
+ .scanread (1'b0),
+ .scanwrite (1'b0),
+ .sclkout0 (),
+ .sclkout1 (),
+ .vcooverrange (),
+ .vcounderrange ());
+ defparam
+ altpll_component.clk0_divide_by = 5,
+ altpll_component.clk0_duty_cycle = 50,
+ altpll_component.clk0_multiply_by = 1,
+ altpll_component.clk0_phase_shift = "0",
+ altpll_component.compensate_clock = "CLK0",
+ altpll_component.gate_lock_counter = 1048575,
+ altpll_component.gate_lock_signal = "YES",
+ altpll_component.inclk0_input_frequency = 20000,
+ altpll_component.intended_device_family = "Cyclone II",
+ altpll_component.invalid_lock_multiplier = 5,
+ altpll_component.lpm_hint = "CBX_MODULE_PREFIX=altpll0",
+ altpll_component.lpm_type = "altpll",
+ altpll_component.operation_mode = "NORMAL",
+ altpll_component.port_activeclock = "PORT_UNUSED",
+ altpll_component.port_areset = "PORT_UNUSED",
+ altpll_component.port_clkbad0 = "PORT_UNUSED",
+ altpll_component.port_clkbad1 = "PORT_UNUSED",
+ altpll_component.port_clkloss = "PORT_UNUSED",
+ altpll_component.port_clkswitch = "PORT_UNUSED",
+ altpll_component.port_configupdate = "PORT_UNUSED",
+ altpll_component.port_fbin = "PORT_UNUSED",
+ altpll_component.port_inclk0 = "PORT_USED",
+ altpll_component.port_inclk1 = "PORT_UNUSED",
+ altpll_component.port_locked = "PORT_USED",
+ altpll_component.port_pfdena = "PORT_UNUSED",
+ altpll_component.port_phasecounterselect = "PORT_UNUSED",
+ altpll_component.port_phasedone = "PORT_UNUSED",
+ altpll_component.port_phasestep = "PORT_UNUSED",
+ altpll_component.port_phaseupdown = "PORT_UNUSED",
+ altpll_component.port_pllena = "PORT_UNUSED",
+ altpll_component.port_scanaclr = "PORT_UNUSED",
+ altpll_component.port_scanclk = "PORT_UNUSED",
+ altpll_component.port_scanclkena = "PORT_UNUSED",
+ altpll_component.port_scandata = "PORT_UNUSED",
+ altpll_component.port_scandataout = "PORT_UNUSED",
+ altpll_component.port_scandone = "PORT_UNUSED",
+ altpll_component.port_scanread = "PORT_UNUSED",
+ altpll_component.port_scanwrite = "PORT_UNUSED",
+ altpll_component.port_clk0 = "PORT_USED",
+ altpll_component.port_clk1 = "PORT_UNUSED",
+ altpll_component.port_clk2 = "PORT_UNUSED",
+ altpll_component.port_clk3 = "PORT_UNUSED",
+ altpll_component.port_clk4 = "PORT_UNUSED",
+ altpll_component.port_clk5 = "PORT_UNUSED",
+ altpll_component.port_clkena0 = "PORT_UNUSED",
+ altpll_component.port_clkena1 = "PORT_UNUSED",
+ altpll_component.port_clkena2 = "PORT_UNUSED",
+ altpll_component.port_clkena3 = "PORT_UNUSED",
+ altpll_component.port_clkena4 = "PORT_UNUSED",
+ altpll_component.port_clkena5 = "PORT_UNUSED",
+ altpll_component.port_extclk0 = "PORT_UNUSED",
+ altpll_component.port_extclk1 = "PORT_UNUSED",
+ altpll_component.port_extclk2 = "PORT_UNUSED",
+ altpll_component.port_extclk3 = "PORT_UNUSED",
+ altpll_component.valid_lock_multiplier = 1;
+
+
+endmodule
+
+// ============================================================
+// CNX file retrieval info
+// ============================================================
+// Retrieval info: PRIVATE: ACTIVECLK_CHECK STRING "0"
+// Retrieval info: PRIVATE: BANDWIDTH STRING "1.000"
+// Retrieval info: PRIVATE: BANDWIDTH_FEATURE_ENABLED STRING "0"
+// Retrieval info: PRIVATE: BANDWIDTH_FREQ_UNIT STRING "MHz"
+// Retrieval info: PRIVATE: BANDWIDTH_PRESET STRING "Low"
+// Retrieval info: PRIVATE: BANDWIDTH_USE_AUTO STRING "1"
+// Retrieval info: PRIVATE: BANDWIDTH_USE_CUSTOM STRING "0"
+// Retrieval info: PRIVATE: BANDWIDTH_USE_PRESET STRING "0"
+// Retrieval info: PRIVATE: CLKBAD_SWITCHOVER_CHECK STRING "0"
+// Retrieval info: PRIVATE: CLKLOSS_CHECK STRING "0"
+// Retrieval info: PRIVATE: CLKSWITCH_CHECK STRING "1"
+// Retrieval info: PRIVATE: CNX_NO_COMPENSATE_RADIO STRING "0"
+// Retrieval info: PRIVATE: CREATE_CLKBAD_CHECK STRING "0"
+// Retrieval info: PRIVATE: CREATE_INCLK1_CHECK STRING "0"
+// Retrieval info: PRIVATE: CUR_DEDICATED_CLK STRING "c0"
+// Retrieval info: PRIVATE: CUR_FBIN_CLK STRING "c0"
+// Retrieval info: PRIVATE: DEVICE_SPEED_GRADE STRING "8"
+// Retrieval info: PRIVATE: DIV_FACTOR0 NUMERIC "1"
+// Retrieval info: PRIVATE: DUTY_CYCLE0 STRING "50.00000000"
+// Retrieval info: PRIVATE: EFF_OUTPUT_FREQ_VALUE0 STRING "10.000000"
+// Retrieval info: PRIVATE: EXPLICIT_SWITCHOVER_COUNTER STRING "0"
+// Retrieval info: PRIVATE: EXT_FEEDBACK_RADIO STRING "0"
+// Retrieval info: PRIVATE: GLOCKED_COUNTER_EDIT_CHANGED STRING "1"
+// Retrieval info: PRIVATE: GLOCKED_FEATURE_ENABLED STRING "1"
+// Retrieval info: PRIVATE: GLOCKED_MODE_CHECK STRING "1"
+// Retrieval info: PRIVATE: GLOCK_COUNTER_EDIT NUMERIC "1048575"
+// Retrieval info: PRIVATE: HAS_MANUAL_SWITCHOVER STRING "1"
+// Retrieval info: PRIVATE: INCLK0_FREQ_EDIT STRING "50.000"
+// Retrieval info: PRIVATE: INCLK0_FREQ_UNIT_COMBO STRING "MHz"
+// Retrieval info: PRIVATE: INCLK1_FREQ_EDIT STRING "100.000"
+// Retrieval info: PRIVATE: INCLK1_FREQ_EDIT_CHANGED STRING "1"
+// Retrieval info: PRIVATE: INCLK1_FREQ_UNIT_CHANGED STRING "1"
+// Retrieval info: PRIVATE: INCLK1_FREQ_UNIT_COMBO STRING "MHz"
+// Retrieval info: PRIVATE: INTENDED_DEVICE_FAMILY STRING "Cyclone II"
+// Retrieval info: PRIVATE: INT_FEEDBACK__MODE_RADIO STRING "1"
+// Retrieval info: PRIVATE: LOCKED_OUTPUT_CHECK STRING "1"
+// Retrieval info: PRIVATE: LONG_SCAN_RADIO STRING "1"
+// Retrieval info: PRIVATE: LVDS_MODE_DATA_RATE STRING "Not Available"
+// Retrieval info: PRIVATE: LVDS_MODE_DATA_RATE_DIRTY NUMERIC "0"
+// Retrieval info: PRIVATE: LVDS_PHASE_SHIFT_UNIT0 STRING "deg"
+// Retrieval info: PRIVATE: MIG_DEVICE_SPEED_GRADE STRING "Any"
+// Retrieval info: PRIVATE: MIRROR_CLK0 STRING "0"
+// Retrieval info: PRIVATE: MULT_FACTOR0 NUMERIC "1"
+// Retrieval info: PRIVATE: NORMAL_MODE_RADIO STRING "1"
+// Retrieval info: PRIVATE: OUTPUT_FREQ0 STRING "10.00000000"
+// Retrieval info: PRIVATE: OUTPUT_FREQ_MODE0 STRING "1"
+// Retrieval info: PRIVATE: OUTPUT_FREQ_UNIT0 STRING "MHz"
+// Retrieval info: PRIVATE: PHASE_RECONFIG_FEATURE_ENABLED STRING "0"
+// Retrieval info: PRIVATE: PHASE_RECONFIG_INPUTS_CHECK STRING "0"
+// Retrieval info: PRIVATE: PHASE_SHIFT0 STRING "0.00000000"
+// Retrieval info: PRIVATE: PHASE_SHIFT_STEP_ENABLED_CHECK STRING "0"
+// Retrieval info: PRIVATE: PHASE_SHIFT_UNIT0 STRING "deg"
+// Retrieval info: PRIVATE: PLL_ADVANCED_PARAM_CHECK STRING "0"
+// Retrieval info: PRIVATE: PLL_ARESET_CHECK STRING "0"
+// Retrieval info: PRIVATE: PLL_AUTOPLL_CHECK NUMERIC "1"
+// Retrieval info: PRIVATE: PLL_ENA_CHECK STRING "0"
+// Retrieval info: PRIVATE: PLL_ENHPLL_CHECK NUMERIC "0"
+// Retrieval info: PRIVATE: PLL_FASTPLL_CHECK NUMERIC "0"
+// Retrieval info: PRIVATE: PLL_FBMIMIC_CHECK STRING "0"
+// Retrieval info: PRIVATE: PLL_LVDS_PLL_CHECK NUMERIC "0"
+// Retrieval info: PRIVATE: PLL_PFDENA_CHECK STRING "0"
+// Retrieval info: PRIVATE: PLL_TARGET_HARCOPY_CHECK NUMERIC "0"
+// Retrieval info: PRIVATE: PRIMARY_CLK_COMBO STRING "inclk0"
+// Retrieval info: PRIVATE: RECONFIG_FILE STRING "altpll0.mif"
+// Retrieval info: PRIVATE: SACN_INPUTS_CHECK STRING "0"
+// Retrieval info: PRIVATE: SCAN_FEATURE_ENABLED STRING "0"
+// Retrieval info: PRIVATE: SELF_RESET_LOCK_LOSS STRING "0"
+// Retrieval info: PRIVATE: SHORT_SCAN_RADIO STRING "0"
+// Retrieval info: PRIVATE: SPREAD_FEATURE_ENABLED STRING "0"
+// Retrieval info: PRIVATE: SPREAD_FREQ STRING "50.000"
+// Retrieval info: PRIVATE: SPREAD_FREQ_UNIT STRING "KHz"
+// Retrieval info: PRIVATE: SPREAD_PERCENT STRING "0.500"
+// Retrieval info: PRIVATE: SPREAD_USE STRING "0"
+// Retrieval info: PRIVATE: SRC_SYNCH_COMP_RADIO STRING "0"
+// Retrieval info: PRIVATE: STICKY_CLK0 STRING "1"
+// Retrieval info: PRIVATE: SWITCHOVER_COUNT_EDIT NUMERIC "1"
+// Retrieval info: PRIVATE: SWITCHOVER_FEATURE_ENABLED STRING "1"
+// Retrieval info: PRIVATE: SYNTH_WRAPPER_GEN_POSTFIX STRING "0"
+// Retrieval info: PRIVATE: USE_CLK0 STRING "1"
+// Retrieval info: PRIVATE: USE_CLKENA0 STRING "0"
+// Retrieval info: PRIVATE: USE_MIL_SPEED_GRADE NUMERIC "0"
+// Retrieval info: PRIVATE: ZERO_DELAY_RADIO STRING "0"
+// Retrieval info: LIBRARY: altera_mf altera_mf.altera_mf_components.all
+// Retrieval info: CONSTANT: CLK0_DIVIDE_BY NUMERIC "5"
+// Retrieval info: CONSTANT: CLK0_DUTY_CYCLE NUMERIC "50"
+// Retrieval info: CONSTANT: CLK0_MULTIPLY_BY NUMERIC "1"
+// Retrieval info: CONSTANT: CLK0_PHASE_SHIFT STRING "0"
+// Retrieval info: CONSTANT: COMPENSATE_CLOCK STRING "CLK0"
+// Retrieval info: CONSTANT: GATE_LOCK_COUNTER NUMERIC "1048575"
+// Retrieval info: CONSTANT: GATE_LOCK_SIGNAL STRING "YES"
+// Retrieval info: CONSTANT: INCLK0_INPUT_FREQUENCY NUMERIC "20000"
+// Retrieval info: CONSTANT: INTENDED_DEVICE_FAMILY STRING "Cyclone II"
+// Retrieval info: CONSTANT: INVALID_LOCK_MULTIPLIER NUMERIC "5"
+// Retrieval info: CONSTANT: LPM_TYPE STRING "altpll"
+// Retrieval info: CONSTANT: OPERATION_MODE STRING "NORMAL"
+// Retrieval info: CONSTANT: PORT_ACTIVECLOCK STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_ARESET STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_CLKBAD0 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_CLKBAD1 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_CLKLOSS STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_CLKSWITCH STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_CONFIGUPDATE STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_FBIN STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_INCLK0 STRING "PORT_USED"
+// Retrieval info: CONSTANT: PORT_INCLK1 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_LOCKED STRING "PORT_USED"
+// Retrieval info: CONSTANT: PORT_PFDENA STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_PHASECOUNTERSELECT STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_PHASEDONE STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_PHASESTEP STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_PHASEUPDOWN STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_PLLENA STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_SCANACLR STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_SCANCLK STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_SCANCLKENA STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_SCANDATA STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_SCANDATAOUT STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_SCANDONE STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_SCANREAD STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_SCANWRITE STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clk0 STRING "PORT_USED"
+// Retrieval info: CONSTANT: PORT_clk1 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clk2 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clk3 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clk4 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clk5 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clkena0 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clkena1 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clkena2 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clkena3 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clkena4 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_clkena5 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_extclk0 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_extclk1 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_extclk2 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: PORT_extclk3 STRING "PORT_UNUSED"
+// Retrieval info: CONSTANT: VALID_LOCK_MULTIPLIER NUMERIC "1"
+// Retrieval info: USED_PORT: @clk 0 0 6 0 OUTPUT_CLK_EXT VCC "@clk[5..0]"
+// Retrieval info: USED_PORT: @extclk 0 0 4 0 OUTPUT_CLK_EXT VCC "@extclk[3..0]"
+// Retrieval info: USED_PORT: c0 0 0 0 0 OUTPUT_CLK_EXT VCC "c0"
+// Retrieval info: USED_PORT: inclk0 0 0 0 0 INPUT_CLK_EXT GND "inclk0"
+// Retrieval info: USED_PORT: locked 0 0 0 0 OUTPUT GND "locked"
+// Retrieval info: CONNECT: @inclk 0 0 1 1 GND 0 0 0 0
+// Retrieval info: CONNECT: @inclk 0 0 1 0 inclk0 0 0 0 0
+// Retrieval info: CONNECT: c0 0 0 0 0 @clk 0 0 1 0
+// Retrieval info: CONNECT: locked 0 0 0 0 @locked 0 0 0 0
+// Retrieval info: GEN_FILE: TYPE_NORMAL altpll0.v TRUE
+// Retrieval info: GEN_FILE: TYPE_NORMAL altpll0.ppf TRUE
+// Retrieval info: GEN_FILE: TYPE_NORMAL altpll0.inc FALSE
+// Retrieval info: GEN_FILE: TYPE_NORMAL altpll0.cmp FALSE
+// Retrieval info: GEN_FILE: TYPE_NORMAL altpll0.bsf TRUE
+// Retrieval info: GEN_FILE: TYPE_NORMAL altpll0_inst.v FALSE
+// Retrieval info: GEN_FILE: TYPE_NORMAL altpll0_bb.v TRUE
+// Retrieval info: LIB_FILE: altera_mf
+// Retrieval info: CBX_MODULE_PREFIX: ON
trunk/altera/altpll0.v
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/altera/altpll0.ppf
===================================================================
--- trunk/altera/altpll0.ppf (nonexistent)
+++ trunk/altera/altpll0.ppf (revision 2)
@@ -0,0 +1,10 @@
+
+
+
+
+
+
+
+
+
+
trunk/altera/altpll0.ppf
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/altera/main.bsf
===================================================================
--- trunk/altera/main.bsf (nonexistent)
+++ trunk/altera/main.bsf (revision 2)
@@ -0,0 +1,29 @@
+/*
+WARNING: Do NOT edit the input and output ports in this file in a text
+editor if you plan to continue editing the block that represents it in
+the Block Editor! File corruption is VERY likely to occur.
+*/
+/*
+Copyright (C) 1991-2013 Altera Corporation
+Your use of Altera Corporation's design tools, logic functions
+and other software and tools, and its AMPP partner logic
+functions, and any output files from any of the foregoing
+(including device programming or simulation files), and any
+associated documentation or information are expressly subject
+to the terms and conditions of the Altera Program License
+Subscription Agreement, Altera MegaCore Function License
+Agreement, or other applicable license agreement, including,
+without limitation, that your use is for the sole purpose of
+programming logic devices manufactured by Altera and sold by
+Altera or its authorized distributors. Please refer to the
+applicable agreement for further details.
+*/
+(header "symbol" (version "1.1"))
+(symbol
+ (rect 16 16 64 64)
+ (text "main" (rect 5 0 23 12)(font "Arial" ))
+ (text "inst" (rect 8 32 20 44)(font "Arial" ))
+ (drawing
+ (rectangle (rect 16 16 32 32)(line_width 1))
+ )
+)
trunk/altera/main.bsf
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/benchmark/benchmark.inf
===================================================================
--- trunk/benchmark/benchmark.inf (nonexistent)
+++ trunk/benchmark/benchmark.inf (revision 2)
@@ -0,0 +1,662 @@
+[Ackermann
+m n;
+if (m==0)
+ return n+1;
+else if (n==0)
+ return Ackermann(m-1,1);
+else
+ return Ackermann(m-1,Ackermann(m,n-1));
+];
+
+[AckermannBench
+result i;
+ @print "Ackermann Benchmark^";
+ @print "Start^";
+ @show_status;
+ result=Ackermann(3,6);
+ @print "End^";
+ @show_status;
+ @print "Result=";
+ @print_num result;
+ @new_line;
+ @print "Start10^";
+ @show_status;
+ result=Ackermann(3,6);
+ result=Ackermann(3,6);
+ result=Ackermann(3,6);
+ result=Ackermann(3,6);
+ result=Ackermann(3,6);
+ result=Ackermann(3,6);
+ result=Ackermann(3,6);
+ result=Ackermann(3,6);
+ result=Ackermann(3,6);
+ @print "End^^";
+ @show_status;
+];
+
+Constant SIZE=8192;
+
+Array flags -> SIZE+1;
+
+[Sieve
+iter count i prime k;
+ @print "Sieve of Eratosthenes Benchmark^";
+ @print "Start10^";
+ @show_status;
+ for (iter = 1: iter <= 10: iter++)
+ {
+ count = 0;
+ for (i = 0: i <= SIZE: i++)
+ i->flags = 1;
+
+ for (i = 0: i <= SIZE: i++)
+ {
+ if (i->flags)
+ {
+ prime = i + i + 3;
+ k = i + prime;
+
+ while (k <= SIZE)
+ {
+ k->flags = 0;
+ k = k + prime;
+ }
+
+ count++;
+ }
+ }
+ }
+ @print "End^";
+ @show_status;
+ @print "Count=";
+ @print_num count;
+ @new_line;
+ @print "Start100^";
+ @show_status;
+ for (iter = 1: iter <= 100: iter++)
+ {
+ count = 0;
+ for (i = 0: i <= SIZE: i++)
+ i->flags = 1;
+
+ for (i = 0: i <= SIZE: i++)
+ {
+ if (i->flags)
+ {
+ prime = i + i + 3;
+ k = i + prime;
+
+ while (k <= SIZE)
+ {
+ k->flags = 0;
+ k = k + prime;
+ }
+
+ count++;
+ }
+ }
+ }
+ @print "End^^";
+ @show_status;
+];
+
+[Mandelbrot
+x y xtemp ytemp x0 y0 x00 y00 i;
+@print "Start Mandelbrot 16-bit^";
+@show_status;
+y0=60;
+do
+{
+ x0=-120;
+ y00=y0*40;
+ do
+ {
+ xtemp=0;
+ ytemp=0;
+ x=0;
+ y=0;
+ i=0;
+ x00=x0*20;
+ do
+ {
+ xtemp=xtemp-ytemp+y00;
+ y=(x*y+x00)/32;
+ if (y>=2*64 || y<=-2*64)
+ {
+ i++;
+ break;
+ }
+ x=xtemp/64;
+ if (x>=2*64 || x<=-2*64)
+ {
+ i++;
+ break;
+ }
+ xtemp=x*x;
+ ytemp=y*y;
+ i++;
+ } until ( xtemp + ytemp >= 64*64*4 || i>=32);
+ i=1-->(i+MandelbrotPalette);
+ x0++;
+ } until (x0>=120);
+ y0--;
+} until (y0==60-230);
+@print "End Mandelbrot 16-bit^^";
+@show_status;
+];
+
+[ Mul32
+r a b
+x1 x2 y1 y2 mul;
+mul=1;
+a=(a-->0)*16+(a->2)/16;
+if (a<0)
+{
+ mul=-mul;
+ a=-a;
+}
+y1=a/256;
+x1=a&$ff;
+b=(b-->0)*16+(b->2)/16;
+if (b<0)
+{
+ mul=-mul;
+ b=-b;
+}
+y2=b/256;
+x2=b&$ff;
+r-->0=0;
+r-->1=x1*x2;
+r++;
+r-->0=r-->0+x1*y2+x2*y1;
+r--;
+r-->0=mul*(r-->0+y1*y2);
+];
+
+[ Add32
+r a b;
+r-->0=0;
+r-->1=(a-->1)&$ff+(b-->1)&$ff;
+r++;
+a++;
+b++;
+r-->0=r-->0+(a-->0)&$ff+(b-->0)&$ff;
+r--;
+a--;
+b--;
+r-->0=r-->0+a-->0+b-->0;
+];
+
+[ Neg32
+r a;
+r-->1=~a-->1+1;
+r-->0=~a-->0;
+if (r-->1==0)
+ r-->0=r-->0+1;
+];
+
+[ Mov32
+r a;
+r-->1=a-->1;
+r-->0=a-->0;
+];
+
+[ Dob32
+r;
+r-->0=2*r-->0;
+if (r-->1<0)
+ r-->0=r-->0+1;
+r-->1=2*r-->1;
+];
+
+[MandelbrotPalette;
+];
+
+[Mandelbrot32
+x y xtemp ytemp x0 y0 x00 y00 i t;
+@print "Start Mandelbrot 32-bit^";
+@show_status;
+y0=60;
+
+xtemp=0;
+ytemp=4;
+y=8;
+x=12;
+t=16;
+x00=20;
+y00=24;
+
+do
+{
+ x0=-120;
+ y00-->0=y0*40/16;
+ y00-->1=0;
+ do
+ {
+ xtemp-->0=0;
+ xtemp-->1=0;
+ ytemp-->0=0;
+ ytemp-->1=0;
+ y-->0=0;
+ y-->1=0;
+ x-->0=0;
+ x-->1=0;
+ x00-->0=x0*40/16;
+ x00-->1=0;
+
+ i=0;
+ do
+ {
+ Neg32(ytemp, ytemp);
+ Add32(t, xtemp, ytemp);
+ Neg32(ytemp, ytemp);
+ Add32(xtemp, t, y00);
+ Mul32(t, x, y);
+ Dob32(t);
+ Add32(y, t, x00);
+ Mov32(x,xtemp);
+ Mul32(xtemp,x,x);
+ Mul32(ytemp,y,y);
+ i++;
+ if (y-->0>=$200 || y-->0<=-$200)
+ break;
+ if (x-->0>=$200 || x-->0<=-$200)
+ break;
+ Add32(t,xtemp,ytemp);
+ } until ( t-->0 >= $400 || i>=32);
+ i=1-->(i+MandelbrotPalette);
+ x0++;
+ } until (x0>=120);
+ y0--;
+} until (y0==60-230);
+@print "End Mandelbrot 32-bit^^";
+@show_status;
+];
+
+Constant Number_Of_Runs = 10000;
+
+Constant Ident_1 = 0;
+Constant Ident_2 = 1;
+Constant Ident_3 = 2;
+Constant Ident_4 = 3;
+Constant Ident_5 = 4;
+
+Constant Ptr_Comp=0;
+Constant Discr=1;
+Constant Enum_Comp=2;
+Constant Int_Comp=3;
+Constant Str_Comp=8;
+Constant E_Comp_2=2;
+Constant Str_2_Comp=6;
+Constant Ch_1_Comp=4;
+Constant Ch_2_Comp=5;
+Constant RecSize=Str_Comp+31;
+
+Array String1-> 'D' 'H' 'R' 'Y' 'S' 'T' 'O' 'N' 'E' ' ' 'P' 'R' 'O' 'G' 'R' 'A' 'M' ',' ' ' '1' ''' 'S' 'T' ' ' 'S' 'T' 'R' 'I' 'N' 'G' 0;
+Array String2-> 'D' 'H' 'R' 'Y' 'S' 'T' 'O' 'N' 'E' ' ' 'P' 'R' 'O' 'G' 'R' 'A' 'M' ',' ' ' '2' ''' 'N' 'D' ' ' 'S' 'T' 'R' 'I' 'N' 'G' 0;
+Array String3-> 'D' 'H' 'R' 'Y' 'S' 'T' 'O' 'N' 'E' ' ' 'P' 'R' 'O' 'G' 'R' 'A' 'M' ',' ' ' '3' ''' 'R' 'D' ' ' 'S' 'T' 'R' 'I' 'N' 'G' 0;
+Array SomeString->'D' 'H' 'R' 'Y' 'S' 'T' 'O' 'N' 'E' ' ' 'P' 'R' 'O' 'G' 'R' 'A' 'M' ',' ' ' 'S' 'O' 'M' 'E' ' ' 'S' 'T' 'R' 'I' 'N' 'G' 0;
+Array Str_1_Loc_Space->31;
+Array Str_2_Loc_Space->31;
+Array Arr_1_Glob-->50;
+Array Arr_2_Glob-->2500;
+Array Ptr_Glob->RecSize;
+Array Next_Ptr_Glob->RecSize;
+Global Int_Glob;
+Global Bool_Glob;
+Global Ch_1_Glob;
+Global Ch_2_Glob;
+
+[strcmp
+a b c1 c2 i;
+while (1)
+{
+ c1=i->a;
+ c2=i->b;
+ if (c1~=c2 || c1*c2==0)
+ return c1-c2;
+ i++;
+}
+];
+
+[strcpy
+a b i;
+while (b->i)
+{
+ a->i=b->i;
+ i++;
+}
+a->i=0;
+];
+
+[memcpy
+a b i;
+while (i)
+{
+ i--;
+ a->i=b->i;
+}
+];
+
+[PrintString
+a i;
+while (a->0)
+{
+ i=a->0;
+ @print_char i;
+ a++;
+}
+];
+
+[Func_1
+Ch_1_Par_Val Ch_2_Par_Val
+Ch_1_Loc Ch_2_Loc;
+
+Ch_1_Loc = Ch_1_Par_Val;
+Ch_2_Loc = Ch_1_Loc;
+if (Ch_2_Loc ~= Ch_2_Par_Val)
+ return (Ident_1);
+else
+{
+ Ch_1_Glob = Ch_1_Loc;
+ return (Ident_2);
+}
+];
+
+[Func_2
+Str_1_Par_Ref Str_2_Par_Ref
+Int_Loc Ch_Loc;
+
+Int_Loc = 2;
+while (Int_Loc <= 2)
+{
+ if (Func_1 (Str_1_Par_Ref->Int_Loc,
+ Str_2_Par_Ref->(Int_Loc+1)) == Ident_1)
+ {
+ Ch_Loc = 'A';
+ Int_Loc ++;
+ }
+}
+if (Ch_Loc >= 'W' && Ch_Loc < 'Z')
+ Int_Loc = 7;
+if (Ch_Loc == 'R')
+ return 1;
+else
+{
+ if (strcmp (Str_1_Par_Ref, Str_2_Par_Ref) > 0)
+ {
+ Int_Loc = Int_Loc + 7;
+ Int_Glob = Int_Loc;
+ return 1;
+ }
+ else
+ return 0;
+}
+];
+
+[Func_3
+Enum_Par_Val
+Enum_Loc;
+
+ Enum_Loc = Enum_Par_Val;
+ if (Enum_Loc == Ident_3)
+ return 1;
+ else
+ return 0;
+];
+
+[Proc_1
+Ptr_Val_Par
+Next_Record;
+ Next_Record = Ptr_Val_Par-->Ptr_Comp;
+ memcpy(Ptr_Val_Par-->Ptr_Comp, Ptr_Glob, RecSize);
+ Ptr_Val_Par-->Int_Comp = 5;
+ Next_Record-->Int_Comp
+ = Ptr_Val_Par-->Int_Comp;
+ Next_Record-->Ptr_Comp = Ptr_Val_Par-->Ptr_Comp;
+ Proc_3 (Next_Record+2*Ptr_Comp);
+ if (Next_Record-->Discr == Ident_1)
+ {
+ Next_Record-->Int_Comp = 6;
+ Next_Record-->Enum_Comp=Proc_6 (Ptr_Val_Par-->Enum_Comp);
+ Next_Record-->Ptr_Comp = Ptr_Glob-->Ptr_Comp;
+ Next_Record-->Int_Comp=Proc_7 (Next_Record-->Int_Comp, 10);
+ }
+ else
+ memcpy(Ptr_Val_Par, Ptr_Val_Par-->Ptr_Comp, RecSize);
+];
+
+[Proc_2
+Int_Par_Ref
+Int_Loc Enum_Loc;
+
+ Int_Loc = Int_Par_Ref + 10;
+ do
+ if (Ch_1_Glob == 'A')
+ {
+ Int_Loc --;
+ Int_Par_Ref = Int_Loc - Int_Glob;
+ Enum_Loc = Ident_1;
+ }
+ until (Enum_Loc == Ident_1);
+return Int_Par_Ref;
+];
+
+[Proc_3
+Ptr_Ref_Par;
+if (Ptr_Glob)
+{
+ Ptr_Ref_Par-->0 = Ptr_Glob-->Ptr_Comp;
+}
+Ptr_Glob-->Int_Comp=Proc_7(10, Int_Glob);
+];
+
+[Proc_4
+Bool_Loc;
+Bool_Loc = Ch_1_Glob == 'A';
+Bool_Glob = Bool_Loc | Bool_Glob;
+Ch_2_Glob = 'B';
+];
+
+[Proc_5;
+Ch_1_Glob = 'A';
+Bool_Glob = 0;
+];
+
+[Proc_6
+Enum_Val_Par
+Enum_Ref_Par;
+
+Enum_Ref_Par = Enum_Val_Par;
+if (~~Func_3 (Enum_Val_Par))
+ Enum_Ref_Par = Ident_4;
+switch (Enum_Val_Par)
+{
+Ident_1:
+ Enum_Ref_Par = Ident_1;
+ break;
+Ident_2:
+ if (Int_Glob > 100) Enum_Ref_Par = Ident_1;
+ else Enum_Ref_Par = Ident_4;
+ break;
+Ident_3:
+ Enum_Ref_Par = Ident_2;
+ break;
+Ident_4:
+ break;
+Ident_5:
+ Enum_Ref_Par = Ident_3;
+ break;
+}
+return Enum_Ref_Par;
+];
+
+[Proc_7
+Int_1_Par_Val Int_2_Par_Val
+Int_Loc;
+
+Int_Loc = Int_1_Par_Val + 2;
+return Int_2_Par_Val + Int_Loc;
+];
+
+[Proc_8
+Arr_1_Par_Ref Int_1_Par_Val Int_2_Par_Val ! Should take Arr_2_Global as argument but limited to 3 args
+Int_Index Int_Loc;
+
+Int_Loc = Int_1_Par_Val + 5;
+Arr_1_Par_Ref-->Int_Loc = Int_2_Par_Val;
+Arr_1_Par_Ref-->(Int_Loc+1) = Arr_1_Par_Ref-->Int_Loc;
+Arr_1_Par_Ref-->(Int_Loc+30) = Int_Loc;
+for (Int_Index = Int_Loc: Int_Index <= Int_Loc+1: Int_Index++)
+Arr_2_Glob-->(50*Int_Loc+Int_Index) = Int_Loc;
+Arr_2_Glob-->(50*Int_Loc+Int_Loc-1) = Arr_2_Glob-->(50*Int_Loc+Int_Loc-1)+1;
+Arr_2_Glob-->(50*(Int_Loc+20)+Int_Loc) = Arr_1_Par_Ref-->Int_Loc;
+Int_Glob = 5;
+];
+
+[Dhrystone
+Run_Index Int_1_Loc Int_2_Loc Int_3_Loc Enum_Loc Ch_Index Str_1_Loc Str_2_Loc temp;
+
+Str_1_Loc=Str_1_Loc_Space;
+Str_2_Loc=Str_2_Loc_Space;
+strcpy(Str_1_Loc, String1);
+
+Ptr_Glob-->Ptr_Comp = Next_Ptr_Glob;
+Ptr_Glob-->Discr = Ident_1;
+Ptr_Glob-->Enum_Comp = Ident_3;
+Ptr_Glob-->Int_Comp = 40;
+strcpy(Ptr_Glob+Str_Comp, SomeString);
+Arr_2_Glob-->(8*50+7) = 10;
+
+@print "Start Dhrystone x10000^";
+@show_status;
+
+for (Run_Index = 1: Run_Index <= Number_Of_Runs: Run_Index++)
+ {
+ Proc_5();
+ Proc_4();
+ Int_1_Loc = 2;
+ Int_2_Loc = 3;
+ strcpy (Str_2_Loc, String2);
+ Enum_Loc = Ident_2;
+ Bool_Glob = ~~Func_2 (Str_1_Loc, Str_2_Loc);
+ while (Int_1_Loc < Int_2_Loc)
+ {
+ Int_3_Loc = 5 * Int_1_Loc - Int_2_Loc;
+ Int_3_Loc = Proc_7 (Int_1_Loc, Int_2_Loc);
+ Int_1_Loc ++;
+ }
+ Proc_8 (Arr_1_Glob, Int_1_Loc, Int_3_Loc);
+ Proc_1 (Ptr_Glob);
+ for (Ch_Index = 'A': Ch_Index <= Ch_2_Glob: Ch_Index++)
+ {
+ if (Enum_Loc == Func_1 (Ch_Index, 'C'))
+ {
+ Enum_Loc=Proc_6 (Ident_1);
+ strcpy (Str_2_Loc, String3);
+ Int_2_Loc = Run_Index;
+ Int_Glob = Run_Index;
+ }
+ }
+ Int_2_Loc = Int_2_Loc * Int_1_Loc;
+ Int_1_Loc = Int_2_Loc / Int_3_Loc;
+ Int_2_Loc = 7 * (Int_2_Loc - Int_3_Loc) - Int_1_Loc;
+ Int_1_Loc = Proc_2 (Int_1_Loc);
+ }
+@print "End Dhrystone^^";
+@show_status;
+
+@print "Int_Glob: ";
+@print_num Int_Glob;
+@print " should be 5^";
+@print "Bool_Glob: ";
+@print_num Bool_Glob;
+@print " should be 1^";
+@print "Ch_1_Glob: '";
+@print_char Ch_1_Glob;
+@print "' should be 'A'^";
+@print "Ch_2_Glob: '";
+@print_char Ch_2_Glob;
+@print "' should be 'B'^";
+@print "Arr_1_Glob[8]: ";
+temp=Arr_1_Glob-->8;
+@print_num temp;
+@print " should be 7^";
+@print "Arr_2_Glob[8][7]: ";
+temp=Arr_2_Glob-->(8*50+7);
+@print_num temp;
+@print " should be Number_Of_Runs+10^";
+
+@print "Ptr_Glob->^";
+@print " Ptr_Comp: ";
+temp=Ptr_Glob-->Ptr_Comp;
+@print_num temp;
+@new_line;
+@print " Discr: ";
+temp=Ptr_Glob-->Discr;
+@print_num temp;
+@print " should be 0^";
+@print " Enum_Comp: ";
+temp=Ptr_Glob-->Enum_Comp;
+@print_num temp;
+@print " should be 2^";
+@print " Int_Comp: ";
+temp=Ptr_Glob-->Int_Comp;
+@print_num temp;
+@print " should be 17^";
+@print " Str_Comp: ";
+temp=Ptr_Glob+Str_Comp;
+PrintString(temp);
+@print " should be DHRYSTONE PROGRAM, SOME STRING^";
+
+@print "Next_Ptr_Glob->^";
+@print " Ptr_Comp: ";
+temp=Next_Ptr_Glob-->Ptr_Comp;
+@print_num temp;
+@print " should be same as above^";
+@print " Discr: ";
+temp=Next_Ptr_Glob-->Discr;
+@print_num temp;
+@print " should be 0^";
+@print " Enum_Comp: ";
+temp=Next_Ptr_Glob-->Enum_Comp;
+@print_num temp;
+@print " should be 1^";
+@print " Int_Comp: ";
+temp=Next_Ptr_Glob-->Int_Comp;
+@print_num temp;
+@print " should be 18^";
+@print " Str_Comp: ";
+temp=Next_Ptr_Glob+Str_Comp;
+PrintString(temp);
+@print " should be DHRYSTONE PROGRAM, SOME STRING^";
+
+@print "Int_1_Loc: ";
+@print_num Int_1_Loc;
+@print " should be 5^";
+@print "Int_2_Loc: ";
+@print_num Int_2_Loc;
+@print " should be 13^";
+@print "Int_3_Loc: ";
+@print_num Int_3_Loc;
+@print " should be 7^";
+@print "Enum_Loc: ";
+@print_num Enum_Loc;
+@print " should be 1^";
+@print "Str_1_Loc: ";
+PrintString(Str_1_Loc);
+@print " should be DHRYSTONE PROGRAM, 1'ST STRING^";
+@print "Str_2_Loc: ";
+PrintString(Str_2_Loc);
+@print " should be DHRYSTONE PROGRAM, 2'ND STRING^^";
+@show_status;
+];
+
+[Main
+t;
+ AckermannBench();
+ Sieve();
+ Dhrystone();
+ Mandelbrot();
+ Mandelbrot32();
+];
trunk/benchmark/benchmark.inf
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/benchmark/benchmark.txt
===================================================================
--- trunk/benchmark/benchmark.txt (nonexistent)
+++ trunk/benchmark/benchmark.txt (revision 2)
@@ -0,0 +1,46 @@
+1.83 -> 11.57 = 9.74s x10 Ackermann => 0.97s for Ackermann
+12.64 -> 22.88 = 10.24s x100 Sieve => 1.02s for x10 Sieve
+6.33 -> 12.44 = 6.11s x10000 Dhrystone => 1636 Dhrystones per second
+ or 0.93 DMIPS or 0.093 DMIP/MHz
+
+Core i7 2.7GHz Early 2011 13-inch MacBook Pro
+zops 14.25s for x500000 => 35087 => 19.97 DMIPS
+Zoom 6.75s for x500000 => 74074 => 42.15 DMIPS
+Frotz 6.15s for x500000 => 81300 => 46.27 DMIPS or 0.017 DMIP/MHz
+
+
+10x Sieve (Lower is better)
+
+Acorn Archimedes 8Mz ARM2 + Acorn C 0.5
+IBM AT (6Mhz) 286 C Compiler 0.7
+** 10MHz Z3 Inform 6 ** 1.0
+VAX 11/780 1.4
+10Mhz 8086 + Lattice 3.6
+IBM PC (5Mhz) Assembly 4.0
+8Mhz 68000 DR C Compiler 6.0
+Apple II Assembly 13.9
+
+
+Ackermann(3,6) (Lower is better)
+
+** 10MHz Z3 Inform 6 ** 1.0
+10MHz ERC (Sparc V7) C 1.1
+RISC I 7.6MHz 3.2
+VAX 11/780 5MHz 5.1
+Z8002 6MHz 9.0
+
+
+DMIPS (Higher is better)
+
+Commodore 64 1MHz 0.02
+Apple IIe 1MHz 0.02
+Apple Macintosh 8MHz 0.4
+Atari ST 8MHz 0.6
+** 10MHz Z3 Inform 6 ** 0.9
+IBM PC/AT 286 8MHz 1.3
+Compaq 386 16MHz 1.7
+ARM2 8MHz 2.6
+Acorn RiscPC ARM 610 30 MHz 18.4
+Frotz (Early 2011 13-MacBook i7 2.7GHz) 46.2
+Early 2011 MacBook Pro i7 2.7GHz O0 5405. or 2.00 DMIPs/MHz
+Early 2011 MacBook Pro i7 2.7GHz O3 14121. or 5.23 DMIPs/MHz
trunk/benchmark/benchmark.txt
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/bios/zfont2.xcf
===================================================================
Cannot display: file marked as a binary type.
svn:mime-type = application/octet-stream
Index: trunk/bios/zfont2.xcf
===================================================================
--- trunk/bios/zfont2.xcf (nonexistent)
+++ trunk/bios/zfont2.xcf (revision 2)
trunk/bios/zfont2.xcf
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Added: svn:mime-type
## -0,0 +1 ##
+application/octet-stream
\ No newline at end of property
Index: trunk/bios/icons.h
===================================================================
--- trunk/bios/icons.h (nonexistent)
+++ trunk/bios/icons.h (revision 2)
@@ -0,0 +1,3633 @@
+/* GIMP header image file format (INDEXED): /Users/charlie/code/verilog/Z2/icons.h */
+
+static unsigned int width = 100;
+static unsigned int height = 480;
+
+/* Call this macro repeatedly. After each use, the pixel data can be extracted */
+
+#define HEADER_PIXEL(data,pixel) {\
+pixel[0] = header_data_cmap[(unsigned char)data[0]][0]; \
+pixel[1] = header_data_cmap[(unsigned char)data[0]][1]; \
+pixel[2] = header_data_cmap[(unsigned char)data[0]][2]; \
+data ++; }
+
+static char header_data_cmap[256][3] = {
+ { 0, 3, 0},
+ { 7, 10, 16},
+ { 9, 21, 39},
+ { 15, 19, 61},
+ { 28, 26, 29},
+ { 38, 31, 98},
+ { 43, 34, 88},
+ { 37, 43, 45},
+ { 42, 42, 51},
+ { 40, 40, 89},
+ { 81, 28, 99},
+ { 62, 43, 27},
+ { 78, 40, 44},
+ { 57, 47, 48},
+ { 41, 50, 69},
+ { 57, 43, 95},
+ { 12, 57, 98},
+ { 53, 50, 59},
+ { 50, 49, 96},
+ { 47, 55, 54},
+ { 69, 45, 92},
+ { 58, 50, 84},
+ { 57, 55, 47},
+ { 59, 59,104},
+ {138, 38,101},
+ { 74, 58,105},
+ { 69, 66, 56},
+ { 75, 63, 72},
+ { 88, 56,106},
+ { 66, 68, 71},
+ { 52, 76, 73},
+ {121, 58, 56},
+ { 61, 77, 63},
+ { 74, 70, 95},
+ { 93, 69, 65},
+ { 59, 78,101},
+ { 92, 68,104},
+ {113, 68, 52},
+ {124, 65, 90},
+ { 95, 77, 57},
+ { 83, 81, 61},
+ { 85, 82, 72},
+ { 81, 82, 85},
+ { 92, 79, 89},
+ { 70, 90, 63},
+ {174, 59, 72},
+ {159, 65, 64},
+ {207, 57, 55},
+ {114, 78,123},
+ {194, 67, 61},
+ {203, 65, 61},
+ {149, 82, 67},
+ { 84,103, 75},
+ { 97, 98,100},
+ { 90,105, 55},
+ { 82,103,103},
+ { 83,101,123},
+ {171, 84, 54},
+ {140, 92, 70},
+ {123,101, 74},
+ {109,105, 80},
+ {211, 77, 68},
+ {132,103, 50},
+ {120,102,105},
+ {119,112, 44},
+ { 99,115, 98},
+ { 98,117, 87},
+ { 89,118,117},
+ {112,113,116},
+ {113,115,106},
+ {195,105, 91},
+ {124,126,128},
+ {111,132,107},
+ {162,116,125},
+ {117,130,120},
+ {199,111, 71},
+ {140,127,107},
+ {134,130,117},
+ {123,136, 91},
+ {170,123, 94},
+ {147,132, 95},
+ {153,133, 69},
+ {184,124, 79},
+ {100,142,155},
+ {127,136,148},
+ {168,132, 77},
+ {212,125, 48},
+ {124,147,135},
+ {144,145,146},
+ {153,145,125},
+ {147,153, 91},
+ {209,141, 47},
+ {178,151, 46},
+ {209,147, 95},
+ {179,150,176},
+ {186,159, 91},
+ {161,161,162},
+ {145,170,151},
+ {200,160, 93},
+ {199,158,129},
+ {175,167,145},
+ {109,181,201},
+ {234,162, 30},
+ {160,169,181},
+ {145,181,150},
+ {148,184,128},
+ {184,173,134},
+ {225,178, 30},
+ {177,178,179},
+ {184,178,164},
+ {150,188,172},
+ {222,185,104},
+ {130,206,222},
+ {233,191, 98},
+ {193,195,196},
+ {218,193,203},
+ {179,208,178},
+ {253,204, 7},
+ {231,193,187},
+ {215,203,160},
+ {207,203,186},
+ {227,202,140},
+ {210,213,214},
+ {227,229,229},
+ {235,230,214},
+ {245,237,194},
+ {251,246,226},
+ {253,255,252},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255}
+ };
+static char header_data[] = {
+ 49,49,49,49,49,50,49,49,49,50,49,49,49,49,49,49,
+ 49,49,49,50,49,50,50,50,50,50,50,50,50,49,49,50,
+ 50,50,50,50,50,50,50,50,49,50,50,50,50,49,50,50,
+ 50,50,50,50,50,49,49,50,50,50,50,50,50,50,50,50,
+ 50,50,50,50,50,50,50,50,50,50,50,50,50,50,49,50,
+ 49,49,61,61,61,61,50,61,61,61,50,50,49,49,50,50,
+ 50,50,50,61,
+ 49,49,49,49,49,50,49,49,49,49,49,50,50,50,50,50,
+ 50,50,50,50,50,50,50,50,50,50,50,50,50,50,50,50,
+ 50,50,50,50,50,50,50,50,50,50,50,50,50,50,50,50,
+ 50,50,50,50,50,50,50,50,50,50,50,50,50,50,50,50,
+ 50,50,50,50,50,50,50,50,50,50,50,50,50,50,50,50,
+ 50,50,50,50,50,50,61,61,61,50,61,50,49,49,50,50,
+ 50,50,61,61,
+ 49,49,49,49,49,49,49,49,49,49,49,49,49,49,49,49,
+ 49,49,49,49,49,49,49,49,49,49,49,49,49,49,49,49,
+ 49,49,49,50,49,49,50,49,49,50,49,49,49,50,61,49,
+ 50,50,50,50,50,61,50,50,50,50,50,50,50,50,50,50,
+ 50,50,50,50,50,50,50,50,50,50,50,50,50,50,50,50,
+ 50,61,50,50,50,50,61,61,61,50,61,61,49,50,50,50,
+ 61,50,61,61,
+ 49,49,49,49,49,49,49,49,49,49,46,51,31,31,31,31,
+ 31,31,31,31,31,31,31,31,31,31,31,31,31,31,31,31,
+ 31,31,31,31,31,31,31,31,31,31,31,31,31,31,31,31,
+ 31,31,31,31,31,31,31,31,31,31,46,46,46,46,46,46,
+ 46,46,46,46,46,46,46,46,46,46,46,46,46,46,46,46,
+ 46,46,46,46,46,46,46,46,46,46,61,50,49,50,50,50,
+ 50,50,61,61,
+ 49,49,49,49,49,49,49,49,49,50,46,27,26,22,26,22,
+ 26,13,13,22,13,19,22,13,26,13,8,26,13,34,13,26,
+ 26,26,13,13,39,13,22,13,8,13,34,22,22,13,26,8,
+ 34,13,8,26,13,22,13,8,22,13,34,63,63,43,43,42,
+ 42,43,27,43,42,43,34,43,43,43,43,42,42,43,43,43,
+ 43,63,43,53,43,43,43,43,27,27,49,50,49,49,50,50,
+ 50,50,61,61,
+ 49,49,49,49,49,49,49,50,49,50,46,8,95,60,95,80,
+ 95,80,59,81,7,60,81,40,95,40,22,95,62,81,59,95,
+ 80,95,81,80,81,59,59,27,7,26,95,60,81,81,95,40,
+ 81,59,26,95,26,95,13,4,81,39,95,76,95,80,80,81,
+ 80,80,60,60,95,60,80,81,80,95,80,80,95,42,80,80,
+ 80,81,60,81,60,80,60,81,41,14,49,50,50,50,50,50,
+ 50,61,50,61,
+ 49,49,49,49,49,49,49,49,49,50,46,4,111,59,111,59,
+ 113,81,85,95,26,95,111,81,111,62,40,111,98,111,85,95,
+ 59,111,98,111,81,95,85,59,26,40,111,81,98,95,111,81,
+ 62,81,59,113,62,113,13,7,113,85,111,80,111,85,98,98,
+ 85,98,98,85,113,111,98,111,80,113,98,59,111,33,95,85,
+ 113,85,95,111,95,111,41,113,41,35,49,50,49,50,50,50,
+ 50,50,50,61,
+ 49,49,49,49,49,49,49,49,49,50,46,8,81,80,95,26,
+ 98,59,85,81,59,98,85,81,85,59,26,85,59,95,98,81,
+ 39,95,85,95,81,85,85,59,26,26,98,81,62,59,95,80,
+ 85,81,81,95,85,111,22,40,98,98,85,85,95,98,95,98,
+ 98,98,95,95,98,98,98,111,59,98,111,63,98,33,95,59,
+ 111,95,95,98,80,111,69,111,53,35,50,50,49,49,50,50,
+ 50,50,50,61,
+ 49,49,49,49,49,49,49,49,49,49,46,7,40,26,39,19,
+ 39,26,85,62,39,39,39,39,26,39,7,26,13,39,39,39,
+ 22,39,39,22,59,13,59,39,13,13,59,40,13,22,39,13,
+ 59,39,39,26,59,59,39,34,79,79,85,76,59,63,41,85,
+ 59,59,41,85,59,76,59,59,63,69,85,43,76,53,76,43,
+ 69,80,69,76,76,76,76,80,68,35,50,50,50,61,61,50,
+ 50,50,61,61,
+ 49,49,49,49,49,49,50,49,49,49,46,7,7,8,17,8,
+ 8,7,98,95,39,26,59,59,7,59,39,26,39,39,26,39,
+ 22,39,39,26,39,26,26,39,39,13,59,22,39,26,39,26,
+ 39,39,39,26,81,13,95,58,79,59,85,80,59,41,41,80,
+ 42,41,42,85,42,85,60,63,69,53,85,53,63,85,63,69,
+ 43,59,53,33,43,33,53,43,56,35,50,50,50,61,50,50,
+ 50,50,61,61,
+ 49,49,49,49,49,49,49,49,49,50,31,8,7,7,8,13,
+ 8,8,113,111,95,85,95,80,59,98,95,95,98,98,81,98,
+ 59,59,95,95,98,95,81,98,85,39,111,62,111,59,95,98,
+ 95,98,95,59,113,13,113,98,111,98,95,111,98,95,95,98,
+ 95,95,98,98,41,111,95,95,98,95,111,98,98,98,95,98,
+ 95,95,80,33,33,33,33,33,33,35,50,50,49,50,50,50,
+ 50,61,61,61,
+ 49,49,49,49,49,49,49,49,49,50,31,8,13,7,7,13,
+ 13,7,95,81,81,98,62,59,59,98,59,98,62,85,81,85,
+ 59,40,111,81,98,81,98,81,59,39,98,59,98,59,111,95,
+ 98,85,95,22,98,4,98,98,98,98,95,85,95,95,95,95,
+ 98,98,98,95,63,95,85,80,98,98,95,98,98,95,98,95,
+ 95,111,95,33,43,33,43,43,43,43,50,50,50,50,50,50,
+ 50,61,61,61,
+ 49,49,49,50,49,49,49,49,49,50,31,7,8,8,7,7,
+ 13,7,22,22,19,26,13,13,7,39,8,22,7,22,13,13,
+ 13,40,62,13,26,26,26,26,22,39,59,8,39,26,95,22,
+ 22,22,39,8,39,7,79,79,59,69,41,41,41,42,41,41,
+ 59,41,41,41,43,42,43,27,63,42,53,63,63,63,63,63,
+ 76,95,80,33,33,43,43,43,43,56,50,50,49,50,50,50,
+ 50,50,61,61,
+ 49,49,49,49,49,49,49,49,49,49,31,7,8,13,8,8,
+ 8,13,2,8,8,8,8,8,4,27,8,2,8,8,8,8,
+ 8,59,59,39,59,62,39,62,59,59,98,8,62,39,81,59,
+ 39,59,59,13,59,59,82,85,79,76,27,80,59,59,59,59,
+ 59,59,14,27,43,33,33,33,33,33,33,33,33,43,43,33,
+ 43,76,43,33,43,43,43,43,43,56,50,50,49,49,50,50,
+ 50,61,61,61,
+ 49,49,49,49,49,49,49,49,49,49,31,7,8,8,13,8,
+ 8,8,8,7,8,8,8,13,8,8,34,8,7,8,8,8,
+ 13,62,95,111,95,95,98,95,62,13,111,19,111,95,81,85,
+ 111,95,98,39,85,98,111,111,111,98,34,111,111,98,95,98,
+ 98,111,41,27,27,33,33,27,33,33,33,33,43,43,33,33,
+ 43,33,33,33,43,43,43,56,56,56,50,50,49,50,50,50,
+ 50,50,61,61,
+ 49,49,49,49,49,49,50,50,49,50,31,8,7,7,13,8,
+ 8,8,13,7,8,8,8,13,13,7,27,8,8,8,8,8,
+ 13,26,80,81,40,85,62,59,39,13,62,7,85,59,62,39,
+ 81,39,85,34,59,93,85,85,85,85,27,85,85,80,85,80,
+ 80,85,80,14,27,27,33,33,33,33,33,43,43,33,33,43,
+ 33,33,43,43,33,43,43,33,56,56,50,50,49,50,61,50,
+ 50,61,61,61,
+ 49,49,49,50,49,49,49,61,49,61,51,19,8,8,8,26,
+ 22,8,8,13,8,8,7,8,13,8,26,34,8,8,8,13,
+ 13,8,13,13,13,34,13,8,13,13,13,13,26,13,26,13,
+ 22,13,26,34,40,79,58,59,76,59,27,42,42,42,63,27,
+ 42,43,41,27,27,33,27,43,27,33,43,43,33,33,33,43,
+ 43,43,43,43,33,33,56,43,53,56,61,50,49,50,50,50,
+ 50,50,61,61,
+ 49,49,49,49,49,50,75,98,75,98,98,81,39,8,7,98,
+ 98,8,7,13,8,8,8,8,13,8,59,98,59,8,8,13,
+ 13,13,8,19,85,85,13,8,8,17,8,62,85,26,81,85,
+ 81,85,85,39,95,98,98,85,95,85,95,85,41,85,85,39,
+ 81,85,85,95,41,33,59,85,80,33,43,33,33,33,33,33,
+ 85,85,43,43,33,33,33,76,80,69,61,61,49,61,50,50,
+ 50,50,61,61,
+ 49,49,49,49,49,50,93,113,75,111,111,113,111,39,7,113,
+ 113,13,8,8,8,8,13,8,8,13,40,113,111,22,7,13,
+ 13,13,8,40,113,113,85,8,13,13,8,111,113,39,111,98,
+ 98,98,111,39,98,98,111,113,113,111,111,111,59,113,113,59,
+ 111,111,111,111,59,33,41,113,113,42,33,33,33,33,33,27,
+ 113,113,43,33,33,33,33,95,113,76,61,50,49,61,50,50,
+ 50,50,50,61,
+ 49,49,49,49,49,50,93,111,75,75,82,95,113,98,7,113,
+ 113,13,8,7,8,13,8,13,7,13,8,81,113,85,8,8,
+ 13,13,8,26,113,113,113,39,8,13,17,111,113,22,22,26,
+ 22,26,26,13,34,58,59,113,113,59,34,69,29,113,113,34,
+ 63,59,41,41,27,27,33,85,113,85,33,33,33,33,43,43,
+ 113,113,43,33,33,33,33,95,113,76,50,50,49,61,50,50,
+ 50,50,50,61,
+ 49,49,49,49,49,50,93,111,75,50,31,22,111,113,40,113,
+ 113,8,13,8,8,13,7,13,8,13,13,40,111,113,26,8,
+ 13,13,13,39,113,98,111,111,34,8,8,98,113,13,8,12,
+ 8,8,8,8,2,34,34,113,113,59,27,35,27,113,113,27,
+ 33,21,21,21,33,27,33,41,111,113,69,33,33,43,43,43,
+ 113,113,43,33,33,33,33,95,113,76,50,50,49,61,50,50,
+ 50,50,50,61,
+ 49,49,49,49,49,50,93,111,75,50,31,2,111,113,59,113,
+ 113,8,13,13,8,8,13,8,8,8,39,95,62,113,85,8,
+ 13,13,13,40,113,95,59,113,98,13,13,111,113,46,46,46,
+ 46,46,31,34,13,34,59,113,113,59,43,27,27,113,113,34,
+ 43,27,27,42,27,27,42,95,80,113,98,33,43,43,43,43,
+ 113,113,43,33,33,56,33,95,113,76,50,50,49,61,50,50,
+ 50,50,50,61,
+ 49,49,49,49,49,50,93,111,75,50,31,26,111,113,40,113,
+ 113,8,8,13,8,8,8,17,8,8,95,113,59,98,113,39,
+ 8,13,13,39,113,95,8,81,113,85,46,111,113,75,93,93,
+ 93,86,93,70,51,34,59,111,113,59,43,34,27,113,113,59,
+ 85,85,85,85,63,33,85,113,59,98,113,76,33,43,33,33,
+ 113,113,33,33,33,35,33,95,113,76,50,50,49,61,50,50,
+ 50,50,61,61,
+ 49,49,49,49,49,50,93,111,75,75,82,95,113,98,7,113,
+ 113,8,7,8,13,8,8,13,8,59,113,111,98,59,113,98,
+ 8,13,13,39,113,95,8,13,98,113,75,111,113,57,111,111,
+ 98,111,113,75,61,46,34,113,113,59,34,34,42,113,113,59,
+ 111,111,111,111,59,59,113,113,98,76,113,98,43,33,33,27,
+ 113,113,33,33,33,33,33,95,113,76,50,50,49,61,50,61,
+ 50,61,50,61,
+ 49,49,49,49,49,50,93,111,75,111,111,113,98,39,7,113,
+ 113,8,13,8,13,13,8,8,13,111,98,80,113,59,95,113,
+ 59,8,13,39,113,95,8,12,70,113,111,111,113,51,51,51,
+ 57,70,70,49,61,61,31,113,113,59,34,34,42,113,113,42,
+ 60,59,59,59,43,98,113,80,113,80,98,113,80,33,33,43,
+ 113,113,35,33,33,35,33,95,113,76,50,50,49,61,50,50,
+ 50,61,50,61,
+ 49,49,49,49,49,50,93,111,75,98,98,85,26,17,19,113,
+ 113,8,34,8,7,13,13,8,81,113,59,7,111,111,59,113,
+ 111,13,13,39,113,85,12,51,46,75,113,111,113,31,31,31,
+ 46,46,46,46,49,61,70,111,113,58,34,34,34,113,113,29,
+ 14,27,27,27,59,113,80,27,111,113,59,113,111,43,43,27,
+ 113,113,43,35,35,35,33,95,113,76,50,50,49,61,50,61,
+ 61,61,50,61,
+ 49,49,49,49,49,50,93,111,75,49,31,7,8,17,26,113,
+ 113,8,27,27,8,8,8,26,113,95,8,2,60,113,62,81,
+ 113,80,8,39,113,98,31,70,46,49,93,113,113,31,31,31,
+ 31,31,31,46,45,61,61,113,113,58,34,34,34,113,113,27,
+ 27,27,43,27,111,111,27,21,59,113,80,85,113,80,33,33,
+ 113,113,33,33,33,33,33,98,113,76,50,50,50,50,61,61,
+ 61,61,61,61,
+ 49,49,49,49,49,50,98,111,75,50,31,2,7,8,22,113,
+ 113,39,39,59,39,26,8,95,113,80,39,39,39,111,111,59,
+ 111,113,34,39,113,98,51,46,46,50,49,98,113,31,51,58,
+ 51,51,51,51,46,50,61,113,113,39,37,58,58,113,113,27,
+ 27,27,27,80,113,85,41,41,41,111,113,59,113,113,42,33,
+ 113,113,43,42,42,42,43,95,113,80,61,61,61,61,50,50,
+ 50,50,61,61,
+ 49,49,49,49,49,50,93,111,75,50,31,7,7,7,19,113,
+ 111,98,98,111,98,85,40,113,111,98,98,111,98,111,113,85,
+ 81,113,85,39,113,98,70,46,46,49,49,51,111,58,111,111,
+ 111,111,111,51,31,49,49,113,113,63,37,58,79,113,113,41,
+ 42,34,41,113,113,111,111,111,98,113,113,95,80,113,95,33,
+ 113,113,111,111,111,111,85,95,113,111,111,111,98,111,50,50,
+ 50,50,50,61,
+ 49,49,49,49,49,50,75,75,49,49,31,7,8,7,8,80,
+ 59,85,85,80,81,59,59,80,62,85,85,85,85,80,80,85,
+ 26,85,81,34,85,82,70,46,46,49,49,31,58,51,85,82,
+ 85,93,98,51,31,46,50,93,93,51,34,79,79,98,98,63,
+ 34,42,60,95,85,95,95,98,98,85,85,95,41,95,95,43,
+ 98,95,95,95,95,95,80,80,98,98,98,98,98,111,50,50,
+ 50,50,50,61,
+ 49,49,49,49,49,49,49,49,49,50,31,7,8,8,8,8,
+ 8,13,13,13,8,17,34,8,8,13,8,17,8,17,8,13,
+ 8,13,19,27,31,51,46,46,45,49,49,31,34,37,34,34,
+ 34,34,34,34,34,31,46,46,31,51,34,79,79,79,79,58,
+ 63,53,41,42,42,42,42,43,27,27,29,27,14,27,43,27,
+ 43,29,29,42,42,42,42,42,42,42,61,61,61,61,50,50,
+ 50,50,50,61,
+ 49,49,49,49,49,49,50,50,49,50,31,7,7,8,8,8,
+ 8,8,8,17,8,8,8,17,8,8,8,8,17,8,8,8,
+ 8,8,14,8,31,31,46,46,51,51,79,41,63,53,53,68,
+ 68,68,68,68,53,71,77,63,43,63,41,79,79,79,70,79,
+ 79,63,43,43,29,29,27,33,14,14,14,14,14,14,14,33,
+ 21,14,14,14,14,33,14,14,14,33,50,50,50,50,50,50,
+ 50,50,50,61,
+ 49,49,49,49,49,49,49,49,49,49,31,7,8,8,17,8,
+ 8,8,8,17,27,17,8,13,13,8,17,17,42,13,17,8,
+ 13,17,8,8,31,31,63,76,89,89,87,42,29,42,53,68,
+ 67,84,88,84,55,71,74,67,53,42,42,79,79,79,79,99,
+ 79,79,73,63,53,42,42,42,42,35,17,27,27,27,27,33,
+ 14,27,29,27,17,35,29,29,29,27,61,50,49,50,50,50,
+ 50,50,61,61,
+ 49,49,49,49,49,49,49,49,49,49,31,7,8,7,8,8,
+ 8,8,8,8,17,27,17,8,13,13,8,13,27,34,8,17,
+ 27,8,29,41,63,68,55,67,87,87,97,42,17,35,42,56,
+ 56,84,88,84,56,84,71,56,53,43,68,58,82,79,93,79,
+ 79,79,79,79,58,63,42,42,42,27,27,27,17,17,27,27,
+ 27,27,17,17,17,29,29,17,29,43,50,50,49,50,50,50,
+ 50,61,50,61,
+ 49,49,49,49,49,49,49,49,49,49,31,8,8,8,8,8,
+ 8,8,8,8,17,17,27,17,8,27,17,8,17,27,27,17,
+ 17,17,80,60,74,55,55,55,67,77,100,53,29,35,56,56,
+ 67,84,96,88,56,88,84,56,43,43,68,34,79,93,85,79,
+ 79,79,79,79,79,63,43,43,27,27,27,29,17,27,27,27,
+ 27,17,17,17,17,17,29,29,42,42,50,50,49,61,50,50,
+ 50,61,50,49,
+ 49,49,49,49,49,49,49,49,49,49,31,13,8,8,8,8,
+ 8,8,17,17,27,17,17,27,17,8,27,17,17,17,27,17,
+ 8,76,78,41,88,55,55,67,67,87,100,77,29,42,56,56,
+ 67,84,96,88,56,88,84,56,43,43,27,13,13,79,79,79,
+ 79,79,79,79,79,58,63,34,43,43,43,27,27,27,27,27,
+ 29,29,29,29,29,43,43,63,63,13,61,50,49,61,50,50,
+ 50,61,61,49,
+ 49,49,49,49,49,49,49,49,49,49,31,27,13,13,8,8,
+ 8,8,8,17,27,27,17,17,27,17,8,13,8,17,13,27,
+ 8,106,44,44,69,74,42,67,67,88,100,100,29,42,56,56,
+ 67,84,96,88,56,88,84,56,43,42,26,19,7,13,58,79,
+ 79,79,79,59,58,58,58,63,63,63,63,43,43,43,43,43,
+ 43,43,43,53,63,63,63,42,13,8,61,50,49,50,50,50,
+ 50,50,61,49,
+ 49,49,49,49,49,49,49,49,49,49,31,13,27,13,13,17,
+ 8,8,8,8,8,17,13,8,17,27,13,17,13,8,8,13,
+ 8,106,52,78,29,89,53,67,67,89,100,119,42,42,56,56,
+ 67,84,96,96,56,96,84,56,43,42,66,66,54,26,13,34,
+ 79,79,82,79,79,79,58,58,58,63,63,59,63,63,63,63,
+ 63,63,63,63,63,34,27,8,8,13,61,50,49,50,50,50,
+ 61,50,61,49,
+ 49,49,49,49,49,49,49,49,49,49,12,8,13,13,13,27,
+ 17,17,8,8,8,17,17,17,8,17,13,17,17,8,8,17,
+ 19,80,66,78,7,88,68,55,67,87,100,119,69,42,56,56,
+ 67,84,96,96,56,96,84,56,43,30,52,78,90,90,40,8,
+ 22,59,79,79,82,79,58,58,79,58,58,79,79,79,79,79,
+ 79,59,59,34,27,8,7,8,8,13,61,50,49,50,50,50,
+ 61,61,61,61,
+ 49,49,49,49,49,49,49,49,49,49,12,7,8,8,13,13,
+ 27,13,17,17,8,8,8,17,17,8,17,13,17,17,8,8,
+ 26,78,40,40,19,69,74,55,67,74,100,106,77,42,56,53,
+ 67,84,96,96,83,96,84,56,56,32,32,52,52,90,90,7,
+ 8,8,13,34,58,58,79,79,79,79,79,79,79,79,79,79,
+ 58,34,13,13,13,8,8,8,7,13,61,50,49,50,50,50,
+ 50,61,61,61,
+ 49,49,49,49,49,49,49,49,49,49,12,8,8,17,8,17,
+ 13,13,27,17,17,8,8,8,8,8,8,17,13,29,41,41,
+ 40,60,39,41,26,44,88,53,67,74,100,100,77,42,53,53,
+ 68,84,88,88,67,88,83,68,53,32,44,78,44,66,105,29,
+ 8,8,8,8,13,13,26,39,59,59,58,58,59,34,34,13,
+ 13,17,8,17,42,8,8,8,8,13,61,49,49,50,50,50,
+ 50,61,61,61,
+ 49,49,49,49,49,49,49,49,49,49,12,8,17,17,8,8,
+ 8,17,17,27,27,17,17,8,8,8,13,26,22,52,78,52,
+ 52,40,40,44,26,40,77,71,69,74,89,100,100,42,53,53,
+ 68,71,88,88,84,88,56,53,29,32,52,90,66,44,72,19,
+ 8,13,13,8,8,8,8,13,13,13,8,8,8,8,8,8,
+ 8,17,29,17,17,7,8,8,8,13,61,50,49,50,50,50,
+ 50,61,61,49,
+ 49,49,49,49,49,49,49,49,49,49,31,17,17,17,17,17,
+ 17,17,8,17,17,27,27,17,8,17,39,32,32,66,78,52,
+ 52,52,52,32,59,39,41,74,67,74,89,100,106,42,53,68,
+ 67,71,88,88,71,71,56,42,7,19,44,78,78,52,87,8,
+ 8,8,8,17,17,13,13,8,8,8,8,8,8,8,8,8,
+ 8,8,8,7,7,8,8,8,7,13,61,61,49,50,50,50,
+ 50,61,61,49,
+ 49,49,49,49,49,49,49,49,49,49,12,8,8,17,17,17,
+ 17,17,17,17,17,17,17,17,17,60,41,52,32,78,66,52,
+ 44,52,44,40,98,34,32,41,53,74,77,89,100,68,68,68,
+ 68,71,71,71,68,53,55,42,30,32,55,32,66,52,65,8,
+ 17,8,17,13,13,17,17,8,8,8,8,8,8,8,8,8,
+ 8,7,7,7,8,8,8,7,17,13,61,61,49,61,50,61,
+ 50,61,61,49,
+ 49,49,49,49,49,49,49,49,49,49,12,8,8,17,17,17,
+ 17,17,17,17,17,17,8,29,41,60,52,66,44,78,66,66,
+ 44,52,19,81,98,37,44,44,42,76,69,68,77,89,77,77,
+ 89,88,88,88,68,56,67,42,32,65,55,19,32,65,41,17,
+ 13,13,13,17,17,8,8,17,8,8,8,8,8,8,8,17,
+ 17,13,13,8,8,8,8,17,29,13,50,49,49,61,50,61,
+ 50,61,61,61,
+ 49,49,49,49,49,49,49,49,49,49,12,8,8,29,27,27,
+ 27,17,17,17,8,8,17,41,60,32,78,66,52,78,78,52,
+ 52,52,32,111,98,37,32,66,32,99,80,53,100,100,100,100,
+ 100,109,109,109,68,83,55,19,44,32,26,26,22,65,29,27,
+ 13,13,17,8,8,17,8,8,8,8,17,17,17,17,13,27,
+ 27,27,17,8,8,8,13,29,29,13,50,49,49,61,50,50,
+ 50,50,50,70,
+ 49,49,49,49,49,49,49,49,49,49,12,17,8,17,17,17,
+ 17,17,17,8,17,13,41,41,40,66,78,52,44,66,78,52,
+ 52,32,44,111,111,34,19,52,44,60,80,76,89,106,106,100,
+ 109,109,96,96,53,68,19,7,52,17,59,59,40,55,55,29,
+ 8,8,8,8,8,8,17,17,17,17,27,13,13,13,17,17,
+ 17,17,8,8,8,8,8,8,8,13,61,50,49,50,50,50,
+ 61,61,61,70,
+ 49,49,49,49,49,49,49,49,49,49,31,17,17,8,17,17,
+ 17,17,8,41,41,41,39,52,52,78,66,52,32,52,78,66,
+ 32,32,60,111,98,19,32,19,52,32,40,76,69,119,109,109,
+ 109,109,96,88,42,29,7,19,32,19,98,98,60,30,55,55,
+ 29,8,8,17,17,13,27,17,17,17,17,17,17,8,8,8,
+ 8,8,8,8,8,8,8,8,8,13,50,50,49,50,50,50,
+ 50,61,50,46,
+ 49,49,49,49,49,49,49,49,49,49,12,8,17,17,17,17,
+ 17,8,42,59,26,40,40,78,66,66,52,44,32,52,78,78,
+ 44,32,80,111,82,19,66,32,32,52,30,26,69,100,100,100,
+ 100,89,77,69,19,19,19,19,19,40,111,111,60,52,32,55,
+ 67,55,27,13,13,27,17,17,17,17,8,8,17,8,8,17,
+ 17,8,8,17,8,8,8,8,8,13,50,50,49,49,61,50,
+ 50,61,50,70,
+ 49,49,49,49,49,49,49,49,49,49,12,8,8,17,17,17,
+ 8,29,59,41,44,66,78,78,66,52,32,32,19,52,66,78,
+ 52,32,121,111,59,32,32,66,19,44,52,19,29,34,59,59,
+ 39,34,34,7,19,19,19,7,19,85,111,111,32,52,30,30,
+ 67,67,53,29,17,17,17,17,17,17,17,17,17,17,17,17,
+ 17,17,8,8,8,8,8,8,8,13,50,49,49,49,61,50,
+ 50,61,50,70,
+ 49,49,49,49,49,49,49,49,49,49,12,17,17,17,17,8,
+ 17,60,44,66,78,66,78,78,52,44,32,19,30,44,44,78,
+ 66,32,111,111,39,52,22,66,52,19,52,32,19,31,31,31,
+ 31,31,13,19,19,19,19,32,26,111,111,85,30,32,44,30,
+ 30,55,55,67,30,29,17,17,17,17,17,17,17,17,17,17,
+ 29,29,8,8,8,8,8,8,8,13,50,49,49,49,61,61,
+ 61,61,61,49,
+ 49,49,49,49,49,49,49,49,49,49,12,17,17,17,17,19,
+ 29,32,78,90,78,66,66,52,44,44,32,7,32,32,44,66,
+ 78,52,111,111,13,52,32,32,66,32,32,66,32,31,46,46,
+ 46,31,32,32,7,32,32,32,59,111,111,39,32,52,65,30,
+ 30,19,30,67,67,67,17,17,17,17,17,17,17,17,17,17,
+ 17,17,17,8,8,17,8,8,8,13,50,50,49,49,61,61,
+ 61,61,61,49,
+ 49,49,49,49,49,49,49,49,49,49,12,17,17,17,17,41,
+ 32,32,90,78,66,66,66,32,32,19,32,7,19,32,44,52,
+ 78,78,111,98,32,32,66,32,66,66,19,52,44,12,49,49,
+ 46,66,66,32,19,32,52,30,98,111,98,19,32,66,52,52,
+ 52,19,19,30,83,83,55,8,17,17,17,17,17,17,17,17,
+ 17,8,8,17,8,17,17,8,8,13,50,50,49,61,61,61,
+ 61,61,49,49,
+ 49,49,49,49,49,49,49,49,49,49,12,8,17,17,41,41,
+ 66,44,78,78,66,32,44,32,19,7,19,19,19,19,44,44,
+ 78,78,113,82,66,32,52,32,44,66,32,32,52,34,61,49,
+ 59,78,32,19,32,32,52,26,98,111,79,32,32,32,44,66,
+ 32,44,30,30,67,83,67,29,17,17,17,17,17,17,17,14,
+ 8,17,26,59,41,8,8,8,8,13,50,49,49,50,61,50,
+ 50,49,49,49,
+ 49,49,49,49,49,49,49,49,49,49,12,8,8,19,89,78,
+ 66,32,44,52,32,19,32,32,7,19,19,7,7,30,52,32,
+ 78,80,111,79,78,78,32,66,32,66,52,19,44,26,61,58,
+ 78,32,19,32,32,40,41,59,98,111,40,32,44,32,66,52,
+ 32,44,32,32,30,67,67,55,27,27,27,27,43,27,27,17,
+ 41,76,99,121,93,17,8,8,8,27,61,49,49,50,50,50,
+ 61,49,49,49,
+ 49,49,49,49,49,49,49,49,49,49,12,17,17,19,78,66,
+ 32,32,19,19,19,19,32,19,7,19,7,7,7,19,52,32,
+ 66,81,111,59,78,78,32,52,52,52,52,32,32,34,46,66,
+ 44,19,32,32,37,82,58,85,98,98,7,32,44,52,52,32,
+ 44,32,52,32,30,83,83,55,17,17,17,17,27,27,39,76,
+ 99,121,93,93,93,29,8,17,8,12,50,49,49,49,49,61,
+ 49,49,61,49,
+ 49,49,49,49,49,49,49,49,49,49,12,8,41,19,32,32,
+ 32,52,7,32,32,19,19,19,7,7,7,7,7,19,44,44,
+ 44,95,111,39,78,78,52,44,66,52,52,44,22,26,59,44,
+ 19,32,52,34,82,59,59,98,98,85,4,32,32,52,19,32,
+ 32,44,44,19,30,83,83,67,14,14,17,13,39,79,99,121,
+ 121,93,58,58,59,29,17,17,17,12,50,49,49,49,49,61,
+ 49,49,49,49,
+ 49,49,49,49,49,49,49,49,49,49,12,8,60,32,32,32,
+ 32,32,32,19,19,19,8,19,19,19,30,19,7,19,32,52,
+ 32,111,111,39,78,66,44,52,66,52,52,52,32,26,52,19,
+ 32,32,44,31,95,26,60,98,98,59,7,32,7,19,19,32,
+ 32,52,32,7,29,83,83,67,42,34,59,79,99,121,93,99,
+ 79,58,41,29,29,17,17,17,27,12,50,49,49,49,49,49,
+ 49,49,49,49,
+ 49,49,49,49,49,49,49,49,49,49,12,8,40,52,66,52,
+ 44,32,32,19,19,19,19,19,19,19,29,17,19,7,19,52,
+ 52,111,111,39,66,66,32,52,66,66,52,52,32,44,32,19,
+ 44,32,32,34,85,39,39,93,93,39,7,7,7,19,7,7,
+ 32,52,32,19,7,55,55,76,80,99,98,93,93,79,79,59,
+ 41,29,17,8,8,8,8,8,17,12,50,49,49,49,49,50,
+ 49,49,49,49,
+ 49,49,49,49,49,49,49,49,49,49,12,8,26,52,66,78,
+ 78,52,22,19,19,30,30,29,17,17,17,17,29,26,19,32,
+ 66,98,111,34,52,78,32,32,66,66,44,52,32,44,44,22,
+ 32,32,32,26,39,39,39,93,82,13,7,13,13,34,13,13,
+ 26,32,19,32,13,59,79,85,93,93,82,79,58,34,29,29,
+ 17,17,17,17,17,17,17,8,8,12,50,49,49,49,49,49,
+ 49,49,49,49,
+ 49,49,49,49,49,49,49,49,49,49,12,29,7,44,78,78,
+ 66,44,19,7,19,29,17,17,13,27,13,17,17,41,19,19,
+ 52,98,98,39,32,66,52,32,52,66,32,32,44,32,52,32,
+ 32,32,32,32,13,7,37,82,79,8,19,58,79,58,79,58,
+ 37,7,26,59,79,93,93,93,79,58,34,42,29,17,14,17,
+ 17,27,27,27,27,27,27,17,17,12,50,49,49,49,49,49,
+ 49,49,49,49,
+ 49,49,49,49,49,49,49,49,49,49,12,41,7,52,78,78,
+ 66,66,32,7,19,13,27,27,17,17,17,8,8,29,19,7,
+ 40,98,93,58,26,52,66,19,32,52,32,32,44,22,66,52,
+ 32,32,32,32,19,7,59,85,59,7,39,79,93,70,93,70,
+ 34,34,82,93,93,82,79,37,41,42,29,17,17,17,17,17,
+ 17,27,17,17,17,17,17,27,27,12,49,49,49,49,49,49,
+ 49,49,49,49,
+ 49,49,49,49,49,49,49,49,49,49,12,60,41,52,78,78,
+ 78,52,32,19,32,29,17,17,17,8,8,17,17,17,41,32,
+ 60,98,98,93,98,85,80,40,32,32,32,32,32,19,66,44,
+ 32,19,32,19,7,7,59,59,26,26,93,51,121,70,93,82,
+ 13,58,93,82,58,34,29,29,30,67,17,14,14,17,17,17,
+ 17,17,17,27,42,17,8,8,8,12,50,49,49,49,49,49,
+ 49,49,49,49,
+ 49,49,49,49,49,49,49,49,49,49,13,60,59,52,78,66,
+ 52,22,32,7,32,30,8,8,8,17,27,27,17,17,29,59,
+ 80,98,82,98,111,111,98,98,85,80,59,60,60,40,52,44,
+ 32,26,32,26,40,39,59,39,22,79,93,46,93,57,79,93,
+ 37,34,37,34,29,19,30,19,30,83,55,17,17,17,8,8,
+ 8,17,17,17,27,17,8,17,8,12,50,49,49,49,49,49,
+ 49,49,49,49,
+ 49,49,49,49,49,49,49,49,49,49,12,41,40,66,78,32,
+ 32,32,32,7,22,30,8,8,17,34,27,17,17,8,8,39,
+ 85,93,58,40,80,95,98,111,111,111,98,85,98,98,62,85,
+ 85,85,95,95,95,81,59,13,59,82,93,51,93,75,79,98,
+ 51,27,13,27,7,32,32,30,67,83,83,55,17,27,17,17,
+ 17,17,8,8,8,17,17,17,17,12,49,49,49,49,49,49,
+ 49,49,49,49,
+ 49,49,49,49,49,49,49,49,49,49,12,29,22,66,78,78,
+ 66,52,19,19,7,30,8,17,17,17,17,17,17,17,13,19,
+ 59,93,22,19,52,52,60,80,81,95,95,85,85,93,85,98,
+ 98,98,98,85,80,59,39,39,79,70,93,82,93,82,70,93,
+ 51,12,13,34,7,52,19,30,67,83,83,83,17,17,27,27,
+ 27,17,8,8,17,8,8,17,17,12,49,49,49,49,49,49,
+ 49,49,49,49,
+ 49,49,49,49,49,49,49,49,49,49,12,8,26,52,78,78,
+ 78,78,19,32,7,29,8,17,17,8,17,17,17,27,13,19,
+ 39,58,32,32,32,52,32,32,44,52,40,59,59,59,60,80,
+ 59,40,26,26,39,59,85,93,58,82,82,93,82,82,51,51,
+ 31,31,12,37,19,32,19,30,67,67,83,83,29,17,27,27,
+ 27,17,17,17,17,17,8,8,8,12,49,49,49,49,49,49,
+ 49,49,49,49,
+ 49,49,49,49,49,49,49,49,49,49,12,17,41,32,66,66,
+ 78,78,19,44,19,8,14,8,17,17,17,17,27,13,17,19,
+ 26,34,52,66,32,44,52,32,32,52,32,32,44,32,19,19,
+ 19,19,39,59,79,93,93,82,37,93,93,93,51,70,31,46,
+ 51,31,12,34,19,32,32,19,55,67,83,83,29,17,17,17,
+ 17,27,13,27,17,13,13,17,17,12,50,49,49,49,49,49,
+ 49,49,49,49,
+ 49,49,46,49,49,49,49,49,49,49,27,29,26,52,66,66,
+ 80,52,32,44,7,14,8,17,17,17,17,27,27,17,8,13,
+ 26,19,32,66,66,32,52,52,32,32,52,32,32,19,19,26,
+ 59,79,93,93,93,79,37,34,26,93,51,70,31,46,51,51,
+ 46,51,12,42,22,19,32,30,30,67,67,83,42,17,17,17,
+ 17,17,17,17,17,13,13,17,17,31,49,49,49,49,49,49,
+ 49,49,49,49,
+ 49,49,70,79,51,49,49,49,49,49,13,29,32,44,66,78,
+ 78,52,44,32,19,8,17,17,14,17,27,27,27,17,17,8,
+ 26,19,32,44,78,52,52,52,44,19,32,32,26,40,59,79,
+ 93,93,82,58,39,26,22,7,7,58,51,46,31,46,70,51,
+ 46,31,31,27,34,26,19,32,19,19,55,67,55,17,17,17,
+ 17,17,8,17,8,17,17,13,17,31,49,49,49,49,49,49,
+ 49,49,49,49,
+ 49,49,46,70,89,79,49,46,46,49,13,8,32,22,52,60,
+ 32,52,66,7,30,8,17,17,17,27,13,27,17,17,17,8,
+ 39,19,32,32,66,78,66,52,44,22,19,39,59,85,82,82,
+ 79,37,34,22,19,7,30,7,8,13,58,51,70,46,46,51,
+ 51,31,31,13,13,26,32,30,19,19,30,55,55,17,17,17,
+ 17,17,17,8,8,17,8,17,17,12,49,49,49,49,49,49,
+ 49,49,49,49,
+ 79,46,49,49,58,89,79,51,79,49,13,8,41,26,78,44,
+ 66,52,52,7,29,8,14,17,27,13,27,17,17,17,8,13,
+ 26,19,44,32,52,52,44,44,40,59,79,82,82,82,58,39,
+ 26,13,7,19,7,7,19,8,30,19,13,59,70,46,51,51,
+ 51,31,37,13,13,8,32,30,30,55,30,30,55,17,17,17,
+ 17,17,17,8,17,17,8,17,17,12,49,49,49,49,49,49,
+ 49,49,49,49,
+ 89,79,46,49,46,77,89,51,79,99,13,8,41,60,78,78,
+ 78,78,66,7,8,17,27,27,17,34,17,17,17,17,17,27,
+ 17,19,52,32,32,40,40,59,82,82,82,58,39,26,22,19,
+ 19,7,7,7,7,7,7,7,55,13,8,13,34,37,51,51,
+ 31,12,12,31,12,22,22,19,55,67,55,32,67,17,17,17,
+ 17,17,8,8,17,8,8,17,17,12,49,49,49,49,49,49,
+ 49,49,49,49,
+ 76,89,79,46,49,77,89,46,89,100,41,8,60,60,66,52,
+ 60,40,60,19,17,27,27,17,14,27,17,17,17,17,27,17,
+ 8,32,32,40,39,79,82,82,82,58,39,22,19,7,19,7,
+ 19,7,8,7,7,7,8,22,29,13,17,8,8,17,12,31,
+ 31,31,31,51,40,44,32,7,30,55,55,55,55,17,14,17,
+ 27,17,17,17,17,8,8,17,8,12,49,49,49,49,49,49,
+ 49,49,49,49,
+ 79,77,80,70,46,89,77,46,100,77,76,8,60,66,60,59,
+ 37,37,13,19,29,13,17,14,17,17,17,17,17,17,17,8,
+ 8,40,59,79,82,82,58,37,34,26,19,19,32,22,7,7,
+ 7,7,7,7,7,8,12,12,27,17,8,17,17,8,8,37,
+ 37,31,34,40,78,52,32,7,7,30,30,55,30,27,17,17,
+ 17,17,17,17,17,17,8,8,8,12,49,49,49,49,49,49,
+ 49,49,49,49,
+ 99,76,79,70,58,89,76,46,89,87,69,8,19,40,46,46,
+ 46,46,12,13,17,17,14,8,17,17,17,14,14,13,29,39,
+ 59,79,79,58,58,37,34,32,19,30,19,19,19,19,7,7,
+ 8,13,13,13,13,12,34,34,67,55,29,17,17,17,8,41,
+ 60,60,32,44,66,52,44,19,19,19,30,30,55,17,27,17,
+ 17,17,17,17,17,17,17,17,8,12,49,49,49,49,49,49,
+ 49,49,49,49,
+ 100,89,79,70,76,76,58,89,89,89,29,8,8,37,45,51,
+ 51,57,51,12,8,14,17,17,17,8,8,13,34,58,58,82,
+ 82,58,39,26,34,31,31,37,34,22,19,13,13,13,13,12,
+ 12,12,37,12,34,40,52,65,72,87,67,17,8,17,17,17,
+ 41,60,44,44,44,52,44,32,32,19,7,30,67,8,17,17,
+ 17,17,17,17,8,17,17,17,13,31,49,49,49,49,49,49,
+ 49,49,49,49,
+ 89,89,99,79,89,77,89,100,79,49,17,17,8,12,51,82,
+ 82,82,70,58,27,14,14,8,17,34,58,79,82,82,58,39,
+ 26,60,66,52,44,34,31,46,46,31,31,31,31,12,12,31,
+ 31,34,34,26,66,52,52,66,65,67,87,55,17,8,17,17,
+ 8,29,66,44,52,52,52,66,52,7,19,19,67,8,8,17,
+ 17,17,17,17,17,17,8,17,17,31,49,49,49,49,49,49,
+ 49,49,49,49,
+ 89,89,100,77,89,100,99,70,49,47,13,8,8,8,58,93,
+ 82,93,70,93,63,42,13,39,58,82,82,82,58,34,29,8,
+ 8,78,66,66,66,52,40,39,34,37,37,37,12,34,26,40,
+ 60,40,32,66,66,32,32,52,52,65,87,87,67,29,17,17,
+ 17,8,80,66,66,52,66,66,44,19,22,30,30,14,17,17,
+ 17,17,17,17,17,17,8,17,8,31,50,50,49,50,49,49,
+ 49,49,49,49,
+ 100,77,89,77,100,89,99,49,47,50,17,17,8,8,82,93,
+ 82,75,75,93,34,59,79,82,82,58,39,29,17,14,14,17,
+ 19,66,44,52,52,66,52,44,32,32,22,22,26,32,44,44,
+ 32,32,52,78,32,19,52,66,66,52,65,72,87,87,67,17,
+ 17,17,41,78,52,52,32,32,32,32,32,65,17,14,14,14,
+ 17,17,13,17,17,8,17,17,8,31,50,50,50,50,49,50,
+ 49,49,49,49,
+ 100,77,77,89,100,76,77,63,49,47,8,8,8,13,93,93,
+ 75,70,75,58,13,82,82,58,34,27,8,14,8,14,17,13,
+ 17,60,66,66,52,52,52,52,44,44,44,44,44,44,52,44,
+ 44,32,66,32,32,32,52,44,44,32,32,30,65,87,87,67,
+ 29,17,17,41,41,32,32,19,32,19,30,55,8,17,14,14,
+ 14,17,17,13,17,17,17,8,17,31,50,50,50,50,50,50,
+ 49,49,49,49,
+ 100,89,77,106,89,74,65,55,63,49,17,17,13,34,93,82,
+ 75,75,93,51,13,37,34,17,14,27,14,17,14,14,27,17,
+ 17,60,66,52,66,78,66,52,52,52,52,52,44,44,52,52,
+ 52,44,52,32,32,32,32,32,32,32,32,32,32,32,65,87,
+ 67,29,14,8,42,41,22,32,44,7,29,17,14,17,17,17,
+ 14,17,8,17,17,27,17,17,17,31,50,50,50,50,50,50,
+ 49,49,49,49,
+ 89,89,89,100,100,69,69,42,65,63,53,42,13,34,75,82,
+ 75,75,82,46,27,34,34,14,27,17,17,17,14,17,42,8,
+ 17,13,66,66,78,66,52,66,66,44,52,44,44,32,32,52,
+ 66,52,32,32,22,32,32,32,32,32,32,32,32,32,30,55,
+ 87,74,29,14,17,17,8,29,29,17,29,8,17,8,17,17,
+ 17,8,8,8,8,17,34,27,8,31,50,50,49,50,50,49,
+ 49,49,49,49,
+ 76,100,100,89,100,76,71,42,69,55,63,53,42,79,75,75,
+ 57,51,46,51,51,34,27,17,34,14,17,8,8,27,27,13,
+ 17,26,60,52,52,52,78,78,66,44,44,44,32,32,32,32,
+ 44,44,22,32,22,32,22,19,19,32,32,32,32,32,22,19,
+ 55,87,87,55,17,8,17,27,14,17,27,17,17,17,17,17,
+ 17,17,8,8,8,8,17,17,8,31,50,50,49,50,50,50,
+ 49,49,49,49,
+ 89,100,89,100,100,100,71,69,53,69,58,79,42,58,58,51,
+ 51,51,46,51,70,34,8,34,17,8,17,17,17,43,8,13,
+ 8,41,40,52,32,66,78,52,66,44,44,32,22,22,32,22,
+ 19,32,19,32,19,19,19,22,32,32,32,32,32,32,32,32,
+ 19,55,87,87,67,17,17,17,17,8,17,27,17,17,17,17,
+ 17,27,17,17,8,17,8,8,8,31,50,50,49,50,50,49,
+ 49,49,49,49,
+ 89,89,89,100,100,100,77,74,58,79,82,58,29,42,17,12,
+ 34,37,37,37,34,17,14,27,2,17,17,17,17,17,17,17,
+ 17,13,78,44,44,78,44,52,66,52,52,32,19,19,7,19,
+ 7,19,19,19,7,19,19,19,19,19,19,19,19,19,32,32,
+ 32,19,65,87,87,55,17,17,17,17,17,17,27,17,8,17,
+ 8,17,27,27,8,8,8,8,8,31,50,50,50,50,49,49,
+ 49,49,49,49,
+ 77,89,89,77,100,100,89,68,58,58,39,42,19,42,8,8,
+ 8,13,8,13,14,14,8,8,17,68,17,17,17,8,13,17,
+ 8,29,78,32,52,52,52,78,78,52,52,44,32,19,19,7,
+ 7,7,19,19,7,7,7,7,7,7,7,7,7,19,19,22,
+ 32,19,19,55,87,87,30,8,17,17,17,8,17,27,17,8,
+ 8,8,17,27,8,8,8,8,8,31,50,50,50,50,50,49,
+ 50,49,49,49,
+ 77,69,69,77,77,100,106,68,69,53,42,69,29,42,8,8,
+ 17,8,8,8,8,8,8,8,88,68,8,17,8,17,17,17,
+ 8,60,32,32,52,44,78,90,90,66,66,44,32,19,32,19,
+ 19,8,7,7,7,7,7,7,7,7,7,19,19,19,19,19,
+ 19,19,19,19,67,87,87,17,17,17,17,17,8,17,27,17,
+ 17,8,8,17,8,8,8,8,8,31,50,50,49,50,50,49,
+ 49,49,49,49,
+ 89,77,89,74,69,100,100,89,71,69,42,69,53,29,8,17,
+ 17,8,8,8,8,8,8,68,103,42,8,17,17,17,17,17,
+ 8,42,41,32,44,52,78,90,90,78,66,44,44,32,32,19,
+ 19,19,8,7,7,7,7,7,7,19,19,19,19,19,32,32,
+ 19,32,32,19,19,67,87,55,8,17,17,17,17,14,17,27,
+ 17,17,17,8,17,8,8,8,8,31,50,50,49,50,50,50,
+ 49,49,49,49,
+ 77,89,89,77,77,100,100,106,71,69,53,53,53,29,8,17,
+ 8,17,29,8,8,8,8,96,56,17,17,42,42,13,17,8,
+ 17,17,59,32,32,66,78,90,90,90,78,52,44,32,32,32,
+ 19,19,19,19,19,19,7,8,8,7,7,7,19,19,32,32,
+ 32,32,32,32,19,19,55,87,29,8,17,8,17,17,8,17,
+ 27,13,17,8,17,8,8,8,8,31,50,50,49,50,50,50,
+ 49,49,49,49,
+ 89,89,77,89,89,89,100,100,77,68,69,42,68,68,8,8,
+ 17,8,53,42,8,8,29,96,56,17,8,74,29,17,17,17,
+ 17,14,59,32,32,66,90,90,90,90,78,52,44,32,32,32,
+ 19,7,30,8,8,19,19,19,8,8,8,8,7,7,19,19,
+ 19,22,32,32,32,19,19,65,67,17,8,17,8,17,17,14,
+ 17,27,27,17,8,17,8,8,8,31,50,50,49,50,49,49,
+ 49,49,49,49,
+ 89,89,89,89,88,89,89,100,106,68,69,53,77,74,29,17,
+ 17,17,17,68,8,17,42,103,56,71,8,67,8,8,17,17,
+ 14,17,59,44,32,66,90,90,90,90,78,52,44,32,19,32,
+ 19,8,55,8,8,8,19,30,19,7,7,8,7,7,7,7,
+ 7,19,19,22,22,22,22,32,67,30,8,8,17,17,8,8,
+ 8,17,27,27,17,8,17,8,8,31,50,50,50,50,49,49,
+ 50,49,49,49,
+ 77,89,89,89,89,74,77,100,106,77,74,77,77,53,53,8,
+ 8,17,8,42,17,88,42,83,56,96,7,29,8,8,29,33,
+ 14,8,60,44,44,78,90,90,90,90,90,66,52,32,19,22,
+ 19,8,55,8,8,8,8,17,30,32,19,19,7,7,7,7,
+ 7,7,19,19,19,19,32,52,55,55,8,8,8,8,8,8,
+ 8,8,17,27,34,17,17,8,8,31,50,50,50,50,50,50,
+ 50,49,49,49,
+ 89,74,100,100,77,77,77,89,89,89,69,88,68,68,53,17,
+ 29,8,8,8,17,87,29,83,103,88,7,42,8,35,33,14,
+ 17,17,60,44,44,78,90,90,90,90,90,66,52,32,19,7,
+ 19,4,55,19,8,17,8,17,17,29,29,30,19,7,7,7,
+ 7,7,7,7,19,19,19,32,65,67,29,8,8,8,17,8,
+ 8,8,8,17,34,34,8,8,8,31,50,50,50,50,50,50,
+ 49,49,49,49,
+ 100,89,100,77,89,77,77,69,77,89,74,53,53,68,68,77,
+ 77,29,8,8,27,67,53,103,96,84,8,88,14,33,33,33,
+ 14,14,60,44,44,78,90,90,90,90,90,78,52,32,7,19,
+ 7,8,55,19,17,17,17,17,17,17,17,29,30,32,19,19,
+ 19,19,19,32,32,32,32,22,32,55,55,17,8,8,17,17,
+ 8,8,8,8,17,27,8,8,8,31,50,50,50,50,50,50,
+ 49,49,49,49,
+ 89,89,77,89,100,77,77,69,77,77,68,42,68,42,68,120,
+ 109,77,42,42,84,83,88,96,56,55,17,88,33,33,33,33,
+ 14,14,40,32,44,78,90,90,90,90,90,78,52,32,7,19,
+ 7,8,55,17,17,17,17,17,17,17,14,14,14,30,30,32,
+ 32,22,19,32,32,52,52,44,32,32,65,67,17,8,8,27,
+ 17,8,8,8,8,8,8,8,8,31,50,50,50,50,50,50,
+ 50,49,49,49,
+ 89,100,89,100,100,89,77,89,69,89,53,68,53,43,71,119,
+ 119,109,100,88,84,83,84,96,56,42,68,56,33,33,33,33,
+ 33,29,60,32,44,66,66,66,78,78,78,78,66,32,19,19,
+ 7,8,55,17,17,17,17,17,17,17,17,14,14,35,29,30,
+ 19,19,19,32,32,32,52,66,66,52,32,67,67,8,8,17,
+ 27,17,8,17,8,8,8,8,8,31,50,50,50,50,50,50,
+ 50,50,49,49,
+ 89,89,100,89,89,89,89,89,53,89,53,88,42,56,71,109,
+ 120,109,96,96,84,55,84,84,83,53,96,56,33,33,33,33,
+ 35,29,60,19,32,44,52,52,44,44,52,52,66,32,32,7,
+ 19,4,67,8,17,17,17,17,17,14,14,33,33,33,33,35,
+ 29,19,32,32,32,32,32,66,66,78,52,32,87,17,8,8,
+ 17,34,17,8,17,8,8,8,8,31,50,50,50,50,50,50,
+ 50,49,50,49,
+ 69,69,89,89,89,77,69,77,68,69,69,89,68,33,68,68,
+ 96,88,71,108,84,71,84,83,83,84,96,35,35,33,35,33,
+ 35,33,60,32,32,44,52,66,66,52,44,32,32,32,19,7,
+ 32,7,55,17,14,14,14,14,14,33,33,33,33,33,33,33,
+ 35,22,44,32,32,32,32,52,66,78,78,32,32,55,8,8,
+ 8,17,34,17,8,17,8,8,8,31,50,50,50,50,50,50,
+ 50,49,49,49,
+ 76,89,89,89,74,69,53,69,69,42,100,74,88,42,68,56,
+ 68,68,88,88,88,96,83,84,56,83,88,35,35,33,35,35,
+ 33,33,42,44,19,52,66,90,90,90,66,32,32,19,7,19,
+ 32,19,55,14,33,14,14,14,33,35,33,33,35,33,35,33,
+ 29,44,32,52,32,44,44,32,52,66,78,44,32,72,29,8,
+ 8,8,27,17,8,8,17,8,8,31,50,50,50,50,50,49,
+ 50,49,49,49,
+ 89,106,89,77,69,53,77,74,53,89,100,68,71,71,56,84,
+ 84,84,84,88,103,84,56,68,84,96,84,35,35,35,35,35,
+ 35,35,29,60,19,52,66,90,90,90,78,66,32,19,19,19,
+ 19,19,30,14,33,35,35,35,35,35,35,35,33,35,29,29,
+ 19,32,19,32,44,32,52,44,32,52,52,32,32,72,29,8,
+ 8,8,8,17,8,8,8,17,8,31,50,50,50,50,50,49,
+ 49,49,49,49,
+ 89,89,77,69,69,53,77,53,77,106,74,68,71,96,68,84,
+ 84,84,84,96,84,84,53,84,96,96,56,35,35,35,35,35,
+ 35,35,35,60,32,32,66,78,90,90,90,78,32,32,19,32,
+ 19,19,30,14,35,35,35,35,35,35,35,35,35,29,8,19,
+ 19,19,19,22,22,22,32,52,44,44,44,32,32,72,8,8,
+ 8,17,8,8,8,8,8,8,8,31,50,50,50,50,50,49,
+ 50,49,49,49,
+ 76,76,77,77,69,69,77,53,100,89,68,89,109,88,88,56,
+ 84,84,96,108,83,56,84,84,84,53,53,35,56,56,35,35,
+ 35,35,35,60,32,32,52,78,90,90,90,78,52,44,19,32,
+ 19,7,55,33,35,35,35,35,35,35,35,35,17,13,8,32,
+ 19,7,19,19,19,19,19,32,44,52,52,52,52,52,8,8,
+ 8,8,8,8,8,8,8,8,8,31,50,50,50,50,50,50,
+ 50,49,49,49,
+ 76,89,77,77,69,69,71,71,89,68,77,100,120,96,88,88,
+ 84,84,96,96,84,56,103,83,83,42,71,35,56,56,35,35,
+ 35,35,35,60,19,32,44,78,90,90,90,78,78,44,32,32,
+ 19,7,65,33,35,35,35,35,35,35,35,35,17,8,8,19,
+ 32,19,32,7,32,19,19,32,32,44,52,44,52,65,14,8,
+ 8,8,17,8,8,8,8,17,8,31,50,49,50,50,50,50,
+ 49,49,49,49,
+ 77,76,76,77,74,53,89,71,71,71,100,120,120,120,96,88,
+ 88,84,84,96,84,84,103,56,56,63,88,71,35,56,56,56,
+ 56,35,35,69,32,32,32,66,90,90,90,90,78,44,32,32,
+ 7,19,52,35,35,35,35,35,35,35,35,35,14,8,8,8,
+ 32,32,19,32,32,19,19,32,32,32,32,32,52,55,56,35,
+ 17,8,8,17,8,8,8,8,8,31,50,50,50,50,50,50,
+ 49,49,49,49,
+ 59,51,51,89,55,69,100,88,68,109,109,120,120,120,109,88,
+ 71,71,56,84,84,96,96,56,68,71,109,109,35,56,56,56,
+ 56,56,56,56,40,32,32,52,90,90,90,90,78,52,44,19,
+ 7,19,32,42,35,35,35,35,35,35,35,35,35,8,8,8,
+ 19,32,32,32,32,19,32,22,32,32,32,40,53,56,56,56,
+ 56,17,8,8,17,8,8,8,8,31,50,50,50,50,49,50,
+ 50,49,49,49,
+ 46,70,76,74,53,100,88,96,77,109,120,120,120,120,109,88,
+ 84,68,68,56,84,88,96,56,84,71,120,109,56,56,56,56,
+ 56,56,56,35,69,32,32,44,78,90,90,90,90,66,52,19,
+ 7,19,22,55,35,35,35,56,35,35,35,35,35,17,8,8,
+ 7,19,32,19,32,32,30,30,30,19,42,56,56,56,56,56,
+ 56,56,35,17,8,8,8,8,8,31,50,50,50,50,49,50,
+ 50,49,49,49,
+ 58,76,89,42,89,100,68,88,89,109,120,120,120,109,96,84,
+ 84,56,68,88,96,84,84,56,84,71,109,68,71,56,56,56,
+ 56,56,56,56,56,60,32,32,66,90,90,90,90,78,44,32,
+ 7,19,22,67,35,35,56,56,56,56,35,35,35,35,17,8,
+ 8,7,7,19,7,19,42,56,35,35,56,56,56,56,56,56,
+ 56,56,56,35,17,8,8,8,8,31,50,50,50,50,50,50,
+ 50,49,49,49,
+ 77,77,69,69,100,71,71,109,109,77,100,109,109,109,88,84,
+ 84,84,84,88,109,88,56,56,71,71,96,68,68,56,56,56,
+ 56,56,56,56,56,60,44,32,44,78,90,90,90,90,44,32,
+ 7,19,32,67,35,56,56,56,56,56,56,56,35,56,35,4,
+ 8,8,8,17,17,17,56,56,56,56,56,56,56,56,56,56,
+ 56,56,56,56,56,35,17,8,8,31,50,49,50,50,50,50,
+ 49,50,49,49,
+ 58,76,69,100,71,71,109,120,120,109,71,71,88,88,71,88,
+ 71,71,71,84,88,71,53,53,71,84,108,88,67,56,56,56,
+ 56,56,56,56,56,69,32,40,32,52,78,90,90,90,52,32,
+ 19,7,32,65,35,56,56,56,56,56,56,56,35,56,56,4,
+ 8,4,8,29,17,8,56,56,56,56,56,56,56,56,56,56,
+ 56,56,56,56,56,56,56,17,14,31,50,50,50,50,50,50,
+ 49,49,49,49,
+ 51,41,77,88,71,109,120,120,120,120,109,88,68,56,56,68,
+ 68,68,84,56,43,68,68,68,88,71,96,68,56,56,56,56,
+ 56,56,56,56,56,53,44,32,32,44,66,78,90,78,78,32,
+ 22,7,32,32,56,56,56,56,56,56,56,56,56,56,56,8,
+ 4,4,4,19,17,8,56,56,56,56,56,56,56,56,56,56,
+ 56,56,56,56,56,56,56,56,29,31,50,50,50,50,50,50,
+ 50,49,49,49,
+ 63,42,88,68,88,120,120,120,120,120,120,96,84,56,56,56,
+ 56,84,56,35,35,68,63,63,51,46,51,51,46,31,46,46,
+ 46,51,46,46,46,46,60,19,32,32,52,78,90,90,78,32,
+ 32,7,30,30,63,46,46,46,46,46,46,46,46,46,46,13,
+ 7,4,4,8,8,8,46,46,46,46,46,46,46,46,46,46,
+ 46,46,46,46,46,46,46,46,46,49,50,50,50,50,50,50,
+ 49,49,49,49,
+ 58,42,71,88,109,120,120,120,120,109,96,84,84,84,84,84,
+ 84,56,35,33,56,71,73,51,50,50,50,50,50,50,50,50,
+ 50,50,50,47,47,50,46,26,32,52,44,78,90,90,90,66,
+ 44,7,19,30,69,47,50,50,47,47,47,47,47,47,47,12,
+ 17,4,4,8,8,7,49,47,47,47,47,47,50,50,50,50,
+ 50,50,50,50,50,50,47,50,50,50,50,50,50,50,50,49,
+ 50,50,49,49,
+ 58,53,68,109,120,120,120,120,120,108,84,71,84,84,84,84,
+ 56,35,33,43,63,73,46,50,47,47,47,47,47,47,47,47,
+ 47,47,47,47,47,47,47,51,32,66,32,66,90,90,90,90,
+ 32,19,7,30,65,47,47,47,47,47,47,47,47,47,47,46,
+ 8,4,8,8,8,29,12,47,50,50,50,50,50,50,50,50,
+ 50,50,50,50,50,50,50,50,50,50,50,50,50,50,50,50,
+ 50,50,50,49,
+ 68,55,53,120,120,120,120,120,108,88,84,84,84,84,84,56,
+ 35,33,56,63,73,46,50,50,70,70,70,61,61,61,61,61,
+ 61,49,50,50,50,49,50,58,44,26,32,52,66,78,78,78,
+ 32,32,30,7,26,49,49,49,45,49,49,49,49,49,49,47,
+ 12,7,8,8,8,56,13,47,50,50,50,50,50,50,50,50,
+ 50,50,50,50,50,50,50,50,50,50,50,50,50,50,50,50,
+ 50,50,50,49,
+ 69,68,31,109,120,120,120,108,88,84,88,84,84,84,56,35,
+ 33,56,63,73,46,50,47,61,124,118,118,118,118,118,118,118,
+ 118,118,70,50,47,31,12,13,22,7,19,19,19,19,32,22,
+ 19,19,19,7,7,12,31,12,12,12,31,12,12,12,45,50,
+ 49,8,4,7,8,29,12,50,50,50,50,50,50,50,50,50,
+ 50,50,50,50,50,50,50,50,50,50,50,50,50,50,50,50,
+ 50,50,50,49,
+ 46,46,49,51,120,120,109,88,84,88,84,84,84,56,35,33,
+ 56,63,73,49,50,50,50,70,127,114,122,123,114,122,123,114,
+ 122,124,70,50,47,12,13,4,31,19,7,4,7,7,22,4,
+ 52,19,7,7,19,31,31,12,45,4,13,13,4,12,31,50,
+ 47,46,8,7,17,13,46,50,50,50,50,50,50,50,50,50,
+ 50,50,50,50,50,50,50,50,50,50,50,61,50,50,50,50,
+ 50,50,49,49,
+ 50,47,50,49,73,109,96,84,88,88,84,84,84,56,35,56,
+ 63,73,49,47,50,50,50,70,124,118,118,118,118,120,122,109,
+ 120,124,70,50,47,31,13,13,70,19,7,7,32,40,52,4,
+ 52,7,7,7,19,31,31,12,46,4,12,12,4,46,13,50,
+ 50,50,46,12,31,46,50,50,50,50,50,50,50,50,50,50,
+ 50,50,50,50,50,50,50,50,50,50,50,61,50,50,50,50,
+ 50,50,49,49,
+ 49,50,50,50,49,73,88,88,88,84,84,84,56,35,56,63,
+ 73,49,47,50,50,50,50,49,49,70,70,70,70,70,70,70,
+ 70,70,49,50,47,46,31,31,49,32,22,19,44,78,90,40,
+ 40,22,52,7,19,7,46,12,31,31,46,31,12,50,12,50,
+ 50,50,47,50,50,50,50,50,50,50,50,50,50,50,50,50,
+ 50,50,50,50,50,50,50,50,50,50,50,61,50,50,50,50,
+ 50,50,50,49,
+ 49,50,50,49,47,49,73,88,84,88,84,56,56,53,63,51,
+ 49,47,50,50,50,50,50,50,47,47,47,47,47,50,50,50,
+ 50,50,50,50,50,49,49,49,50,60,52,32,32,90,90,90,
+ 90,78,78,32,32,32,46,49,49,50,50,50,49,50,49,50,
+ 50,50,50,47,47,50,50,50,50,50,50,50,50,50,50,50,
+ 50,50,50,49,50,50,50,50,49,50,49,49,49,50,49,49,
+ 49,49,49,46,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,1,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,4,4,4,4,4,4,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,1,0,1,1,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,71,109,53,29,88,42,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,1,68,53,0,0,0,
+ 0,0,0,29,68,29,53,29,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,1,71,127,108,71,108,71,
+ 29,7,4,7,7,7,7,7,19,19,4,4,29,29,29,22,
+ 19,19,7,22,19,4,7,29,19,4,53,71,7,7,19,0,
+ 0,19,22,29,71,53,0,0,22,19,42,122,108,22,0,4,
+ 22,7,4,88,122,88,114,69,4,13,4,1,4,22,19,0,
+ 0,19,29,29,7,19,7,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,4,109,127,114,96,108,
+ 120,109,88,96,88,96,96,96,114,88,29,69,108,122,120,108,
+ 108,114,88,109,120,71,68,114,122,88,122,123,88,108,122,88,
+ 88,122,108,88,123,96,4,42,114,114,96,114,123,122,71,53,
+ 114,122,68,88,114,71,114,96,88,114,114,71,96,120,114,68,
+ 71,96,122,114,96,109,122,68,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,42,124,123,120,88,
+ 71,122,124,122,96,114,114,122,122,53,29,108,114,114,114,114,
+ 123,114,120,109,109,108,88,108,122,122,122,114,123,114,108,108,
+ 108,123,108,88,123,53,13,114,96,42,68,114,108,114,114,88,
+ 96,123,108,88,108,68,114,123,122,96,96,108,122,96,114,108,
+ 114,108,108,122,123,96,108,71,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,42,124,122,124,96,
+ 68,120,122,124,114,122,114,120,120,17,7,96,123,114,53,96,
+ 124,71,122,124,71,71,122,122,114,123,122,122,127,123,71,53,
+ 88,122,123,114,123,53,13,122,122,29,42,122,108,108,123,123,
+ 108,114,114,108,120,71,122,124,127,108,42,71,122,96,114,108,
+ 108,123,108,68,123,122,68,29,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,53,120,108,88,108,
+ 108,88,68,109,120,108,96,108,108,19,4,88,123,114,71,88,
+ 109,53,88,120,96,71,108,114,114,114,114,108,96,122,108,71,
+ 96,114,108,71,108,71,4,68,122,96,71,108,108,108,114,114,
+ 108,108,108,96,108,88,108,96,108,122,88,88,108,96,108,96,
+ 96,123,114,68,88,122,108,42,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,1,7,19,4,8,4,0,0,0,7,29,19,7,29,
+ 42,7,4,29,53,68,68,29,29,7,1,29,96,108,88,29,
+ 19,7,4,42,71,29,7,29,19,7,7,19,7,29,29,4,
+ 7,29,4,7,53,29,1,1,29,68,42,19,7,19,19,7,
+ 29,7,7,19,19,19,19,7,4,29,29,7,7,7,7,4,
+ 13,88,108,71,4,7,22,0,0,7,4,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,1,96,120,68,71,29,1,4,0,0,0,7,7,4,
+ 4,4,7,1,19,108,96,7,0,1,7,7,29,68,53,4,
+ 0,1,1,69,120,42,0,0,0,1,4,0,0,0,1,1,
+ 0,0,4,96,108,4,0,0,29,108,71,0,1,4,0,0,
+ 0,0,0,0,1,1,1,1,1,0,0,0,0,0,0,0,
+ 1,42,53,4,0,0,0,0,69,114,29,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,109,124,77,109,68,42,71,71,29,53,88,68,7,
+ 7,69,88,53,42,109,122,88,42,29,68,88,42,29,68,71,
+ 29,13,53,109,124,53,0,0,7,68,88,42,13,42,71,88,
+ 29,13,71,123,122,4,1,42,96,123,88,29,68,88,42,29,
+ 42,42,53,68,68,53,68,71,68,42,42,29,53,53,68,68,
+ 68,68,29,7,53,68,42,53,114,123,53,42,71,42,0,0,
+ 0,0,0,0,
+ 0,0,0,88,120,96,120,114,114,120,120,96,120,124,96,7,
+ 22,109,122,120,71,108,122,122,122,108,114,122,108,71,96,123,
+ 114,108,96,96,122,71,29,19,53,108,123,114,88,122,122,122,
+ 114,114,88,108,114,7,53,114,71,108,114,108,71,96,122,122,
+ 122,114,120,114,114,114,122,114,122,108,122,114,122,122,114,108,
+ 108,122,109,88,122,122,114,96,96,114,88,114,122,71,0,0,
+ 0,0,0,0,
+ 0,0,0,88,120,71,120,124,127,114,88,88,120,124,120,29,
+ 53,120,120,124,96,108,108,108,123,127,123,108,88,108,114,122,
+ 123,124,96,68,114,88,71,68,88,114,123,122,88,114,108,114,
+ 127,127,68,88,114,19,96,122,42,96,123,127,42,42,123,108,
+ 122,123,124,120,71,88,114,88,114,88,108,123,127,123,108,96,
+ 108,124,120,88,114,88,122,96,68,114,71,108,123,96,7,0,
+ 0,0,0,0,
+ 0,0,1,109,122,88,120,114,122,122,71,71,108,120,120,42,
+ 68,120,109,120,108,114,114,108,122,114,122,96,71,114,122,114,
+ 122,114,114,109,120,71,1,4,96,123,114,114,96,114,108,114,
+ 122,122,108,114,114,19,68,122,96,114,114,122,96,71,96,29,
+ 88,123,122,120,29,71,122,96,114,53,53,120,122,122,71,96,
+ 114,120,120,96,108,68,96,114,96,114,88,96,114,114,71,42,
+ 0,0,0,0,
+ 0,0,4,71,88,71,71,53,53,88,71,42,71,96,53,4,
+ 7,71,88,71,68,88,71,71,71,42,71,88,42,42,88,88,
+ 68,42,71,77,71,29,0,0,29,88,71,68,68,71,71,71,
+ 68,42,71,71,68,13,0,68,88,68,53,42,88,88,19,0,
+ 19,53,42,42,7,42,88,71,71,29,7,53,42,53,19,29,
+ 88,88,71,68,71,29,19,71,88,68,53,71,71,42,53,42,
+ 0,0,0,0,
+ 0,0,1,1,1,1,1,1,1,1,4,1,1,1,0,0,
+ 1,1,1,1,1,1,1,1,1,1,1,4,1,0,1,0,
+ 0,0,0,1,1,0,0,0,0,0,1,1,1,1,1,1,
+ 1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,1,1,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,1,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,1,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,4,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,1,1,0,0,0,0,
+ 0,0,0,0,0,0,0,0,1,1,1,1,0,0,0,0,
+ 0,0,0,0,1,4,13,13,4,0,0,1,1,1,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,1,1,29,68,68,68,71,69,53,
+ 42,29,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,1,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,4,22,26,11,4,4,1,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,4,29,71,88,96,108,114,123,114,
+ 88,53,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,1,1,0,0,0,0,0,0,
+ 0,1,4,29,34,39,11,0,0,7,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,4,19,29,53,71,96,108,114,122,123,114,
+ 96,71,4,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1,
+ 0,0,0,0,0,0,0,0,1,1,0,0,0,0,0,0,
+ 1,13,34,11,11,37,11,0,0,7,1,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,4,29,29,42,68,71,96,88,88,114,123,123,
+ 108,68,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,1,1,0,0,0,1,1,13,
+ 34,37,37,37,37,37,37,0,0,7,1,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,4,4,29,53,68,71,68,53,88,108,108,
+ 71,13,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,4,8,4,0,0,4,8,4,4,4,
+ 7,7,4,7,7,7,7,4,4,8,7,4,4,7,22,37,
+ 58,37,58,58,37,37,11,0,0,19,22,7,4,1,1,4,
+ 4,0,0,0,0,4,4,7,7,7,4,7,8,13,19,7,
+ 4,0,0,0,0,0,4,13,29,29,68,108,96,68,88,88,
+ 53,13,0,0,0,0,4,0,4,42,42,19,7,7,4,4,
+ 7,0,0,0,
+ 0,0,0,0,0,29,71,96,71,19,7,68,96,71,68,77,
+ 96,109,88,88,77,68,68,88,88,71,68,29,7,34,37,37,
+ 51,58,51,51,62,62,39,0,1,42,69,69,53,53,77,69,
+ 4,0,0,4,42,68,96,96,96,88,88,88,108,108,114,108,
+ 96,42,19,53,42,8,4,1,7,19,71,122,122,96,114,123,
+ 114,53,1,0,1,29,68,42,71,114,122,114,96,88,53,42,
+ 42,0,0,0,
+ 0,0,0,0,4,68,108,123,114,88,88,114,123,120,109,120,
+ 124,124,124,124,109,96,96,122,122,114,96,29,13,37,37,37,
+ 37,37,62,81,92,92,54,0,1,69,100,76,89,119,119,109,
+ 29,0,1,42,96,108,114,122,108,88,96,108,122,122,108,122,
+ 122,88,88,114,114,88,29,1,4,42,96,122,122,122,122,123,
+ 122,71,0,0,8,71,88,108,122,122,123,123,122,108,42,4,
+ 4,0,0,0,
+ 0,0,0,0,4,71,114,124,124,122,123,122,122,120,109,120,
+ 124,124,124,109,77,96,114,122,114,114,96,29,13,12,12,58,
+ 62,62,92,107,107,92,64,0,1,89,119,106,119,125,119,109,
+ 89,29,1,29,88,114,96,114,114,88,88,96,114,122,96,114,
+ 114,96,122,123,122,114,88,17,4,42,108,122,122,123,123,122,
+ 114,88,4,1,29,42,68,96,96,96,108,108,114,96,13,0,
+ 0,0,0,0,
+ 0,0,0,0,4,53,96,108,114,122,122,114,108,96,109,120,
+ 120,114,108,88,88,96,114,114,108,96,68,8,11,39,62,81,
+ 92,92,107,107,107,92,64,0,1,89,125,125,125,119,106,100,
+ 100,53,1,7,88,108,88,96,114,114,96,88,114,123,122,114,
+ 108,114,122,123,122,114,96,42,8,42,108,123,122,114,123,123,
+ 108,71,8,8,29,42,71,71,42,53,96,88,71,53,1,0,
+ 0,0,0,0,
+ 0,0,0,1,4,42,88,96,114,114,114,108,114,108,109,120,
+ 120,114,114,114,122,114,122,114,96,88,69,40,64,92,92,92,
+ 92,92,92,107,107,92,64,4,4,80,125,125,125,119,119,106,
+ 100,69,0,4,68,88,96,114,122,122,96,96,114,123,127,122,
+ 114,122,123,122,114,96,88,42,4,29,96,114,96,68,96,96,
+ 88,68,4,4,68,114,122,114,96,108,114,114,68,8,0,0,
+ 0,0,0,0,
+ 0,0,0,1,4,42,88,122,123,123,123,122,123,124,124,124,
+ 124,123,123,123,123,123,123,122,114,96,80,92,107,107,107,92,
+ 92,92,92,92,81,64,39,4,13,106,125,125,125,125,119,100,
+ 109,89,7,4,29,53,71,108,122,114,108,108,114,123,123,114,
+ 122,123,123,122,114,96,88,68,4,13,71,96,88,71,88,88,
+ 88,68,4,4,71,114,114,88,88,114,114,114,71,1,0,0,
+ 0,0,0,0,
+ 0,0,0,0,7,68,88,114,120,122,123,123,127,127,124,124,
+ 122,114,114,123,123,122,122,114,108,96,80,92,92,92,92,92,
+ 92,92,92,62,37,37,11,4,39,125,125,125,125,125,119,106,
+ 106,89,29,4,7,29,68,108,114,114,114,108,108,122,114,108,
+ 122,123,114,108,114,114,108,88,29,29,71,114,122,122,123,114,
+ 108,71,8,4,68,96,96,71,71,88,96,88,19,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,4,53,71,108,108,96,96,108,114,109,96,88,
+ 71,71,96,108,88,88,88,88,88,71,60,92,92,64,64,81,
+ 62,37,51,58,37,37,37,11,39,125,125,125,125,125,100,106,
+ 100,77,29,8,42,71,96,122,114,114,114,88,71,88,88,88,
+ 108,108,108,114,122,122,114,96,29,68,96,122,122,122,123,122,
+ 108,68,4,29,71,108,114,122,114,88,71,42,1,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,7,42,68,53,53,53,42,53,42,7,22,
+ 69,96,108,96,53,68,88,88,71,71,53,40,54,39,11,51,
+ 51,37,51,51,37,58,37,11,40,119,125,119,125,125,106,106,
+ 100,88,42,42,71,96,114,123,123,123,120,88,13,13,29,42,
+ 71,108,122,123,123,123,122,88,13,68,114,114,114,122,114,122,
+ 114,68,8,68,108,114,123,123,123,114,88,29,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,1,1,1,1,1,0,4,7,7,4,0,0,68,
+ 120,124,122,122,108,88,108,114,96,71,17,1,13,12,37,51,
+ 51,37,58,58,37,58,37,4,40,125,125,119,125,125,125,119,
+ 125,119,89,69,68,88,122,123,127,123,123,108,4,0,8,42,
+ 96,122,123,123,123,123,114,71,4,13,71,88,96,108,108,88,
+ 88,53,19,68,96,96,114,114,108,108,88,4,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,1,1,1,1,0,0,0,1,0,0,4,29,88,
+ 114,114,114,114,108,88,71,71,68,42,1,1,34,37,37,58,
+ 51,58,51,58,37,37,11,0,22,125,125,119,125,125,125,125,
+ 125,125,106,76,69,88,114,122,122,122,122,88,0,0,13,42,
+ 96,114,114,122,122,114,88,42,4,4,29,71,88,96,88,53,
+ 68,71,71,88,96,96,108,108,108,88,22,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,1,1,1,1,1,1,1,1,0,7,69,96,109,
+ 114,120,109,108,88,71,71,29,4,4,0,4,39,58,37,51,
+ 51,58,58,37,39,62,39,11,40,119,125,125,125,125,125,125,
+ 125,106,60,22,42,71,103,108,108,108,108,68,0,0,4,53,
+ 96,114,114,114,122,108,68,4,1,17,71,114,114,114,114,108,
+ 114,123,123,123,123,123,123,114,108,68,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,1,1,1,1,1,1,1,1,7,71,120,124,124,
+ 124,124,127,124,108,96,88,29,13,13,4,7,39,58,37,58,
+ 51,37,62,62,64,81,64,64,64,121,125,125,125,125,125,119,
+ 106,89,22,1,42,80,106,108,114,114,122,96,7,8,68,108,
+ 122,122,122,123,123,114,53,1,1,29,96,114,114,123,127,123,
+ 123,123,123,123,127,124,123,122,71,7,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,1,1,1,1,1,0,1,68,108,114,124,124,
+ 124,123,123,122,122,108,68,29,29,13,4,4,39,58,37,51,
+ 51,37,62,64,64,64,64,64,81,119,125,125,119,125,119,89,
+ 89,69,4,8,69,92,92,109,123,127,123,96,29,68,114,123,
+ 123,123,123,123,123,108,42,1,8,29,88,96,88,114,123,123,
+ 123,127,123,122,123,122,122,114,88,29,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,1,1,1,1,1,1,0,4,69,96,108,114,114,
+ 109,96,88,88,88,68,29,13,8,13,4,4,34,58,37,51,
+ 58,37,64,64,64,64,81,92,64,119,125,125,119,125,119,106,
+ 100,68,8,14,41,92,64,89,122,122,108,53,29,68,96,122,
+ 123,123,123,114,108,69,1,0,4,29,71,88,71,88,108,108,
+ 96,96,88,71,71,88,88,88,71,68,7,0,0,0,0,0,
+ 0,0,0,0,
+ 0,1,1,1,1,1,1,1,19,19,7,68,108,122,114,108,
+ 96,88,71,53,8,13,17,8,13,29,1,1,34,58,37,51,
+ 58,39,64,64,81,64,92,92,64,119,125,125,125,125,125,119,
+ 119,100,41,40,64,92,64,80,114,114,88,53,8,42,96,114,
+ 122,122,114,108,96,69,29,1,1,29,88,96,108,108,96,71,
+ 42,17,17,42,68,88,88,68,88,114,71,0,0,0,0,0,
+ 0,0,0,0,
+ 0,1,1,1,1,1,1,7,71,71,68,96,122,124,124,124,
+ 122,108,71,53,29,29,42,29,13,4,0,1,39,58,37,58,
+ 51,37,54,92,64,81,92,64,54,119,125,125,125,125,125,125,
+ 125,95,64,64,107,92,64,89,122,124,124,108,13,29,96,108,
+ 96,108,108,114,114,122,96,42,4,29,88,122,123,123,114,96,
+ 88,53,29,53,108,122,122,108,114,122,96,4,0,0,0,0,
+ 0,0,0,0,
+ 0,0,1,1,1,1,4,53,96,114,123,122,123,124,124,120,
+ 109,88,53,53,42,42,53,29,1,0,0,4,59,58,37,58,
+ 58,39,64,92,81,64,64,54,40,106,125,125,125,119,106,120,
+ 106,92,64,64,81,92,64,96,123,124,126,122,17,4,53,71,
+ 88,108,122,123,123,123,122,88,1,1,71,122,123,123,96,80,
+ 95,95,64,54,100,123,123,122,122,122,108,42,0,0,0,0,
+ 0,0,0,0,
+ 0,0,1,1,1,4,42,71,108,122,122,122,114,114,114,96,
+ 53,8,13,29,22,29,29,29,4,0,1,4,59,58,37,37,
+ 37,64,92,92,81,64,11,11,40,119,125,125,125,125,106,89,
+ 89,81,64,11,7,60,76,96,114,114,114,88,4,0,4,29,
+ 88,114,123,127,127,123,114,88,1,4,71,96,114,122,88,54,
+ 64,92,64,80,89,114,123,123,123,122,108,71,7,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,4,42,68,71,96,108,96,108,108,108,96,71,
+ 29,22,53,53,42,42,42,53,53,42,8,4,39,37,11,37,
+ 62,92,92,92,64,39,11,0,22,125,125,125,125,125,100,89,
+ 88,71,29,4,17,71,108,114,96,108,114,71,0,0,0,29,
+ 88,96,114,122,114,96,108,71,13,42,71,96,108,96,71,68,
+ 68,19,0,42,71,96,96,96,108,96,68,53,19,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,29,88,96,114,108,88,96,114,122,114,108,96,
+ 88,96,108,96,96,108,88,71,88,88,69,64,64,62,62,37,
+ 37,62,92,92,62,37,11,0,26,119,125,125,125,125,106,77,
+ 88,71,17,29,71,108,123,123,114,122,123,108,4,0,4,29,
+ 68,96,114,114,88,88,114,88,29,42,88,122,114,71,88,108,
+ 96,42,0,4,68,53,53,71,71,53,42,68,29,1,0,0,
+ 0,0,0,0,
+ 0,0,0,4,53,108,123,123,122,96,109,122,122,114,114,108,
+ 96,114,108,114,123,122,96,88,108,114,89,92,92,92,92,81,
+ 62,62,62,39,37,51,37,4,26,119,125,125,125,125,119,77,
+ 71,68,4,19,88,122,127,123,122,122,123,114,7,0,29,42,
+ 68,108,123,122,96,114,123,108,29,8,71,123,123,108,114,123,
+ 114,71,4,7,42,71,108,114,108,96,88,96,71,29,1,0,
+ 0,0,0,0,
+ 0,0,1,4,53,96,123,123,108,96,122,123,123,123,122,109,
+ 88,108,122,122,123,123,96,53,96,122,106,92,92,107,107,92,
+ 92,92,81,62,62,58,37,11,26,119,125,119,119,125,125,120,
+ 71,29,1,4,53,96,114,114,114,114,114,88,4,1,29,42,
+ 71,108,123,123,114,123,127,114,29,13,71,108,114,114,114,123,
+ 122,88,7,19,53,108,122,123,122,122,114,114,108,68,0,0,
+ 0,0,0,0,
+ 0,1,1,4,42,88,114,114,96,88,114,122,114,108,108,88,
+ 53,88,108,108,96,108,96,42,42,96,89,64,92,92,117,107,
+ 92,92,92,92,92,81,39,4,22,106,125,119,119,120,119,125,
+ 100,41,1,1,29,88,96,96,108,114,114,88,4,4,13,42,
+ 53,71,108,108,108,108,114,88,13,29,88,96,96,88,96,96,
+ 71,42,1,7,42,71,96,122,114,108,114,122,108,53,0,0,
+ 0,0,0,0,
+ 0,0,1,1,29,88,88,96,96,96,108,88,71,88,108,96,
+ 88,88,88,71,68,88,96,88,68,88,71,42,40,62,64,92,
+ 92,92,92,107,107,92,54,4,4,89,119,125,119,109,109,119,
+ 119,60,7,13,53,88,108,114,123,123,123,108,29,4,42,68,
+ 53,71,88,96,108,96,96,71,13,29,71,108,114,108,108,96,
+ 71,13,1,7,29,42,71,108,108,71,88,108,88,29,4,0,
+ 0,0,0,0,
+ 0,0,0,0,7,71,96,108,114,114,114,96,88,122,123,124,
+ 124,122,108,88,71,96,123,123,120,114,108,71,42,11,11,39,
+ 64,92,92,107,107,92,62,11,13,76,100,119,119,109,88,100,
+ 89,26,22,53,88,114,123,123,123,123,114,88,29,4,53,96,
+ 88,108,122,122,122,122,114,88,29,8,68,114,123,122,122,122,
+ 114,53,1,7,68,68,71,96,108,96,88,88,71,53,29,0,
+ 0,0,0,0,
+ 0,0,0,0,1,29,88,108,122,123,122,88,88,114,123,124,
+ 124,120,96,71,42,71,114,123,124,123,122,88,42,11,11,37,
+ 11,39,81,92,92,92,64,11,4,76,100,119,119,100,96,84,
+ 13,1,4,42,108,123,127,123,122,114,108,88,13,1,8,68,
+ 108,114,123,123,122,122,114,88,8,4,68,96,108,114,114,96,
+ 88,53,1,4,53,88,88,108,108,108,114,122,108,88,53,0,
+ 0,0,0,0,
+ 0,0,0,0,1,1,29,71,88,114,96,42,29,53,71,88,
+ 88,71,42,4,7,42,68,88,96,96,88,42,4,13,11,37,
+ 37,37,37,62,81,92,62,11,22,77,69,89,106,76,42,29,
+ 4,8,8,8,68,96,96,96,71,42,53,53,4,0,0,8,
+ 53,68,88,108,108,96,88,68,4,8,71,96,88,88,88,68,
+ 68,53,0,0,13,68,96,96,68,42,71,108,114,108,68,0,
+ 0,0,0,0,
+ 0,0,0,0,1,1,0,7,22,22,7,1,1,0,4,4,
+ 4,4,1,0,0,1,4,4,4,4,0,0,0,1,4,11,
+ 11,37,51,37,37,37,11,11,26,68,8,19,41,7,4,8,
+ 17,13,13,13,42,42,17,29,13,4,4,4,0,0,0,0,
+ 1,1,4,17,29,29,29,8,1,4,29,42,29,29,29,13,
+ 29,13,0,0,1,13,42,13,1,1,4,29,42,42,19,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,11,37,37,58,51,37,11,26,71,42,17,19,29,53,71,
+ 71,68,71,88,88,68,42,42,53,53,53,42,4,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,4,11,37,57,37,0,22,96,96,71,53,68,96,108,
+ 108,108,108,108,108,108,96,68,68,88,88,88,68,29,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,1,8,34,37,39,41,96,123,123,114,88,88,108,114,
+ 122,122,108,122,122,114,108,88,53,88,108,114,114,96,29,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,29,68,88,108,123,127,127,127,123,114,114,123,127,
+ 127,127,123,127,127,127,123,114,108,122,127,127,127,108,53,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,29,96,122,127,127,127,127,123,123,114,122,127,127,
+ 127,127,127,127,127,127,127,122,122,127,127,127,127,122,53,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,4,42,88,114,122,123,122,108,96,71,88,114,123,
+ 123,123,123,123,123,122,114,96,88,96,122,123,123,114,29,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,1,42,88,108,122,123,122,122,108,88,96,108,108,
+ 96,71,96,122,114,108,108,96,88,108,114,122,114,88,4,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,42,96,114,123,127,127,127,127,127,123,123,122,123,
+ 114,71,108,123,123,123,123,122,123,127,127,122,122,96,7,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,68,122,123,127,127,127,127,127,127,127,122,123,127,
+ 127,114,123,127,127,127,127,127,127,127,127,123,127,123,42,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,29,114,127,127,127,127,127,127,123,122,123,127,127,
+ 123,114,114,127,127,127,127,127,127,127,127,127,127,122,29,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,4,68,96,114,122,122,114,114,108,108,122,114,96,
+ 88,71,88,114,114,122,123,123,123,123,123,123,114,68,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,4,7,8,29,29,19,13,42,53,88,108,108,
+ 108,108,108,108,108,71,42,29,42,53,53,53,29,4,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,8,53,108,123,127,
+ 127,127,127,127,123,71,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,1,8,68,122,127,127,
+ 127,127,123,127,127,88,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,1,8,71,123,127,127,
+ 127,127,127,127,123,53,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,1,13,68,108,123,127,
+ 127,127,127,127,96,1,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,4,29,71,114,123,
+ 122,122,123,122,88,8,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,29,108,123,123,
+ 122,114,96,122,122,53,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,42,122,127,127,
+ 127,122,108,127,127,68,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,1,42,123,127,127,
+ 127,123,123,127,123,68,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,1,0,0,4,42,123,127,127,
+ 127,123,127,127,123,53,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,7,29,29,17,8,4,4,4,19,88,122,122,
+ 108,108,123,123,108,29,1,4,8,29,29,1,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,1,68,71,71,53,42,29,4,1,19,53,88,96,
+ 88,88,114,122,88,13,4,17,53,71,71,29,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,1,42,68,68,68,42,8,4,4,42,88,108,122,
+ 122,122,123,123,108,42,8,8,42,71,71,53,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,4,29,68,71,71,53,42,53,42,68,122,127,127,
+ 127,127,127,127,123,71,13,13,68,71,71,71,19,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,1,42,88,108,108,108,88,88,108,88,68,114,127,127,
+ 127,127,127,127,123,88,42,71,108,108,108,108,71,1,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,1,53,96,122,122,114,88,68,108,108,53,71,96,108,
+ 114,114,114,108,108,71,71,108,114,122,122,122,108,29,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,1,19,53,96,114,108,71,53,88,108,96,88,88,88,
+ 96,96,71,42,71,88,108,114,114,122,114,114,114,71,1,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,42,71,96,122,123,114,96,108,122,123,127,123,123,
+ 123,122,71,53,108,123,123,123,123,123,122,114,122,114,13,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,1,68,122,123,127,127,127,114,123,127,127,127,127,127,
+ 127,127,122,114,123,127,127,127,127,127,127,123,127,123,42,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,1,42,114,127,127,127,114,96,122,123,127,127,127,127,
+ 127,127,123,114,127,127,127,127,127,127,127,127,127,123,29,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,1,1,19,88,122,114,108,68,68,88,96,114,123,127,127,
+ 123,123,96,68,108,114,108,122,123,127,123,127,127,96,7,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,1,29,96,114,120,108,88,96,108,96,96,108,122,122,
+ 108,108,114,114,114,88,96,122,122,122,114,114,108,71,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,42,122,127,127,127,127,127,127,123,122,122,123,122,
+ 108,114,127,127,127,123,123,127,123,123,123,114,122,96,7,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,19,108,127,127,127,127,127,127,127,127,127,127,123,
+ 123,123,123,127,127,127,127,127,127,127,127,127,127,123,53,19,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 4,53,42,42,88,127,127,114,123,127,123,122,127,127,127,122,
+ 96,122,114,114,122,127,127,127,127,127,127,127,127,123,68,29,
+ 7,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 7,68,88,108,96,88,68,29,42,71,68,68,96,108,96,53,
+ 17,42,68,42,68,114,122,114,96,88,96,88,71,88,71,53,
+ 19,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,1,13,42,29,1,0,0,0,0,1,1,4,4,1,1,
+ 0,0,1,1,1,29,42,13,1,1,1,1,1,4,22,22,
+ 4,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 4,68,29,0,0,0,4,53,53,68,42,42,68,68,53,29,
+ 29,68,42,29,53,7,0,29,68,8,13,29,29,42,19,29,
+ 53,22,7,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 19,108,96,29,71,68,29,108,96,114,108,68,108,88,114,88,
+ 71,122,96,88,108,53,42,68,88,96,96,88,88,108,88,71,
+ 53,7,4,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,4,
+ 42,88,114,71,114,114,29,108,71,69,88,42,88,68,108,71,
+ 68,123,108,71,108,68,71,96,68,88,114,88,108,108,96,108,
+ 68,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 19,22,42,29,29,29,4,42,19,4,7,7,29,17,29,22,
+ 53,71,42,19,42,29,22,53,17,13,53,42,29,29,29,53,
+ 42,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 29,53,42,68,42,42,53,42,29,29,42,8,8,42,29,53,
+ 96,68,29,29,29,53,42,29,53,71,53,29,42,53,29,29,
+ 42,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,4,
+ 96,96,108,114,88,88,114,96,108,108,96,42,71,114,96,114,
+ 114,88,96,71,96,108,88,88,108,114,96,88,114,114,68,96,
+ 88,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,4,
+ 109,77,53,68,42,53,68,68,68,71,68,19,53,71,68,88,
+ 53,42,42,19,68,71,53,53,71,68,53,68,88,71,22,71,
+ 68,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 42,22,0,0,1,4,0,0,0,0,4,0,0,0,0,0,
+ 0,0,0,0,0,4,0,0,0,0,0,4,4,0,0,4,
+ 4,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,
+ 66,66,42,33,33,33,33,33,21,21,33,33,21,33,23,21,
+ 21,21,23,23,23,23,23,23,23,23,21,21,23,21,21,21,
+ 21,21,21,21,21,21,36,36,36,36,36,36,36,25,21,21,
+ 21,21,21,33,21,21,21,21,21,21,21,21,21,21,21,21,
+ 21,21,21,21,21,21,33,21,21,21,21,21,21,21,21,25,
+ 36,36,21,36,21,21,21,21,21,21,21,21,21,36,36,33,
+ 36,43,66,66,
+ 68,68,33,18,18,18,9,18,18,18,18,18,9,18,18,18,
+ 18,18,9,9,18,9,9,9,9,9,18,9,9,18,18,9,
+ 9,9,9,9,9,15,15,15,28,28,28,28,28,15,15,15,
+ 6,6,9,18,9,6,6,9,9,15,6,6,9,9,6,6,
+ 6,25,20,15,6,15,20,15,20,6,6,6,15,15,15,15,
+ 15,20,20,20,20,15,15,15,15,15,15,15,20,20,20,28,
+ 28,33,69,69,
+ 53,42,33,23,23,18,23,18,18,18,18,18,18,18,18,18,
+ 23,18,18,18,23,18,18,18,18,18,18,18,18,18,18,18,
+ 18,18,18,18,18,15,25,15,20,28,28,28,28,20,15,15,
+ 15,18,9,9,9,9,18,18,15,18,9,9,9,9,9,18,
+ 6,15,20,15,15,15,15,15,20,15,15,15,15,15,20,20,
+ 20,28,20,20,28,20,25,25,25,20,28,20,20,20,25,28,
+ 28,33,68,68,
+ 53,42,33,23,23,18,33,18,18,18,23,18,18,18,18,23,
+ 23,18,18,23,23,18,18,18,18,18,18,18,9,18,18,18,
+ 18,18,18,18,18,15,18,25,25,25,28,25,20,15,18,9,
+ 18,15,9,9,9,9,18,9,9,18,18,9,18,18,9,18,
+ 15,9,20,15,15,20,15,15,20,15,15,15,20,15,15,25,
+ 28,28,36,28,25,25,25,25,25,20,36,25,25,25,25,48,
+ 28,33,68,68,
+ 53,42,33,23,23,18,23,18,23,23,23,23,23,23,23,23,
+ 23,18,18,23,18,18,18,18,18,18,18,18,18,18,18,18,
+ 9,18,18,18,18,18,18,15,25,25,28,25,15,15,15,18,
+ 23,18,9,9,18,9,9,9,18,18,18,21,18,9,9,9,
+ 15,15,20,15,20,21,15,15,15,15,25,15,36,20,18,25,
+ 20,28,48,28,28,25,25,25,25,25,25,25,25,25,36,36,
+ 25,33,69,69,
+ 65,32,29,23,23,18,18,23,23,23,23,23,23,23,23,23,
+ 18,18,18,18,18,23,18,18,18,18,18,18,23,18,23,18,
+ 18,9,18,18,18,25,18,15,15,18,15,18,15,15,15,18,
+ 9,9,9,9,18,9,9,9,9,18,18,21,9,9,9,9,
+ 15,21,9,15,20,21,15,15,15,6,20,15,15,20,15,20,
+ 20,36,48,28,20,20,25,25,25,25,25,25,20,25,36,28,
+ 36,33,66,66,
+ 53,42,33,23,23,18,23,18,23,23,23,23,23,23,23,23,
+ 18,18,18,18,18,23,18,18,18,18,18,18,18,18,18,18,
+ 18,9,18,9,18,25,9,18,15,18,18,18,15,6,18,9,
+ 9,9,18,9,9,9,18,18,9,18,18,18,9,9,9,18,
+ 25,18,18,15,15,15,6,6,15,6,6,15,15,15,15,20,
+ 20,28,28,28,20,20,25,25,25,25,25,25,25,36,28,28,
+ 25,33,69,69,
+ 53,42,33,23,23,23,23,23,23,23,23,23,23,23,23,23,
+ 18,23,18,18,23,18,18,18,18,18,18,18,18,18,18,18,
+ 18,18,18,18,9,18,18,18,9,9,9,9,9,9,18,9,
+ 9,9,18,18,18,18,9,18,18,18,18,18,9,9,9,15,
+ 36,15,18,15,15,15,15,6,15,6,6,15,15,6,15,20,
+ 20,20,28,20,20,15,25,25,25,25,25,25,20,25,28,28,
+ 28,33,68,68,
+ 63,42,33,23,18,23,23,25,23,23,23,18,23,23,23,23,
+ 23,23,18,23,23,18,18,18,18,18,23,18,18,18,18,18,
+ 18,18,18,18,9,9,18,18,18,9,9,6,9,9,9,9,
+ 18,18,9,9,18,18,18,18,23,18,18,9,9,9,9,15,
+ 18,15,15,6,15,15,15,6,15,6,18,9,15,15,15,15,
+ 20,20,28,20,20,20,20,25,25,25,36,28,28,28,28,28,
+ 28,36,68,68,
+ 53,42,33,23,25,23,25,23,23,23,23,23,23,23,23,23,
+ 23,23,18,18,18,18,18,18,18,18,23,18,25,18,18,18,
+ 18,18,18,18,9,18,18,18,18,9,9,18,9,9,9,9,
+ 18,9,9,9,18,18,36,18,18,23,18,18,9,9,18,15,
+ 6,9,15,15,6,15,15,6,9,9,9,9,15,25,15,15,
+ 20,20,20,28,28,20,25,25,20,25,36,28,28,28,28,28,
+ 28,33,69,69,
+ 65,32,29,25,25,23,23,23,18,18,23,23,23,23,23,23,
+ 18,18,23,18,18,18,18,18,23,25,25,18,25,18,15,23,
+ 18,18,18,18,9,18,18,9,18,18,18,18,9,9,9,9,
+ 9,9,18,9,18,15,23,18,18,18,18,18,9,18,15,15,
+ 15,15,15,15,15,15,6,25,9,9,6,6,15,18,18,25,
+ 15,25,25,15,28,20,25,36,25,25,25,36,28,28,28,48,
+ 28,33,66,66,
+ 53,42,33,25,25,23,23,23,18,18,18,23,23,23,23,23,
+ 18,18,23,23,23,23,23,23,23,25,25,25,25,25,25,25,
+ 25,18,18,18,18,18,9,9,18,18,18,18,9,18,9,9,
+ 9,9,18,9,18,15,15,15,18,18,18,25,20,18,15,15,
+ 15,15,15,15,15,15,9,36,18,9,6,6,9,18,20,15,
+ 15,28,20,25,15,15,15,79,58,28,36,36,36,36,36,48,
+ 36,36,69,69,
+ 53,42,33,25,25,23,23,18,18,18,18,18,23,23,23,23,
+ 23,23,23,23,18,9,18,6,23,25,25,25,25,25,25,25,
+ 25,18,18,18,18,9,9,9,9,9,9,9,9,9,9,9,
+ 15,15,18,18,18,18,15,15,18,18,18,25,15,18,15,18,
+ 15,15,15,15,15,15,15,15,6,15,9,6,18,15,20,15,
+ 25,25,15,48,20,20,15,107,86,28,36,36,36,25,25,36,
+ 36,36,68,68,
+ 53,42,33,25,25,23,23,18,18,18,18,18,23,23,23,23,
+ 23,6,6,18,18,23,23,33,23,25,25,25,25,25,25,25,
+ 25,18,18,18,18,18,9,9,9,9,9,9,9,9,9,15,
+ 15,15,18,25,18,18,20,15,18,18,15,15,9,15,18,18,
+ 15,15,15,15,15,15,5,20,21,6,18,15,15,6,15,15,
+ 15,6,15,25,28,20,36,117,75,28,36,25,20,28,28,28,
+ 28,36,68,68,
+ 53,42,33,25,23,23,23,23,18,18,18,18,18,23,23,23,
+ 23,33,43,63,80,95,85,92,28,25,25,25,48,48,28,25,
+ 25,18,18,18,18,18,9,9,9,9,9,9,15,9,9,15,
+ 15,15,15,15,18,20,25,21,15,15,15,15,9,15,15,15,
+ 15,15,20,28,20,6,43,92,92,59,6,36,6,6,6,15,
+ 6,34,62,15,15,6,34,117,45,43,85,58,25,28,28,36,
+ 36,36,69,69,
+ 65,32,35,25,23,23,25,23,18,18,18,18,18,18,18,18,
+ 33,113,107,107,107,102,107,117,24,23,25,25,15,5,15,25,
+ 25,18,18,18,9,9,9,9,9,9,9,9,15,15,18,18,
+ 15,15,15,15,18,21,36,21,15,15,15,15,15,15,15,15,
+ 20,20,28,48,28,43,107,102,102,117,38,15,15,6,6,15,
+ 6,85,102,10,27,59,85,117,45,107,102,117,58,28,28,36,
+ 28,33,65,65,
+ 65,42,29,25,23,23,23,21,18,18,18,18,18,18,23,18,
+ 33,92,91,117,91,38,102,102,24,6,15,15,43,43,25,18,
+ 25,23,18,18,18,9,9,9,6,9,18,9,18,15,18,18,
+ 15,15,15,15,15,21,36,18,18,18,15,15,6,15,18,15,
+ 20,20,28,28,15,107,102,45,75,117,49,5,15,6,15,36,
+ 6,62,86,38,107,102,117,102,86,117,86,117,49,28,28,36,
+ 28,33,69,69,
+ 53,42,33,23,23,23,23,33,18,23,18,18,18,18,23,23,
+ 23,23,58,117,70,10,107,91,51,43,15,59,92,107,85,25,
+ 18,18,18,18,18,9,9,9,6,9,18,18,18,15,20,20,
+ 15,15,6,15,15,18,20,15,18,18,9,15,18,9,9,15,
+ 15,15,20,28,58,117,91,75,102,102,45,21,39,6,58,91,
+ 6,107,75,91,102,45,117,86,102,117,91,86,49,28,20,36,
+ 20,33,69,69,
+ 53,42,33,23,23,23,23,23,18,18,18,18,18,18,18,23,
+ 18,6,80,117,45,36,117,102,107,107,38,107,102,107,102,10,
+ 18,18,18,18,18,9,9,9,6,6,9,9,15,15,5,6,
+ 6,6,6,6,15,15,15,15,18,18,18,34,85,6,15,18,
+ 15,15,36,91,107,117,102,102,102,75,24,62,107,10,107,102,
+ 10,117,57,117,86,24,117,86,107,117,102,102,102,38,25,25,
+ 28,36,68,68,
+ 53,42,33,23,23,23,23,18,18,18,18,18,18,18,18,23,
+ 18,18,92,117,24,60,117,86,102,117,46,117,102,102,86,24,
+ 18,18,18,9,9,9,9,9,6,6,9,9,6,6,34,18,
+ 6,6,6,9,6,6,6,5,9,9,5,92,107,24,59,5,
+ 15,15,27,102,102,117,86,75,102,91,10,107,102,10,117,86,
+ 31,117,57,117,102,91,117,102,102,86,86,86,57,24,36,20,
+ 48,21,69,69,
+ 65,42,35,18,23,23,18,18,18,18,18,18,18,18,18,18,
+ 18,9,107,102,24,81,117,24,92,107,75,117,102,91,86,51,
+ 15,9,9,9,9,5,6,9,6,6,9,9,6,33,117,38,
+ 6,6,9,6,9,6,9,21,5,15,9,81,75,70,107,28,
+ 6,15,18,38,31,107,102,91,117,102,24,117,86,51,117,86,
+ 91,117,102,102,102,102,75,75,45,24,38,38,10,28,25,20,
+ 28,21,65,65,
+ 65,32,35,18,23,23,18,18,18,18,18,18,18,18,18,18,
+ 21,23,117,86,10,92,107,24,107,107,102,102,102,91,91,46,
+ 10,18,9,9,9,34,34,6,6,9,6,6,6,59,117,24,
+ 5,6,5,5,6,6,33,107,34,62,62,27,24,102,117,86,
+ 20,15,15,5,5,58,102,102,117,107,91,117,102,102,102,102,
+ 102,86,86,45,38,38,28,28,10,10,10,28,15,25,28,28,
+ 20,33,65,65,
+ 53,42,33,23,23,18,18,18,18,18,18,18,18,18,18,18,
+ 18,63,117,70,28,107,86,24,81,91,75,38,31,38,38,38,
+ 43,18,18,9,6,107,86,20,6,6,59,62,5,92,107,24,
+ 18,5,33,62,34,9,59,117,107,107,86,10,5,107,86,117,
+ 82,6,15,6,34,85,102,102,117,107,102,86,91,86,46,46,
+ 38,28,28,10,10,10,28,15,28,25,25,25,28,20,20,25,
+ 20,36,71,71,
+ 53,42,33,23,23,18,18,18,18,18,18,18,18,18,18,23,
+ 18,80,117,45,15,59,45,20,23,28,28,5,5,5,5,85,
+ 92,28,18,6,21,117,75,10,6,6,92,102,10,107,91,38,
+ 92,36,107,102,117,38,92,102,117,86,24,10,9,62,45,117,
+ 86,10,6,34,107,102,91,102,117,49,38,28,28,10,10,10,
+ 10,15,20,15,25,25,25,28,28,20,28,20,25,25,20,28,
+ 28,36,71,71,
+ 63,42,33,23,23,23,18,18,18,18,18,18,18,18,9,18,
+ 18,33,58,24,18,18,15,18,5,6,6,18,18,18,9,107,
+ 102,24,18,9,41,117,45,20,6,6,92,75,10,117,86,102,
+ 102,86,117,86,117,46,107,86,117,57,38,27,9,91,102,102,
+ 61,10,6,107,117,49,24,91,117,24,5,5,5,6,6,25,
+ 25,20,25,28,25,25,28,28,28,20,20,20,25,48,28,28,
+ 28,25,71,71,
+ 69,42,33,23,23,23,18,18,18,18,18,18,18,18,23,18,
+ 18,33,59,15,6,5,18,9,9,18,18,18,9,18,9,117,
+ 75,10,6,9,62,117,86,92,34,6,107,75,38,117,107,117,
+ 45,102,117,91,91,86,117,45,117,102,102,75,6,91,86,46,
+ 24,15,18,117,86,10,5,107,102,24,6,6,25,21,15,20,
+ 20,20,28,28,28,28,28,25,25,25,20,28,25,25,20,20,
+ 36,21,69,69,
+ 52,32,29,23,23,23,18,18,18,18,18,18,18,33,85,20,
+ 9,92,107,10,33,33,18,21,59,5,9,6,9,6,33,117,
+ 86,82,34,6,92,117,102,117,86,36,117,45,58,117,86,117,
+ 75,107,117,102,102,102,102,24,62,91,75,45,15,21,10,20,
+ 15,6,9,117,102,38,62,117,75,10,18,6,25,15,5,20,
+ 20,28,25,28,36,28,25,25,25,25,28,28,36,15,20,20,
+ 20,33,66,66,
+ 53,42,33,25,23,23,23,23,18,18,18,18,23,85,117,24,
+ 9,117,102,24,81,92,10,81,107,38,9,59,81,43,59,117,
+ 102,117,102,10,107,102,45,117,75,58,117,45,92,117,24,81,
+ 107,57,91,86,75,57,24,10,9,20,20,6,9,5,5,107,
+ 58,6,6,81,117,107,107,102,24,5,36,15,6,20,36,43,
+ 36,25,28,28,48,25,25,25,25,25,25,25,25,15,25,15,
+ 20,21,69,69,
+ 63,42,33,25,23,25,25,23,23,18,18,23,18,92,117,24,
+ 18,117,75,10,81,91,46,107,117,75,62,107,107,102,93,117,
+ 46,107,102,24,117,75,38,117,86,102,117,102,102,86,10,9,
+ 62,24,21,38,31,38,15,6,6,5,6,9,9,18,33,117,
+ 49,6,5,6,58,91,86,24,10,27,33,85,6,62,102,38,
+ 36,28,28,28,36,36,48,25,28,25,15,25,15,15,20,20,
+ 20,33,71,71,
+ 68,42,33,23,23,25,23,23,23,23,15,9,5,117,102,24,
+ 59,117,91,58,92,75,91,117,107,75,107,86,51,91,102,102,
+ 24,117,91,82,117,45,59,117,102,91,86,75,46,38,15,5,
+ 6,6,5,62,107,107,92,15,6,9,15,6,9,9,59,117,
+ 24,6,18,6,20,15,86,38,62,102,58,117,10,107,102,24,
+ 25,28,25,28,28,48,25,20,25,18,15,15,25,15,28,20,
+ 25,21,71,71,
+ 69,42,33,25,25,25,25,25,23,5,25,27,34,117,107,91,
+ 107,117,107,86,117,75,58,117,57,38,117,70,36,58,117,86,
+ 38,117,102,102,102,24,18,58,51,38,28,15,5,5,6,6,
+ 6,6,85,107,86,91,117,46,9,5,5,5,6,6,92,107,
+ 24,59,85,91,85,5,107,92,107,86,102,102,24,117,86,10,
+ 25,25,25,28,28,25,25,25,25,25,15,15,25,15,20,20,
+ 20,33,69,69,
+ 65,32,29,25,25,25,25,25,15,43,92,107,107,117,107,102,
+ 117,107,45,51,117,70,58,117,61,62,117,102,92,102,107,45,
+ 20,62,75,51,38,10,25,15,15,6,5,5,6,9,6,5,
+ 5,33,117,91,46,91,117,45,6,21,27,27,34,5,107,102,
+ 70,107,86,117,86,10,59,117,117,24,117,102,51,117,45,10,
+ 28,28,48,28,36,25,25,25,25,15,25,15,20,20,15,15,
+ 20,33,66,66,
+ 69,42,33,25,25,25,28,25,59,107,107,102,102,117,70,38,
+ 107,107,24,81,117,86,91,117,102,107,91,91,91,75,51,24,
+ 9,15,15,5,5,43,92,10,25,15,15,18,18,6,6,18,
+ 34,92,117,102,102,107,86,24,21,92,102,107,102,10,117,75,
+ 107,86,24,117,75,28,85,117,117,31,107,102,107,117,46,33,
+ 28,48,25,25,25,36,25,15,25,15,20,28,20,20,20,25,
+ 20,33,69,69,
+ 68,42,36,28,25,25,36,43,117,102,70,38,91,117,24,5,
+ 117,117,102,107,107,102,86,86,82,51,38,28,20,15,6,15,
+ 18,5,5,6,15,81,117,24,28,20,15,5,5,5,6,34,
+ 107,117,117,102,91,91,75,5,92,102,75,117,86,24,117,49,
+ 117,75,37,117,91,82,117,86,91,86,58,102,107,117,102,82,
+ 28,28,25,25,15,25,25,15,15,20,20,28,20,20,20,20,
+ 20,36,71,71,
+ 68,42,36,48,28,25,36,92,117,45,10,5,107,102,24,18,
+ 81,91,91,70,38,38,28,20,15,5,5,5,5,6,9,9,
+ 18,18,21,25,15,107,102,24,28,15,20,34,34,15,6,33,
+ 82,91,117,86,86,102,91,10,117,86,10,117,75,82,117,86,
+ 117,102,107,102,102,91,86,24,21,86,102,102,107,107,86,46,
+ 28,36,25,15,15,15,36,15,25,25,20,28,20,28,28,36,
+ 25,33,71,71,
+ 69,42,33,28,28,25,25,117,102,24,15,18,117,91,24,18,
+ 23,36,28,5,5,5,5,6,5,9,18,9,18,18,18,18,
+ 18,5,92,51,5,117,86,51,43,20,85,102,107,82,6,6,
+ 6,15,92,102,102,117,102,51,117,91,92,117,102,102,107,102,
+ 86,91,86,38,38,38,20,10,21,117,86,46,117,86,24,20,
+ 20,33,15,15,15,15,25,25,25,25,25,28,28,28,28,36,
+ 20,33,69,69,
+ 65,32,29,28,28,36,28,117,102,24,18,62,117,70,28,18,
+ 5,5,6,9,18,18,18,9,21,18,18,9,6,6,18,18,
+ 18,33,117,102,38,117,102,107,102,38,117,102,117,102,10,6,
+ 5,6,39,102,102,117,107,102,102,107,102,86,91,75,31,38,
+ 28,20,20,5,5,5,5,6,43,117,57,58,117,45,5,9,
+ 15,15,15,15,15,15,18,15,15,25,20,28,48,28,28,25,
+ 25,33,66,66,
+ 69,42,33,25,25,36,25,92,117,91,81,117,102,24,18,23,
+ 23,23,23,23,23,18,18,9,92,28,6,6,33,21,6,9,
+ 33,107,117,102,86,117,86,107,117,57,117,102,102,75,28,6,
+ 20,92,107,102,102,117,86,75,38,58,31,10,20,20,5,5,
+ 5,5,5,9,6,6,15,15,18,107,102,107,91,24,6,15,
+ 15,15,15,15,15,15,15,15,15,25,20,28,28,36,28,36,
+ 36,33,69,69,
+ 68,42,33,25,25,25,25,43,107,107,107,102,45,28,23,33,
+ 14,14,14,21,33,21,23,39,117,57,15,62,92,92,34,9,
+ 33,107,117,46,86,117,24,107,102,86,117,102,91,91,51,5,
+ 92,117,102,57,91,117,24,5,5,5,5,5,5,6,6,9,
+ 9,6,6,15,15,15,15,15,15,36,82,57,24,15,15,15,
+ 15,15,15,15,15,15,15,15,15,15,15,28,20,28,28,48,
+ 36,33,71,71,
+ 68,42,33,25,23,25,25,15,33,58,51,38,10,35,14,8,
+ 2,2,2,2,2,14,60,107,117,102,82,117,91,107,102,10,
+ 18,92,107,24,92,102,24,107,102,102,102,102,91,91,45,20,
+ 117,102,24,5,102,102,24,5,9,9,9,9,9,9,9,9,
+ 15,15,6,15,15,15,15,15,20,6,15,5,5,6,6,15,
+ 6,15,15,15,15,15,15,15,15,25,25,28,28,25,28,28,
+ 25,33,68,68,
+ 68,42,33,25,23,23,25,25,5,15,15,5,35,7,4,2,
+ 2,2,2,2,2,2,64,117,107,70,102,102,24,92,117,24,
+ 9,107,117,86,107,86,10,92,91,86,38,38,38,28,15,33,
+ 117,86,10,27,117,86,10,9,6,9,9,9,9,15,9,9,
+ 9,9,15,15,15,15,15,20,20,20,15,6,9,6,6,6,
+ 6,15,18,15,15,15,15,15,15,25,28,20,28,25,20,20,
+ 25,33,69,69,
+ 65,32,29,25,23,23,23,23,25,18,18,35,14,2,2,2,
+ 2,2,2,2,2,2,4,107,91,24,117,102,38,107,102,24,
+ 9,92,102,91,86,45,20,18,36,20,5,5,5,5,6,9,
+ 107,102,85,107,117,45,15,9,6,9,9,9,18,6,9,9,
+ 9,9,9,15,15,20,20,20,20,20,15,15,15,6,6,6,
+ 6,15,18,15,15,15,15,25,25,25,25,20,20,20,20,25,
+ 25,33,66,66,
+ 69,42,33,23,25,23,23,23,23,18,23,29,2,2,2,2,
+ 2,2,2,2,2,2,2,117,102,91,107,107,107,107,45,28,
+ 18,33,38,38,20,15,9,5,5,6,9,9,9,9,6,6,
+ 39,107,102,102,57,10,9,6,9,9,9,9,21,9,6,6,
+ 6,6,15,15,15,20,28,28,20,15,15,15,6,6,6,6,
+ 15,15,18,15,15,15,25,25,28,28,36,28,20,20,20,25,
+ 20,33,69,69,
+ 68,42,33,23,25,23,23,23,23,23,33,2,2,2,2,2,
+ 2,2,2,2,2,2,7,107,107,102,75,82,82,38,10,18,
+ 18,5,5,5,5,9,9,6,9,9,6,6,9,6,9,6,
+ 5,33,37,38,10,6,9,6,9,9,9,9,9,9,15,6,
+ 15,6,15,15,20,15,36,28,15,15,15,15,6,6,6,6,
+ 6,15,15,15,18,15,25,36,48,48,28,25,20,20,20,20,
+ 20,33,71,71,
+ 68,42,33,23,23,23,23,23,25,23,35,2,2,2,2,2,
+ 2,2,2,2,2,2,2,22,31,43,28,15,6,5,9,18,
+ 18,18,9,9,9,9,9,6,9,6,6,6,18,6,9,9,
+ 6,5,5,5,6,9,9,9,9,6,6,6,6,6,6,6,
+ 6,15,15,15,15,15,36,20,18,15,9,6,6,6,6,6,
+ 6,15,15,15,18,15,28,48,48,48,28,28,28,20,20,20,
+ 15,33,71,71,
+ 68,42,33,23,23,23,23,23,23,23,29,2,2,2,2,2,
+ 2,2,2,2,2,2,2,3,3,16,9,6,6,9,18,9,
+ 9,9,9,9,9,9,6,6,9,9,6,9,6,9,6,9,
+ 9,9,6,9,9,6,6,6,18,18,21,21,21,21,21,18,
+ 15,6,6,6,20,15,15,15,9,15,9,9,6,6,6,6,
+ 9,15,15,15,15,20,20,48,48,48,48,28,36,20,20,20,
+ 15,33,71,71,
+ 65,32,35,25,23,23,23,23,23,23,19,2,2,2,2,2,
+ 2,2,2,2,2,2,2,2,2,14,18,9,9,9,18,9,
+ 9,9,6,9,9,9,9,9,6,9,9,9,6,6,6,6,
+ 9,6,9,9,6,15,18,21,29,32,40,52,52,52,40,41,
+ 42,21,9,6,6,15,15,6,6,9,9,9,6,6,6,6,
+ 6,6,15,15,15,20,20,28,48,48,48,28,28,20,20,20,
+ 15,33,66,66,
+ 65,32,35,25,23,23,23,23,18,25,29,2,2,2,2,2,
+ 2,2,2,2,2,2,2,2,2,14,18,9,9,9,18,18,
+ 9,9,9,9,5,5,5,5,9,9,9,9,6,6,9,9,
+ 9,15,6,15,18,14,30,44,44,54,54,54,54,54,54,54,
+ 54,66,40,27,21,6,6,9,6,9,9,9,6,6,9,6,
+ 9,15,15,15,18,20,20,25,28,48,48,28,20,20,20,20,
+ 15,33,65,65,
+ 68,42,33,23,23,23,23,23,18,23,33,7,2,2,2,2,
+ 2,2,2,2,2,2,2,2,2,35,18,18,18,9,9,18,
+ 9,5,9,6,21,33,27,27,9,6,6,6,9,6,6,6,
+ 9,6,15,21,30,44,44,54,54,54,54,54,54,54,54,54,
+ 54,64,54,54,60,14,6,9,6,9,9,9,9,9,6,6,
+ 6,6,6,6,15,15,15,20,20,28,25,20,20,20,28,20,
+ 15,33,71,71,
+ 68,42,33,23,23,23,23,23,25,18,23,19,2,2,2,2,
+ 2,2,2,2,2,2,2,2,19,23,6,5,6,18,18,6,
+ 9,27,21,59,62,92,107,62,6,6,9,9,9,9,6,6,
+ 9,20,35,30,44,44,44,44,44,54,54,54,54,54,54,54,
+ 54,54,54,54,64,78,29,3,9,6,6,5,5,6,6,9,
+ 20,15,21,6,15,15,15,25,18,20,15,15,20,20,28,20,
+ 15,33,71,71,
+ 63,42,33,23,23,23,25,23,23,18,23,35,19,7,2,2,
+ 2,2,2,2,2,2,2,7,29,15,21,27,18,21,62,41,
+ 5,107,59,107,59,92,117,14,6,6,9,9,6,6,6,6,
+ 20,42,32,44,44,44,44,44,54,54,54,54,54,54,54,54,
+ 54,64,64,78,54,64,54,42,3,9,9,21,14,27,42,60,
+ 63,69,76,9,15,15,15,25,15,15,6,15,20,20,20,15,
+ 15,33,71,71,
+ 69,42,33,23,23,23,25,23,23,18,25,23,42,26,19,2,
+ 2,2,2,2,2,19,32,29,18,39,92,92,92,27,117,107,
+ 39,107,92,92,9,39,117,9,15,9,15,15,6,6,15,20,
+ 42,30,32,32,44,44,44,44,54,54,54,54,54,54,54,52,
+ 52,90,90,90,54,54,64,78,29,29,60,78,78,90,78,60,
+ 66,78,76,9,15,15,15,20,15,18,25,25,15,15,15,15,
+ 15,33,65,65,
+ 65,32,29,23,23,23,25,23,23,23,23,18,23,42,41,26,
+ 22,19,19,19,64,92,92,81,21,107,92,62,107,85,117,107,
+ 107,107,107,9,9,6,117,34,6,18,6,15,6,15,15,33,
+ 30,30,32,44,44,44,44,44,44,54,54,54,54,54,52,32,
+ 44,90,92,90,64,64,64,64,78,54,44,44,40,40,29,42,
+ 44,54,33,15,15,15,15,15,33,42,76,76,36,15,15,15,
+ 20,27,66,66,
+ 68,42,33,23,23,23,25,23,23,25,23,23,18,23,33,53,
+ 41,53,41,42,107,107,81,117,85,117,21,5,92,107,107,92,
+ 64,107,107,5,6,21,107,59,27,9,15,15,9,15,36,30,
+ 30,30,32,44,44,44,44,44,54,54,54,54,54,54,78,78,
+ 78,90,90,90,54,54,54,54,54,78,21,9,6,5,14,60,
+ 44,42,6,9,6,6,18,18,35,55,59,89,76,20,15,15,
+ 15,33,69,69,
+ 63,42,33,23,23,23,23,23,23,25,18,23,18,18,18,23,
+ 33,33,33,23,107,92,5,117,92,117,62,34,107,81,81,92,
+ 18,81,92,9,59,92,92,81,92,6,9,15,18,20,43,35,
+ 30,30,30,44,66,44,44,54,54,78,54,52,44,32,44,54,
+ 78,54,52,52,52,54,54,54,54,54,43,5,6,21,78,54,
+ 42,18,6,6,18,33,33,35,30,19,41,41,80,43,33,21,
+ 15,33,71,71,
+ 68,42,33,23,23,23,23,23,23,25,18,23,18,23,18,18,
+ 18,9,18,9,81,117,34,117,62,81,107,107,107,41,27,39,
+ 92,39,107,27,117,92,59,59,117,5,9,15,20,36,35,35,
+ 30,30,30,32,54,44,54,66,44,52,54,52,52,44,32,54,
+ 44,30,30,32,54,54,54,54,54,54,66,9,60,52,54,30,
+ 25,6,6,6,21,56,43,56,30,30,30,29,60,53,53,33,
+ 15,33,71,71,
+ 69,42,33,23,23,23,23,23,23,23,23,23,18,18,23,23,
+ 23,18,18,18,41,117,107,92,18,39,92,41,62,107,62,15,
+ 117,59,117,62,117,9,5,27,117,21,9,15,20,33,16,30,
+ 30,30,14,30,52,52,78,32,32,30,30,30,52,66,52,52,
+ 30,30,30,54,54,54,32,22,54,54,54,60,90,44,30,21,
+ 6,9,6,6,9,18,18,33,35,35,35,35,42,21,25,18,
+ 15,33,74,74,
+ 52,32,29,25,23,23,23,23,23,23,23,23,18,18,23,23,
+ 23,18,18,18,34,92,92,62,6,107,107,18,41,117,107,62,
+ 117,62,117,92,117,62,39,34,107,34,9,20,28,35,16,30,
+ 30,30,35,30,30,30,52,44,30,32,52,32,44,32,66,30,
+ 30,44,54,54,54,22,2,2,7,54,54,54,32,29,18,6,
+ 6,9,6,6,6,6,6,9,16,16,16,16,21,6,6,6,
+ 6,33,66,66,
+ 68,42,33,23,23,23,23,23,23,25,23,23,18,18,18,23,
+ 18,18,18,18,81,107,62,117,34,117,92,92,25,117,81,107,
+ 117,92,107,92,92,107,107,39,62,33,15,18,48,16,16,35,
+ 30,30,30,30,14,35,30,52,32,44,54,44,44,44,44,44,
+ 54,54,54,54,54,1,2,2,1,44,54,54,19,21,6,9,
+ 6,6,6,6,6,6,6,6,9,18,18,9,15,6,6,15,
+ 15,33,69,69,
+ 68,42,33,23,23,23,23,23,18,23,23,18,18,18,18,18,
+ 18,18,18,18,59,117,81,117,62,117,92,117,59,117,59,39,
+ 107,92,62,59,9,39,39,21,18,9,9,25,48,16,35,30,
+ 30,32,30,30,30,30,30,32,30,44,52,44,54,54,44,54,
+ 54,54,54,54,54,27,2,2,1,7,54,54,27,6,6,6,
+ 6,6,6,6,6,6,6,6,6,9,9,6,6,9,6,6,
+ 15,33,71,71,
+ 63,42,33,25,23,23,23,23,23,25,25,25,25,25,25,18,
+ 18,18,18,18,21,117,92,59,85,107,62,92,92,85,39,5,
+ 27,33,18,18,5,5,5,6,5,9,15,28,38,16,30,30,
+ 32,30,30,35,30,30,30,30,30,32,44,54,54,54,54,54,
+ 54,54,54,54,44,53,2,2,2,4,54,54,21,6,6,6,
+ 6,9,6,6,9,9,6,6,9,6,6,6,6,18,6,6,
+ 15,33,71,71,
+ 69,42,33,25,23,23,23,23,23,23,25,25,25,25,23,23,
+ 18,18,18,18,6,117,59,6,62,62,36,36,43,23,23,18,
+ 6,6,9,6,9,9,9,9,9,6,18,36,53,30,30,30,
+ 30,35,35,30,44,44,44,44,44,44,54,54,54,54,54,54,
+ 54,54,54,54,53,42,2,2,2,1,54,54,33,6,9,6,
+ 6,9,6,15,9,9,9,9,9,6,6,6,9,15,6,6,
+ 15,33,69,69,
+ 66,32,29,23,23,23,23,23,23,23,23,23,25,23,23,23,
+ 18,18,18,18,9,60,21,18,36,25,15,15,5,15,18,18,
+ 18,18,18,9,9,9,18,9,6,18,27,30,30,30,35,35,
+ 16,35,30,44,44,44,54,54,54,54,54,54,54,54,54,54,
+ 54,54,54,44,103,8,2,2,2,1,54,54,33,6,9,6,
+ 6,9,9,9,9,9,18,18,6,9,9,9,9,15,6,15,
+ 6,27,66,66,
+ 69,42,33,23,23,23,23,23,25,23,23,25,25,23,23,23,
+ 18,18,18,23,18,6,18,25,15,15,25,25,18,18,18,18,
+ 18,18,18,18,9,9,6,9,21,42,30,30,29,16,16,16,
+ 35,30,32,44,44,44,44,44,54,54,54,54,54,54,54,54,
+ 54,54,54,88,68,1,2,2,1,4,54,54,21,6,9,6,
+ 9,9,9,9,9,15,9,9,9,9,9,9,6,15,6,20,
+ 6,33,69,69,
+ 68,42,33,25,23,23,23,23,25,23,25,18,25,25,23,23,
+ 18,18,18,18,18,18,18,23,23,23,25,18,18,18,18,18,
+ 18,18,18,9,9,6,21,27,30,30,35,25,48,16,16,16,
+ 35,30,30,30,32,44,44,44,44,54,54,54,54,54,54,54,
+ 54,54,53,114,2,1,2,1,1,22,54,54,9,6,6,6,
+ 9,6,9,9,15,15,9,15,9,9,9,18,6,15,6,18,
+ 15,33,71,71,
+ 63,42,33,23,23,23,23,23,25,23,25,25,25,25,23,23,
+ 18,23,18,18,18,18,18,23,23,23,18,18,18,18,18,18,
+ 18,18,9,9,9,27,42,30,30,14,21,25,36,14,16,16,
+ 35,30,8,2,7,26,44,44,44,44,54,54,54,54,54,54,
+ 44,96,71,43,1,2,1,1,1,44,54,44,6,6,6,9,
+ 6,9,9,9,9,9,18,9,9,9,15,9,6,15,6,15,
+ 15,33,71,71,
+ 68,42,33,25,25,25,23,23,23,23,23,23,23,23,23,23,
+ 18,23,18,18,18,18,18,18,23,23,18,18,18,18,18,18,
+ 18,18,18,18,9,78,30,30,30,29,14,30,30,14,16,16,
+ 35,30,1,2,2,8,42,42,32,65,44,52,52,52,65,69,
+ 71,122,53,1,1,1,1,1,1,54,44,40,9,9,6,9,
+ 6,9,9,9,9,9,9,9,9,9,9,9,15,15,15,15,
+ 15,33,69,69,
+ 66,32,42,25,25,25,23,23,23,23,23,23,23,23,23,23,
+ 23,18,23,23,18,18,18,18,18,18,18,18,18,18,18,18,
+ 18,18,18,18,9,42,14,35,30,30,30,30,14,30,16,16,
+ 16,19,1,2,2,2,8,17,17,96,42,108,71,84,114,53,
+ 53,68,2,1,1,1,1,1,22,54,44,17,9,9,6,9,
+ 9,9,9,9,9,9,9,18,9,9,9,9,15,15,15,15,
+ 15,33,66,66,
+ 69,42,33,36,25,23,25,23,23,23,23,23,23,23,23,23,
+ 23,23,18,23,18,18,18,18,18,18,18,18,18,18,18,18,
+ 18,18,18,18,9,9,18,21,21,14,21,21,14,14,16,16,
+ 16,35,1,2,2,2,1,2,2,33,14,84,29,53,71,2,
+ 2,2,1,2,1,1,1,1,44,44,44,9,9,9,9,6,
+ 9,9,9,9,9,9,9,18,9,9,9,6,15,6,15,15,
+ 15,33,69,69,
+ 63,42,33,36,25,23,25,23,23,23,23,23,23,23,23,23,
+ 18,23,18,23,18,18,18,18,18,23,18,18,18,18,18,18,
+ 18,18,18,18,9,9,9,15,9,15,15,15,20,36,33,16,
+ 16,16,19,2,2,2,1,1,1,1,2,2,1,1,1,1,
+ 1,1,4,12,12,12,12,22,44,44,17,6,6,18,21,9,
+ 9,9,9,9,9,9,9,18,9,9,9,15,15,15,15,18,
+ 15,33,71,71,
+ 68,42,33,36,36,36,25,23,21,23,23,23,23,23,23,23,
+ 18,23,18,23,18,18,18,18,18,18,18,18,18,18,18,9,
+ 18,18,18,9,9,9,9,9,9,9,6,6,15,28,48,35,
+ 16,16,35,19,2,2,2,1,1,1,1,1,1,1,1,4,
+ 2,4,2,2,12,46,46,31,40,32,9,9,6,9,9,9,
+ 6,6,9,15,9,9,9,15,9,18,9,18,15,15,15,18,
+ 15,33,71,71,
+ 68,42,33,36,36,36,25,23,23,23,23,23,23,23,23,23,
+ 23,23,18,18,18,18,18,18,18,18,18,18,18,18,18,9,
+ 18,18,18,9,9,9,9,9,9,9,9,9,9,18,28,36,
+ 16,16,16,35,30,1,1,2,2,2,1,1,1,4,4,12,
+ 12,31,31,4,12,31,46,46,46,12,6,18,6,9,6,9,
+ 6,6,9,15,9,9,9,18,9,18,15,18,15,15,20,18,
+ 20,33,69,69,
+ 65,30,29,36,36,36,25,25,23,23,23,23,23,23,23,23,
+ 23,23,18,23,18,18,18,18,18,18,18,18,18,18,18,18,
+ 18,18,9,9,9,9,9,9,9,9,9,9,9,9,18,25,
+ 33,16,16,16,35,30,7,1,1,1,1,2,2,4,2,2,
+ 12,46,46,46,12,4,31,46,49,46,38,21,6,9,6,15,
+ 9,9,9,9,18,9,9,18,18,18,15,18,15,20,20,25,
+ 25,27,66,66,
+ 65,42,29,25,36,36,25,25,23,23,23,23,25,25,23,23,
+ 23,18,25,23,18,18,18,23,18,18,18,18,18,18,18,18,
+ 18,18,9,9,9,9,9,9,9,9,9,9,9,9,6,18,
+ 25,21,16,16,16,35,30,19,8,1,1,1,4,1,1,1,
+ 12,31,46,49,46,12,12,31,46,49,46,46,38,20,18,9,
+ 9,9,9,9,18,9,9,18,18,18,15,18,15,20,25,28,
+ 28,43,65,65,
+ 68,42,33,36,36,36,36,48,36,36,36,36,36,36,23,36,
+ 25,23,23,23,23,18,18,18,18,18,18,18,18,18,18,18,
+ 18,18,18,18,9,9,9,9,9,9,9,9,9,9,6,9,
+ 18,25,33,16,16,16,35,35,35,30,14,2,2,2,4,4,
+ 12,12,31,46,46,46,12,12,31,46,45,49,46,46,12,9,
+ 9,9,9,9,18,9,9,18,18,18,15,18,18,25,20,36,
+ 28,43,68,68,
+ 68,42,33,25,25,48,28,48,48,48,48,48,48,28,28,28,
+ 28,25,25,25,25,21,18,18,18,18,18,18,18,18,18,18,
+ 18,18,18,18,9,9,9,9,9,9,9,9,9,9,6,9,
+ 9,9,25,21,35,16,16,16,16,35,35,35,35,35,35,14,
+ 14,4,12,31,46,46,46,12,12,31,46,46,49,70,46,9,
+ 18,9,18,9,9,9,9,18,18,18,18,18,20,25,28,28,
+ 48,43,71,71,
+ 68,42,33,48,48,48,48,73,73,73,73,73,73,63,48,48,
+ 48,48,48,48,48,28,28,25,25,25,25,18,23,18,18,18,
+ 18,18,18,18,18,9,9,9,9,9,18,9,9,9,9,9,
+ 9,9,9,15,25,33,35,16,16,16,16,35,35,35,35,16,
+ 16,12,2,12,46,46,45,46,31,31,46,46,46,46,46,9,
+ 18,15,18,9,6,9,18,9,18,15,15,20,25,25,28,28,
+ 73,63,69,69,
+ 69,42,63,73,99,85,99,82,93,121,121,119,119,119,119,99,
+ 58,73,73,73,73,48,48,48,28,28,25,25,33,18,18,18,
+ 18,18,18,18,18,9,9,9,9,9,9,9,9,9,9,9,
+ 9,9,9,15,15,20,20,21,21,33,16,35,35,14,14,14,
+ 33,36,4,12,31,46,46,46,46,46,46,46,46,31,20,9,
+ 9,9,23,33,33,33,43,33,33,25,15,20,25,28,28,48,
+ 48,63,65,65,
+ 60,32,59,93,111,113,98,75,93,113,98,82,82,70,82,98,
+ 58,58,58,58,79,58,73,73,48,48,48,28,25,25,25,18,
+ 18,18,18,18,9,9,9,9,9,9,9,9,6,9,9,9,
+ 9,6,9,9,15,15,15,15,25,21,25,23,21,21,23,23,
+ 25,18,12,4,12,31,46,46,46,31,31,31,12,21,9,9,
+ 21,33,63,88,100,100,100,100,77,71,33,25,25,28,28,36,
+ 48,43,65,65,
+ 68,42,58,75,111,113,113,93,75,113,93,46,46,46,57,99,
+ 79,51,51,51,58,58,51,58,58,73,38,48,48,28,28,25,
+ 18,18,18,18,9,9,9,9,9,9,9,9,9,9,9,9,
+ 9,9,9,9,9,9,18,9,18,18,18,18,18,18,18,18,
+ 18,9,18,12,2,12,31,46,31,12,12,12,21,18,18,18,
+ 77,77,106,106,106,106,106,106,106,106,88,71,25,28,28,36,
+ 48,43,71,71,
+ 68,42,76,93,98,98,93,93,98,111,121,98,98,98,121,121,
+ 121,51,51,51,51,51,51,51,51,58,51,58,73,48,48,28,
+ 28,25,18,9,9,9,9,9,9,9,9,9,9,9,9,9,
+ 9,9,9,9,9,9,9,9,18,18,18,18,18,9,18,18,
+ 9,18,18,20,12,12,12,12,12,12,21,9,9,6,33,63,
+ 106,106,106,106,89,89,100,89,106,106,106,106,63,36,28,36,
+ 36,43,71,71,
+ 68,42,58,75,93,93,57,75,111,111,111,111,111,111,111,111,
+ 121,79,51,51,51,51,51,51,51,51,51,58,51,58,73,48,
+ 48,48,25,23,18,18,9,9,9,9,9,9,9,9,9,9,
+ 9,9,9,9,9,9,9,9,18,18,18,18,18,9,9,18,
+ 18,9,6,18,18,20,21,20,21,9,9,18,18,21,77,106,
+ 106,106,106,106,41,40,80,69,106,106,106,106,100,63,36,36,
+ 28,33,71,71,
+ 69,42,37,57,75,82,82,93,98,98,93,93,98,111,111,113,
+ 111,121,79,51,51,51,51,51,51,51,51,51,51,51,51,58,
+ 73,48,28,28,25,25,9,9,9,9,9,9,9,9,9,9,
+ 9,9,9,9,9,9,9,9,9,9,18,18,9,18,9,9,
+ 9,9,9,18,9,9,9,18,9,9,9,18,6,77,100,106,
+ 106,106,106,106,26,60,76,60,106,106,106,106,106,89,43,20,
+ 25,27,69,69,
+ 65,32,58,75,75,93,82,93,82,57,57,46,46,82,111,111,
+ 113,121,121,51,51,51,51,51,51,51,31,51,51,51,51,51,
+ 51,51,38,48,28,28,18,9,9,9,9,9,9,9,9,9,
+ 9,9,9,9,9,9,9,9,18,18,18,9,9,9,18,9,
+ 9,9,18,9,9,9,9,18,9,9,9,9,21,89,106,106,
+ 106,106,106,89,80,100,76,80,106,119,106,106,106,106,63,36,
+ 25,43,66,66,
+ 53,42,58,75,75,75,75,93,70,46,46,46,46,82,98,93,
+ 98,98,121,99,58,51,51,51,51,51,37,31,51,51,51,51,
+ 51,51,51,38,48,48,28,25,9,9,9,9,9,9,9,9,
+ 9,9,18,9,9,9,9,9,18,9,9,18,9,9,18,9,
+ 9,9,9,9,9,6,9,9,9,9,9,9,68,106,106,106,
+ 106,106,60,26,80,60,89,60,89,89,106,106,106,106,100,36,
+ 25,33,69,69,
+ 68,42,51,57,46,57,57,75,82,70,79,79,99,98,98,46,
+ 46,93,121,121,79,51,51,51,51,51,51,51,51,51,51,51,
+ 51,51,51,37,31,38,28,25,18,9,9,9,9,9,9,9,
+ 9,9,18,9,9,9,9,9,9,9,9,18,9,9,9,9,
+ 18,9,9,9,9,9,9,9,9,9,18,9,77,106,106,106,
+ 106,106,22,7,60,22,89,26,76,22,106,106,106,106,106,36,
+ 25,33,71,71,
+ 68,43,31,46,46,46,57,57,75,82,93,93,98,93,98,82,
+ 82,98,121,99,58,51,31,51,31,51,37,51,51,51,51,51,
+ 31,51,51,51,37,37,73,36,48,25,25,9,9,9,9,18,
+ 9,9,9,9,9,9,9,9,9,9,9,18,9,9,9,9,
+ 9,9,9,9,9,9,9,9,9,18,9,21,89,106,106,106,
+ 106,106,26,26,60,26,89,4,80,26,106,106,106,106,106,63,
+ 25,33,71,71,
+ 69,42,31,46,46,57,57,57,75,75,75,75,75,75,93,98,
+ 98,79,58,51,58,98,58,51,31,31,31,31,37,51,51,51,
+ 51,51,51,51,51,51,51,37,48,28,28,18,9,9,9,18,
+ 9,18,9,9,9,9,9,9,9,9,9,9,9,9,9,9,
+ 9,9,9,9,9,9,18,9,9,21,9,21,89,106,106,106,
+ 80,106,60,76,76,60,76,26,89,76,119,106,106,106,106,63,
+ 36,43,69,69,
+ 52,32,58,57,57,57,57,57,57,46,57,57,75,82,98,79,
+ 58,37,31,31,82,111,98,58,51,37,37,31,31,51,51,51,
+ 51,51,51,51,51,51,51,51,51,36,28,28,18,9,9,18,
+ 18,18,9,9,9,18,9,9,9,18,9,18,9,9,9,9,
+ 9,9,9,9,9,9,9,9,9,18,9,21,89,106,106,106,
+ 26,76,26,80,60,106,76,80,76,80,106,106,106,106,106,63,
+ 28,27,66,66,
+ 69,42,58,57,57,57,57,57,57,46,46,57,57,82,93,51,
+ 31,31,31,46,57,98,111,98,79,51,31,37,51,51,51,51,
+ 31,31,51,51,51,51,51,51,57,58,48,28,25,9,18,23,
+ 23,9,9,9,9,9,9,9,9,18,9,18,9,9,9,9,
+ 9,9,9,9,9,9,9,9,18,18,18,18,77,106,106,106,
+ 22,40,4,76,41,80,76,60,40,60,22,80,106,106,106,43,
+ 28,33,69,69,
+ 68,43,37,57,57,57,57,57,57,57,57,57,57,57,58,37,
+ 58,51,46,57,79,98,111,111,111,79,58,51,51,51,51,51,
+ 51,31,31,51,51,51,51,51,51,58,58,28,28,15,25,23,
+ 18,9,18,9,9,9,9,9,18,9,9,9,18,18,9,9,
+ 9,18,9,9,9,9,9,9,18,21,18,9,77,106,106,106,
+ 40,60,26,76,41,60,60,26,41,22,7,80,106,106,106,36,
+ 28,33,71,71,
+ 68,42,31,57,57,46,46,57,57,57,57,49,57,51,37,51,
+ 79,82,82,93,98,98,91,93,98,121,99,51,51,37,51,51,
+ 51,51,31,51,51,51,51,51,51,37,37,37,36,25,25,18,
+ 9,18,9,18,9,9,9,9,18,9,9,9,18,18,18,9,
+ 9,18,18,18,18,18,9,9,18,18,18,18,33,100,106,106,
+ 106,106,89,89,76,76,60,60,60,26,41,106,106,106,71,36,
+ 28,43,68,68,
+ 69,42,37,57,46,46,46,46,46,57,49,57,51,37,37,51,
+ 82,75,82,82,93,93,93,46,51,79,58,37,51,37,37,51,
+ 51,51,51,51,51,51,51,51,51,37,31,31,34,36,28,15,
+ 18,18,9,18,18,18,9,18,18,18,18,9,9,18,18,18,
+ 18,18,18,18,18,18,18,9,18,18,18,18,20,89,106,106,
+ 106,106,106,106,106,106,106,106,106,100,106,106,106,106,63,48,
+ 48,43,69,69,
+ 52,32,37,46,46,46,46,46,46,57,57,51,37,37,58,57,
+ 51,51,51,58,51,82,79,31,31,37,37,37,37,37,37,37,
+ 51,51,51,51,51,51,51,51,51,51,31,37,51,38,28,28,
+ 15,18,18,18,18,18,18,18,18,18,18,18,18,18,18,18,
+ 18,18,18,18,21,18,18,18,18,18,21,20,21,36,100,106,
+ 106,106,106,106,106,106,106,106,106,119,119,106,106,63,48,48,
+ 36,43,66,66,
+ 69,42,37,46,46,46,46,46,46,57,51,37,37,37,58,37,
+ 31,37,37,37,37,37,37,37,37,31,37,37,37,37,37,37,
+ 37,37,31,31,51,37,37,51,51,51,51,51,51,51,36,28,
+ 28,15,18,18,18,23,18,18,18,18,18,18,23,18,18,18,
+ 18,25,20,25,36,21,18,18,18,18,21,15,21,20,63,89,
+ 106,106,106,106,106,106,106,106,106,106,119,100,89,48,48,48,
+ 48,43,69,69,
+ 63,42,31,46,46,46,46,57,57,57,51,51,58,58,58,37,
+ 37,37,37,37,37,37,37,37,37,37,51,58,51,51,58,58,
+ 37,37,58,37,37,37,31,37,51,51,51,51,51,37,37,28,
+ 28,28,23,23,18,23,18,18,18,18,18,23,21,18,18,18,
+ 25,21,20,36,36,20,25,25,18,18,21,25,21,20,36,63,
+ 89,106,106,106,119,106,106,106,106,119,89,88,36,48,48,48,
+ 48,33,71,71,
+ 53,42,37,57,46,57,57,57,57,57,57,57,57,57,57,57,
+ 57,58,51,51,51,46,46,57,57,75,57,75,75,82,82,82,
+ 82,85,85,85,58,51,37,31,31,31,51,51,51,37,31,38,
+ 28,28,25,23,18,18,18,18,18,18,18,18,18,18,23,25,
+ 25,25,25,36,43,25,25,36,25,18,21,21,25,25,20,36,
+ 36,89,77,106,100,106,106,106,89,106,36,48,28,48,36,48,
+ 48,43,71,71,
+ 53,42,51,57,57,57,57,57,57,57,57,57,57,57,57,57,
+ 57,75,57,46,46,46,57,57,57,75,75,75,75,75,86,93,
+ 98,98,98,98,98,98,93,82,79,58,51,51,51,51,51,58,
+ 38,28,25,18,18,18,18,18,18,18,18,18,18,18,25,20,
+ 20,25,25,36,36,25,20,36,25,20,25,25,25,25,25,25,
+ 20,36,28,71,36,89,48,89,48,48,28,28,48,48,48,48,
+ 28,43,71,71,
+ 65,42,37,57,57,57,57,57,57,57,57,57,46,57,57,57,
+ 57,57,57,57,51,51,57,57,57,57,57,57,57,57,57,82,
+ 85,79,58,82,95,95,98,98,98,95,85,31,51,79,79,85,
+ 59,25,36,21,21,21,21,21,21,21,21,21,21,21,21,21,
+ 21,21,36,21,36,36,21,36,21,21,36,21,36,36,36,36,
+ 36,20,25,36,25,36,25,36,36,36,36,36,36,36,36,36,
+ 36,43,66,66,
+ 65,42,42,34,34,34,34,34,34,34,34,34,27,34,34,34,
+ 27,34,34,34,34,34,34,27,27,27,34,27,34,34,34,41,
+ 41,27,27,27,41,40,40,40,40,40,40,27,13,41,40,41,
+ 40,27,27,17,17,17,17,17,17,17,17,17,17,17,17,17,
+ 17,17,27,17,17,27,27,27,29,29,27,27,27,29,27,27,
+ 27,27,27,27,27,17,27,27,27,27,27,27,27,27,27,27,
+ 63,53,65,65,
+ 53,42,42,42,42,42,42,42,42,42,42,42,42,42,42,42,
+ 42,42,42,42,29,42,42,42,29,42,42,29,29,29,42,29,
+ 29,29,29,29,29,29,29,29,29,29,29,29,29,29,29,29,
+ 29,29,29,29,29,42,29,29,29,29,29,29,29,29,29,29,
+ 29,29,42,29,29,29,29,29,29,29,29,29,42,29,29,29,
+ 42,29,42,42,29,29,29,29,29,29,29,42,42,42,29,29,
+ 71,71,68,68,
+ 71,68,68,68,68,68,68,68,68,68,68,68,68,68,68,68,
+ 68,68,68,68,68,68,68,68,68,68,68,68,68,68,68,68,
+ 68,68,68,68,68,68,68,68,68,68,68,68,68,68,68,68,
+ 68,68,68,68,68,68,68,68,68,68,68,68,68,68,68,68,
+ 68,68,68,68,68,68,68,53,68,68,68,68,68,68,68,68,
+ 68,68,68,68,68,68,68,68,68,68,68,68,68,68,68,68,
+ 71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,
+ 74,74,74,69,74,65,65,65,65,65,69,69,65,65,69,65,
+ 69,69,65,65,65,65,65,65,65,65,65,65,74,74,74,65,
+ 74,65,74,74,65,65,74,65,65,65,65,65,65,65,65,65,
+ 65,65,65,65,65,65,65,65,65,65,65,65,65,65,65,65,
+ 65,65,65,65,65,65,65,65,65,65,65,65,65,65,65,65,
+ 65,65,65,65,65,65,65,65,65,65,65,65,65,65,65,65,
+ 65,65,65,65,
+ 72,72,72,97,97,97,97,97,87,97,97,97,72,97,97,72,
+ 65,97,97,97,72,97,97,87,87,97,97,97,72,72,65,65,
+ 65,65,65,65,72,72,65,72,65,65,65,65,65,65,65,65,
+ 65,65,65,65,65,65,65,65,65,65,65,65,65,65,65,65,
+ 65,65,65,65,65,65,65,65,65,65,65,65,65,65,65,65,
+ 65,65,65,65,65,65,65,65,65,65,65,65,65,65,65,65,
+ 65,65,65,65,
+ 77,77,96,124,124,124,123,127,122,127,127,127,114,123,123,123,
+ 108,123,122,123,114,123,122,127,114,123,127,123,96,71,71,71,
+ 71,71,71,71,71,71,69,71,71,71,71,68,69,71,69,69,
+ 69,69,71,69,69,71,69,68,69,69,69,69,68,69,68,69,
+ 71,68,69,69,68,69,71,69,68,69,69,71,71,71,71,71,
+ 88,71,71,71,71,71,71,71,71,71,88,71,71,71,88,71,
+ 71,71,71,71,
+ 71,71,96,127,127,108,96,127,123,127,123,123,123,122,88,127,
+ 123,122,88,88,123,122,88,127,123,123,127,114,123,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,68,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,68,96,108,96,96,88,
+ 114,88,108,71,96,96,96,96,88,96,108,96,71,96,108,96,
+ 96,88,71,71,
+ 71,71,96,127,127,88,71,127,123,114,96,96,122,122,96,127,
+ 122,123,108,108,122,123,108,127,123,122,127,88,127,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,68,71,68,68,71,68,71,68,68,71,
+ 71,71,71,71,71,71,71,71,68,71,68,96,96,88,88,71,
+ 108,71,108,88,88,88,88,88,88,88,88,96,71,96,96,88,
+ 88,88,71,71,
+ 74,74,77,120,120,77,74,120,109,77,69,69,88,120,120,120,
+ 77,120,120,120,96,120,120,109,96,109,120,69,120,74,69,69,
+ 69,69,69,69,69,69,69,69,69,69,69,69,69,69,69,69,
+ 69,69,69,69,69,69,69,69,69,69,69,69,69,69,69,69,
+ 69,69,69,69,69,69,69,69,69,69,69,69,69,69,69,69,
+ 69,69,69,74,69,69,69,69,69,69,69,69,69,69,69,69,
+ 69,69,69,69,
+ 72,72,72,72,72,72,72,72,72,72,72,66,72,72,72,72,
+ 66,72,72,72,72,72,72,72,72,72,72,72,72,72,66,72,
+ 66,66,66,66,72,66,66,66,66,66,66,66,66,66,66,66,
+ 66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,
+ 66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,
+ 52,66,52,66,66,66,66,66,66,66,66,66,66,66,52,66,
+ 66,66,66,66,
+ 68,68,68,53,68,68,88,71,88,88,88,71,88,71,71,71,
+ 88,71,71,71,88,88,103,103,108,103,88,96,69,69,69,69,
+ 71,69,69,69,69,69,69,71,69,69,71,69,69,69,69,69,
+ 69,69,69,69,69,69,69,69,69,69,69,68,69,69,69,69,
+ 69,69,69,69,69,69,69,68,68,69,68,68,69,69,69,69,
+ 77,69,71,71,71,77,69,77,71,71,71,71,77,71,77,77,
+ 77,69,69,69,
+ 68,68,68,68,68,68,84,84,84,84,103,84,103,84,84,84,
+ 103,84,84,84,84,103,115,115,115,103,103,103,88,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,68,71,71,71,71,71,71,68,71,68,68,68,71,
+ 71,71,68,71,71,71,68,71,68,71,71,71,71,71,71,71,
+ 96,96,96,96,96,96,96,96,88,96,96,96,88,96,108,96,
+ 96,88,71,71,
+ 68,68,84,68,68,68,68,84,68,68,96,103,103,84,84,103,
+ 96,84,84,56,68,84,103,103,114,103,103,103,88,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,68,68,71,71,71,68,71,
+ 71,71,68,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 96,96,96,96,88,88,96,96,88,96,96,96,71,96,108,96,
+ 88,88,63,63,
+ 68,68,84,68,68,68,68,68,68,84,114,84,96,88,103,114,
+ 108,103,68,56,56,84,103,103,103,103,103,103,88,71,69,69,
+ 69,77,69,69,69,69,69,69,69,69,69,69,69,69,69,69,
+ 69,69,69,69,69,69,69,69,69,69,69,69,69,69,69,69,
+ 69,69,69,69,69,69,69,69,69,69,69,69,69,69,69,69,
+ 69,77,69,69,69,74,74,74,69,74,69,74,69,69,77,69,
+ 69,74,69,69,
+ 68,68,71,68,68,68,68,68,68,88,114,84,84,84,108,103,
+ 108,103,68,56,56,84,103,96,103,103,103,103,74,72,66,66,
+ 66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,
+ 66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,
+ 66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,
+ 66,66,52,66,66,66,66,66,66,52,66,66,66,66,66,52,
+ 66,66,66,66,
+ 68,68,68,68,68,68,84,84,84,88,84,84,88,88,88,88,
+ 88,88,84,84,84,84,103,88,103,103,103,103,88,71,71,69,
+ 69,69,69,69,69,69,69,69,69,69,69,69,69,69,69,69,
+ 69,69,69,69,69,69,69,69,69,69,69,69,68,68,68,69,
+ 69,68,69,69,69,69,69,69,69,69,69,69,69,69,69,69,
+ 69,69,69,69,69,69,69,69,69,69,69,69,69,74,69,69,
+ 69,69,69,69,
+ 68,68,68,68,68,84,103,103,84,96,88,71,96,103,71,96,
+ 96,84,103,88,96,103,103,96,103,103,103,103,88,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 68,71,71,68,71,68,68,68,68,68,68,68,63,63,68,68,
+ 68,71,68,71,71,71,68,68,71,71,71,71,71,71,71,88,
+ 88,96,88,96,96,88,96,96,88,96,71,88,96,96,71,96,
+ 96,71,71,71,
+ 68,68,68,68,68,68,84,84,84,84,84,84,84,84,84,103,
+ 84,68,84,84,84,103,122,103,103,103,103,103,88,63,71,71,
+ 71,71,71,71,71,71,71,71,71,71,63,71,68,71,71,68,
+ 71,71,71,71,63,68,63,68,68,68,63,68,71,68,68,68,
+ 71,68,68,71,68,71,71,68,63,68,63,71,71,71,68,88,
+ 88,96,96,96,108,96,108,96,96,108,88,96,108,88,88,108,
+ 96,71,71,71,
+ 68,68,68,68,68,68,53,68,68,68,55,88,88,71,88,88,
+ 68,68,53,53,55,88,122,114,103,103,103,103,74,69,69,69,
+ 69,69,69,68,69,68,68,69,69,69,69,69,68,68,69,69,
+ 68,68,69,69,69,69,69,69,69,69,69,69,69,69,69,69,
+ 69,69,69,69,69,69,69,69,69,69,69,69,69,69,69,69,
+ 69,74,77,69,74,74,77,77,74,77,69,77,74,69,74,77,
+ 74,69,69,69,
+ 66,66,66,66,66,65,66,66,66,65,66,72,72,65,65,65,
+ 65,65,65,65,65,87,96,96,87,87,87,87,52,66,66,52,
+ 66,66,66,66,66,66,66,66,66,66,66,52,66,52,66,66,
+ 52,52,52,52,52,52,52,52,52,52,52,52,52,52,66,52,
+ 66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,66,
+ 52,52,66,66,66,66,52,52,66,52,66,66,52,66,66,52,
+ 66,66,66,66,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,123,127,114,
+ 108,108,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,122,122,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,123,122,127,127,123,127,127,88,
+ 96,88,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,122,127,127,88,71,
+ 71,53,123,123,123,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,123,123,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,122,114,88,68,96,88,71,88,
+ 68,42,108,114,114,114,122,123,123,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,123,127,123,123,127,127,127,
+ 123,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,114,88,114,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,114,108,96,53,71,108,88,96,71,
+ 96,53,114,114,114,108,108,114,114,122,122,114,122,122,123,123,
+ 127,127,127,127,127,123,127,122,122,122,123,122,122,122,123,122,
+ 122,123,123,123,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,114,96,88,68,96,114,127,127,127,127,127,127,127,
+ 127,122,114,122,114,122,114,123,127,127,114,122,96,71,96,71,
+ 42,68,114,114,114,114,114,114,114,114,122,122,123,123,123,127,
+ 127,123,122,122,122,127,122,114,122,122,122,122,122,122,123,123,
+ 122,123,123,123,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,123,114,122,122,108,96,96,96,71,122,127,127,122,123,123,
+ 122,114,114,114,114,114,122,127,127,123,114,108,71,88,88,71,
+ 42,96,108,114,114,114,114,114,114,114,114,114,114,114,122,122,
+ 123,122,122,122,122,122,123,122,122,123,123,123,123,122,123,123,
+ 123,122,123,123,123,127,123,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,123,
+ 123,96,114,108,123,96,108,88,88,71,42,96,122,114,122,122,
+ 122,114,114,114,114,96,127,127,127,122,122,88,88,96,88,88,
+ 53,108,114,114,114,114,122,122,122,122,122,122,122,114,123,123,
+ 123,123,123,122,114,122,123,123,123,123,122,114,122,123,123,122,
+ 123,123,123,122,122,123,123,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,123,123,122,
+ 114,123,127,96,96,108,71,71,71,68,68,71,114,114,114,122,
+ 114,114,114,108,108,114,114,127,123,88,71,88,96,96,68,29,
+ 71,114,108,108,108,114,114,96,114,114,123,122,108,114,123,122,
+ 123,123,123,123,123,123,123,123,123,122,122,123,122,122,122,122,
+ 122,122,122,123,123,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,123,123,122,122,114,
+ 96,88,96,108,108,88,42,71,71,53,71,42,42,114,114,114,
+ 114,114,108,122,127,127,108,127,68,68,68,71,53,71,53,42,
+ 108,108,108,53,53,71,68,53,42,71,71,53,53,68,88,114,
+ 122,122,114,114,122,122,122,123,122,123,123,122,122,123,123,122,
+ 123,123,123,123,127,123,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,123,122,122,114,
+ 96,88,114,29,96,88,71,114,88,68,68,42,42,114,123,122,
+ 122,114,114,127,127,123,122,122,53,68,68,88,53,71,68,53,
+ 71,108,127,123,96,68,71,71,68,53,42,53,53,53,53,88,
+ 108,114,122,122,122,122,122,122,114,114,114,114,114,114,114,114,
+ 114,114,122,122,122,123,123,127,123,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,123,114,
+ 96,71,108,88,68,108,96,108,88,71,53,42,29,53,114,114,
+ 114,108,127,127,122,123,108,123,68,53,96,88,71,71,42,68,
+ 71,88,88,123,123,114,88,88,88,71,68,68,68,42,68,68,
+ 53,71,108,122,122,122,122,122,122,114,114,122,122,122,114,122,
+ 122,114,122,123,122,123,123,123,127,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,122,114,114,
+ 114,88,71,88,96,88,88,96,88,68,53,53,29,71,114,114,
+ 114,123,127,122,122,114,108,96,53,88,96,71,88,68,42,42,
+ 88,96,88,114,108,127,127,96,68,53,68,68,53,68,71,68,
+ 68,53,68,71,96,122,123,114,122,88,88,96,88,96,108,96,
+ 114,122,108,114,108,123,123,127,123,127,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,123,122,122,
+ 114,108,88,71,88,71,88,68,53,53,42,42,19,88,114,114,
+ 114,127,127,123,122,123,96,42,71,96,96,96,108,96,42,53,
+ 122,88,108,122,123,88,123,127,122,96,53,71,71,71,96,96,
+ 71,88,71,88,71,88,71,88,71,96,88,88,88,88,96,88,
+ 96,96,88,88,96,108,122,122,123,123,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,123,122,114,108,114,
+ 114,108,88,71,88,68,68,68,53,53,42,29,29,88,88,96,
+ 108,114,127,127,127,108,68,71,96,114,108,96,88,96,96,53,
+ 96,114,123,123,127,122,53,114,127,123,114,88,71,96,96,88,
+ 88,96,96,96,96,96,88,71,88,114,114,96,96,108,108,114,
+ 114,114,114,114,108,114,114,122,122,123,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,123,122,114,96,96,96,
+ 88,71,68,71,88,68,88,71,53,42,42,29,53,71,88,123,
+ 123,71,122,122,114,53,71,88,96,108,114,108,108,96,96,96,
+ 71,108,127,122,123,122,114,88,53,68,122,122,123,96,96,114,
+ 122,108,108,108,96,108,88,71,71,88,96,108,122,114,114,108,
+ 114,114,122,114,108,108,114,122,123,123,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,122,88,108,96,88,88,
+ 88,68,71,42,53,71,96,88,53,42,29,71,88,88,123,127,
+ 127,123,71,71,68,53,88,96,88,108,88,88,96,71,13,42,
+ 42,96,122,127,127,127,122,108,68,96,68,96,123,123,122,88,
+ 96,108,108,108,96,114,108,96,88,108,96,71,96,88,96,88,
+ 108,96,96,108,108,114,96,114,122,123,127,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,123,122,114,96,96,88,88,
+ 88,88,71,68,53,88,68,71,71,53,42,42,88,123,127,114,
+ 114,88,71,88,71,88,88,96,96,96,53,71,108,53,29,29,
+ 71,108,123,123,123,123,122,88,114,123,127,123,108,88,114,108,
+ 122,123,114,127,122,96,108,114,122,114,108,96,88,71,96,88,
+ 96,96,96,108,108,108,108,108,122,122,123,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,123,122,114,108,96,71,68,53,
+ 71,71,88,96,108,108,71,53,53,42,29,42,123,127,122,71,
+ 96,96,114,88,88,108,96,88,88,88,29,71,88,29,29,29,
+ 88,96,123,127,123,127,122,123,127,123,123,123,127,127,122,88,
+ 96,127,114,122,127,88,68,96,123,123,123,127,127,122,114,88,
+ 96,96,88,96,88,108,96,108,114,122,123,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,123,123,122,108,96,71,71,68,
+ 71,71,88,108,114,68,53,71,42,29,42,122,127,127,88,88,
+ 88,68,68,88,88,108,108,88,53,88,53,71,53,29,42,88,
+ 88,88,108,123,127,122,122,108,127,123,123,127,127,114,123,127,
+ 108,71,71,68,122,96,96,96,88,96,122,122,122,123,127,123,
+ 122,114,108,96,71,88,96,108,122,114,123,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,123,122,108,96,88,71,71,
+ 88,88,42,114,88,68,42,42,42,68,108,127,123,127,71,71,
+ 71,96,108,108,88,88,108,71,88,71,53,88,42,42,71,108,
+ 96,96,108,127,123,127,108,114,123,122,108,127,123,114,96,123,
+ 71,88,88,96,53,53,71,96,88,88,68,88,123,122,123,127,
+ 123,123,127,127,123,114,96,96,96,114,123,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,123,114,114,96,96,71,71,71,
+ 88,68,114,96,68,68,17,42,42,108,88,122,123,108,68,88,
+ 123,127,88,71,88,88,71,96,88,53,68,53,42,53,108,96,
+ 96,108,96,123,123,123,108,96,88,71,88,96,71,96,68,88,
+ 96,71,71,71,53,88,68,53,88,96,88,88,96,96,108,123,
+ 123,127,122,123,123,123,127,123,114,108,122,127,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,123,122,108,88,88,71,88,71,
+ 68,68,114,68,53,53,42,42,122,96,88,114,123,88,96,123,
+ 127,123,42,13,29,68,71,53,42,71,68,108,29,68,108,108,
+ 108,108,96,114,123,122,71,68,68,123,123,123,123,122,42,108,
+ 114,108,96,68,42,53,71,71,53,71,71,88,88,88,108,96,
+ 114,122,127,127,127,127,122,122,127,127,122,114,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,122,122,114,108,96,88,88,96,
+ 88,96,88,88,71,71,71,114,123,68,123,114,123,71,122,127,
+ 123,96,29,42,42,42,42,68,88,71,88,53,29,53,108,108,
+ 108,108,96,127,127,88,108,68,71,122,127,127,127,123,114,53,
+ 96,71,71,71,88,53,68,88,88,53,53,96,88,71,71,108,
+ 108,96,96,88,123,127,127,127,123,123,123,114,123,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,123,122,108,96,114,88,88,88,
+ 88,88,88,71,68,71,114,127,123,108,114,88,96,122,127,127,
+ 96,29,42,42,42,53,71,88,88,71,96,71,42,53,114,96,
+ 96,108,96,123,114,108,71,88,88,108,127,123,122,114,96,53,
+ 71,88,88,71,53,42,53,88,53,53,68,42,71,96,68,68,
+ 96,114,108,96,96,114,108,96,71,68,71,88,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,123,122,114,96,96,88,88,88,
+ 88,71,71,71,71,114,127,127,108,114,88,71,114,127,127,123,
+ 68,53,42,42,53,88,88,88,71,88,88,42,53,42,108,96,
+ 96,96,96,114,127,108,71,88,88,88,127,96,96,88,88,71,
+ 71,88,68,53,71,71,42,42,42,88,71,42,68,88,42,29,
+ 42,88,108,114,114,96,7,29,53,53,68,114,127,108,123,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,123,122,96,88,88,88,71,68,
+ 71,71,71,68,108,127,127,123,88,96,71,123,127,127,127,114,
+ 127,53,53,68,53,88,96,88,96,88,53,68,68,42,96,108,
+ 108,108,108,108,122,68,68,71,68,42,88,68,71,71,68,68,
+ 53,68,68,88,88,71,42,42,42,68,53,53,68,88,53,68,
+ 53,53,68,96,114,68,42,42,53,53,68,108,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,123,122,96,88,71,71,71,88,
+ 88,71,88,114,114,127,122,122,88,88,123,127,127,127,127,127,
+ 71,29,29,42,53,88,96,88,88,71,71,68,53,42,88,114,
+ 108,108,88,88,88,42,53,71,88,88,68,68,71,88,71,71,
+ 68,71,88,96,71,53,29,68,88,71,68,42,88,71,53,68,
+ 88,88,88,71,71,42,42,53,53,68,71,96,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,123,114,88,88,88,71,88,88,
+ 71,96,122,88,96,108,71,88,88,123,127,127,122,108,71,68,
+ 29,29,29,29,88,96,88,71,53,53,68,29,42,42,96,114,
+ 88,68,29,53,71,71,53,42,53,53,29,68,68,42,42,53,
+ 53,68,88,71,53,42,29,53,71,88,53,68,88,71,71,71,
+ 88,108,88,96,108,53,29,68,53,53,53,108,127,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,122,108,96,88,88,71,88,88,
+ 88,88,68,71,71,71,53,53,127,123,123,127,96,68,53,29,
+ 29,29,29,71,96,88,88,53,53,53,42,53,42,88,108,96,
+ 96,96,88,88,68,71,71,68,71,71,71,71,68,68,68,71,
+ 84,109,96,68,68,53,53,53,29,53,71,53,53,71,96,96,
+ 88,53,88,108,88,42,42,53,42,53,71,108,123,127,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,123,108,96,96,71,71,96,96,
+ 96,88,108,96,108,96,108,71,88,88,88,96,71,68,53,29,
+ 68,53,29,88,88,68,53,53,53,53,71,114,108,96,96,96,
+ 88,96,71,88,88,88,88,96,88,88,108,96,88,88,96,114,
+ 114,120,126,124,120,124,124,110,120,109,96,71,68,29,42,88,
+ 96,96,71,88,68,29,68,53,53,68,88,114,122,123,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,123,122,96,96,88,96,96,108,
+ 96,88,108,96,96,96,108,96,88,96,96,88,96,71,71,68,
+ 71,68,68,68,68,53,71,53,42,29,42,42,42,42,53,53,
+ 68,71,71,88,88,96,88,108,71,53,68,108,114,103,103,119,
+ 119,120,120,124,120,120,126,116,126,126,126,126,126,124,120,120,
+ 109,108,108,88,29,53,53,71,71,68,108,114,122,123,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,123,122,114,108,96,88,88,96,
+ 96,96,96,96,108,96,88,96,88,88,96,88,68,96,96,88,
+ 71,71,88,88,88,71,71,71,71,88,53,42,29,29,29,29,
+ 22,42,53,71,71,88,122,88,53,42,53,42,108,114,126,126,
+ 119,126,126,126,126,120,124,110,120,109,120,120,126,126,126,126,
+ 126,126,126,126,124,120,97,109,88,88,96,96,122,123,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,122,114,96,88,96,88,88,
+ 96,88,71,71,88,88,88,88,71,108,123,108,68,68,96,114,
+ 108,114,108,88,88,88,88,71,88,88,71,88,88,88,88,88,
+ 71,71,71,88,88,114,96,53,53,53,53,68,53,120,126,126,
+ 126,106,126,126,126,124,109,123,124,120,124,124,126,126,120,109,
+ 120,120,124,126,126,124,116,126,126,126,124,124,124,120,123,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,123,114,114,96,96,96,96,
+ 96,88,71,71,68,71,88,108,108,123,123,123,114,68,42,88,
+ 108,108,114,96,96,96,88,88,71,96,88,88,96,88,88,88,
+ 96,96,96,108,108,123,96,42,53,42,68,42,42,120,124,124,
+ 126,126,106,126,126,124,110,124,126,124,120,124,124,126,126,126,
+ 124,120,109,109,109,104,116,126,126,126,126,126,126,126,120,123,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,123,123,122,96,88,88,88,88,
+ 71,88,88,96,122,127,122,123,123,123,123,123,122,122,122,108,
+ 96,71,71,96,108,96,96,88,88,71,88,88,88,71,88,108,
+ 96,96,96,108,114,123,53,53,42,53,42,68,53,124,124,109,
+ 100,109,119,119,126,120,110,120,109,124,120,109,109,126,126,126,
+ 126,126,126,126,126,116,124,126,126,126,126,126,126,126,124,96,
+ 122,127,127,127,
+ 127,127,127,127,127,127,127,127,127,123,114,96,96,96,108,108,
+ 108,114,88,42,96,122,127,123,123,122,122,122,127,123,123,123,
+ 123,122,88,68,68,108,108,114,96,88,96,88,88,96,96,108,
+ 108,114,108,108,123,114,42,68,53,68,68,29,68,124,126,126,
+ 126,126,126,119,125,124,110,124,124,109,109,120,120,126,120,120,
+ 120,124,124,126,126,104,126,126,126,126,126,126,126,126,126,71,
+ 96,123,127,127,
+ 127,127,127,127,127,127,127,127,127,123,114,114,96,108,96,108,
+ 108,96,108,42,53,53,108,123,127,127,127,127,122,123,123,127,
+ 127,122,108,114,122,108,96,114,114,114,108,108,108,96,96,108,
+ 108,114,108,123,127,88,53,42,53,42,42,42,68,96,109,109,
+ 120,120,124,126,106,120,122,116,116,120,120,120,124,126,126,109,
+ 109,120,109,120,124,104,126,126,126,126,126,126,126,126,126,68,
+ 71,114,127,127,
+ 127,127,127,127,127,127,127,127,127,123,122,108,96,88,96,96,
+ 108,108,114,53,71,88,108,108,122,127,127,127,127,123,122,108,
+ 122,123,127,123,123,127,123,71,88,114,114,96,71,71,96,108,
+ 88,68,96,127,127,53,53,53,42,42,42,42,71,126,124,124,
+ 120,109,109,109,100,106,110,116,116,116,116,104,104,116,116,120,
+ 120,120,120,109,126,116,126,126,118,118,124,124,124,126,124,53,
+ 68,108,127,127,
+ 127,127,127,127,127,127,127,127,127,123,122,114,108,88,71,88,
+ 96,114,114,71,88,108,127,123,96,88,53,88,53,42,53,122,
+ 123,88,88,71,108,88,96,68,42,108,114,114,108,108,108,71,
+ 96,96,96,123,108,68,53,53,53,53,68,53,53,89,109,120,
+ 124,126,115,114,124,106,110,116,124,124,124,126,126,126,116,116,
+ 116,116,116,104,104,116,124,118,124,126,124,118,118,118,109,53,
+ 68,108,127,127,
+ 127,127,127,127,127,127,127,127,127,123,122,114,108,108,108,108,
+ 96,96,114,88,114,122,127,127,88,17,42,88,53,42,96,123,
+ 88,68,53,42,71,71,68,53,53,108,114,114,114,114,114,114,
+ 122,114,114,123,108,42,71,68,68,68,68,68,109,126,120,109,
+ 109,109,94,94,96,120,110,120,115,114,77,77,109,109,120,124,
+ 126,126,126,124,126,124,118,124,120,120,124,120,100,120,109,53,
+ 71,114,127,127,
+ 127,127,127,127,127,127,127,127,127,123,114,114,108,108,108,108,
+ 114,108,114,71,114,122,127,123,122,42,71,88,53,53,127,68,
+ 71,53,42,42,71,68,53,42,53,114,114,108,114,108,114,114,
+ 114,114,108,123,108,68,42,68,42,42,42,42,109,120,126,126,
+ 126,126,115,108,94,116,116,109,115,120,100,89,100,100,89,109,
+ 126,126,126,126,118,118,126,126,126,124,124,126,126,126,109,53,
+ 71,122,127,127,
+ 127,127,127,127,127,127,127,127,127,122,114,108,108,88,88,96,
+ 108,108,96,114,114,123,123,123,53,42,71,88,53,68,127,68,
+ 68,53,42,42,88,71,53,42,53,114,114,114,108,108,108,96,
+ 108,108,114,123,114,71,29,42,42,29,29,42,120,120,109,120,
+ 109,120,119,125,83,104,116,124,115,94,94,115,115,94,94,96,
+ 124,126,126,118,124,100,120,120,124,126,126,126,126,126,96,53,
+ 88,122,127,127,
+ 127,127,127,127,127,127,127,127,123,123,114,96,96,88,96,96,
+ 96,108,88,122,123,122,123,114,68,29,53,71,68,71,127,68,
+ 29,53,42,42,96,68,53,42,68,108,108,108,114,114,114,114,
+ 114,114,123,122,127,88,53,53,42,42,42,42,124,126,126,126,
+ 126,125,116,110,78,106,126,120,126,124,109,109,120,109,118,115,
+ 115,94,94,94,115,115,118,120,109,120,126,126,126,126,88,53,
+ 88,123,127,127,
+ 127,127,127,127,127,127,127,127,123,122,108,88,88,96,88,88,
+ 88,96,108,122,123,122,122,114,68,42,53,53,68,71,127,71,
+ 53,29,42,42,68,53,42,29,42,96,96,108,108,96,96,108,
+ 108,108,123,123,88,88,42,42,53,29,42,53,109,120,109,124,
+ 121,116,110,88,119,121,120,124,126,126,120,109,120,109,126,126,
+ 118,118,126,124,124,118,115,115,115,115,94,115,115,118,71,53,
+ 96,123,127,127,
+ 127,127,127,127,127,127,127,127,123,122,96,108,96,88,88,96,
+ 96,88,122,122,123,123,122,123,68,42,42,53,71,88,123,68,
+ 68,29,42,42,42,53,42,42,53,96,96,114,114,108,96,96,
+ 96,114,127,114,29,71,29,42,42,29,42,68,126,126,125,120,
+ 116,110,88,94,126,121,121,120,124,120,120,120,120,120,126,118,
+ 124,124,109,120,124,124,126,126,126,126,126,124,118,115,53,68,
+ 108,123,127,127,
+ 127,127,127,127,127,127,127,123,123,114,96,88,88,88,88,88,
+ 88,88,108,114,123,127,123,108,68,53,42,53,53,71,114,71,
+ 53,53,42,53,53,53,53,53,42,108,108,108,114,108,108,96,
+ 96,123,123,114,42,68,68,42,42,68,68,71,126,125,119,110,
+ 104,94,115,126,109,120,119,106,121,121,121,119,106,120,118,124,
+ 126,120,109,120,120,109,124,126,126,126,126,126,126,124,53,68,
+ 108,127,127,127,
+ 127,127,127,127,127,127,127,127,123,114,108,108,96,96,96,96,
+ 96,108,88,96,123,127,122,123,42,53,53,68,88,108,96,68,
+ 42,42,42,42,42,53,68,68,29,71,108,108,108,108,108,96,
+ 108,127,127,71,42,53,53,42,42,68,68,89,121,116,110,97,
+ 94,120,109,109,109,109,120,119,119,126,125,119,99,115,119,121,
+ 121,120,119,119,119,126,126,126,126,126,126,126,116,104,53,71,
+ 114,127,127,127,
+ 127,127,127,127,127,127,127,127,123,108,96,88,88,88,88,108,
+ 108,96,96,88,114,127,122,53,68,53,88,122,122,96,71,42,
+ 68,53,53,42,29,42,42,68,42,53,108,108,108,96,108,96,
+ 123,127,123,42,29,42,53,42,29,53,53,80,106,89,63,73,
+ 124,126,126,126,124,124,109,124,119,124,115,115,118,124,123,115,
+ 115,114,120,119,119,121,121,121,125,125,125,105,104,83,53,71,
+ 114,127,127,127,
+ 127,127,127,127,127,127,127,127,123,108,88,88,71,88,88,96,
+ 96,114,96,68,122,123,122,42,53,108,123,88,42,53,68,42,
+ 42,53,42,68,68,42,42,53,42,53,114,114,114,114,114,68,
+ 108,123,71,29,29,53,42,42,42,29,42,114,124,97,73,99,
+ 99,70,70,70,99,99,122,120,120,114,115,120,124,126,126,126,
+ 126,124,124,124,124,122,115,115,120,120,104,97,120,89,53,88,
+ 122,127,127,127,
+ 127,127,127,127,127,127,127,123,122,114,96,96,96,96,96,88,
+ 96,96,108,71,122,127,114,42,68,123,71,71,29,53,53,42,
+ 42,42,29,71,71,68,42,53,42,68,122,122,114,108,108,71,
+ 53,71,108,42,29,29,71,42,53,53,53,124,126,110,115,126,
+ 126,126,126,120,100,99,120,73,73,118,119,120,109,120,126,126,
+ 126,126,126,126,120,120,120,120,124,124,110,103,118,71,53,88,
+ 122,127,127,127,
+ 127,127,127,127,127,127,127,127,122,122,96,71,71,71,88,88,
+ 88,96,96,88,122,127,122,42,88,127,68,68,53,42,53,42,
+ 29,29,53,53,53,53,42,42,42,71,114,108,96,96,96,96,
+ 88,114,122,68,29,29,53,53,42,53,53,126,125,96,115,126,
+ 126,126,126,124,124,120,115,123,126,99,93,119,126,120,120,124,
+ 124,126,126,124,100,109,109,120,109,116,110,114,120,68,68,96,
+ 123,127,127,127,
+ 127,127,127,127,127,127,127,127,114,96,96,88,88,88,88,88,
+ 88,96,96,88,122,123,127,68,96,123,53,53,42,29,29,42,
+ 42,42,42,68,42,29,53,68,42,96,114,108,96,108,96,96,
+ 96,114,122,68,42,68,53,42,42,53,69,126,124,103,96,109,
+ 109,120,124,126,124,115,124,126,126,118,99,99,125,109,109,109,
+ 120,126,126,126,126,126,126,124,124,116,101,114,109,68,68,108,
+ 123,127,127,127,
+ 127,127,127,127,127,127,127,127,123,108,96,71,88,88,96,88,
+ 88,96,88,88,122,122,127,88,88,122,53,53,42,42,42,42,
+ 29,29,42,53,29,42,42,42,42,88,108,96,96,88,96,96,
+ 108,114,122,88,29,42,42,53,42,13,96,126,108,108,94,94,
+ 115,114,114,114,122,126,126,126,124,118,126,70,95,120,120,120,
+ 120,109,124,126,126,126,126,126,126,105,101,124,124,53,68,108,
+ 123,127,127,127,
+ 127,127,127,127,127,127,127,127,122,122,96,96,88,88,71,88,
+ 96,88,96,88,114,114,127,96,88,122,42,71,42,42,29,42,
+ 29,42,42,42,42,42,53,42,29,71,108,88,96,96,96,53,
+ 68,108,114,88,29,29,53,71,42,29,109,124,109,120,122,115,
+ 94,94,94,94,115,122,124,124,118,124,126,124,75,119,126,126,
+ 120,124,124,124,126,126,126,126,126,116,101,126,120,53,71,114,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,108,114,88,71,88,88,88,
+ 71,71,71,71,114,122,127,123,88,123,53,68,68,53,29,42,
+ 19,29,42,29,42,53,42,53,68,88,88,108,88,96,96,96,
+ 108,88,88,114,68,42,68,42,29,53,119,123,110,124,126,126,
+ 126,126,126,109,108,88,94,103,108,108,94,103,118,93,119,119,
+ 106,106,120,124,126,109,109,109,124,116,101,124,77,53,71,122,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,123,96,68,71,68,68,71,
+ 68,68,88,96,88,122,122,127,108,127,68,68,42,29,42,53,
+ 42,29,42,42,29,42,53,42,42,108,108,114,108,114,114,108,
+ 114,71,123,108,71,68,53,42,42,42,121,118,97,126,126,126,
+ 126,126,126,126,124,124,120,120,118,124,124,124,94,70,118,126,
+ 126,119,119,119,119,119,106,119,119,105,103,120,88,53,88,122,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,88,68,53,88,96,96,
+ 108,114,114,114,96,114,108,127,122,108,42,53,42,42,42,42,
+ 42,29,29,68,29,29,53,53,53,96,88,88,108,108,96,96,
+ 108,96,114,123,68,42,53,53,42,68,124,124,97,120,124,124,
+ 124,124,109,120,124,124,126,126,118,126,126,126,126,94,70,124,
+ 126,126,126,124,124,124,119,125,109,77,96,119,77,53,88,123,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,114,71,68,71,71,71,
+ 96,71,42,68,71,96,71,127,127,114,68,68,42,42,53,29,
+ 42,53,42,53,42,29,29,42,53,96,96,96,88,96,71,96,
+ 71,108,122,114,53,42,68,68,29,68,126,115,120,124,120,109,
+ 109,120,126,109,120,120,120,100,118,126,124,126,126,124,94,70,
+ 126,126,126,124,109,109,124,115,94,83,94,115,63,68,96,123,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,114,96,53,68,71,71,
+ 71,29,42,29,53,71,42,114,127,108,68,42,68,53,42,29,
+ 42,53,42,42,42,53,42,29,88,123,122,114,114,114,114,108,
+ 71,108,123,71,68,42,53,42,29,68,126,108,119,121,126,126,
+ 126,126,126,124,120,109,120,120,118,126,126,124,124,124,114,73,
+ 99,126,124,126,120,120,109,115,109,110,123,126,53,68,96,123,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,123,114,88,96,96,
+ 88,96,88,88,68,53,53,53,127,88,71,29,53,68,42,42,
+ 53,68,68,42,29,53,42,29,53,88,96,96,108,108,122,108,
+ 42,122,108,108,68,42,42,29,69,88,127,96,124,125,121,121,
+ 121,121,121,125,119,120,109,109,124,126,126,120,109,120,120,115,
+ 70,118,120,109,120,120,124,118,97,101,114,120,53,68,108,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,123,122,114,108,96,
+ 96,108,122,114,96,88,88,71,71,71,88,53,53,53,53,29,
+ 42,42,29,29,13,29,29,29,17,17,42,17,29,29,42,68,
+ 88,88,96,114,53,42,29,42,42,83,101,101,112,112,122,122,
+ 109,100,119,119,121,121,121,119,119,125,125,119,119,100,124,126,
+ 94,70,70,99,99,79,99,118,104,101,120,109,53,71,114,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,122,88,
+ 71,108,96,122,96,108,88,108,114,114,108,88,108,88,108,71,
+ 42,29,19,29,42,17,13,13,13,13,13,13,13,13,29,29,
+ 29,29,71,88,42,42,68,88,68,84,101,101,112,101,101,101,
+ 101,101,101,101,112,112,123,123,118,118,118,119,121,121,121,121,
+ 119,109,96,73,73,94,118,73,115,114,70,89,53,71,114,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,123,
+ 114,68,53,71,88,53,68,53,88,108,123,108,108,114,123,114,
+ 96,71,42,42,8,8,13,13,8,13,29,19,7,13,13,13,
+ 29,42,42,53,108,88,122,122,108,121,121,105,119,116,110,83,
+ 83,101,101,101,101,101,112,101,112,112,112,103,103,114,114,118,
+ 118,99,121,121,121,121,106,122,109,67,105,73,53,88,122,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,123,114,
+ 96,71,53,42,68,53,68,71,114,108,88,108,123,114,108,123,
+ 123,123,108,68,96,53,17,53,71,68,53,88,68,53,42,42,
+ 13,29,29,53,53,42,71,123,88,124,126,116,119,121,119,119,
+ 113,121,121,119,106,104,112,112,112,112,112,101,101,101,101,83,
+ 101,112,103,88,108,109,109,124,118,89,106,72,53,88,122,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,122,
+ 96,96,88,71,88,68,68,71,88,71,68,88,68,53,53,71,
+ 68,68,68,68,96,71,71,108,114,96,88,88,88,68,29,29,
+ 42,22,13,29,19,29,29,29,53,126,126,120,109,109,109,109,
+ 109,124,119,125,121,121,121,113,121,112,112,112,112,112,112,112,
+ 101,112,101,101,101,83,101,88,84,94,73,68,53,96,123,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 123,114,108,123,96,88,88,88,71,71,71,68,42,68,42,42,
+ 29,29,17,42,29,42,68,53,42,42,53,29,42,8,29,42,
+ 8,13,8,8,13,17,4,29,53,126,126,126,104,126,126,124,
+ 120,120,120,109,120,120,120,126,121,121,112,112,126,126,124,124,
+ 123,122,112,112,112,112,94,101,83,89,118,68,68,96,123,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,123,108,114,114,122,127,71,53,68,42,53,
+ 42,42,42,42,29,29,42,53,53,42,29,42,53,53,53,68,
+ 42,29,13,13,29,13,42,13,88,126,126,126,124,116,124,126,
+ 126,126,126,126,126,126,126,126,126,113,116,101,122,126,126,126,
+ 126,126,120,109,115,94,83,112,109,79,103,53,68,108,123,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,114,88,122,88,53,
+ 53,68,53,53,71,68,53,53,71,88,71,96,71,88,68,71,
+ 68,53,53,71,88,68,96,88,96,126,126,126,126,116,120,109,
+ 100,120,120,124,126,126,126,126,126,126,113,112,112,124,126,126,
+ 126,126,126,115,94,83,123,126,126,112,101,53,71,114,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,114,123,127,123,127,123,123,
+ 122,114,108,122,122,123,122,122,114,114,108,122,122,108,114,114,
+ 108,114,123,123,123,123,123,123,120,109,109,109,124,126,110,124,
+ 126,126,124,124,126,126,126,126,126,126,125,113,112,112,124,124,
+ 126,126,115,115,101,126,126,109,120,101,83,53,71,114,127,127,
+ 127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,127,
+ 127,127,127,127,127,127,127,127,124,126,126,124,126,126,126,104,
+ 126,124,124,124,126,126,126,126,124,124,126,121,121,112,112,112,
+ 112,101,94,112,83,120,120,124,124,112,83,53,88,122,127,127,
+ 127,127,127,127,
+ 96,96,96,96,96,96,96,96,96,96,96,96,96,96,96,96,
+ 96,96,96,96,96,96,96,96,96,96,96,96,96,96,96,96,
+ 96,96,96,96,96,96,96,96,96,96,96,96,96,96,96,96,
+ 96,96,96,96,96,96,96,96,124,126,126,126,126,126,126,124,
+ 116,126,109,120,126,126,124,124,120,120,120,126,121,111,119,116,
+ 116,103,103,101,101,101,101,101,83,112,67,17,53,88,96,96,
+ 96,96,96,96,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,126,126,126,126,126,126,126,126,
+ 116,104,120,109,109,126,120,100,109,109,109,126,124,120,106,121,
+ 111,93,99,108,106,121,90,104,87,101,29,7,42,68,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,88,126,124,89,109,126,126,126,126,
+ 126,104,126,126,126,126,124,120,120,100,120,126,126,124,124,120,
+ 120,114,124,103,109,125,125,121,113,121,29,19,42,68,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,88,126,124,120,109,120,124,124,100,
+ 120,120,104,126,126,125,119,125,118,115,94,115,115,124,126,126,
+ 126,115,124,101,109,120,124,126,126,119,19,19,42,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,96,110,120,109,120,120,124,120,100,
+ 109,120,120,104,125,121,125,125,73,119,121,121,99,99,73,73,
+ 114,94,126,101,109,120,120,120,126,120,7,19,53,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,68,120,123,110,124,124,124,126,126,124,
+ 120,120,120,120,120,126,126,124,115,120,109,100,106,119,125,125,
+ 120,121,121,109,109,120,120,120,120,96,7,29,53,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,71,96,88,108,88,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,68,120,126,122,124,126,120,120,120,124,
+ 126,125,121,125,110,124,126,124,115,126,126,124,120,120,120,109,
+ 109,120,124,103,124,121,126,126,124,88,7,29,68,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,114,96,68,88,88,68,71,71,71,68,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,68,124,126,126,110,126,126,124,120,120,
+ 121,121,126,126,126,104,126,115,94,120,120,120,120,124,124,126,
+ 126,126,122,103,126,125,121,124,100,53,7,29,68,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,68,123,71,71,71,71,108,71,108,71,114,
+ 108,96,96,88,88,96,96,88,88,88,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,68,109,126,126,126,110,126,126,126,121,
+ 121,126,124,126,126,124,104,124,115,126,124,124,120,109,120,126,
+ 124,126,114,114,126,126,119,121,120,53,7,42,68,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,68,122,88,68,71,88,114,71,114,71,114,
+ 68,88,114,88,122,88,96,96,114,88,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,124,126,126,124,116,125,121,125,
+ 126,126,124,109,120,120,104,96,118,126,126,126,120,124,124,126,
+ 126,126,103,122,126,126,126,121,125,29,7,42,68,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,88,122,96,108,88,114,96,114,71,114,
+ 71,96,96,108,108,108,88,96,88,108,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,68,88,109,120,114,119,120,126,
+ 126,126,126,126,126,126,126,97,124,126,126,126,120,120,120,109,
+ 109,120,103,124,126,126,126,126,121,7,17,42,71,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,71,71,88,71,71,71,88,71,71,71,
+ 71,71,88,71,71,88,71,71,88,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,53,17,7,19,29,42,53,
+ 71,109,100,109,120,120,124,94,104,126,126,126,126,126,126,126,
+ 126,126,101,126,126,126,126,126,124,8,19,53,71,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,68,53,42,29,29,19,19,
+ 7,7,7,7,19,29,53,38,97,97,124,126,126,126,126,126,
+ 126,126,101,126,126,126,126,126,96,7,29,53,71,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,68,68,68,53,53,
+ 53,42,42,29,29,29,19,19,7,17,8,29,42,53,77,109,
+ 120,122,103,126,126,126,126,120,19,7,29,53,71,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,71,68,68,71,71,71,68,68,71,71,
+ 71,71,71,71,71,53,71,68,71,71,53,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,68,68,68,68,53,53,53,42,42,29,29,29,19,7,7,
+ 7,7,29,42,68,71,53,7,7,19,42,68,71,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,29,71,68,71,68,71,68,42,71,68,
+ 71,68,68,71,71,53,42,53,71,71,42,71,71,71,71,68,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,68,68,68,53,53,53,42,
+ 42,29,29,19,19,7,7,19,19,29,53,71,71,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,19,71,53,42,68,42,53,29,53,53,
+ 42,42,42,42,71,68,53,29,42,42,42,42,42,42,29,53,
+ 53,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,68,
+ 68,68,68,53,53,53,42,42,53,53,71,71,71,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,53,53,53,53,68,53,53,53,53,53,
+ 53,53,68,53,71,53,71,53,53,68,53,53,53,53,53,68,
+ 53,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,71,
+ 71,71,71,71
+ };
trunk/bios/icons.h
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/bios/encoding.txt
===================================================================
--- trunk/bios/encoding.txt (nonexistent)
+++ trunk/bios/encoding.txt (revision 2)
@@ -0,0 +1,2 @@
+abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ
+0123456789.,!?_#'"/\-:()
trunk/bios/encoding.txt
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/bios/makefont.c
===================================================================
--- trunk/bios/makefont.c (nonexistent)
+++ trunk/bios/makefont.c (revision 2)
@@ -0,0 +1,130 @@
+#include
+#include "zfont2.h"
+
+unsigned char data[128][10];
+
+void calculateKeyboard(char *output, char *line1, int s)
+{
+ int x=0;
+ int g=0;
+ int h=s/2;
+ int sections=1;
+ char *sep=strchr(line1,'|');
+ while (sep)
+ {
+ sections+=2;
+ sep=strchr(sep+1,'|');
+ }
+ g=120-sections*h+h;
+ while (*line1)
+ {
+ int w=0;
+ sep=line1;
+ if (*sep=='|')
+ w=data['|'][0];
+ else
+ {
+ while (*sep!='|' && *sep!='\0')
+ {
+ w+=data[*sep][0];
+ sep++;
+ }
+ }
+ while (x+w/2
trunk/bios/makefont.c
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/bios/compressicon.c
===================================================================
--- trunk/bios/compressicon.c (nonexistent)
+++ trunk/bios/compressicon.c (revision 2)
@@ -0,0 +1,67 @@
+#include
+#include
+#include
+#include "icons.h"
+
+#define MIN(a,b) ((a)<(b)?(a):(b))
+
+int main(int argc, char **argv)
+{
+ char *data=malloc(sizeof(header_data));
+ char *p=data;
+ FILE *f;
+ int i=0;
+ while (i<320)
+ {
+ *(p++)=0;
+ *(p++)=(45.0f*sinf((3.14f/2.0f)*(160.0f-fabsf(160.0f-i))/160.0f));
+ i++;
+ }
+ i=0;
+ while (i<128)
+ {
+ unsigned char r=header_data_cmap[i][0];
+ unsigned char g=header_data_cmap[i][1];
+ unsigned char b=header_data_cmap[i][2];
+ *(p++)=(r&0xF8)|(g>>5);
+ *(p++)=((g<<3)&0xE0)|(b>>3);
+ i++;
+ }
+ i=0;
+ while (i
trunk/bios/compressicon.c
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/bios/combinedata.c
===================================================================
--- trunk/bios/combinedata.c (nonexistent)
+++ trunk/bios/combinedata.c (revision 2)
@@ -0,0 +1,52 @@
+#include
+
+unsigned char data[512*1024]={0};
+
+#define CALLBACK_BASE 0x1E58B
+#define INIT_CALLBACK CALLBACK_BASE+0
+#define PRINT_CALLBACK CALLBACK_BASE+5
+#define PRINTCHAR_CALLBACK CALLBACK_BASE+10
+#define PRINTNUM_CALLBACK CALLBACK_BASE+15
+#define READ_CALLBACK CALLBACK_BASE+20
+
+char* roms[4]={
+ "Zork1.z3",
+ "hhgg.z3",
+ "curses.z3",
+ "Planetfa.z3"
+};
+
+int main()
+{
+ FILE *f;
+ int i;
+ for (i=0; i<4; i++)
+ {
+ f=fopen(roms[i], "rb");
+ fread(&data[0x20000*i], 1, 0x20000, f);
+ fclose(f);
+ if (i==0)
+ {
+ f=fopen("icons.dat", "rb");
+ fread(&data[0x20000*i+0x16800], 1, 30*1024, f);
+ fclose(f);
+ }
+ f=fopen("zfont.dat", "rb");
+ fread(&data[0x20000*i+0x1E000], 1, 0x500, f);
+ fclose(f);
+ f=fopen("encoding.txt", "rb");
+ fread(&data[0x20000*i+0x1E500], 1, 0x80, f);
+ fclose(f);
+ data[0x20000*i+0x1E57F]=i;
+ f=fopen("bios.z3", "rb");
+ fseek(f, 0x1E580, SEEK_SET);
+ fread(&data[0x20000*i+0x1E580], 1, 0x20000-0x1E580, f);
+ fclose(f);
+ }
+
+ f=fopen("rom.z3","wb");
+ fwrite(data, 1, sizeof(data), f);
+ fclose(f);
+
+ printf("Done\n");
+}
trunk/bios/combinedata.c
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/bios/bios.inf
===================================================================
--- trunk/bios/bios.inf (nonexistent)
+++ trunk/bios/bios.inf (revision 2)
@@ -0,0 +1,1626 @@
+Constant Border = 4;
+Constant ScrWidth = 240-Border+1;
+Constant ScrHeight = 320;
+Constant BufferHeight = 630;
+Constant LineStride = 30;
+Constant LineStrideW = 15;
+Constant TextHeight = 10;
+Constant KeyboardStartLine = 240;
+Constant KeyboardTextSpacing = 20;
+Constant KeyboardSepSpacing = 5;
+Constant StatusHeight = 10;
+! S c o r e : 0 M o v e s : 0
+Constant ScoreXOffset = (240-Border-(4+5+4+3+4+2+4)-(3+6+4+4+4+4+2+4));
+
+Constant DictionaryOff = 8;
+
+Constant cAbbrev = 0;
+Constant cEncodO = 2;
+Constant cEncodB = 4;
+Constant cScrenX = 8;
+Constant cScrenY = 10;
+Constant cFontO = 12;
+Constant cFontB = 14;
+Constant cBitMpW = 16;
+Constant cScroll = 18;
+Constant cTextO = 20;
+Constant cTextB = 22;
+Constant cTextSep = 24;
+Constant cScrollStart = 26;
+Constant cLastScroll = 28;
+Constant MaxTextSize = $400;
+
+Constant contextW = $D800; ! $1B000
+
+! Code should start at $1E580
+! Font + Encoding data is directly before
+! Game must be smaller than $1E000 (120Kb) to fit in BIOS
+
+! BitMap buffer is at the top of the high RAM (18.75Kb)
+! Scratch buffer is below the bitmap and is 1Kb
+! Context structure is just below that
+! Bottom of high RAM are the stacks growing up
+
+[Main;
+! Used as a table to pick out addresses of functions
+SysInit();
+SysPrint();
+SysPrintChar();
+SysPrintNum();
+SysRead();
+SysShowStatus();
+SysQuit();
+SysException();
+];
+
+[DecodeText
+inputO inputW !inputs
+outputO outputB encodingO encodingB alpha charWord c char charOut; !temps
+
+outputO=cTextO==>contextW;
+outputB=cTextB==>contextW;
+encodingO=cEncodO==>contextW;
+encodingB=cEncodB==>contextW;
+do
+{
+ charWord = inputO-->inputW;
+ for (c=0: c<3: c++)
+ {
+ if (c==0) char=(charWord&$7c00)/1024;
+ else if (c==1) char=(charWord&$3e0)/32;
+ else char=charWord&31;
+
+ charOut=-1;
+ if (alpha<=2 && char<=5)
+ {
+ if (char==0)
+ {
+ charOut=' ';
+ alpha=0;
+ }
+ else
+ {
+ if (char>=4)
+ alpha=char-3;
+ else
+ alpha=char+2;
+ }
+ }
+ else if (char==6 && alpha==2)
+ {
+ alpha=6;
+ }
+ else
+ {
+ if (alpha<=2)
+ {
+ charOut=encodingO->(encodingB+26*alpha+char-6);
+ alpha=0;
+ }
+ else if (alpha<=5)
+ {
+ cTextB==>contextW=outputB;
+ outputB=DecodeText(0, (cAbbrev==>contextW)-->(32*(alpha-3)+char));
+ outputB=cTextB==>contextW;
+ alpha=0;
+ }
+ else if (alpha==6)
+ {
+ alpha=32+char*32;
+ }
+ else
+ {
+ charOut=(alpha-32)|char;
+ alpha=0;
+ }
+ }
+ if (charOut>=0)
+ {
+ outputO=>outputB=charOut;
+ outputB++;
+ }
+ }
+ inputW++;
+}
+until (charWord<0);
+outputO=>outputB=0;
+
+cTextB==>contextW=outputB;
+];
+
+[TextToConsole
+textO textB
+fontO fontB bitmapW char ptr a b w x y checkWrap firstLine;
+
+x=cScrenX==>contextW;
+y=cScrenY==>contextW;
+fontO=cFontO==>contextW;
+fontB=cFontB==>contextW;
+bitmapW=cBitMpW==>contextW;
+char=textO=>textB;
+if (x~=Border)
+ checkWrap=1;
+firstLine=1;
+while (char)
+{
+ if (char<=32)
+ {
+ checkWrap=1;
+ }
+ else if (checkWrap)
+ {
+ w=x;
+ ptr=textB;
+ while (textO=>ptr>32)
+ {
+ w=w+(fontO->(fontB+(textO=>ptr)*TextHeight));
+ ptr++;
+ }
+ if (w>ScrWidth)
+ {
+ x=ScrWidth+1;
+ }
+ checkWrap=0;
+ }
+ ptr=fontB+char*TextHeight;
+ w=fontO->ptr;
+ if (x+w>ScrWidth || char==10)
+ {
+ y=y+TextHeight;
+ if (y>BufferHeight-TextHeight)
+ y=0;
+ if (y>(KeyboardStartLine-StatusHeight)-TextHeight || cScrollStart==>contextW)
+ {
+ cScrollStart==>contextW=1;
+ a=cScroll==>contextW;
+ a=a+TextHeight;
+ if (a>=BufferHeight)
+ a=0;
+ if (a==cLastScroll==>contextW)
+ {
+ cScrenY==>contextW=y;
+ b=UpdateScreen();
+ b=GetKey();
+ }
+ cScroll==>contextW=a;
+ }
+ a=y*LineStride;
+ b=LineStride*5;
+ while (b)
+ {
+ a==>bitmapW=0;
+ a=a+2;
+ b--;
+ }
+ x=Border;
+ firstLine=0;
+ }
+ if (x~=Border || char>32 || firstLine)
+ {
+ if (cTextSep==>contextW==0 || char==124)
+ {
+ a=7-(x&7);
+ b=2;
+ while (a)
+ {
+ b=b*2;
+ a--;
+ }
+ a=x/8+y*LineStride;
+ for (char=0: char<9: char++)
+ {
+ ptr++;
+ a==>bitmapW=(a==>bitmapW)|((fontO->ptr)*b);
+ a=a+LineStride;
+ }
+ }
+ x=x+w;
+ }
+ textB++;
+ char=textO=>textB;
+}
+cScrenX==>contextW=x;
+cScrenY==>contextW=y;
+];
+
+[UpdateScreen
+ptr size sizeW bitmapW scroll; !temps
+ bitmapW=cBitMpW==>contextW;
+ scroll=cScroll==>contextW;
+ cLastScroll==>contextW=cScrenY==>contextW;
+ @write_reg 32 0;
+ @write_reg 33 0;
+ @blit1 0 LineStride $49c2 $49c2;
+ @write_reg 32 0;
+ @write_reg 33 1;
+ ptr=bitmapW+(LineStrideW*BufferHeight);
+ sizeW=LineStride*(StatusHeight-1);
+ @blit1 ptr sizeW $49c2 $ff14;
+ @write_reg 32 0;
+ @write_reg 33 StatusHeight;
+ ptr=bitmapW+(LineStrideW*scroll);
+ sizeW=LineStride*(KeyboardStartLine-StatusHeight);
+ if (scroll > BufferHeight - (KeyboardStartLine-StatusHeight))
+ {
+ size=BufferHeight-scroll;
+ sizeW=LineStride*size;
+ @blit1 ptr sizeW $ff14 $49c2;
+ ptr=bitmapW;
+ size=size+StatusHeight;
+ @write_reg 32 0;
+ @write_reg 33 size;
+ sizeW=LineStride*(KeyboardStartLine-size);
+ }
+ @blit1 ptr sizeW $ff14 $49c2;
+];
+
+[InitScreenRegs;
+@write_reg $0000 $0000; ! Setting primes the screen for data
+@write_reg $0001 $0100;
+@write_reg $0002 $0700;
+@write_reg $0003 $1030;
+@write_reg $0008 $0302;
+@write_reg $0009 $0000;
+@write_reg $000A $0008;
+@write_reg $0010 $0790;
+@write_reg $0011 $0005;
+@write_reg $0012 $0000;
+@write_reg $0013 $0000;
+@write_reg $0010 $12B0;
+@write_reg $0011 $0007;
+@write_reg $0012 $008C;
+@write_reg $0013 $1700;
+@write_reg $0029 $0022;
+@write_reg $0030 $0000;
+@write_reg $0031 $0505;
+@write_reg $0032 $0205;
+@write_reg $0035 $0206;
+@write_reg $0036 $0408;
+@write_reg $0037 $0000;
+@write_reg $0038 $0504;
+@write_reg $0039 $0206;
+@write_reg $003C $0206;
+@write_reg $003D $0408;
+@write_reg $0050 $0000;
+@write_reg $0051 $00EF;
+@write_reg $0052 $0000;
+@write_reg $0053 $013F;
+@write_reg $0060 $A700;
+@write_reg $0061 $0001;
+@write_reg $0090 $0033;
+@write_reg $0081 $0000;
+@write_reg $0082 $0000;
+@write_reg $0083 $013F;
+];
+
+[InitContext;
+cAbbrev==>contextW=$18-->0;
+cEncodO==>contextW=$FFFF; !1E500
+cEncodB==>contextW=$E501;
+cScrenX==>contextW=Border;
+!cScrenY==>contextW=0;
+cFontO ==>contextW=$FFFF; ! $1E000
+cFontB ==>contextW=$E001;
+cBitMpW==>contextW=-(BufferHeight+StatusHeight)*LineStrideW;
+!cScroll==>contextW=0;
+cTextO==>contextW=cBitMpW==>contextW;
+cTextB==>contextW=cBitMpW==>contextW-MaxTextSize;
+!cTextSep==>contextW=0;
+!cScrollStart==>contextW=0;
+!cLastScroll==>contextW=0;
+];
+
+[SysPrint
+printO printW;
+printW=DecodeText(printO&1, printW);
+if (printO&2) ! Doing print_ret so need to add a newline
+{
+ printO=cTextO==>contextW;
+ printW=cTextB==>contextW;
+ printO=>printW=10;
+ printW++;
+ printO=>printW=0;
+}
+cTextB==>contextW=(cBitMpW==>contextW-MaxTextSize); ! reset buffer as Decode modifies it
+printW=TextToConsole(cTextO==>contextW, cTextB==>contextW);
+rtrue;
+];
+
+[SysPrintChar
+char
+textO textB;
+textO=cTextO==>contextW;
+textB=cTextB==>contextW;
+textO=>textB=char;
+textB++;
+textO=>textB=0;
+char=TextToConsole(textO, textB-1);
+rtrue;
+];
+
+[SysPrintNum
+num
+digit writeStarted demon temp;
+demon=10000;
+if (num<0)
+{
+ temp=SysPrintChar('-');
+ demon=-demon;
+}
+while (1)
+{
+ digit=num/demon;
+ if (digit<0) ! Not sure why I need to do this but fixes -32678
+ digit=-digit;
+ if (digit>0 || writeStarted || demon==1 || demon==-1)
+ {
+ temp=SysPrintChar('0'+digit);
+ writeStarted=1;
+ if (demon==1 || demon==-1)
+ break;
+ }
+ num=num-digit*demon;
+ demon=demon/10;
+}
+rtrue;
+];
+
+[DrawKeyboard
+size ptr line i;
+! Use print system to make keyboard bitmaps then blit to screen
+for (: i<2: i++)
+{
+ cTextSep==>contextW=i;
+ @print " q | w | e | r | t | y | u | i | o | p^";
+ @print " a | s | d | f | g | h | j | k | l^";
+ @print " z | x | c | v | b | n | m | ,^";
+ @print " Delete | Space | Enter^";
+}
+cTextSep==>contextW=0;
+@write_reg 32 0;
+size=LineStride*TextHeight;
+ptr=cBitMpW==>contextW;
+i=ptr+4*TextHeight*LineStrideW;
+line=KeyboardStartLine+1+KeyboardSepSpacing;
+while (ptrcontextW;
+i=ptr+LineStrideW*TextHeight*8;
+while (ptrptr=0;
+ ptr++;
+}
+cScrenY==>contextW=0;
+];
+
+[GameSelect
+palette
+imageO imageP c col count p x y winW winWMax;
+
+c=320;
+@write_reg 32 0;
+@write_reg 33 0;
+while (c)
+{
+ p=240;
+ while (p)
+ {
+ col=0;
+ if ((c+p)&7 && (c-p)&7)
+ col=((0-->(palette+c))*(0-->(palette+(p+40))))&$7E0;
+ p--;
+ @write_reg 34 col;
+ }
+ c--;
+ y++;
+}
+palette=palette+320;
+
+imageP=(palette+128)*2+1;
+imageO=$FFFF;
+p=4;
+while (p)
+{
+ winW=127;
+ winWMax=226;
+ if (p&1)
+ {
+ winW=13;
+ winWMax=112;
+ }
+ y=167;
+ if (p&2)
+ {
+ y=33;
+ }
+ @write_reg $0050 winW;
+ @write_reg $0051 winWMax;
+ @write_reg 32 winW;
+ @write_reg 33 y;
+ count=100*120;
+ while (count>0)
+ {
+ c=imageO->imageP;
+ col=0-->(palette+(c&$7F));
+ imageP++;
+ if (c&$80)
+ {
+ c=imageO->imageP;
+ imageP++;
+ count=count-c;
+ while (c)
+ {
+ @write_reg 34 col;
+ c--;
+ }
+ }
+ else
+ {
+ @write_reg 34 col;
+ count--;
+ }
+ }
+ p--;
+}
+@write_reg $0050 $0000;
+@write_reg $0051 $00EF;
+@write_reg $0007 $0133; ! Turn on the screen
+
+do !wait for touch
+{
+ @get_touch $93 -> x;
+ if (x<1000)
+ y--;
+ else
+ y=10;
+} until (y==0);
+@get_touch $95 -> x; ! x
+@get_touch $1A -> y; ! y
+p=0;
+if (x>510)
+ p++;
+if (y>508)
+ p=p+2;
+
+if (p>0)
+ @switch_bank p;
+];
+
+[MandelbrotPalette;
+@db 0;
+@db $f800; @db $fb80; @db $f800; @db $fc00;
+@db $f800; @db $fc00; @db $f800; @db $fc00;
+@db $ff80; @db $df80; @db $bf80; @db $9f80;
+@db $7f80; @db $5f80; @db $3f80; @db $1f80;
+@db $1f81; @db $1f85; @db $1f89; @db $1f8d;
+@db $1f91; @db $1f96; @db $1f9a; @db $1f9e;
+@db $1d9f; @db $191f; @db $151f; @db $111f;
+@db $0d1f; @db $091f; @db $049f; @db $009f;
+];
+
+[Mandelbrot
+x y xtemp ytemp x0 y0 x00 y00 i;
+@write_reg 32 0;
+@write_reg 33 StatusHeight;
+y0=60;
+do
+{
+ x0=-120;
+ y00=y0*40;
+ do
+ {
+ xtemp=0;
+ ytemp=0;
+ y=0;
+ i=0;
+ x00=x0*20;
+ do
+ {
+ i++;
+ xtemp=xtemp-ytemp+y00;
+ y=(x*y+x00)/32;
+ if (y>=2*64 || y<=-2*64)
+ break;
+ x=xtemp/64;
+ if (x>=2*64 || x<=-2*64)
+ break;
+ xtemp=x*x;
+ ytemp=y*y;
+ } until ( xtemp + ytemp >= 64*64*4 || i>=32);
+ i=1-->(i+MandelbrotPalette);
+ @write_reg 34 i;
+ x0++;
+ } until (x0>=120);
+ y0--;
+} until (y0==60-(KeyboardStartLine-StatusHeight));
+@write_reg $0007 $0133; ! Turn on the screen
+x=GetKey();
+];
+
+[SpaceInvadersSpriteData;
+!squid1 Offset:0
+@db 8*(8|16);
+@db 8*(4|8|16|32);
+@db 8*(2|4|8|16|32|64);
+@db 8*(1|2|8|16|64|128);
+@db 8*(1|2|4|8|16|32|64|128);
+@db 8*(4|32);
+@db 8*(2|8|16|64);
+@db 8*(1|4|32|128);
+!ant1 Offset:16
+@db 4*(4|256);
+@db 4*(8|128);
+@db 4*(4|8|16|32|64|128|256);
+@db 4*(2|4|16|32|64|256|512);
+@db 4*(1|2|4|8|16|32|64|128|256|512|1024);
+@db 4*(1|4|8|16|32|64|128|256|1024);
+@db 4*(1|4|256|1024);
+@db 4*(8|16|64|128);
+!bug1 Offset:32
+@db 4*(16|32|64|128);
+@db 4*(2|4|8|16|32|64|128|256|512|1024);
+@db 4*(1|2|4|8|16|32|64|128|256|512|1024|2048);
+@db 4*(1|2|4|32|64|512|1024|2048);
+@db 4*(1|2|4|8|16|32|64|128|256|512|1024|2048);
+@db 4*(4|8|16|128|256|512);
+@db 4*(2|4|32|64|512|1024);
+@db 4*(4|8|256|512);
+!squid2 Offset:48
+@db 8*(8|16);
+@db 8*(4|8|16|32);
+@db 8*(2|4|8|16|32|64);
+@db 8*(1|2|8|16|64|128);
+@db 8*(1|2|4|8|16|32|64|128);
+@db 8*(2|8|16|64);
+@db 8*(1|128);
+@db 8*(2|64);
+!ant2 Offset:64
+@db 4*(4|256);
+@db 4*(1|8|128|1024);
+@db 4*(1|4|8|16|32|64|128|256|1024);
+@db 4*(1|2|4|16|32|64|256|512|1024);
+@db 4*(1|2|4|8|16|32|64|128|256|512|1024);
+@db 4*(2|4|8|16|32|64|128|256|512);
+@db 4*(4|256);
+@db 4*(2|512);
+!bug2 Offset:80
+@db 4*(16|32|64|128);
+@db 4*(2|4|8|16|32|64|128|256|512|1024);
+@db 4*(1|2|4|8|16|32|64|128|256|512|1024|2048);
+@db 4*(1|2|4|32|64|512|1024|2048);
+@db 4*(1|2|4|8|16|32|64|128|256|512|1024|2048);
+@db 4*(8|16|128|256);
+@db 4*(4|8|32|64|256|512);
+@db 4*(1|2|1024|2048);
+!ship Offset:96
+@db 2*(64);
+@db 2*(32|64|128);
+@db 2*(32|64|128);
+@db 2*(2|4|8|16|32|64|128|256|512|1024|2048);
+@db 2*(1|2|4|8|16|32|64|128|256|512|1024|2048|4096);
+@db 2*(1|2|4|8|16|32|64|128|256|512|1024|2048|4096);
+@db 2*(1|2|4|8|16|32|64|128|256|512|1024|2048|4096);
+@db 2*(1|2|4|8|16|32|64|128|256|512|1024|2048|4096);
+!explosion Offset:112
+@db 4*(16|256);
+@db 4*(2|32|128|2048);
+@db 4*(4|1024);
+@db 4*(8|512);
+@db 4*(1|2|2048); !|4096); Should really but messes with clear
+@db 4*(8|512);
+@db 4*(4|32|128|1024);
+@db 4*(2|16|256|2048);
+!ufo Offset:128
+@db $03c0;
+@db $0ff0;
+@db $3ffc;
+@db $6db6;
+@db $ffff;
+@db $3bdc;
+@db $1008;
+!base Offset:142
+@db $0FFF; @db $C000;
+@db $1FFF; @db $E000;
+@db $3FFF; @db $F000;
+@db $7FFF; @db $F800;
+@db $FFFF; @db $FC00;
+@db $FFFF; @db $FC00;
+@db $FFFF; @db $FC00;
+@db $FFFF; @db $FC00;
+@db $FFFF; @db $FC00;
+@db $FFFF; @db $FC00;
+@db $FFFF; @db $FC00;
+@db $FFFF; @db $FC00;
+@db $FE03; @db $FC00;
+@db $FC01; @db $FC00;
+@db $F800; @db $FC00;
+@db $F800; @db $FC00;
+];
+
+[SpaceInvaders
+sprites x y i k s xd u c sx t p l ud j;
+
+!copy sprite data
+sprites=contextW-MaxTextSize/2;
+i=SpaceInvadersSpriteData;
+k=i+71;
+c=sprites;
+while (ic=1-->i;
+ i++;
+ c++;
+}
+!copy 4x bases as will get destroyed
+c=sprites+135;
+k=i+32;
+t=4;
+while (t)
+{
+ i=SpaceInvadersSpriteData+71;
+ while (ic=1-->i;
+ i++;
+ c++;
+ }
+ t--;
+}
+
+s=sprites;
+k=s+5;
+t=26;
+c=234;
+while (ss=0; !dying
+ 200==>s=8; !startX
+ 210==>s=t; !startY
+ 220==>s=$7ff; !alive
+ c==>sprites=0; !bullet direction
+ s++;
+ t=t+16;
+ c=c+6;
+}
+184==>sprites=0; ! dead timer
+186==>sprites=0; ! ufo dead timer
+188==>sprites=255; ! ufo position
+
+@write_reg $0050 0;
+@write_reg $0051 $EF;
+@write_reg 32 0;
+@write_reg 33 StatusHeight;
+@blit1 0 6900 0 0;
+xd=2;
+sx=115;
+l=3;
+ud=1;
+while (1)
+{
+ if (random(50)==1 && ~~184==>sprites)
+ {
+ x=random(11)-1;
+ k=x;
+ t=1;
+ while (k)
+ {
+ t=t*2;
+ k--;
+ }
+ s=228;
+ y=0;
+ for (k=0: k<5: k++)
+ {
+ if (s==>sprites&t)
+ {
+ x=(s-20)==>sprites+16*x;
+ y=(s-10)==>sprites+6;
+ break;
+ }
+ s=s-2;
+ }
+ if (y)
+ {
+ for (k=0: k<4: k++)
+ {
+ s=236+6*k;
+ if (~~(s+4)==>sprites)
+ {
+ s==>sprites=x+8;
+ (s+2)==>sprites=y;
+ (s+4)==>sprites=1;
+ break;
+ }
+ }
+ }
+ }
+
+ x=188==>sprites;
+ if (x<240-16)
+ {
+ i=2;
+ while (i)
+ {
+ @write_reg $0050 x;
+ @write_reg 32 x;
+ y=x+15;
+ @write_reg $0051 y;
+ @write_reg 33 16;
+ i--;
+ if (i)
+ {
+ @blit1 0 14 0 0;
+ x++;
+ 188==>sprites=x;
+ if (x>=240-16)
+ break;
+ }
+ else
+ {
+ s=sprites+64;
+ x=186==>sprites;
+ k=$f800;
+ if (x)
+ {
+ s=sprites+56;
+ x--;
+ 186==>sprites=x;
+ if (~~x)
+ {
+ 188==>sprites=255;
+ k=0;
+ }
+ }
+ @blit1 s 14 0 k;
+ }
+ }
+ }
+ else if (random(2000)==1)
+ {
+ 188==>sprites=0;
+ }
+
+ ud--;
+ if (ud<=0)
+ {
+ k=0;
+ do
+ {
+ u--;
+ if (u<0)
+ u=4;
+ x=220==>(sprites+u);
+ k++;
+ } until (x || k>5);
+ if (~~x)
+ {
+ break; ! all enemies dead
+ }
+ if (x&$007)
+ ud++;
+ if (x&$038)
+ ud++;
+ if (x&$1C0)
+ ud++;
+ if (x&$600)
+ ud++;
+ k=5;
+ while (k)
+ {
+ k--;
+ t=220==>(sprites+k);
+ if (t)
+ j=k;
+ x=x|t;
+ }
+ k=x;
+ c=8;
+ while (~~(x&1))
+ {
+ c=c-16;
+ x=x/2;
+ }
+ t=54;
+ while (~~(k&$400))
+ {
+ k=k*2;
+ t=t+16;
+ }
+ x=200==>(sprites+u)+xd;
+ if (x>t || x(sprites+u);
+ @write_reg $0050 0;
+ @write_reg $0051 $EF;
+ @write_reg 32 0;
+ @write_reg 33 y;
+ @blit1 0 240 0 0;
+ 210==>(sprites+u)=y+10;
+ if (u==j)
+ xd=-xd;
+ if (y>202) !touched the ship row
+ {
+ if (~~184==>sprites)
+ {
+ 184==>sprites=150;
+ }
+ l=0;
+ }
+ }
+ 200==>(sprites+u)=x;
+ }
+ s=sprites+135;
+ for (k=0: k<4: k++)
+ {
+ x=35+k*50;
+ @write_reg $50 x;
+ @write_reg 32 x;
+ x=x+31;
+ @write_reg $51 x;
+ @write_reg 33 195;
+ @blit1 s 64 0 $7E0;
+ s=s+32;
+ }
+ for (k=0: k<5: k++)
+ {
+ x=200==>(sprites+k);
+ y=210==>(sprites+k);
+ p=220==>(sprites+k);
+ c=y/8;
+ c=(31-c)*32*64+c+1;
+ s=sprites;
+ if (x&2)
+ s=s+24;
+ if (k>=3)
+ s=s+16;
+ else if (k>=1)
+ s=s+8;
+ t=1;
+ for (i=0: i<11: i++)
+ {
+ @write_reg $0050 x;
+ @write_reg 32 x;
+ @write_reg 33 y;
+ x=x+15;
+ @write_reg $0051 x;
+ x++;
+ if (p&t)
+ {
+ j=190==>(sprites+k);
+ if (j&t)
+ {
+ if (j&$8000)
+ {
+ @blit1 s 16 0 0;
+ p=p&~t;
+ }
+ else
+ {
+ j=sprites+56;
+ @blit1 j 16 0 c;
+ }
+ }
+ else
+ {
+ @blit1 s 16 0 c;
+ }
+ }
+ t=t*2;
+ }
+ 220==>(sprites+k)=p;
+ p=190==>(sprites+k);
+ if (p)
+ {
+ if (p&$8000)
+ p=0;
+ else
+ p=p+$1000;
+ 190==>(sprites+k)=p;
+ }
+ }
+ s=230;
+ for (j=-3: j<5: j++)
+ {
+ k=j;
+ if (k<0)
+ k=0;
+ x=(s+4)==>sprites;
+ if (x)
+ {
+ y=(s+2)==>sprites;
+ i=s==>sprites;
+ @write_reg 32 i;
+ @write_reg $50 i;
+ @write_reg $51 i;
+ @write_reg 33 y;
+ @write_reg 34 0;
+ @write_reg 34 0;
+ if (x>0)
+ {
+ @write_reg 34 0;
+ @write_reg 34 0;
+ }
+ if (k) !not player bullet
+ {
+ if (234==>sprites)
+ {
+ t=232==>sprites;
+ if (y<=t && y+4>=t)
+ {
+ t=230==>sprites;
+ if (i<=t+2 && i>=t-2)
+ {
+ ! two bullets hit
+ 234==>sprites=0;
+ (s+4)==>sprites=0;
+ ! erase player bullet
+ @write_reg 32 t;
+ @write_reg $50 t;
+ @write_reg $51 t;
+ t=232==>sprites;
+ @write_reg 33 t;
+ @write_reg 34 0;
+ @write_reg 34 0;
+ }
+ }
+ }
+ }
+ y=y+x;
+ (s+2)==>sprites=y;
+ if (x<0)
+ {
+ for (i=0: i<5: i++)
+ {
+ t=210==>(sprites+i);
+ if (y>=t && ysprites-200==>(sprites+i);
+ if (t>=0 && t<11*16)
+ {
+ p=1;
+ c=0;
+ while (t>16)
+ {
+ p=p*2;
+ t=t-16;
+ c=c+16;
+ }
+ if (t>2 && t<14)
+ {
+ if (220==>(sprites+i)&p)
+ {
+ 190==>(sprites+i)=190==>(sprites+i)|p;
+ (s+4)==>sprites=0;
+ p=200==>(sprites+i)+c;
+ t=210==>(sprites+i);
+ break;
+ }
+ }
+ }
+ }
+ }
+ }
+ if (y>=195 && y<195+16)
+ {
+ t=s==>sprites-35;
+ if (t>=0 && t<4*50)
+ {
+ i=t/50;
+ t=t-i*50;
+ if (t<22)
+ {
+ k=(270-1)+(64*i)+(4*(y-195));
+ t=t+7;
+ while (t>=12)
+ {
+ t=t-8;
+ k=k++;
+ }
+ p=1;
+ t=15-t;
+ while (t)
+ {
+ t--;
+ p=p*2;
+ }
+ t=k==>sprites;
+ if (t&p)
+ {
+ p=p/8;
+ k==>sprites=t&~(p*(1|2|4|8|16));
+ if (y<195+15)
+ (k+4)==>sprites=(k+4)==>sprites&~(p*(2|4|8));
+ if (y<195+14)
+ (k+8)==>sprites=(k+8)==>sprites&~(p*(1|4|16));
+ if (y>195)
+ (k-4)==>sprites=(k-4)==>sprites&~(p*(2|4|8));
+ if (y>196)
+ (k-8)==>sprites=(k-8)==>sprites&~(p*(1|4|16));
+ (s+4)==>sprites=0;
+ }
+ }
+ }
+ }
+ i=s==>sprites;
+ if (x>0 && i>sx+1 && i=220 && y<217+8)
+ {
+ (s+4)==>sprites=0;
+ if (~~184==>sprites)
+ {
+ 184==>sprites=150;
+ l--;
+ }
+ }
+ ;
+ if (x<0 && y>=16 && y<=16+7 && i>=188==>sprites && i<188==>sprites+16)
+ {
+ (s+4)==>sprites=0;
+ 186==>sprites=8;
+ }
+ if (y>=217+8 || y<=StatusHeight)
+ (s+4)==>sprites=0;
+ if ((s+4)==>sprites)
+ {
+ @write_reg 33 y;
+ @write_reg 34 $7E0;
+ @write_reg 34 $7E0;
+ if (x>0)
+ {
+ @write_reg 34 $7E0;
+ @write_reg 34 $7E0;
+ }
+ }
+ }
+ if (j>=0)
+ s=s+6;
+ }
+ if (~~184==>sprites)
+ {
+ @get_touch $93 -> x;
+ if (x<1000)
+ {
+ @get_touch $1A -> y; ! y
+ if (y<720)
+ {
+ @get_touch $95 -> x; ! x
+ x=x-90;
+ x=x*2;
+ x=x/7;
+ x=x-7;
+ if (x>sx)
+ sx++;
+ else
+ sx--;
+ }
+ else if (234==>sprites==0)
+ {
+ 230==>sprites=sx+8;
+ 232==>sprites=217;
+ 234==>sprites=-1;
+ }
+ }
+ }
+ @write_reg $0050 sx;
+ @write_reg 32 sx;
+ x=sx+15;
+ @write_reg $0051 x;
+ @write_reg 33 218;
+ s=sprites+96/2;
+ k=$7e0;
+ if (184==>sprites)
+ {
+ if (184==>sprites>130)
+ s=sprites+56;
+ else
+ k=0;
+ 184==>sprites=184==>sprites-1;
+ }
+ else if (l<=0)
+ {
+ break;
+ }
+ @blit1 s 16 0 k;
+
+ s=sprites+96/2;
+ for (k=0: k<3: k++)
+ {
+ x=10+16*k;
+ @write_reg $0050 x;
+ @write_reg 32 x;
+ x=x+15;
+ @write_reg $0051 x;
+ @write_reg 33 230;
+ if (k0)
+ @print "You win!^^";
+else
+ @print "Bad luck.^^";
+];
+
+[SysInit
+temp;
+temp=InitContext();
+temp=InitScreenRegs();
+if (~~($FFFF->$E580))
+ temp=GameSelect($B400);
+temp=DrawKeyboard();
+@new_line;
+! Set flags
+0->1=(0->1&$8f)|$40; ! Status line availible, Screen splitting not availible + variable pitch font
+0->10=0->10&$fe; ! Transcripting off
+];
+
+[FindInDictionary
+text parse
+encodingO encodingB textO textB count limit i a c;
+
+encodingO=cEncodO==>contextW;
+textO=cTextO==>contextW;
+textB=cTextB==>contextW;
+limit=textB+6;
+
+i=6;
+while (i)
+{
+ i--;
+ textO=>(textB+i)=5;
+}
+
+count=parse->2;
+text=text+parse->3;
+
+while (count && textB0;
+ text++;
+ count--;
+ if (c==32)
+ {
+ textO=>textB=0;
+ textB++;
+ }
+ else
+ {
+ i=0;
+ a=3;
+ encodingB=cEncodB==>contextW;
+ while (c~=encodingO->encodingB)
+ {
+ encodingB++;
+ i++;
+ if (i==26)
+ {
+ if (a==5)
+ break;
+ a++;
+ i=0;
+ }
+ }
+ if (a>3)
+ {
+ textO=>textB=a;
+ textB++;
+ }
+ if (i==26)
+ {
+ textO=>textB=6;
+ textB++;
+ textO=>textB=(c/32)&$1F;
+ textB++;
+ textO=>textB=c&$1F;
+ textB++;
+ }
+ else
+ {
+ textO=>textB=6+i;
+ textB++;
+ }
+ }
+}
+
+textB=cTextB==>contextW;
+a=textO=>(textB )*1024+textO=>(textB+1)*32+textO=>(textB+2);
+c=textO=>(textB+3)*1024+textO=>(textB+4)*32+textO=>(textB+5);
+c=c|$8000;
+
+i=DictionaryOff-->0;
+parse-->0=0;
+i=i+i->0+1;
+limit=i->0;
+i++;
+count=i-->0;
+i=i+2;
+while (count)
+{
+ if (i-->0==a && i-->1==c)
+ {
+ parse-->0=i;
+ break;
+ }
+ i=i+limit;
+ count--;
+}
+];
+
+[UpdateStatus
+x y scoreX;
+
+x=cBitMpW==>contextW+BufferHeight*LineStrideW;
+y=StatusHeight*LineStrideW;
+while (y)
+{
+ 0==>x=0;
+ x++;
+ y--;
+}
+
+x=cScrenX==>contextW;
+y=cScrenY==>contextW;
+cScrenX==>contextW=Border;
+cScrenY==>contextW=BufferHeight;
+@print_obj sys__glob0;
+scoreX=ScoreXOffset;
+if (sys__glob1>=1000)
+ scoreX=scoreX-12;
+else if (sys__glob1>=100)
+ scoreX=scoreX-8;
+else if (sys__glob1>=10)
+ scoreX=scoreX-4;
+if (sys__glob2>=1000)
+ scoreX=scoreX-12;
+else if (sys__glob2>=100)
+ scoreX=scoreX-8;
+else if (sys__glob2>=10)
+ scoreX=scoreX-4;
+cScrenX==>contextW=scoreX;
+print "Score:";
+@print_num sys__glob1;
+print " Moves:";
+@print_num sys__glob2;
+cScrenX==>contextW=x;
+cScrenY==>contextW=y;
+];
+
+[GetKey
+x y
+startY scrollStart scrollLimit scroll;
+
+startY=1024;
+scrollLimit=cScroll==>contextW;
+while (1)
+{
+ scrollStart=scroll;
+ do !wait for not-touch
+ {
+ if (startY~=1024)
+ {
+ @get_touch $1A -> y; ! y
+ scroll=scrollStart+(startY-y)/3;
+ if (scroll>0)
+ {
+ scroll=0;
+ }
+ else if (scroll<-BufferHeight+KeyboardStartLine-StatusHeight)
+ {
+ scroll=-BufferHeight+KeyboardStartLine-StatusHeight;
+ }
+ cScroll==>contextW=((scrollLimit+scroll+BufferHeight+BufferHeight)%BufferHeight);
+ x=UpdateScreen();
+ }
+ @get_touch $93 -> x;
+ } until (x>=1000);
+ y=10;
+ do !wait for touch
+ {
+ @get_touch $93 -> x;
+ if (x<1000)
+ y--;
+ else
+ y=10;
+ } until (y==0);
+ startY=1024;
+ @get_touch $95 -> x; ! x
+ @get_touch $1A -> y; ! y
+ if (y>=870)
+ {
+ if (x<=374) ! delete
+ return 0;
+ if (x<=649)
+ return ' ';
+ return 10;
+ }
+ else if (y>=820)
+ {
+ if (x<=256)
+ return 'z';
+ if (x<=340)
+ return 'x';
+ if (x<=423)
+ return 'c';
+ if (x<=506)
+ return 'v';
+ if (x<=590)
+ return 'b';
+ if (x<=673)
+ return 'n';
+ if (x<=753)
+ return 'm';
+ return ',';
+ }
+ else if (y>=770)
+ {
+ if (x<=215)
+ return 'a';
+ if (x<=298)
+ return 's';
+ if (x<=381)
+ return 'd';
+ if (x<=465)
+ return 'f';
+ if (x<=548)
+ return 'g';
+ if (x<=631)
+ return 'h';
+ if (x<=711)
+ return 'j';
+ if (x<=794)
+ return 'k';
+ return 'l';
+ }
+ else if (y>=720)
+ {
+ if (x<=173)
+ return 'q';
+ if (x<=253)
+ return 'w';
+ if (x<=336)
+ return 'e';
+ if (x<=416)
+ return 'r';
+ if (x<=499)
+ return 't';
+ if (x<=583)
+ return 'y';
+ if (x<=666)
+ return 'u';
+ if (x<=753)
+ return 'i';
+ if (x<=836)
+ return 'o';
+ return 'p';
+ }
+ else
+ {
+ startY=y;
+ }
+}
+];
+
+[EraseConsole
+sx sy
+i ptr endptr bitmapW mask;
+
+bitmapW=cBitMpW==>contextW;
+
+i=sx;
+mask=1;
+while (sx&15)
+{
+ mask=mask*2;
+ sx++;
+}
+mask=~(mask-1);
+sx=i/16;
+
+while (sy<=cScrenY==>contextW)
+{
+ for (i=0: i<10: i++)
+ {
+ ptr=(sy+i)*LineStride+sx+sx;
+ endptr=(sy+i+1)*LineStride;
+ ptr==>bitmapW=ptr==>bitmapW&mask;
+ ptr=ptr+2;
+ while (ptrbitmapW=0;
+ ptr=ptr+2;
+ }
+ }
+ sx=0;
+ sy=sy+10;
+ mask=0;
+}
+];
+
+[SysRead
+parse text
+count i c sep dict readingWord parse0 temp;
+
+temp=UpdateStatus();
+temp=UpdateScreen();
+@write_reg $0007 $0133; ! Turn on the screen
+
+c=cScrenX==>contextW;
+sep=cScrenY==>contextW;
+dict=cScroll==>contextW;
+
+do
+{
+ i++;
+ text->i=GetKey();
+ if (text->i==0)
+ {
+ if (i>=2)
+ i=i-2;
+ else
+ i--;
+ }
+ temp=EraseConsole(c,sep);
+ cScrenX==>contextW=c;
+ cScrenY==>contextW=sep;
+ cScroll==>contextW=dict;
+ text->(i+1)=0;
+ temp=TextToConsole(1, text);
+ temp=UpdateScreen();
+} until (i && text->i==10);
+text->i=0;
+text->0=(i-1);
+
+i=0;
+if (text->0==10)
+{
+ if (text->1=='m' && text->2=='a' && text->3=='n' && text->4=='d' && text->5=='e'
+ && text->6=='l' && text->7=='b' && text->8=='r' && text->9=='o' && text->10=='t')
+ {
+ parse0=Mandelbrot();
+ i=1;
+ }
+}
+else if (text->0==6)
+{
+ if (text->1=='i' && text->2=='n' && text->3=='v' && text->4=='a' && text->5=='d' && text->6=='e')
+ {
+ parse0=SpaceInvaders();
+ i=1;
+ }
+}
+if (i)
+{
+ text->0=4;
+ text->1='w';
+ text->2='a';
+ text->3='i';
+ text->4='t';
+ text->5=0;
+}
+
+parse0=parse;
+parse++;
+parse++;
+
+parse0->1=0;
+count=text->0;
+i=1;
+while (count)
+{
+ c=text->i;
+ if (c==32)
+ {
+ sep=-1;
+ }
+ else
+ {
+ dict=DictionaryOff-->0;
+ sep=dict->0;
+ while (sep)
+ {
+ if (c==dict->sep)
+ break;
+ sep--;
+ }
+ }
+ if (readingWord)
+ {
+ if (sep)
+ {
+ parse0->1=parse0->1+1;
+ parse->2=i-parse->3;
+ temp=FindInDictionary(text, parse);
+ parse=parse+4;
+ readingWord=0;
+ if (sep>0)
+ {
+ parse->3=i;
+ readingWord=1;
+ }
+ }
+ }
+ else if (sep>=0)
+ {
+ parse->3=i;
+ readingWord=1;
+ }
+ count--;
+ i++;
+}
+if (readingWord)
+{
+ parse0->1=parse0->1+1;
+ parse->2=i-parse->3;
+ temp=FindInDictionary(text, parse);
+}
+];
+
+[SysShowStatus
+temp;
+temp=UpdateStatus();
+temp=UpdateScreen();
+];
+
+[PressKeyToReboot
+temp;
+@print "Press any key to reboot]";
+temp=UpdateScreen();
+temp=GetKey();
+@switch_bank 0;
+];
+
+[SysQuit
+temp;
+@print "^[Quit. ";
+temp=PressKeyToReboot();
+];
+
+[SysException
+pc oper0 oper1;
+@print "^[Exception PC/2=";
+@print_num pc;
+@print " OP0=";
+@print_num oper0;
+@print " OP1=";
+@print_num oper1;
+@print "]^[";
+pc=PressKeyToReboot();
+];
trunk/bios/bios.inf
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/bios/zfont2.h
===================================================================
--- trunk/bios/zfont2.h (nonexistent)
+++ trunk/bios/zfont2.h (revision 2)
@@ -0,0 +1,625 @@
+/* GIMP header image file format (INDEXED): /Users/charlie/code/verilog/Z2/zfont2.h */
+
+static unsigned int width = 512;
+static unsigned int height = 11;
+
+/* Call this macro repeatedly. After each use, the pixel data can be extracted */
+
+#define HEADER_PIXEL(data,pixel) {\
+pixel[0] = header_data_cmap[(unsigned char)data[0]][0]; \
+pixel[1] = header_data_cmap[(unsigned char)data[0]][1]; \
+pixel[2] = header_data_cmap[(unsigned char)data[0]][2]; \
+data ++; }
+
+static char header_data_cmap[256][3] = {
+ {255,255,255},
+ { 0, 0, 0},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255},
+ {255,255,255}
+ };
+static char header_data[] = {
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,1,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,1,0,1,0,1,0,0,1,0,1,0,0,
+ 0,0,1,0,0,0,0,1,0,1,0,0,0,0,1,0,
+ 0,0,0,0,0,0,1,0,0,0,0,1,0,1,0,0,
+ 0,0,0,1,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,1,0,0,1,
+ 1,1,0,0,1,1,0,0,0,1,1,1,0,0,1,1,
+ 1,0,0,0,1,0,0,0,1,1,1,0,0,1,1,1,
+ 0,0,1,1,1,0,0,1,1,1,0,0,1,1,1,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1,
+ 1,1,0,0,0,0,0,1,1,1,0,0,0,0,0,1,
+ 0,0,0,0,1,1,1,0,0,0,1,1,1,0,0,0,
+ 1,1,0,0,0,0,1,1,1,0,0,1,1,1,0,0,
+ 1,1,1,0,0,0,1,0,1,0,0,0,1,0,0,0,
+ 0,1,0,0,0,1,0,1,0,0,0,1,0,0,0,0,
+ 1,0,0,0,1,0,0,0,1,0,0,1,0,0,0,1,
+ 1,1,0,0,0,1,1,1,0,0,0,1,1,1,0,0,
+ 0,1,1,0,0,0,0,0,1,1,0,0,1,1,1,0,
+ 0,1,0,1,0,0,1,0,1,0,0,1,0,0,0,1,
+ 0,0,1,0,1,0,0,1,0,1,0,0,1,1,1,0,
+ 0,0,1,1,0,1,0,0,0,1,1,0,0,0,1,1,
+ 0,0,0,0,0,0,0,0,0,1,0,0,0,0,0,0,
+ 0,0,0,1,0,0,0,0,0,0,0,0,0,0,0,0,
+ 1,0,0,0,0,0,0,0,0,0,1,1,0,0,0,0,
+ 0,0,0,0,1,0,0,0,0,0,1,0,0,0,1,0,
+ 0,0,1,0,0,0,0,1,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,1,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,1,1,0,0,1,0,0,
+ 1,1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,1,0,0,0,0,0,0,1,0,1,0,0,
+ 0,1,1,1,0,0,0,0,0,1,0,0,0,1,0,1,
+ 0,0,0,0,0,0,1,0,0,0,1,0,0,0,1,0,
+ 0,0,1,1,1,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,1,0,0,1,
+ 0,1,0,0,0,1,0,0,0,1,0,1,0,0,1,0,
+ 1,0,0,0,1,0,0,0,1,0,0,0,0,0,0,1,
+ 0,0,1,0,1,0,0,1,0,1,0,0,1,0,1,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1,
+ 0,1,0,0,0,0,1,0,0,0,1,0,0,0,1,0,
+ 1,0,0,0,1,0,1,0,0,0,1,0,1,0,0,0,
+ 1,0,1,0,0,0,1,0,0,0,0,1,0,0,0,0,
+ 1,0,1,0,0,0,1,0,1,0,0,0,1,0,0,0,
+ 0,1,0,0,0,1,0,1,0,0,0,1,0,0,0,0,
+ 1,1,0,1,1,0,0,0,1,1,0,1,0,0,0,1,
+ 0,1,0,0,0,1,0,1,0,0,0,1,0,1,0,0,
+ 0,1,0,1,0,0,0,1,0,1,0,0,0,1,0,0,
+ 0,1,0,1,0,0,1,0,1,0,0,1,0,0,0,1,
+ 0,0,1,0,1,0,0,1,0,1,0,0,0,0,1,0,
+ 0,0,1,0,0,1,0,0,0,0,1,0,0,0,1,1,
+ 0,0,0,0,0,0,0,0,0,0,1,0,0,0,0,0,
+ 0,0,0,1,0,0,0,0,0,0,0,0,0,0,0,0,
+ 1,0,0,0,0,0,0,0,0,0,1,0,0,0,0,0,
+ 0,0,0,0,1,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,1,0,0,0,0,1,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,1,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,1,0,0,0,1,0,0,
+ 0,1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,1,0,0,0,0,0,1,1,1,1,1,0,
+ 0,1,1,1,0,0,0,0,1,0,0,0,0,1,0,1,
+ 0,0,0,0,0,0,0,0,0,0,1,0,0,0,1,0,
+ 0,0,1,1,1,0,0,0,0,0,1,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,1,0,0,0,1,
+ 0,1,0,0,0,1,0,0,0,0,0,1,0,0,0,0,
+ 1,0,0,0,1,0,0,0,1,1,1,0,0,1,1,1,
+ 0,0,0,0,1,0,0,1,0,1,0,0,1,0,1,0,
+ 0,0,0,0,0,0,0,0,0,0,0,1,1,0,0,0,
+ 0,0,0,0,0,0,0,1,1,0,0,0,0,0,0,0,
+ 0,1,0,0,0,1,0,1,1,1,0,1,0,0,1,0,
+ 1,0,0,0,1,0,1,0,0,0,1,0,0,0,0,0,
+ 1,0,1,0,0,0,1,0,0,0,0,1,0,0,0,0,
+ 1,0,0,0,0,0,1,0,1,0,0,0,1,0,0,0,
+ 0,1,0,0,0,1,1,0,0,0,0,1,0,0,0,0,
+ 1,1,0,1,1,0,0,0,1,1,0,1,0,0,0,1,
+ 0,1,0,0,0,1,0,1,0,0,0,1,0,1,0,0,
+ 0,1,0,1,0,0,0,1,0,0,0,0,0,1,0,0,
+ 0,1,0,1,0,0,1,0,1,0,0,1,0,1,0,1,
+ 0,0,0,1,0,0,0,1,0,1,0,0,0,1,0,0,
+ 0,0,1,0,0,0,1,0,0,0,1,0,0,1,0,0,
+ 1,0,0,0,0,0,0,0,0,0,0,0,0,1,1,1,
+ 0,0,0,1,1,1,0,0,0,1,1,1,0,0,1,1,
+ 1,0,0,0,1,1,1,0,0,1,1,1,0,0,1,1,
+ 1,0,0,0,1,1,1,0,0,0,1,0,0,0,1,0,
+ 0,0,1,0,1,0,0,1,0,0,0,1,1,1,1,1,
+ 0,0,0,1,1,1,0,0,0,1,1,1,0,0,0,1,
+ 1,1,0,0,0,1,1,1,0,0,0,1,1,0,0,1,
+ 1,1,0,0,1,1,1,0,0,1,0,1,0,0,1,0,
+ 1,0,0,1,0,1,0,1,0,1,0,1,0,1,0,1,
+ 0,0,0,1,1,1,0,0,0,1,0,0,0,1,0,0,
+ 0,1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,1,0,0,0,0,0,0,1,0,1,0,0,
+ 0,1,1,0,0,0,0,0,1,0,0,0,0,1,0,1,
+ 0,0,0,0,0,0,0,0,0,0,1,0,0,0,1,0,
+ 0,0,0,1,0,0,0,0,0,0,1,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,1,0,0,0,1,
+ 0,1,0,0,0,1,0,0,0,0,1,1,0,0,0,1,
+ 0,0,0,1,0,1,0,0,0,0,1,0,0,1,0,1,
+ 0,0,0,1,0,0,0,1,1,1,0,0,1,0,1,0,
+ 0,0,0,0,0,0,0,0,0,1,1,0,0,0,0,1,
+ 1,1,1,0,0,0,0,0,0,1,1,0,0,0,0,0,
+ 1,0,0,0,0,1,0,1,0,1,0,1,0,0,1,0,
+ 1,0,0,0,1,1,0,0,0,0,1,0,0,0,0,0,
+ 1,0,1,0,0,0,1,1,1,0,0,1,1,1,0,0,
+ 1,1,1,0,0,0,1,1,1,0,0,0,1,0,0,0,
+ 0,1,0,0,0,1,1,0,0,0,0,1,0,0,0,0,
+ 1,1,0,1,1,0,0,0,1,1,1,1,0,0,0,1,
+ 0,1,0,0,0,1,1,1,0,0,0,1,0,1,0,0,
+ 0,1,1,1,0,0,0,0,1,1,0,0,0,1,0,0,
+ 0,1,0,1,0,0,1,0,1,0,0,1,0,1,0,1,
+ 0,0,0,1,0,0,0,0,1,0,0,0,0,1,0,0,
+ 0,0,1,0,0,0,1,0,0,0,1,0,0,1,0,0,
+ 1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1,
+ 0,0,0,1,0,1,0,0,0,1,0,1,0,0,1,0,
+ 1,0,0,0,1,0,1,0,0,0,1,0,0,0,1,0,
+ 1,0,0,0,1,0,1,0,0,0,1,0,0,0,1,0,
+ 0,0,1,1,0,0,0,1,0,0,0,1,0,1,0,1,
+ 0,0,0,1,0,1,0,0,0,1,0,1,0,0,0,1,
+ 0,1,0,0,0,1,0,1,0,0,0,1,0,0,0,1,
+ 0,0,0,0,0,1,0,0,0,1,0,1,0,0,1,0,
+ 1,0,0,1,0,1,0,1,0,1,0,1,0,1,0,1,
+ 0,0,0,0,0,1,0,0,0,1,0,0,0,1,0,0,
+ 0,1,0,0,0,1,1,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,1,1,1,1,1,0,
+ 0,0,1,1,0,0,0,0,1,0,0,0,0,0,1,0,
+ 1,0,0,0,0,0,0,0,0,0,1,0,0,0,1,0,
+ 0,0,0,0,0,0,0,0,1,1,1,1,1,0,0,0,
+ 0,0,0,1,1,1,0,0,0,0,0,1,0,0,0,1,
+ 0,1,0,0,0,1,0,0,0,0,1,0,0,0,0,0,
+ 1,0,0,1,0,1,0,0,0,0,1,0,0,1,0,1,
+ 0,0,0,1,0,0,0,1,0,1,0,0,1,1,1,0,
+ 1,0,0,0,1,0,0,0,1,1,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,1,1,0,0,0,0,
+ 1,0,0,0,0,1,0,1,1,1,1,0,0,0,1,1,
+ 1,0,0,0,1,0,1,0,0,0,1,0,0,0,0,0,
+ 1,0,1,0,0,0,1,0,0,0,0,1,0,0,0,0,
+ 1,0,1,0,0,0,1,0,1,0,0,0,1,0,0,0,
+ 0,1,0,0,0,1,0,1,0,0,0,1,0,0,0,0,
+ 1,0,1,0,1,0,0,0,1,0,1,1,0,0,0,1,
+ 0,1,0,0,0,1,0,0,0,0,0,1,0,1,0,0,
+ 0,1,1,0,0,0,0,0,0,1,0,0,0,1,0,0,
+ 0,1,0,1,0,0,1,0,1,0,0,1,1,0,1,1,
+ 0,0,0,1,0,0,0,0,1,0,0,0,0,1,0,0,
+ 0,0,1,0,0,0,1,0,0,0,1,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,1,1,1,
+ 0,0,0,1,0,1,0,0,0,1,0,0,0,0,1,0,
+ 1,0,0,0,1,1,1,0,0,0,1,0,0,0,1,0,
+ 1,0,0,0,1,0,1,0,0,0,1,0,0,0,1,0,
+ 0,0,1,1,0,0,0,1,0,0,0,1,0,1,0,1,
+ 0,0,0,1,0,1,0,0,0,1,0,1,0,0,0,1,
+ 0,1,0,0,0,1,0,1,0,0,0,1,0,0,0,0,
+ 1,1,0,0,0,1,0,0,0,1,0,1,0,0,1,0,
+ 1,0,0,1,0,1,0,1,0,0,1,0,0,1,0,1,
+ 0,0,0,0,1,0,0,0,1,0,0,0,0,1,0,0,
+ 0,0,1,0,0,0,0,1,1,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,1,0,1,0,0,
+ 0,1,1,1,0,0,0,1,0,0,0,0,0,1,0,1,
+ 0,0,0,0,0,0,0,0,0,0,1,0,0,0,1,0,
+ 0,0,0,0,0,0,0,0,0,0,1,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,1,0,0,0,0,1,
+ 0,1,0,0,0,1,0,0,0,1,0,0,0,0,1,0,
+ 1,0,0,1,1,1,0,0,1,0,1,0,0,1,0,1,
+ 0,0,0,1,0,0,0,1,0,1,0,0,0,0,1,0,
+ 0,0,0,0,0,0,0,0,0,1,1,0,0,0,0,1,
+ 1,1,1,0,0,0,0,0,0,1,1,0,0,0,0,0,
+ 0,0,0,0,0,0,1,0,0,0,0,0,0,0,1,0,
+ 1,0,0,0,1,0,1,0,0,0,1,0,1,0,0,0,
+ 1,0,1,0,0,0,1,0,0,0,0,1,0,0,0,0,
+ 1,0,1,0,0,0,1,0,1,0,0,0,1,0,0,0,
+ 0,1,0,0,0,1,0,1,0,0,0,1,0,0,0,0,
+ 1,0,1,0,1,0,0,0,1,0,1,1,0,0,0,1,
+ 0,1,0,0,0,1,0,0,0,0,0,1,1,1,0,0,
+ 0,1,0,1,0,0,0,1,0,1,0,0,0,1,0,0,
+ 0,1,0,1,0,0,1,1,1,0,0,1,1,0,1,1,
+ 0,0,1,0,1,0,0,0,1,0,0,0,1,0,0,0,
+ 0,0,1,0,0,0,0,1,0,0,1,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,1,0,1,
+ 0,0,0,1,0,1,0,0,0,1,0,0,0,0,1,0,
+ 1,0,0,0,1,0,0,0,0,0,1,0,0,0,1,0,
+ 1,0,0,0,1,0,1,0,0,0,1,0,0,0,1,0,
+ 0,0,1,0,1,0,0,1,0,0,0,1,0,1,0,1,
+ 0,0,0,1,0,1,0,0,0,1,0,1,0,0,0,1,
+ 0,1,0,0,0,1,0,1,0,0,0,1,0,0,0,1,
+ 0,1,0,0,0,1,0,0,0,1,0,1,0,0,0,1,
+ 0,0,0,0,1,0,1,0,0,1,0,1,0,1,0,1,
+ 0,0,0,1,0,0,0,0,0,1,0,0,0,1,0,0,
+ 0,1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,1,0,0,0,0,0,0,1,0,1,0,0,
+ 0,1,1,1,0,0,0,1,0,1,0,0,0,1,0,1,
+ 0,0,0,0,0,0,0,0,0,0,1,0,0,0,1,0,
+ 0,0,0,0,0,0,0,0,0,0,1,0,0,0,0,0,
+ 1,0,0,0,0,0,0,0,1,0,1,0,0,0,0,1,
+ 1,1,0,0,1,1,1,0,0,1,1,1,0,0,1,1,
+ 1,0,0,0,0,1,0,0,1,1,1,0,0,1,1,1,
+ 0,0,0,1,0,0,0,1,1,1,0,0,0,0,1,0,
+ 1,0,0,0,1,0,0,0,0,0,0,1,1,0,0,0,
+ 0,0,0,0,0,0,0,1,1,0,0,0,0,0,0,0,
+ 1,0,0,0,0,0,0,1,1,1,0,0,0,0,1,0,
+ 1,0,0,0,1,1,1,0,0,0,1,1,1,0,0,0,
+ 1,1,0,0,0,0,1,1,1,0,0,1,0,0,0,0,
+ 1,1,1,0,0,0,1,0,1,0,0,0,1,0,0,1,
+ 1,1,0,0,0,1,0,1,0,0,0,1,1,1,0,0,
+ 1,0,0,0,1,0,0,0,1,0,0,1,0,0,0,1,
+ 1,1,0,0,0,1,0,0,0,0,0,1,1,1,1,0,
+ 0,1,0,1,0,0,0,1,1,1,0,0,0,1,0,0,
+ 0,1,1,1,0,0,0,1,0,0,0,1,0,0,0,1,
+ 0,0,1,0,1,0,0,0,1,0,0,0,1,1,1,0,
+ 0,0,1,0,0,0,0,1,0,0,1,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,1,1,1,
+ 0,0,0,1,1,1,0,0,0,1,1,1,0,0,1,1,
+ 1,0,0,0,1,1,1,0,0,0,1,0,0,0,1,1,
+ 1,0,0,0,1,0,1,0,0,0,1,0,0,0,1,0,
+ 0,0,1,0,1,0,0,1,1,0,0,1,0,1,0,1,
+ 0,0,0,1,0,1,0,0,0,1,1,1,0,0,0,1,
+ 1,1,0,0,0,1,1,1,0,0,0,1,0,0,0,1,
+ 1,1,0,0,0,1,1,0,0,1,1,1,0,0,0,1,
+ 0,0,0,0,1,0,1,0,0,1,0,1,0,1,1,1,
+ 0,0,0,1,1,1,0,0,0,1,0,0,0,1,0,0,
+ 0,1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,1,0,0,0,0,0,0,0,0,0,0,0,1,0,
+ 1,0,0,0,0,0,0,0,0,0,0,1,0,1,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,1,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,1,1,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,1,0,0,0,0,0,0,0,1,0,0,0,0,0,
+ 0,0,0,1,1,1,1,1,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 1,0,0,0,0,0,0,0,0,0,0,0,0,0,1,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1,
+ 0,0,0,0,0,0,0,1,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1,
+ 0,0,0,0,0,0,0,0,0,1,0,0,0,1,0,0,
+ 0,1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,1,1,0,0,0,0,0,1,1,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,1,1,
+ 1,0,0,0,0,0,0,0,0,0,0,0,0,1,1,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,1,
+ 0,0,0,0,0,0,0,1,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 0,0,0,0,0,0,0,0,0,0,0,0,0,1,1,0,
+ 0,0,0,0,0,0,0,0,0,1,1,0,0,1,0,0,
+ 1,1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,
+ 1,1,0,0,1,0,1,1,1,0,1,1,1,1,1,0,
+ 0,1,1,1,0,0,0,1,1,1,0,0,0,1,1,1,
+ 1,0,0,0,0,0,1,0,0,0,1,1,0,1,1,0,
+ 0,0,1,1,1,0,0,0,1,1,1,1,1,0,0,1,
+ 1,0,0,1,1,1,0,0,1,0,1,1,1,0,0,1,
+ 1,1,0,0,1,1,1,0,0,1,1,1,0,0,1,1,
+ 1,0,0,1,1,1,0,0,1,1,1,0,0,1,1,1,
+ 0,0,1,1,1,0,0,1,1,1,0,0,1,1,1,0,
+ 1,0,0,1,1,0,0,0,1,1,1,1,1,0,0,1,
+ 1,1,1,0,0,0,0,1,1,1,1,1,0,0,0,1,
+ 1,1,0,0,0,1,1,1,1,1,1,1,0,0,1,1,
+ 1,0,0,0,1,1,1,0,0,0,1,1,1,0,0,0,
+ 1,1,1,0,0,0,1,1,1,0,0,1,1,1,0,0,
+ 1,1,1,0,0,0,1,1,1,0,0,0,1,0,0,1,
+ 1,1,0,0,0,1,1,1,0,0,0,1,1,1,0,0,
+ 1,1,1,1,1,0,0,0,1,1,1,1,0,0,0,1,
+ 1,1,0,0,0,1,1,1,0,0,0,1,1,1,1,0,
+ 0,1,1,1,0,0,0,1,1,1,0,0,1,1,1,0,
+ 0,1,1,1,0,0,1,1,1,0,0,1,1,1,1,1,
+ 0,0,1,1,1,0,0,1,1,1,0,0,1,1,1,0,
+ 0,0,1,1,0,1,1,1,0,1,1,0,0,1,1,1,
+ 1,0,0,1,1,1,1,1,0,1,1,0,0,1,1,1,
+ 0,0,0,1,1,1,0,0,0,1,1,1,0,0,1,1,
+ 1,0,0,0,1,1,1,0,0,1,1,1,0,0,1,1,
+ 1,0,0,0,1,1,1,0,0,0,1,0,0,1,1,0,
+ 0,0,1,1,1,0,0,1,1,0,0,1,1,1,1,1,
+ 0,0,0,1,1,1,0,0,0,1,1,1,0,0,0,1,
+ 1,1,0,0,0,1,1,1,0,0,0,1,1,0,0,1,
+ 1,1,0,0,1,1,1,0,0,1,1,1,0,0,1,1,
+ 1,0,0,1,1,1,1,1,0,1,1,1,0,1,1,1,
+ 0,0,0,1,1,1,0,0,1,1,1,0,0,1,0,0,
+ 1,1,1,0,0,1,1,1,1,0,0,0,0,0,0,0
+ };
trunk/bios/zfont2.h
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/bios/touchscreeninfo.txt
===================================================================
--- trunk/bios/touchscreeninfo.txt (nonexistent)
+++ trunk/bios/touchscreeninfo.txt (revision 2)
@@ -0,0 +1,18 @@
+Touch screen
+
+SenseY=+5 or sense
+SenseX=+5 or sense
+DY=Z/GND;
+DX=Z/GND/+5;
+
+ GND
+Sense/Z
+ +5
+
+ Sense
+ +5/GND
+ Z
+
+ Sense
+ Sense/GND
+ +5
trunk/bios/touchscreeninfo.txt
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/README.md
===================================================================
--- trunk/README.md (nonexistent)
+++ trunk/README.md (revision 2)
@@ -0,0 +1,29 @@
+Z3
+==
+
+A Verilog implementation of the Infocom Z-Machine V3.
+
+Back in the very early 80s Infocom, the purveyor of very fine text based video games, when faced with the problems of porting their games to the plethora of home computers availible back in the day invented a virtual machine optimised for running interactive fiction. Then interpreters were written for all the popular computers of the day so they could all run the same code.
+
+This virtual machine was never meant to be implemented in hardware although now thanks to cheap FPGAs it can be! The Z-Machine CPU is written in Verilog and included here. So using a Cyclone II EP2C5 FPGA (~$10), a TFT LCD Arduino Shield (~$3), 512Kb 8-bit flash (~$2), 128Kb 8-bit SRAM (~$2) and a ADC ($2) you too could have a functioning Z-Machine system.
+
+Check out the following YouTube video to see it in action...
+
+[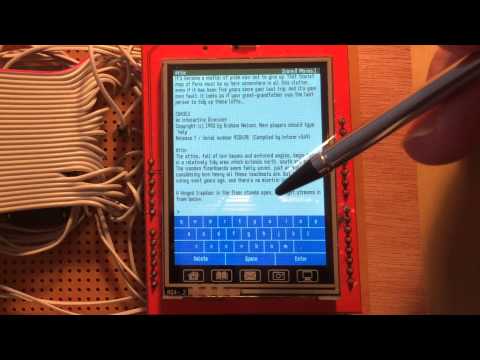](http://www.youtube.com/watch?v=HuQZq6DQQDY)
+
+The spec the Z3 follows is...
+http://inform-fiction.org/zmachine/standards/z1point0/index.html. Specifically version 3, the so called "Standard" games. These were released between 1982 and 1987 and covers most Infocom games.
+
+Z3 passes the "CZECH - Comprehensive Z-machine Emulation CHecker" by Amir Karger. And is known to run Zork I, Hitchhiker's Guide to the Galaxy, Planetfall and Curses (by Graham Nelson).
+
+To provide some input/output a terminal is implemented in a BIOS (in Z-machine code) which exists in the ROM above 120Kb. The IO related opcodes (like @print) vector into the BIOS which provides the terminal. A small number of extra op codes were added so the BIOS could interface with an LCD touchscreen.
+
+Save/Restore isn't currently supported (as my hardware hasn't any storage) and is the only notable obmission.
+
+To compare the performance of the Z-Machine to other machines from around the same time. I ported the Dhrystone benchmark (and others) to Inform (a language for writting Z-Machine code). Using this benchmark the Z3 at 10MHz is around 0.93 DMIPS which is compariable to the performance of an Atari ST running native code.
+
+The BIOS contains two easter eggs. By typing "invade" a fairly complete Space Invaders clone will start. Also "mandelbrot" draws a mandelbrot fractal.
+
+For (very brief) instructions to build, check instructions.txt.
+
+Disclaimer: This is my first Verilog project so might not be written all that well
trunk/README.md
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
Index: trunk/z3.vl
===================================================================
--- trunk/z3.vl (nonexistent)
+++ trunk/z3.vl (revision 2)
@@ -0,0 +1,1625 @@
+module boss(clk, adcDout, reset, data, waddress, we, pe, romCS, ramCS, led0, led1, led2, lcdCS, lcdRS, lcdReset, nadcCS, a17, a18);
+
+input clk;
+input adcDout;
+input reset;
+
+inout [7:0] data;
+
+output [16:0] waddress;
+output we;
+output pe;
+output romCS;
+output ramCS;
+output led0;
+output led1;
+output led2;
+output lcdCS;
+output lcdRS;
+output lcdReset;
+output nadcCS;
+output a17;
+output a18;
+
+reg [16:0] address;
+reg [7:0] dataOut;
+reg printEnable;
+reg writeEnable;
+reg rlcdRS;
+reg rlcdReset;
+reg adcCS;
+reg a17;
+reg a18;
+
+wire [16:0] waddress;
+wire we;
+wire pe;
+wire romCS;
+wire ramCS;
+wire led0;
+wire led1;
+wire led2;
+wire lcdCS;
+wire lcdRS;
+wire lcdReset;
+wire nadcCS;
+
+
+//`define HARDWARE_PRINT
+//`define REAL_HARDWARE
+
+`define CALLBACK_BASE 'h1E58B
+`define INIT_CALLBACK 'h0
+`define PRINT_CALLBACK 'h1
+`define PRINTCHAR_CALLBACK 'h2
+`define PRINTNUM_CALLBACK 'h3
+`define READ_CALLBACK 'h4
+`define STATUS_CALLBACK 'h5
+`define QUIT_CALLBACK 'h6
+`define EXCEPTION_CALLBACK 'h7
+
+`define OPER_LARGE 2'b00
+`define OPER_SMALL 2'b01
+`define OPER_VARI 2'b10
+`define OPER_OMIT 2'b11
+
+`define STATE_RESET 0
+`define STATE_FETCH_OP 1
+`define STATE_READ_TYPES 2
+`define STATE_READ_OPERS 3
+`define STATE_READ_INDIRECT 4
+`define STATE_READ_STORE 5
+`define STATE_READ_BRANCH 6
+`define STATE_DO_OP 7
+`define STATE_READ_FUNCTION 8
+`define STATE_CALL_FUNCTION 9
+`define STATE_RET_FUNCTION 10
+`define STATE_STORE_REGISTER 11
+`define STATE_PRINT 12
+`define STATE_DIVIDE 13
+`define STATE_HALT 14
+`define STATE_PRINT_CHAR 15
+
+`define OP_0 2'b00
+`define OP_1 2'b01
+`define OP_2 2'b10
+`define OP_VAR 2'b11
+
+`define OP0_RTRUE 'h0
+`define OP0_RFALSE 'h1
+`define OP0_PRINT 'h2
+`define OP0_PRINTRET 'h3
+`define OP0_NOP 'h4
+`define OP0_SAVE 'h5
+`define OP0_RESTORE 'h6
+`define OP0_RESTART 'h7
+`define OP0_RETPOP 'h8
+`define OP0_POP 'h9
+`define OP0_QUIT 'hA
+`define OP0_NEWLINE 'hB
+`define OP0_SHOWSTATUS 'hC
+`define OP0_VERIFY 'hD
+`define OP0_DYNAMIC 'hF
+
+`define OP1_JZ 'h0
+`define OP1_GETSIBLING 'h1
+`define OP1_GETCHILD 'h2
+`define OP1_GETPARENT 'h3
+`define OP1_GETPROPLEN 'h4
+`define OP1_INC 'h5
+`define OP1_DEC 'h6
+`define OP1_PRINTADDR 'h7
+`define OP1_SWITCHBANK 'h8
+`define OP1_REMOVEOBJ 'h9
+`define OP1_PRINTOBJ 'hA
+`define OP1_RET 'hB
+`define OP1_JUMP 'hC
+`define OP1_PRINTPADDR 'hD
+`define OP1_LOAD 'hE
+`define OP1_NOT 'hF
+
+`define OP2_JE 'h1
+`define OP2_JL 'h2
+`define OP2_JG 'h3
+`define OP2_DEC_CHK 'h4
+`define OP2_INC_CHK 'h5
+`define OP2_JIN 'h6
+`define OP2_TEST 'h7
+`define OP2_OR 'h8
+`define OP2_AND 'h9
+`define OP2_TESTATTR 'hA
+`define OP2_SETATTR 'hB
+`define OP2_CLEARATTR 'hC
+`define OP2_STORE 'hD
+`define OP2_INSERTOBJ 'hE
+`define OP2_LOADW 'hF
+`define OP2_LOADB 'h10
+`define OP2_GETPROP 'h11
+`define OP2_GETPROPADDR 'h12
+`define OP2_GETNEXTPROP 'h13
+`define OP2_ADD 'h14
+`define OP2_SUB 'h15
+`define OP2_MUL 'h16
+`define OP2_DIV 'h17
+`define OP2_MOD 'h18
+`define OP2_WRITEREG 'h1E
+
+`define OPVAR_CALL 'h0
+`define OPVAR_STOREW 'h1
+`define OPVAR_STOREB 'h2
+`define OPVAR_PUTPROP 'h3
+`define OPVAR_SREAD 'h4
+`define OPVAR_PRINTCHAR 'h5
+`define OPVAR_PRINTNUM 'h6
+`define OPVAR_RANDOM 'h7
+`define OPVAR_PUSH 'h8
+`define OPVAR_PULL 'h9
+`define OPVAR_GETTOUCH 'h1E
+`define OPVAR_BLIT1 'h1F
+
+`define PRINTEFFECT_FETCH 0
+`define PRINTEFFECT_FETCHAFTER 1
+`define PRINTEFFECT_RET1 2
+`define PRINTEFFECT_ABBREVRET 3
+
+reg forceStaticRead;
+reg forceDynamicRead;
+
+reg [3:0] state;
+reg [3:0] phase;
+
+reg [16:0] pc;
+reg [16:0] curPC;
+reg [15:0] globalsAddress;
+reg [15:0] objectTable;
+
+reg readHigh;
+
+reg [4:0] op;
+reg [1:0] operNum;
+reg [1:0] operTypes [4:0];
+reg [15:0] operand [3:0];
+reg [2:0] operandIdx;
+reg [13:0] branch;
+reg [7:0] store;
+reg negate;
+
+reg delayedBranch;
+reg divideAddOne;
+
+reg [15:0] returnValue;
+
+reg [6:0] opsToRead;
+reg [6:0] currentLocal;
+reg [16:0] csStack;
+reg [15:0] stackAddress;
+
+reg [16:0] temp;
+reg [15:0] random;
+
+reg [1:0] alphabet;
+reg [1:0] long;
+reg nextLoadIsDynamic;
+reg lastWriteWasDraw;
+
+reg adcClk;
+reg adcDin;
+
+reg [7:0] cachedReg;
+reg [15:0] cachedValue;
+
+// xp<=adcConfig[0]?0:'bZ;
+// yp<=adcConfig[1]?1:'bZ;
+// xm<=adcConfig[2]?1:'bZ;
+// ym<=adcConfig[3]?0:'bZ;
+// XP dataOut[6]
+// XM lcsRS
+// YP dataOut[7]
+// YM lcdWR
+
+initial
+begin
+ state=`STATE_RESET;
+ forceDynamicRead=0;
+ forceStaticRead=0;
+ writeEnable=0;
+ printEnable=0;
+ phase=0;
+ readHigh=0;
+ nextLoadIsDynamic=0;
+ adcCS=0;
+ a17=0;
+ a18=0;
+ cachedReg=0;
+ cachedValue=0;
+ lastWriteWasDraw=0;
+end
+
+assign waddress[16:2] = address[16:2];
+assign waddress[0] = adcCS?adcClk:address[0];
+assign waddress[1] = adcCS?adcDin:address[1];
+assign data[5:0] = (writeEnable || printEnable)?dataOut[5:0]:6'bZ;
+assign data[6] = (writeEnable || printEnable)?dataOut[6]:((adcCS && operand[0][0])?0:'bZ);
+assign data[7] = (writeEnable || printEnable)?dataOut[7]:((adcCS && operand[0][1])?1:'bZ);
+assign romCS = !(!adcCS && !printEnable && !writeEnable && !forceDynamicRead && (forceStaticRead || address[16]==1));
+assign ramCS = !(!adcCS && !printEnable && (writeEnable || forceDynamicRead || (!forceStaticRead && address[16]==0)));
+assign pe = adcCS?(operand[0][3]?0:'bZ):(!printEnable || !clk);
+assign we = !writeEnable;
+assign led0 = !address[12];
+assign led1 = !address[13];
+assign led2 = !address[14];
+assign lcdCS = !printEnable || !clk;
+assign lcdRS = adcCS?(operand[0][2]?1:'bZ):rlcdRS;
+assign lcdReset = rlcdReset;
+assign nadcCS = !adcCS;
+
+task StoreB;
+ begin
+ if (phase==0) begin
+ cachedReg<=15; nextLoadIsDynamic<=0; address<=operand[0]+operand[1]; writeEnable<=1; dataOut<=operand[2][7:0]; phase<=phase+1;
+ end else begin
+ address<=pc; writeEnable<=0; state<=`STATE_FETCH_OP;
+ end
+ end
+endtask
+
+task StoreW;
+ begin
+ case (phase)
+ 0: begin cachedReg<=15; nextLoadIsDynamic<=0; address<=operand[0]+operand[1]*2; writeEnable<=1; dataOut<=operand[2][15:8]; phase<=phase+1; end
+ 1: begin address<=address+1; dataOut<=operand[2][7:0]; phase<=phase+1; end
+ default: begin address<=pc; writeEnable<=0; state<=`STATE_FETCH_OP; end
+ endcase
+ end
+endtask
+
+task StoreRegisterAndBranch;
+ input [7:0] regNum;
+ input [15:0] value;
+ input doBranch;
+ begin
+ state<=`STATE_STORE_REGISTER;
+ store<=regNum;
+ returnValue<=value;
+ delayedBranch<=doBranch;
+ phase<=0;
+ end
+endtask
+
+task StoreResultAndBranch;
+ input [15:0] result;
+ input doBranch;
+ begin
+ if (store>=16) begin
+ address<=globalsAddress+2*(store-16);
+ end else if (store==0) begin
+ address<=2*stackAddress;
+ stackAddress<=stackAddress+1;
+ end else begin
+ address<=csStack+8+2*(store-1);
+ end
+ dataOut<=result[15:8];
+ writeEnable<=1;
+ state<=`STATE_STORE_REGISTER;
+ returnValue[7:0]<=result[7:0];
+ delayedBranch<=doBranch;
+ phase<=1;
+ end
+endtask
+
+task StoreResult;
+ input [15:0] result;
+ begin
+ StoreResultAndBranch(result, negate); // negate means won't branch
+ end
+endtask
+
+task StoreResultSlow;
+ input [15:0] result;
+ begin
+ StoreRegisterAndBranch(store, result, negate); // negate means won't branch
+ end
+endtask
+
+task ReturnFunction;
+ input [15:0] value;
+ begin
+ returnValue<=value;
+ phase<=0;
+ state<=`STATE_RET_FUNCTION;
+ end
+endtask
+
+task CallFunction;
+ input doubleReturn;
+ input noStoreOnReturn;
+ begin
+ negate<=doubleReturn;
+ delayedBranch<=noStoreOnReturn;
+ phase<=0;
+ state<=`STATE_CALL_FUNCTION;
+ end
+endtask
+
+task DoBranch;
+ input result;
+ begin
+ if ((!result)==negate) begin
+ if (branch==0) begin
+ ReturnFunction(0);
+ end else if (branch==1) begin
+ ReturnFunction(1);
+ end else begin
+ pc<=$signed(pc)+$signed(branch)-2;
+ address<=$signed(pc)+$signed(branch)-2;
+ state<=`STATE_FETCH_OP;
+ end
+ end else begin
+ address<=pc;
+ state<=`STATE_FETCH_OP;
+ end
+ end
+endtask
+
+task LoadAndStore;
+ input [16:0] loadAddress;
+ input word;
+ begin
+ if (phase==0) begin
+ store<=data;
+ forceDynamicRead<=nextLoadIsDynamic;
+ nextLoadIsDynamic<=0;
+ address<=loadAddress;
+ temp[7:0]<=0;
+ phase<=word?phase+1:2;
+ end else if (phase==1) begin
+ temp[7:0]<=data;
+ address<=address+1;
+ phase<=phase+1;
+ end else begin
+ forceDynamicRead<=0;
+ StoreResultSlow((temp[7:0]<<8)|data);
+ end
+ end
+endtask
+
+function [16:0] GetObjectAddr;
+ input [7:0] object;
+ begin
+ GetObjectAddr=objectTable+2*31+9*(object-1);
+ end
+endfunction
+
+task TestAttr;
+ begin
+ if (phase==0) begin
+ address<=GetObjectAddr(operand[0])+(operand[1]/8); phase<=phase+1;
+ end else begin
+ DoBranch(data[7-(operand[1]&7)]);
+ end
+ end
+endtask
+
+task SetAttr;
+ begin
+ case (phase)
+ 0: begin address<=GetObjectAddr(operand[0])+(operand[1]/8); phase<=phase+1; end
+ 1: begin dataOut<=data|(1<<(7-(operand[1]&7))); writeEnable<=1; phase<=phase+1; end
+ default: begin writeEnable<=0; address<=pc; state=`STATE_FETCH_OP; end
+ endcase
+ end
+endtask
+
+task ClearAttr;
+ begin
+ case (phase)
+ 0: begin address<=GetObjectAddr(operand[0])+(operand[1]/8); phase<=phase+1; end
+ 1: begin dataOut<=data&(~(1<<(7-(operand[1]&7)))); writeEnable<=1; phase<=phase+1; end
+ default: begin writeEnable<=0; address<=pc; state=`STATE_FETCH_OP; end
+ endcase
+ end
+endtask
+
+task JumpIfParent;
+ begin
+ if (phase==0) begin
+ address<=GetObjectAddr(operand[0])+4; phase<=phase+1;
+ end else begin
+ DoBranch(operand[1]==data);
+ end
+ end
+endtask
+
+task GetRelative;
+ input [1:0] offset;
+ input branch;
+ begin
+ if (phase==0) begin
+ address<=GetObjectAddr(operand[0])+4+offset; phase<=phase+1;
+ end else begin
+ StoreResultAndBranch(data, branch?data!=0:negate);
+ end
+ end
+endtask
+
+task FindProp;
+ begin
+ case (phase[2:0])
+ 0: begin store<=data; address<=GetObjectAddr(operand[0])+7; phase<=phase+1; end
+ 1: begin temp[16]<=0; temp[15:8]<=data; temp[7:0]<=0; address<=address+1; phase<=phase+1; end
+ 2: begin address<=temp|data; phase<=phase+1; end
+ 3: begin address<=address+2*data+1; phase<=phase+1; end
+ 4: begin
+ if (data==0) begin // end of search (get default)
+ address<=objectTable+2*(operand[1]-1);
+ phase<=phase+1;
+ end else if (data[4:0]==operand[1]) begin // found property
+ address<=address+1;
+ if (data[7:5]==0) // only 1 byte
+ phase<=6;
+ else
+ phase<=5;
+ end else begin // skip over data
+ address<=address+data[7:5]+2;
+ end
+ end
+ 5: begin temp[7:0]<=data; address<=address+1; phase<=phase+1; end
+ default: begin StoreResultSlow((temp[7:0]<<8)|data); end
+ endcase
+ end
+endtask
+
+task SetProp;
+ begin
+ case (phase[2:0])
+ 0: begin address<=GetObjectAddr(operand[0])+7; phase<=phase+1; end
+ 1: begin temp[16]<=0; temp[15:8]<=data; temp[7:0]<=0; address<=address+1; phase<=phase+1; end
+ 2: begin address<=temp|data; phase<=phase+1; end
+ 3: begin address<=address+2*data+1; phase<=phase+1; end
+ 4: begin
+`ifndef REAL_HARDWARE
+ if (data==0) begin // end of search
+ state<=`STATE_HALT;
+ end else
+`endif
+ if (data[4:0]==operand[1]) begin // found property
+ if (data[7:5]==0) begin // only 1 byte
+ dataOut<=operand[2][7:0];
+ phase<=6;
+ address<=address+1;
+ writeEnable<=1;
+ end else begin
+ dataOut<=operand[2][15:8];
+ phase<=5;
+ address<=address+1;
+ writeEnable<=1;
+ end
+ end else begin // skip over data
+ address<=address+data[7:5]+2;
+ end
+ end
+ 5: begin dataOut<=operand[2][7:0]; address<=address+1; phase<=phase+1; end
+ default: begin state<=`STATE_FETCH_OP; address<=pc; writeEnable<=0; end
+ endcase
+ end
+endtask
+
+task FindPropAddrLen;
+ begin
+ case (phase[2:0])
+ 0: begin store<=data; address<=GetObjectAddr(operand[0])+7; phase<=phase+1; end
+ 1: begin temp[16]<=0; temp[15:8]<=data; temp[7:0]<=0; address<=address+1; phase<=phase+1; end
+ 2: begin address<=temp|data; phase<=phase+1; end
+ 3: begin address<=address+2*data+1; phase<=phase+1; end
+ default: begin
+ if (data==0) begin // end of search
+ StoreResultSlow(0);
+ end else if (data[4:0]==operand[1]) begin // found property
+ StoreResultSlow(address+1);
+ end else begin // skip over data
+ address<=address+data[7:5]+2;
+ end
+ end
+ endcase
+ end
+endtask
+
+task FindNextProp;
+ begin
+ case (phase[2:0])
+ 0: begin store<=data; address<=GetObjectAddr(operand[0])+7; phase<=phase+1; end
+ 1: begin temp[16]<=0; temp[15:8]<=data; temp[7:0]<=0; address<=address+1; phase<=phase+1; end
+ 2: begin address<=temp|data; phase<=phase+1; end
+ 3: begin address<=address+2*data+1; phase<=phase+1; end
+ default: begin
+ if (operand[1]==0)
+ StoreResultSlow(data[4:0]);
+`ifndef REAL_HARDWARE
+ else if (data==0) // end of search
+ state<=`STATE_HALT;
+`endif
+ else begin // skip over data
+ if (data[4:0]==operand[1])
+ operand[1]<=0;
+ address<=address+data[7:5]+2;
+ end
+ end
+ endcase
+ end
+endtask
+
+task GetPropLen;
+ begin
+ if (phase==0) begin
+ address<=operand[0]-1; phase<=phase+1;
+ end else begin
+ StoreResultSlow((operand[0]==0)?0:(data[7:5]+1));
+ end
+ end
+endtask
+
+task Pull;
+ input return;
+ begin
+ case (phase)
+ 0: begin
+ address<=2*(stackAddress-1);
+ forceDynamicRead<=1;
+ phase<=phase+1;
+ end
+ 1: begin
+ temp[7:0]<=data;
+ stackAddress<=address>>1;
+ address<=address+1;
+ phase<=phase+1;
+ end
+ default: begin
+ forceDynamicRead<=0;
+ if (return) begin
+ ReturnFunction((temp[7:0]<<8)|data);
+ end else begin
+ if (operand[0]==0)
+ stackAddress<=stackAddress-1;
+ StoreRegisterAndBranch(operand[0], (temp[7:0]<<8)|data, negate);
+ end
+ end
+ endcase
+
+ end
+endtask
+
+task Print;
+ input [16:0] addr;
+ input [1:0] effect;
+ begin
+ temp<=addr;
+ state<=`STATE_PRINT;
+ returnValue[1:0]<=effect;
+ delayedBranch<=0;
+ phase<=0;
+ end
+endtask
+
+task PrintObj;
+ begin
+ case (phase[1:0])
+ 0: begin address<=GetObjectAddr(operand[0])+7; phase<=phase+1; end
+ 1: begin store<=data; address<=address+1; phase<=phase+1; end
+ default: Print((store<<8)+data+1, `PRINTEFFECT_FETCH);
+ endcase
+ end
+endtask
+
+task RemoveObject;
+ begin
+ case (phase[3:0])
+ 0: begin address<=GetObjectAddr(operand[0])+4; /*obj.parent*/ phase<=phase+1; end
+ 1: begin
+ if (data==0) begin
+ phase<=(operand[1]!=0)?6:9;
+ end else begin
+ phase<=phase+1;
+ end
+ temp[15:8]<=data;
+ writeEnable<=1;
+ dataOut<=operand[1];
+ end
+ 2: begin writeEnable<=0; address<=address+1; /*obj.sibling*/ phase<=phase+1; end
+ 3: begin temp[7:0]<=data; writeEnable<=1; dataOut<=0; phase<=phase+1; end
+ 4: begin writeEnable<=0; address<=GetObjectAddr(temp[15:8])+6; /*parent.child*/ phase<=phase+1; end
+ 5: begin
+ if (data==operand[0]) begin // found object
+ dataOut<=temp[7:0];
+ writeEnable<=1;
+ phase<=(operand[1]!=0)?phase+1:9;
+ end else begin
+ address<=GetObjectAddr(data)+5; /*follow sibling*/
+ end
+ end
+
+ 6: begin address<=GetObjectAddr(operand[1])+6; writeEnable<=0; phase<=phase+1; end
+ 7: begin temp[7:0]<=data; writeEnable<=1; dataOut<=operand[0]; phase<=phase+1; end
+ 8: begin address<=GetObjectAddr(operand[0])+5; dataOut<=temp[7:0]; phase<=phase+1; end
+ default: begin
+ writeEnable<=0;
+ state<=`STATE_FETCH_OP;
+ address<=pc;
+ end
+ endcase
+ end
+endtask
+
+task Random;
+ begin
+ case (phase)
+ 0: begin if ($signed(operand[0])<0) begin random<=operand[0]; state<=`STATE_FETCH_OP; end else begin random<=random^(random<<13); end phase<=phase+1; end
+ 1: begin random<=random^(random>>9); phase<=phase+1; end
+ 2: begin random<=random^(random<<7); phase<=phase+1; end
+ default: begin state<=`STATE_DIVIDE; delayedBranch<=1; divideAddOne<=1; operand[1]<=operand[0]; operand[0]<=random&'h7FFF; phase<=0; end
+ endcase
+ end
+endtask
+
+task PrintNum;
+ begin
+`ifdef HARDWARE_PRINT
+ case (phase)
+ 0: begin negate<=0; operand[1]<=1; operand[2][2:0]<=2; operand[3][3:0]<=0; phase<=phase+1; end
+ 1,2,3,4: begin operand[1]<=(operand[1]<<3)+(operand[1]<<1); printEnable<=0; phase<=phase+1; end
+ 5: begin
+ if (operand[0]>=operand[1]) begin
+ operand[0]<=operand[0]-operand[1];
+ operand[3][3:0]<=operand[3][3:0]+1;
+ negate<=1;
+ printEnable<=0;
+ end else begin
+ printEnable<=negate;
+ dataOut<=operand[3][3:0]+48;
+ operand[3][3:0]<=0;
+ operand[1]<=1;
+ operand[2][2:0]<=operand[2][2:0]+1;
+ phase<=operand[2][2:0];
+ end
+ end
+ default: begin printEnable<=0; state<=`STATE_FETCH_OP; end
+ endcase
+`else
+ operand[0]<=`PRINTNUM_CALLBACK;
+ operand[1]<=operand[0];
+ operTypes[1]<=`OPER_LARGE;
+ CallFunction(0, 1);
+`endif
+ end
+endtask
+
+task PrintChar;
+ input [7:0] char;
+ begin
+ $display("Print char: %d\n", char);
+`ifdef HARDWARE_PRINT
+ printEnable<=1;
+ dataOut<=char;
+ state<=`STATE_PRINT_CHAR;
+`else
+ operand[0]<=`PRINTCHAR_CALLBACK;
+ operand[1]<=char;
+ operTypes[1]<=`OPER_LARGE;
+ CallFunction(0, 1);
+`endif
+ end
+endtask
+
+task SRead;
+ begin
+ operand[0]<=`READ_CALLBACK;
+ operand[2]<=operand[0]; // Am swapping arguments just to save space on FPGA
+ operTypes[2]<=`OPER_LARGE;
+ CallFunction(0, 1);
+ end
+endtask
+
+task WriteReg;
+ begin
+ case (phase)
+ 0: begin lastWriteWasDraw<=(operand[0]=='h22); if (operand[0]=='h22 && lastWriteWasDraw) begin rlcdRS<=1; phase<=4; end else begin rlcdRS<=0; phase<=phase+1; end end
+ 1: begin dataOut<=operand[0][15:8]; printEnable<=1; phase<=phase+1; end
+ 2: begin dataOut<=operand[0][7:0]; if (operand[0]==0) phase<=4; else phase<=phase+1; end
+ 3: begin rlcdRS<=1; printEnable<=0; phase<=phase+1; end
+ 4: begin dataOut<=operand[1][15:8]; printEnable<=1; phase<=phase+1; end
+ 5: begin dataOut<=operand[1][7:0]; phase<=phase+1; end
+ default: begin printEnable<=0; address<=pc; state<=`STATE_FETCH_OP; end
+ endcase
+ end
+endtask
+
+task Blit1;
+ begin
+ case (phase)
+ 0: begin forceDynamicRead<=1; rlcdRS<=0; phase<=phase+1; end
+ 1: begin dataOut<=0; printEnable<=1; phase<=phase+1; end
+ 2: begin address<=operand[0]*2; dataOut<=34; returnValue<=0; phase<=phase+1; end
+ 3: begin
+ rlcdRS<=1;
+ printEnable<=0;
+ if (operand[1]==0) begin
+ forceDynamicRead<=0;
+ address<=pc;
+ state<=`STATE_FETCH_OP;
+ end
+ phase<=phase+1;
+ end
+ 4: begin
+ store<=data;
+ dataOut<=data[7]?operand[3][15:8]:operand[2][15:8];
+ printEnable<=1;
+ phase<=6;
+ end
+ 5: begin
+ dataOut<=store[7]?operand[3][15:8]:operand[2][15:8];
+ printEnable<=1;
+ phase<=phase+1;
+ end
+ default: begin
+ dataOut<=store[7]?operand[3][7:0]:operand[2][7:0];
+ store[7:1]=store[6:0];
+ returnValue<=returnValue+1;
+ if (returnValue[2:0]==7) begin
+ operand[1]=operand[1]-1;
+ phase<=3;
+ address<=address+1;
+ end else begin
+ phase<=5;
+ end
+ end
+ endcase
+ end
+endtask
+
+task GetTouch;
+ begin
+ case (phase)
+ 0: begin
+ adcCS<=1;
+ adcClk<=0;
+ adcDin<=1;
+ operand[1]<='hffff;
+ operand[2]<=1;
+ phase<=1;
+ end
+ 1: begin
+ operand[2][3:0]<=operand[2][3:0]+1;
+ if (operand[2][3:0]==0) begin // Max clock rate for ADC is ~ 1MHz so divide our clock by 16
+ adcClk<=1;
+ operand[1]<=(operand[1]<<1)+adcDout;
+ phase<=(!operand[1][9])?3:2;
+ end
+ end
+ 2: begin
+ operand[2][3:0]<=operand[2][3:0]+1;
+ if (operand[2][3:0]==0) begin // Max clock rate for ADC is ~ 1MHz so divide our clock by 16
+ operand[0][8:4]<=(operand[0][8:4]>>1);
+ adcDin<=operand[0][4];
+ adcClk<=0;
+ phase<=1;
+ end
+ end
+ default: begin adcCS<=0; StoreResultSlow(operand[1]&'h3FF); end
+ endcase
+ end
+endtask
+
+task DoOp;
+ begin
+ case (operNum)
+ `OP_0: begin
+ if (phase==0)
+ $display("PC:%h Doing op0:%d Store:%h Branch:%h/%d", curPC, op, operand[0], store, branch, negate);
+ case (op[3:0])
+ `OP0_RTRUE: ReturnFunction(1);
+ `OP0_RFALSE: ReturnFunction(0);
+ `OP0_PRINT: Print(pc, `PRINTEFFECT_FETCHAFTER);
+ `OP0_PRINTRET: Print(pc, `PRINTEFFECT_RET1);
+ `OP0_SAVE,`OP0_RESTORE: DoBranch(0);
+ `OP0_RESTART: state<=`STATE_RESET;
+ `OP0_RETPOP: Pull(1);
+ `OP0_QUIT: begin operand[0]<=`QUIT_CALLBACK; CallFunction(0,1); end
+ `OP0_NEWLINE: PrintChar(10);
+ `OP0_SHOWSTATUS: begin operand[0]<=`STATUS_CALLBACK; CallFunction(0,1); end
+ `OP0_VERIFY: DoBranch(1);
+ default: state<=`STATE_HALT;
+ endcase
+ end
+ `OP_1: begin
+ if (phase==0)
+ $display("PC:%h Doing op1:%d Operands:%h Store:%h Branch:%h/%d", curPC, op, operand[0], store, branch, negate);
+ case (op[3:0])
+ `OP1_JZ: DoBranch(operand[0]==0);
+ `OP1_GETSIBLING: GetRelative(1,1);
+ `OP1_GETCHILD: GetRelative(2,1);
+ `OP1_GETPARENT: GetRelative(0,0);
+ `OP1_GETPROPLEN: GetPropLen();
+ `OP1_INC: StoreResult(operand[0]+1);
+ `OP1_DEC: StoreResult(operand[0]-1);
+ `OP1_PRINTADDR: Print(operand[0], `PRINTEFFECT_FETCH);
+ `OP1_SWITCHBANK: begin a17<=operand[0][0]; a18<=operand[0][1]; state<=`STATE_RESET; end
+ `OP1_REMOVEOBJ: begin operNum<=`OP_2; op<=`OP2_INSERTOBJ; operand[1]<=0; end
+ `OP1_PRINTOBJ: PrintObj();
+ `OP1_RET: ReturnFunction(operand[0]);
+ `OP1_JUMP: begin pc<=$signed(pc)+$signed(operand[0])-2; address<=$signed(pc)+$signed(operand[0])-2; state<=`STATE_FETCH_OP; end
+ `OP1_PRINTPADDR: Print(2*operand[0], `PRINTEFFECT_FETCH);
+ `OP1_LOAD: StoreResult(operand[0]);
+ `OP1_NOT: StoreResult(~operand[0]);
+ default: state<=`STATE_HALT;
+ endcase
+ end
+ `OP_2: begin
+ if (phase==0)
+ $display("PC:%h Doing op2:%d Operands:%h %h Store:%h Branch:%h/%d/%h", curPC, op, operand[0], operand[1], store, branch, negate, $signed(pc)+$signed(branch-2));
+ case (op)
+ `OP2_JE: DoBranch(operand[0]==operand[1] || (operTypes[2]!=`OPER_OMIT && operand[0]==operand[2]) || (operTypes[3]!=`OPER_OMIT && operand[0]==operand[3]));
+ `OP2_JL: DoBranch($signed(operand[0])<$signed(operand[1]));
+ `OP2_JG: DoBranch($signed(operand[0])>$signed(operand[1]));
+ `OP2_INC_CHK: StoreResultAndBranch(operand[0]+1, $signed(operand[0])+1>$signed(operand[1]));
+ `OP2_DEC_CHK: StoreResultAndBranch(operand[0]-1, $signed(operand[0])-1<$signed(operand[1]));
+ `OP2_JIN: JumpIfParent();
+ `OP2_TEST: DoBranch((operand[0]&operand[1])==operand[1]);
+ `OP2_OR: StoreResult(operand[0]|operand[1]);
+ `OP2_AND: StoreResult(operand[0]&operand[1]);
+ `OP2_TESTATTR: TestAttr();
+ `OP2_SETATTR: SetAttr();
+ `OP2_CLEARATTR: ClearAttr();
+ `OP2_STORE: begin if (operand[0]==0) stackAddress<=stackAddress-1; StoreRegisterAndBranch(operand[0], operand[1], negate); end
+ `OP2_INSERTOBJ: RemoveObject();
+ `OP2_LOADW: LoadAndStore(operand[0]+2*operand[1], 1);
+ `OP2_LOADB: LoadAndStore(operand[0]+operand[1], 0);
+ `OP2_GETPROP: FindProp();
+ `OP2_GETPROPADDR: FindPropAddrLen();
+ `OP2_GETNEXTPROP: FindNextProp();
+ `OP2_ADD: StoreResult($signed(operand[0])+$signed(operand[1]));
+ `OP2_SUB: StoreResult($signed(operand[0])-$signed(operand[1]));
+ `OP2_MUL: StoreResult($signed(operand[0])*$signed(operand[1]));
+ `OP2_DIV: begin store<=data; state=`STATE_DIVIDE; delayedBranch<=0; divideAddOne<=0; end
+ `OP2_MOD: begin store<=data; state=`STATE_DIVIDE; delayedBranch<=1; divideAddOne<=0; end
+ `OP2_WRITEREG: WriteReg();
+ default: state<=`STATE_HALT;
+ endcase
+ end
+ default: begin // 'OP_VAR
+ if (phase==0) begin
+ if (operTypes[0]==`OPER_OMIT)
+ $display("PC:%h Doing opvar:%d Operands: Store:%h Branch:%h/%d/%h", curPC, op, store, branch, negate, $signed(pc)+$signed(branch-2));
+ else if (operTypes[1]==`OPER_OMIT)
+ $display("PC:%h Doing opvar:%d Operands:%h Store:%h Branch:%h/%d/%h", curPC, op, operand[0], store, branch, negate, $signed(pc)+$signed(branch-2));
+ else if (operTypes[2]==`OPER_OMIT)
+ $display("PC:%h Doing opvar:%d Operands:%h %h Store:%h Branch:%h/%d/%h", curPC, op, operand[0], operand[1], store, branch, negate, $signed(pc)+$signed(branch-2));
+ else if (operTypes[3]==`OPER_OMIT)
+ $display("PC:%h Doing opvar:%d Operands:%h %h %h Store:%h Branch:%h/%d/%h", curPC, op, operand[0], operand[1], operand[2], store, branch, negate, $signed(pc)+$signed(branch-2));
+ else
+ $display("PC:%h Doing opvar:%d Operands:%h %h %h %h Store:%h Branch:%h/%d/%h", curPC, op, operand[0], operand[1], operand[2], operand[3], store, branch, negate, $signed(pc)+$signed(branch-2));
+ end
+ case (op)
+ `OPVAR_CALL: if (operand[0]==0) StoreResultSlow(0); else CallFunction(0, 0);
+ `OPVAR_STOREW: StoreW();
+ `OPVAR_STOREB: StoreB();
+ `OPVAR_PUTPROP: SetProp();
+ `OPVAR_SREAD: SRead();
+ `OPVAR_PRINTCHAR: PrintChar(operand[0]);
+ `OPVAR_PRINTNUM: PrintNum();
+ `OPVAR_RANDOM: Random();
+ `OPVAR_PUSH: StoreRegisterAndBranch(0, operand[0], negate);
+ `OPVAR_PULL: Pull(0);
+ `OPVAR_GETTOUCH: GetTouch();
+ `OPVAR_BLIT1: Blit1();
+ default: state<=`STATE_HALT;
+ endcase
+ end
+ endcase
+ end
+endtask
+
+`ifdef HARDWARE_PRINT
+task DoPrint;
+ input [4:0] char;
+ begin
+ if (delayedBranch) begin
+ store[4:0]<=char;
+ operand[1]<=phase+1;
+ delayedBranch<=0;
+ phase<=6;
+ end else if (long==1) begin
+ operand[3][4:0]=char;
+ long<=long+1;
+ end else if (long==2) begin
+ printEnable<=1;
+ dataOut<=(operand[3][4:0]<<5)|char;
+ long<=0;
+ end else begin
+ phase<=phase+1;
+ if (char==0) begin
+ printEnable<=1;
+ dataOut<=32;
+ end else if (char==4 || char==5) begin
+ printEnable<=0;
+ alphabet=char-3;
+ end else if (char>=6) begin
+ printEnable<=!(alphabet==2 && char==6);
+ if (alphabet==2) begin
+ case (char)
+ 6: long<=1;
+ 7: dataOut<=10;
+ 8,9,10,11,12,13,14,15,16,17: dataOut<=char-8+48;
+ 18: dataOut<=46;
+ 19: dataOut<=44;
+ 20: dataOut<=33;
+ 21: dataOut<=63;
+ 22: dataOut<=95;
+ 23: dataOut<=35;
+ 24: dataOut<=39;
+ 25: dataOut<=34;
+ 26: dataOut<=47;
+ 27: dataOut<=92;
+ 28: dataOut<=45;
+ 29: dataOut<=58;
+ 30: dataOut<=40;
+ 31: dataOut<=41;
+ endcase
+ end else begin
+ dataOut<=char-6+((alphabet==0)?97:65);
+ end
+ alphabet<=0;
+ end else begin
+ printEnable<=0;
+ store[7:5]<=(char-1);
+ delayedBranch<=1;
+ end
+ end
+ end
+endtask
+`endif
+
+task FinishReadingOps;
+begin
+ case (operNum)
+ `OP_1: begin
+ case (op[3:0])
+ `OP1_INC, `OP1_DEC, `OP1_LOAD: state<=`STATE_READ_INDIRECT;
+ `OP1_GETSIBLING,`OP1_GETCHILD,`OP1_GETPARENT,`OP1_GETPROPLEN,`OP1_NOT: state<=`STATE_READ_STORE;
+ `OP1_JZ: state<=`STATE_READ_BRANCH;
+ default: state<=`STATE_DO_OP;
+ endcase
+ pc<=pc+1;
+ end
+ `OP_2: begin
+ case (op[4:0])
+ `OP2_INC_CHK,`OP2_DEC_CHK: begin pc<=pc+1; state<=`STATE_READ_INDIRECT; end
+ `OP2_OR,`OP2_AND,`OP2_ADD,`OP2_SUB,`OP2_MUL: begin pc<=pc+1; state<=`STATE_READ_STORE; end
+ `OP2_LOADW,`OP2_LOADB,`OP2_GETPROP ,`OP2_GETPROPADDR,`OP2_GETNEXTPROP,`OP2_DIV,`OP2_MOD: begin pc<=pc+2; state<=`STATE_DO_OP; end
+ `OP2_JE,`OP2_JL,`OP2_JG,`OP2_JIN,`OP2_TEST,`OP2_TESTATTR: begin pc<=pc+1; state<=`STATE_READ_BRANCH; end
+ default: begin pc<=pc+1; state<=`STATE_DO_OP; end
+ endcase
+ end
+ default: begin // `OP_VAR
+ pc<=pc+1;
+ case (op[4:0])
+ `OPVAR_CALL,`OPVAR_RANDOM,`OPVAR_GETTOUCH: state<=`STATE_READ_STORE;
+ default: state<=`STATE_DO_OP;
+ endcase
+ end
+ endcase
+end
+endtask
+
+always @ (posedge clk)
+begin
+ case(state)
+ `STATE_RESET: begin
+ case (phase)
+ 0: begin printEnable<=0; random<=1; forceStaticRead<=1; address<='h6; phase<=phase+1; end
+ 1: begin address<='h7; phase<=phase+1; pc[15:8]<=data; pc[16]<=0; end
+ 2: begin address<='hA; phase<=phase+1; pc[7:0]<=data; end
+ 3: begin address<='hB; phase<=phase+1; objectTable[15:8]<=data; end
+ 4: begin address<='hC; phase<=phase+1; objectTable[7:0]<=data; end
+ 5: begin address<='hD; phase<=phase+1; globalsAddress[15:8]<=data; end
+ 6: begin address<=0; phase<=phase+1; globalsAddress[7:0]<=data; end
+ 7: begin dataOut<=data; writeEnable<=1; phase<=8; end
+ 8: begin writeEnable<=0; address<=address+1; if (address[15:0]=='hffff) phase<=9; else phase<=7; end
+ 9: begin writeEnable<=1; dataOut<=0; address<=address+1; if (address[15:0]=='hffff) begin writeEnable<=0; phase<=10; end end
+ 10: begin rlcdReset<=address[10]; rlcdRS<=1; printEnable<=0; address<=address+1; if (address[10:0]=='h7ff) phase<=11; end
+ default: begin
+ cachedReg<=15;
+ address<=pc;
+ forceStaticRead<=0;
+ stackAddress<=64*1024/2;
+ csStack<=65*1024;
+ operand[0]<=`INIT_CALLBACK;
+ operTypes[1]<=`OPER_OMIT;
+ CallFunction(0, 1);
+ end
+ endcase
+ end
+ `STATE_CALL_FUNCTION: begin
+ case (phase)
+ 0: begin $display("Call function %h", operand[0]*2); address<=csStack; writeEnable<=1; dataOut<=(delayedBranch<<2)|(negate<<1)|pc[16]; phase<=phase+1; end
+ 1: begin address<=address+1; dataOut<=pc[15:8]; phase<=phase+1; end
+ 2: begin address<=address+1; dataOut<=pc[7:0]; phase<=4; end
+ 3: begin end // pointless phase but for some reason saves gates
+ 4: begin address<=address+1; dataOut<=stackAddress[15:8]; phase<=phase+1; end
+ 5: begin address<=address+1; dataOut<=stackAddress[7:0]; phase<=phase+1; end
+ 6: begin address<=address+1; dataOut<=store; phase<=(operand[0][15:3]==0)?phase+1:10; end
+ 7: begin address<=`CALLBACK_BASE+5*(operand[0]&7); writeEnable<=0; phase<=phase+1; end
+ 8: begin address<=address+1; operand[0][15:8]<=data; phase<=phase+1; end
+ 9: begin operand[0][7:0]<=data; phase<=phase+1; end
+ default: begin
+ cachedReg<=15;
+ csStack<=csStack+38;
+ address<=2*operand[0];
+ state<=`STATE_READ_FUNCTION;
+ writeEnable<=0;
+ phase<=0;
+ end
+ endcase
+ end
+ `STATE_RET_FUNCTION: begin
+ case (phase)
+ 0: begin csStack<=csStack-38; address<=csStack-38; forceDynamicRead<=1; phase<=phase+1; end
+ 1: begin pc[16]<=data[0]; negate<=data[1]; delayedBranch<=data[2]; address<=address+1; phase<=phase+1; end
+ 2: begin pc[15:8]<=data; address<=address+1; phase<=phase+1; end
+ 3: begin pc[7:0]<=data; address<=address+1; phase<=phase+1; end
+ 4: begin stackAddress[15:8]<=data; address<=address+1; phase<=phase+1; end
+ 5: begin stackAddress[7:0]<=data; address<=address+1; phase<=phase+1; end
+ default: begin
+ forceDynamicRead<=0;
+ cachedReg<=15;
+ if (negate) begin
+ phase<=0;
+ end else if (delayedBranch) begin
+ state<=`STATE_FETCH_OP;
+ address<=pc;
+ end else begin
+ StoreRegisterAndBranch(data, returnValue, negate);
+ end
+ end
+ endcase
+ end
+ `STATE_READ_FUNCTION: begin
+ case (phase)
+ 0: begin
+ if (data==0) begin
+ state<=`STATE_FETCH_OP;
+ end else begin
+ opsToRead<=4*data-1;
+ phase<=phase+1;
+ end
+ currentLocal<=0;
+ pc<=address+1;
+ address<=address+1;
+ end
+ default: begin
+ if (opsToRead&1) begin
+ if (currentLocal<6 && operTypes[(currentLocal>>1)+1]!=`OPER_OMIT) begin
+ dataOut<=currentLocal[0]?operand[(currentLocal>>1)+1][7:0]:operand[(currentLocal>>1)+1][15:8];
+ end else begin
+ dataOut<=data;
+ end
+ address<=csStack+8+currentLocal;
+ writeEnable<=1;
+ currentLocal<=currentLocal+1;
+ end else begin
+ pc<=pc+1;
+ address<=pc+1;
+ writeEnable<=0;
+ end
+ if (opsToRead==0) begin
+ state<=`STATE_FETCH_OP;
+ end
+ opsToRead<=opsToRead-1;
+ end
+ endcase
+ end
+ `STATE_FETCH_OP: begin
+ phase<=0;
+ operTypes[2]<=`OPER_OMIT;
+ operTypes[3]<=`OPER_OMIT;
+ operTypes[4]<=`OPER_OMIT;
+ curPC<=pc;
+ $display("Fetching op at %h", pc);
+ if (!reset) begin
+ state<=`STATE_RESET;
+ a17<=0;
+ a18<=0;
+ end else begin
+ case (data[7:6])
+ 2'b10: begin
+ // short form
+ op[4:0]<=data[3:0];
+ operTypes[0]<=data[5:4];
+ operTypes[1]<=`OPER_OMIT;
+ if (data[5:4]==2'b11) begin
+ operNum[1:0]<=`OP_0;
+ case (data[3:0])
+ `OP0_POP: begin cachedReg<=15; stackAddress<=stackAddress-1; end
+ `OP0_NOP: begin /*nop*/ end
+ `OP0_DYNAMIC: nextLoadIsDynamic<=1;
+ `OP0_SAVE,`OP0_RESTORE,`OP0_VERIFY: state<=`STATE_READ_BRANCH;
+ default: state<=`STATE_DO_OP;
+ endcase
+ end else begin
+ operNum[1:0]<=`OP_1;
+ state<=`STATE_READ_OPERS;
+ end
+ end
+ 2'b11: begin
+ // variable form
+ op[4:0]<=data[4:0];
+ operNum[1:0]<=(data[5]?`OP_VAR:`OP_2);
+ state<=`STATE_READ_TYPES;
+ end
+ default: begin
+ // long form
+ op[4:0]<=data[4:0];
+ operNum[1:0]<=`OP_2;
+ operTypes[0]<=(data[6] ? `OPER_VARI : `OPER_SMALL);
+ operTypes[1]<=(data[5] ? `OPER_VARI : `OPER_SMALL);
+ state<=`STATE_READ_OPERS;
+ end
+ endcase
+ end
+ operandIdx<=0;
+ pc<=pc+1;
+ address<=pc+1;
+ end
+ `STATE_READ_TYPES: begin
+ operTypes[0]<=data[7:6];
+ operTypes[1]<=data[5:4];
+ operTypes[2]<=data[3:2];
+ operTypes[3]<=data[1:0];
+ address<=pc+1;
+ if (data[7:6]==`OPER_OMIT)
+ FinishReadingOps();
+ else begin
+ state<=`STATE_READ_OPERS;
+ pc<=pc+1;
+ end
+ end
+ `STATE_READ_OPERS: begin
+ case (operTypes[operandIdx])
+ `OPER_SMALL: begin
+ operand[operandIdx]<=data[7:0];
+ address<=pc+1;
+ operandIdx<=operandIdx+1;
+ if (operTypes[operandIdx+1]==`OPER_OMIT)
+ FinishReadingOps();
+ else
+ pc<=pc+1;
+ end
+ `OPER_LARGE: begin
+ address<=pc+1;
+ if (!readHigh) begin
+ operand[operandIdx][15:8]<=data[7:0];
+ readHigh<=1;
+ pc<=pc+1;
+ end else begin
+ operand[operandIdx][7:0]<=data[7:0];
+ operandIdx<=operandIdx+1;
+ readHigh<=0;
+ if (operTypes[operandIdx+1]==`OPER_OMIT)
+ FinishReadingOps();
+ else
+ pc<=pc+1;
+ end
+ end
+ default: begin // OPER_VARI
+ case (phase)
+ 0: begin
+ if (data==0) begin
+ stackAddress<=stackAddress-1;
+ end
+ if (cachedReg!=15 && data==cachedReg) begin
+ operand[operandIdx]<=cachedValue;
+ address<=pc+1;
+ operandIdx<=operandIdx+1;
+ if (operTypes[operandIdx+1]==`OPER_OMIT)
+ FinishReadingOps();
+ else
+ pc<=pc+1;
+ if (cachedReg==0)
+ cachedReg<=15;
+ end else begin
+ if (data>=16) begin
+ address<=globalsAddress+2*(data-16);
+ end else if (data==0) begin
+ address<=2*(stackAddress-1);
+ end else begin
+ address<=csStack+8+2*(data-1);
+ end
+ forceDynamicRead<=1;
+ phase<=phase+1;
+ end
+ end
+ 1: begin
+ operand[operandIdx]<=(data<<8);
+ address<=address+1;
+ phase<=phase+1;
+ end
+ default: begin
+ forceDynamicRead<=0;
+ operand[operandIdx]<=operand[operandIdx]|data;
+ address<=pc+1;
+ operandIdx<=operandIdx+1;
+ if (operTypes[operandIdx+1]==`OPER_OMIT)
+ FinishReadingOps();
+ else
+ pc<=pc+1;
+ phase<=0;
+ end
+ endcase
+ end
+ endcase
+ end
+ `STATE_READ_INDIRECT: begin
+ case (phase)
+ 0: begin
+ cachedReg<=15;
+ store<=operand[0];
+ if (operand[0]>=16) begin
+ address<=globalsAddress+2*(operand[0]-16);
+ end else if (operand[0]==0) begin
+ if (op[4:0]!=`OP1_LOAD)
+ stackAddress<=stackAddress-1;
+ address<=2*(stackAddress-1);
+ end else begin
+ address<=csStack+8+2*(operand[0]-1);
+ end
+ forceDynamicRead<=1;
+ phase<=phase+1;
+ end
+ 1: begin
+ operand[0][15:8]<=data;
+ address<=address+1;
+ phase<=phase+1;
+ end
+ default: begin
+ forceDynamicRead<=0;
+ operand[0][7:0]<=data;
+ if (op[4:0]==`OP1_LOAD)
+ state<=`STATE_READ_STORE;
+ else if (operNum==`OP_2)
+ state<=`STATE_READ_BRANCH;
+ else
+ state<=`STATE_DO_OP;
+ address<=pc;
+ phase<=0;
+ end
+ endcase
+ end
+ `STATE_READ_STORE: begin
+ case (op[4:0])
+ `OP1_GETSIBLING, `OP1_GETCHILD: state<=`STATE_READ_BRANCH;
+ default: state<=`STATE_DO_OP;
+ endcase
+ store<=data;
+ pc<=pc+1;
+ address<=pc+1;
+ end
+ `STATE_READ_BRANCH: begin
+ if (!readHigh) begin
+ if (data[6]) begin
+ branch<=data[5:0];
+ negate<=!data[7];
+ state<=`STATE_DO_OP;
+ end else begin
+ branch[13:8]<=data[5:0];
+ negate<=!data[7];
+ readHigh<=1;
+ end
+ end else begin
+ branch[7:0]<=data;
+ readHigh<=0;
+ state<=`STATE_DO_OP;
+ end
+ pc<=pc+1;
+ address<=pc+1;
+ end
+ `STATE_DO_OP: begin
+ DoOp();
+ end
+ `STATE_STORE_REGISTER: begin
+ case (phase)
+ 0: begin
+ if (store>=16) begin
+ address<=globalsAddress+2*(store-16);
+ end else if (store==0) begin
+ address<=2*stackAddress;
+ stackAddress<=stackAddress+1;
+ end else begin
+ address<=csStack+8+2*(store-1);
+ end
+ dataOut<=returnValue[15:8];
+ writeEnable<=1;
+ phase<=phase+1;
+ end
+ 1: begin
+ cachedReg<=store;
+ cachedValue[15:8]<=dataOut;
+ cachedValue[7:0]<=returnValue[7:0];
+ dataOut<=returnValue[7:0];
+ address<=address+1;
+ phase<=phase+1;
+ end
+ default: begin
+ DoBranch(delayedBranch);
+ writeEnable<=0;
+ phase<=0;
+ end
+ endcase
+ end
+ `STATE_DIVIDE: begin
+ case (phase)
+ 0: begin
+ negate<=operand[0][15]^operand[1][15];
+ readHigh<=operand[0][15];
+ if ($signed(operand[0])<0)
+ operand[0]<=-operand[0];
+ else
+ operand[0]<=operand[0];
+`ifndef REAL_HARDWARE
+ if (operand[1]==0)
+ state=`STATE_HALT;
+ else
+`endif
+ if ($signed(operand[1])<0)
+ operand[1]<=-operand[1];
+ operand[2]<=1;
+ operand[3]<=0;
+ phase<=phase+1;
+ end
+ 1: begin
+ if (operand[1][15] || operand[0]<=operand[1]) begin
+ phase<=phase+1;
+ end else begin
+ operand[1]<=operand[1]<<1;
+ operand[2]<=operand[2]<<1;
+ end
+ end
+ 2: begin
+ if (operand[1]>operand[0]) begin
+ if (operand[2]==1) begin
+ if (negate) begin
+ operand[0]<=-operand[3];
+ end else begin
+ operand[0]<=operand[3];
+ end
+ if (readHigh) begin
+ operand[1]<=-operand[0];
+ end else begin
+ operand[1]<=operand[0];
+ end
+ readHigh<=0;
+ phase<=phase+1;
+ end else begin
+ operand[1]<=operand[1]>>1;
+ operand[2]<=operand[2]>>1;
+ end
+ end else begin
+ operand[0]<=operand[0]-operand[1];
+ operand[3]<=operand[3]+operand[2];
+ end
+ end
+ default: begin
+ StoreResultSlow(delayedBranch?(operand[1]+divideAddOne):operand[0]);
+ end
+ endcase
+ end
+ `STATE_PRINT: begin
+`ifdef HARDWARE_PRINT
+ case (phase)
+ 0: begin delayedValue[15:8]<=data; address<=address+1; phase<=phase+1; end
+ 1: begin delayedValue[7:0] <=data; address<=address+1; phase<=phase+1; end
+ 2,3,4: begin DoPrint(delayedValue[14:10]); delayedValue[14:5]<=delayedValue[9:0]; end
+ 5: begin
+ printEnable<=0;
+ if (delayedValue[15]) begin
+ alphabet<=0;
+ long<=0;
+ case (returnValue[1:0])
+ `PRINTEFFECT_FETCH: begin
+ address<=pc;
+ state<=`STATE_FETCH_OP;
+ end
+ `PRINTEFFECT_FETCHAFTER: begin
+ pc<=address;
+ state<=`STATE_FETCH_OP;
+ end
+ `PRINTEFFECT_RET1: begin
+ ReturnFunction(1);
+ end
+ `PRINTEFFECT_ABBREVRET: begin
+ address<=temp;
+ delayedValue<=operand[0];
+ phase<=operand[1];
+ returnValue[1:0]<=returnValue[3:2];
+ end
+ endcase
+ end else begin
+ phase<=0;
+ end
+ end
+ // branch to abbrev
+ 6: begin
+ address<='h18;
+ phase<=phase+1;
+ temp<=address;
+ operand[0]<=delayedValue;
+ returnValue[3:2]=returnValue[1:0];
+ returnValue[1:0]=`PRINTEFFECT_ABBREVRET;
+ end
+ 7: begin address<=address+1; operand[2][7:0]=data; phase<=phase+1; end
+ 8: begin address<=(operand[2][7:0]<<8)|data+2*store; phase<=phase+1; end
+ 9: begin address<=address+1; operand[2][7:0]<=data; phase<=phase+1; end
+ default: begin address<=2*((operand[2][7:0]<<8)|data); phase<=0; end
+ endcase
+`else
+ if (data[7] || returnValue[1:0]!=`PRINTEFFECT_FETCHAFTER) begin
+ if (returnValue[1:0]==`PRINTEFFECT_FETCHAFTER)
+ pc<=address+2;
+ operand[0]<=`PRINT_CALLBACK;
+ $display("Print from %h\n", temp);
+ operand[1]<=temp[0]|((returnValue[1:0]==`PRINTEFFECT_RET1)?2:0); operand[2]<=temp[16:1];
+ operTypes[1]=`OPER_LARGE; operTypes[2]=`OPER_LARGE;
+ CallFunction(returnValue[1:0]==`PRINTEFFECT_RET1, 1);
+ end else begin
+ address<=address+2;
+ end
+`endif
+ end
+`ifdef HARDWARE_PRINT
+ `STATE_PRINT_CHAR: begin
+ address<=pc;
+ printEnable<=0;
+ state<=`STATE_FETCH_OP;
+ end
+`endif
+ default: begin
+ $display("HALT");
+ operand[1]<=(pc>>1);
+ operand[2]<=operand[0];
+ operand[3]<=operand[1];
+ operand[0]<=`EXCEPTION_CALLBACK;
+ //operTypes[0]<=`OPER_LARGE;
+ operTypes[1]<=`OPER_LARGE;
+ operTypes[2]<=`OPER_LARGE;
+ operTypes[3]<=`OPER_LARGE;
+ CallFunction(0,1);
+ end
+ endcase
+end
+
+endmodule
+
+module main();
+
+reg clk;
+reg adcDout;
+reg reset;
+wire [7:0] data;
+wire [16:0] address;
+wire writeEnable;
+wire printEnable;
+wire romCS;
+wire ramCS;
+wire led0;
+wire led1;
+wire led2;
+wire lcdCS;
+wire lcdRS;
+wire lcdReset;
+wire adcCS;
+wire a17;
+wire a18;
+
+integer file,readData,i,j,numOps,cycles,stateCycles[15:0],opCycles[255:0],ops[255:0];
+integer touch,touchX, touchY, touchZ;
+reg [7:0] mem [128*1024:0];
+reg [7:0] dynamicMem [128*1024:0];
+
+assign data=(!romCS ? mem[address] : (!ramCS ? (!writeEnable ? 8'bZ : dynamicMem[address] ) : 8'bZ ) );
+
+initial
+begin
+ $display("Welcome");
+ file=$fopen("rom.z3","rb");
+ readData=$fread(mem, file);
+ $display("File read:%h %d", file, readData);
+ $fclose(file);
+ touch=$fopen("touch.txt","r");
+ adcDout=0;
+ reset=1;
+ numOps=0;
+ cycles=0;
+ mem['h1E57F]=1; // Suppress game select screen as it's slow
+ for (i=0; i<16; i=i+1)
+ stateCycles[i]=0;
+ for (i=0; i<256; i=i+1) begin
+ opCycles[i]=0;
+ ops[i]=0;
+ end
+ //$monitor("%h %h %h State:%d(%d) Op:%h Num:%h OperType:%h%h%h%h OperIdx:%d Operand:%h %h Store:%h Branch:%h", clk, data, address, b.state, b.phase, b.op, b.operNum, b.operTypes[0], b.operTypes[1], b.operTypes[2], b.operTypes[3], b.operandIdx, b.operand[0], b.operand[1], b.store, b.branch);
+ for (i=0; i*505*/10000000; i=i+1) begin
+ clk=1;
+ #5 clk=0;
+ if (!ramCS && !writeEnable) begin
+ dynamicMem[address]=data;
+ end
+`ifdef HARDWARE_PRINT
+ if (!printEnable) begin
+ $display("PRINT: %c", data);
+ end
+`endif
+ if (b.state==`STATE_HALT) begin
+ $display("Halt");
+ i=10000000;
+ end
+ if (b.operNum==`OP_VAR && b.op==`OPVAR_GETTOUCH && b.phase==0 && b.state==`STATE_DO_OP) begin
+ b.phase=3;
+ if ($fscanf(touch, "%d %d %d", touchX, touchY, touchZ)==-1) begin
+ i=10000000;
+ end
+ $display("Read: %d %d %d", touchX, touchY, touchZ);
+ case (b.operand[0])
+ 'h93: b.operand[1]=touchZ;
+ 'h95: b.operand[1]=touchX;
+ 'h1A: b.operand[1]=touchY;
+ default: begin $display("Bad touch"); $finish; end
+ endcase
+ end
+ if (b.operNum==`OP_VAR && b.op==`OPVAR_BLIT1 && b.phase==0 && b.state==`STATE_DO_OP) begin
+ b.operand[1]=1;
+ end
+ //if (b.curPC<'h1e000)
+ begin
+ if (b.state==`STATE_FETCH_OP) begin
+ ops[b.op+(32*b.operNum)]=ops[b.op+(32*b.operNum)]+1;
+ numOps=numOps+1;
+ end
+ if (b.state!=`STATE_RESET) begin
+ cycles=cycles+1;
+ opCycles[b.op+(32*b.operNum)]=opCycles[b.op+(32*b.operNum)]+1;
+ end
+ stateCycles[b.state]=stateCycles[b.state]+1;
+ end
+ $display("Mem req: %h WE:%d PE:%d%d%d%d RoS:%d RaS:%d D:%h LEDs:%d%d%d ADC:%d%d%d%d%d%d%d Ops:%d/%d", address, !writeEnable, printEnable, lcdCS, lcdRS, lcdReset, !romCS, !ramCS, data, !led0, !led1, !led2, !adcCS, address[0], address[1], lcdRS, printEnable, data[7], data[6], numOps, cycles);
+ end
+ for (i=0; i<16; i=i+1)
+ if (stateCycles[i]>0)
+ $display("State:%d %d", i, stateCycles[i]);
+ for (i=0; i<256; i=i+1)
+ if (opCycles[i]>0)
+ $display("OpCyc:%d/%d %d/%d=%d", i/32, i%32, opCycles[i], ops[i], opCycles[i]/ops[i]);
+ file=$fopen("ram.dat", "wb");
+ for (i=0; i<'h20000; i=i+16) begin
+ $fwrite(file, "%05h: ", i);
+ for (j=0; j<16; j=j+2)
+ $fwrite(file, "%02x%02x ", dynamicMem[i+j][7:0], dynamicMem[i+j+1][7:0]);
+ for (j=0; j<16; j=j+1)
+ $fwrite(file, "%c", (dynamicMem[i+j][7:0]>=32)?dynamicMem[i+j][7:0]:46);
+ $fwrite(file, "\n");
+ end
+ $fwrite(file, "\nBitmap:\n");
+ for (i='h1b500; i<'h20000; i=i+30) begin
+ for (j=0; j<30; j=j+1)
+ $fwrite(file, "%d%d%d%d%d%d%d%d",
+ dynamicMem[i+j][7], dynamicMem[i+j][6],
+ dynamicMem[i+j][5], dynamicMem[i+j][4],
+ dynamicMem[i+j][3], dynamicMem[i+j][2],
+ dynamicMem[i+j][1], dynamicMem[i+j][0]);
+ $fwrite(file, "\n");
+ end
+ $fclose(file);
+ $fclose(touch);
+ $finish;
+end
+
+boss b(clk, adcDout, reset, data, address, writeEnable, printEnable, romCS, ramCS, led0, led1, led2, lcdCS, lcdRS, lcdReset, adcCS, a17, a18);
+
+endmodule
+
trunk/z3.vl
Property changes :
Added: svn:executable
## -0,0 +1 ##
+*
\ No newline at end of property
© copyright 1999-2024
OpenCores.org, equivalent to Oliscience, all rights reserved. OpenCores®, registered trademark.